Library for setting up the Parallax X-Band Motion Detector.
Dependents: Parallax_XBAND_Demo Motion_Detection_Parallax
Parallax_X-Band.h
00001 #ifndef PARALLAX_X_BAND_H 00002 #define PARALLAX_X_BAND_H 00003 #include "mbed.h" 00004 00005 /** 00006 * Wrapper for the Parallax X-Band Motion Detector 00007 */ 00008 class xband 00009 { 00010 public: 00011 00012 /** 00013 * Initialize the xband motion detector and setup interrupts on specified pins. 00014 * Default is to be disabled. 00015 * 00016 * @param pin_enable Enable pin. Connected to EN on xband. 00017 * @param pin_input Data pin. Connected to OUT on xband. 00018 */ 00019 xband(PinName pin_enable, PinName pin_input); 00020 00021 /** 00022 * Destructor 00023 */ 00024 ~xband(); 00025 00026 /** 00027 * Subroutine to enable and disable the detector. 00028 * 00029 * @param enable True to enable, False to disable. 00030 */ 00031 void enable(bool enable); 00032 00033 /** 00034 * Get count value and return it as an integer. 00035 * 00036 * @return Integer value of current count. 00037 */ 00038 int read_count(); 00039 00040 /** 00041 * Resets the count variable to 0. 00042 */ 00043 void reset_count(); 00044 00045 /** 00046 * Checks if a new velocity value has been calculated. 00047 * 00048 * @return True if a new velocity value is avaliable. False if the value has 00049 * already been read. 00050 */ 00051 bool velocityack(); 00052 00053 /** 00054 * Get current velocity reading in ticks/sec. Sets a flag to false to indicate a stale value after reading. 00055 * 00056 * @return Float value of currently measured velocity. 00057 */ 00058 float read_velocity(); 00059 00060 /** 00061 * Default overload to read velocity. 00062 * 00063 * @return Float value of currently measured velocity. 00064 */ 00065 operator float(); 00066 00067 private: 00068 /** 00069 * Interrupt service routine to collect data on the input pin. 00070 */ 00071 void xband_RiseISR(); 00072 00073 /** 00074 * Interrupt service routine to calculate the velocity of the moving object. 00075 */ 00076 void xband_velocityISR(); 00077 00078 // Setup pin input types. 00079 DigitalOut _pin_enable; 00080 InterruptIn _pin_input; 00081 00082 // Stores count value. 00083 volatile int _count; 00084 // Old count value. 00085 volatile int _lastcount; 00086 // Store calculated velocity. 00087 float _velocity; 00088 // Velocity read status flag. 00089 bool _velocityflag; 00090 00091 // Timer for differentiation 00092 Timer _sampletime; 00093 00094 // Interrupt to calculate velocity. 00095 Ticker _samplevelocity; 00096 }; 00097 00098 #endif
Generated on Wed Jul 13 2022 08:09:31 by
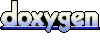