Library for setting up the Parallax X-Band Motion Detector.
Dependents: Parallax_XBAND_Demo Motion_Detection_Parallax
Parallax_X-Band.cpp
00001 #include "Parallax_X-Band.h" 00002 00003 /** 00004 * Initialize the xband motion detector and setup interrupts. Default is to be disabled. 00005 * 00006 * @param pin_enable Enable pin. Connected to EN on xband. 00007 * @param pin_input Data pin. Connected to OUT on xband. 00008 */ 00009 xband::xband(PinName pin_enable, PinName pin_input) 00010 : _pin_enable(pin_enable), _pin_input(pin_input) 00011 { 00012 // Default disabled. 00013 _pin_enable=0; 00014 00015 // Initialize count to be 0. 00016 _count = 0; 00017 00018 // No velocity reading has been calculated yet. 00019 _velocityflag = false; 00020 00021 // Setup interrupt on input pin. 00022 _pin_input.rise(this, &xband::xband_RiseISR); 00023 00024 // Timer for count differntiation. 00025 _sampletime.start(); 00026 00027 // Interrupt to calculate the velocity. Default every half second. 00028 _samplevelocity.attach(this, &xband::xband_velocityISR, 0.5); 00029 } 00030 00031 /** 00032 * Destructor 00033 */ 00034 xband::~xband() {} 00035 00036 00037 00038 /** 00039 * Subroutine to enable and disable the detector. 00040 * 00041 * @param enable True to enable, False to disable. 00042 */ 00043 void xband::enable(bool enable) 00044 { 00045 _pin_enable=enable; 00046 } 00047 00048 /** 00049 * Interrupt service routine to collect data on the input pin. 00050 */ 00051 void xband::xband_RiseISR() 00052 { 00053 // Increment count for every positive rising edge pulse on input pin. 00054 _count++; 00055 } 00056 00057 /** 00058 * Interrupt service routine to calculate the velocity of the moving object. 00059 */ 00060 void xband::xband_velocityISR() 00061 { 00062 // Differentiate current count value change during time slice. 00063 _velocity = ((_count - _lastcount)/(_sampletime.read_ms()/1000.0)); 00064 00065 // Set a flag to indicate a new velocity calculation. 00066 _velocityflag = true; 00067 00068 // Reset timer for next differentiation. 00069 _sampletime.reset(); 00070 _lastcount = _count; 00071 } 00072 00073 /** 00074 * Get count value and return it as an integer. 00075 * 00076 * @return Integer value of current count. 00077 */ 00078 int xband::read_count() 00079 { 00080 return _count; 00081 } 00082 00083 /** 00084 * Get current velocity reading in ticks/sec. Sets a flag to false to indicate a stale value after reading. 00085 * 00086 * @return Float value of currently measured velocity. 00087 */ 00088 float xband::read_velocity() 00089 { 00090 _velocityflag = false; 00091 return _velocity; 00092 } 00093 00094 /** 00095 * Resets the count variable to 0. 00096 */ 00097 void xband::reset_count() 00098 { 00099 _count = 0; 00100 } 00101 00102 /** 00103 * Checks if a new velocity value has been calculated. 00104 * 00105 * @return True if a new velocity value is avaliable. False if the value has 00106 * already been read. 00107 */ 00108 bool xband::velocityack() 00109 { 00110 return _velocityflag; 00111 } 00112 00113 /** 00114 * Default overload to read velocity. 00115 * 00116 * @return Float value of currently measured velocity. 00117 */ 00118 xband::operator float() { 00119 return read_velocity(); 00120 }
Generated on Wed Jul 13 2022 08:09:31 by
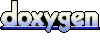