Library for VS1053 chip with non blocking call to check dreq and cancel playback function
Dependents: VS1053Player MP3_test MP3_final_S4P_ANDRIAMARO_RAKOTO_1
Fork of VS1053lib by
VS1053Codec Class Reference
This library drive a VS1053 codec to play VAW/MP3/Ogg audio file. More...
#include <VLSIcodec.h>
Public Member Functions | |
VS1053Codec (PinName mosi, PinName miso, PinName sclk, PinName cs, PinName dreq, PinName rst, PinName xdcs) | |
Initialize the SPI connection to the codec and other services pins. | |
void | init (void) |
Initialize the connection to the VS1053 codec. | |
void | testdreq (void) |
Test the dreq pin. | |
bool | checkdreq (void) |
Checks dreq and returns true if the VS1053 can take data. | |
void | reset (void) |
Reset the codec. | |
void | writedata (unsigned char data) |
write a byte to the codec | |
void | setbassboost (unsigned char bass, unsigned char gfreq) |
Set the bass boost value. | |
void | settrebleboost (char treble, unsigned int gfreq) |
Set treable boost value. | |
void | setvolume (unsigned char vRight, unsigned char vLeft) |
Set the volume. | |
void | getplaytime (char *playtime) |
Get the current decodet time. | |
void | resetplaytime () |
Clears the playtime string ready for the next file. | |
void | sinetest (unsigned char pitch) |
Start a sine test to verify codec functionality. | |
void | loadpatch (void) |
Load a VLSI patch code inside the codec. | |
void | loadoggpatch (void) |
Load a VLSI patch code to encode OGG audio file. | |
void | loadgapatch (void) |
Load a VLSI patch code that add a 23 band Graphics Analizer. | |
void | setvmeter (void) |
Start the Vmeter functionallity. | |
void | getvmeterval (unsigned char *left, unsigned char *right) |
Get vmeter values. | |
void | lowpower (void) |
Put the codec in reset for reduce power consuption. | |
void | VoggEncoding_Start (void) |
Start the encoding. | |
unsigned int | VoggEncoding_ReadBuffer (unsigned char *voggSample) |
Read the encoded samples. | |
unsigned int | VoggEncoding_Stop (unsigned char *voggSample) |
Stop the encoding and return the last buffer encoded. |
Detailed Description
This library drive a VS1053 codec to play VAW/MP3/Ogg audio file.
VS1053Codec codec( p11, p12, p13, p21, p22, p23, p24); int main( void) { codec.init(); // initialize the codec codec.loadpatch(); // load the patch code (rev 1.5) codec.setbassboost( 15, 150); // set the bass boost value codec.settrebleboost( 7, 15000); // set the treable boost value codec.setvolume( 0x0, 0x0); // set the volume // snd_piano is a Ogg file stored in flash memory. int cnt=sizeof(snd_piano)/sizeof(snd_piano[0]); // printf("Start playing file snd_piano [%d]\n\r", cnt); // int iSize=0; while( iSize < cnt) { int count=32; codec.testdreq(); while( count--) { codec.writedata( snd_piano[ iSize++]); if ( iSize > cnt) break; } } }
Definition at line 72 of file VLSIcodec.h.
Constructor & Destructor Documentation
VS1053Codec | ( | PinName | mosi, |
PinName | miso, | ||
PinName | sclk, | ||
PinName | cs, | ||
PinName | dreq, | ||
PinName | rst, | ||
PinName | xdcs | ||
) |
Initialize the SPI connection to the codec and other services pins.
- Parameters:
-
mosi,miso,sclk the SPI connection pins cs the chip select SPI pin dreq If DREQ is high, VS1053b can take at least 32 bytes of SDI data or one SCI command. DREQ is turned low when the stream buffer is too full and for the duration of a SCI command. rst hardware reset, active low xdcs the xdcs pin is the "data chip select".
Definition at line 132 of file VLSIcodec.cpp.
Member Function Documentation
bool checkdreq | ( | void | ) |
Checks dreq and returns true if the VS1053 can take data.
Crucially this call does not block allow other operations to be performed.
Definition at line 146 of file VLSIcodec.cpp.
void getplaytime | ( | char * | playtime ) |
Get the current decodet time.
- Parameters:
-
playtime a pointer to a string where the function will write the time in the format MM:SS
Definition at line 474 of file VLSIcodec.cpp.
void getvmeterval | ( | unsigned char * | left, |
unsigned char * | right | ||
) |
Get vmeter values.
Values from 0 to 31 are valid for both channels Calculates the peak sample value from both channels and returns the values in 3 dB
- Parameters:
-
left left channel value right right channel value
Definition at line 366 of file VLSIcodec.cpp.
void init | ( | void | ) |
Initialize the connection to the VS1053 codec.
Initialize the VS1053.
Never return if fail.
Definition at line 163 of file VLSIcodec.cpp.
void loadgapatch | ( | void | ) |
Load a VLSI patch code that add a 23 band Graphics Analizer.
Definition at line 265 of file VLSIcodec.cpp.
void loadoggpatch | ( | void | ) |
Load a VLSI patch code to encode OGG audio file.
This file will encode at fixed quality. Read the VLSIcodec_OGG.h for more...
Definition at line 298 of file VLSIcodec.cpp.
void loadpatch | ( | void | ) |
Load a VLSI patch code inside the codec.
The library is rev 1.5 This patch enable some features like VMeter.
Definition at line 233 of file VLSIcodec.cpp.
void lowpower | ( | void | ) |
Put the codec in reset for reduce power consuption.
Put the VS1053 in low power mode (RESET)
Re-init the codec for wake-up.
- Parameters:
-
none
- Return values:
-
none
Definition at line 155 of file VLSIcodec.cpp.
void reset | ( | void | ) |
Reset the codec.
Definition at line 630 of file VLSIcodec.cpp.
void resetplaytime | ( | ) |
Clears the playtime string ready for the next file.
Definition at line 489 of file VLSIcodec.cpp.
void setbassboost | ( | unsigned char | bass, |
unsigned char | gfreq | ||
) |
Set the bass boost value.
- Parameters:
-
bass Bass gain in dB, range from 0 to 15 freq Limit frequency for bass boost, 10 Hz steps (range from 20 to 150)
Definition at line 397 of file VLSIcodec.cpp.
void settrebleboost | ( | char | treble, |
unsigned int | gfreq | ||
) |
Set treable boost value.
- Parameters:
-
treable Bass gain in dB, range from -8 to 7 freq Limit frequency for bass boost, 1000 Hz steps (range from 1000 to 15000)
Definition at line 445 of file VLSIcodec.cpp.
void setvmeter | ( | void | ) |
Start the Vmeter functionallity.
Definition at line 357 of file VLSIcodec.cpp.
void setvolume | ( | unsigned char | vRight, |
unsigned char | vLeft | ||
) |
Set the volume.
Note, that after hardware reset the volume is set to full volume. Resetting the software does not reset the volume setting.
- Parameters:
-
right vol right channel attenuation from maximum volume, 0.5dB steps (0x00 = full volume, 0xFE= muted, 0xFF analog power down mode) left vol left channel attenuation from maximum volume, 0.5dB steps (0x00 = full volume, 0xFE= muted, 0xFF analog power down mode)
Definition at line 521 of file VLSIcodec.cpp.
void sinetest | ( | unsigned char | pitch ) |
Start a sine test to verify codec functionality.
- Parameters:
-
pitch is a composite value. Use 0x7E to play a tone at 5KHz sampled at 22050Hz. Use zero to stop to play. codec.sinetest( 0x7E); // start the sine test... wait( 1); // wait 1 sec.. codec.sinetest( 0x00); // stop the sine test...
Definition at line 527 of file VLSIcodec.cpp.
void testdreq | ( | void | ) |
Test the dreq pin.
Wait untile the dreq is released.
If high the VS1053 can take data. The function never return until the pin goes high.
- Parameters:
-
none
- Return values:
-
none
Definition at line 142 of file VLSIcodec.cpp.
unsigned int VoggEncoding_ReadBuffer | ( | unsigned char * | voggSample ) |
Read the encoded samples.
- Parameters:
-
*voggSample pointer to array to store the sample
- Returns:
- number of byte write.
Definition at line 735 of file VLSIcodec.cpp.
void VoggEncoding_Start | ( | void | ) |
Start the encoding.
Reset and then load the patch to record a audio stream.
Definition at line 667 of file VLSIcodec.cpp.
unsigned int VoggEncoding_Stop | ( | unsigned char * | voggSample ) |
Stop the encoding and return the last buffer encoded.
- Parameters:
-
*voggSample pointer to array to store the sample
- Returns:
- number of byte write.
Definition at line 782 of file VLSIcodec.cpp.
void writedata | ( | unsigned char | data ) |
Generated on Fri Jul 22 2022 21:24:21 by
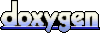