Library for VS1053 chip with non blocking call to check dreq and cancel playback function
Dependents: VS1053Player MP3_test MP3_final_S4P_ANDRIAMARO_RAKOTO_1
Fork of VS1053lib by
VLSIcodec.h
00001 /* mbed VLSIcodec library. To access VS1053 MPEG3 codec. 00002 00003 Copyright (c) 2010 NXP 3790 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #ifndef __VLSICODEC_H 00025 #define __VLSICODEC_H 00026 00027 #include "mbed.h" 00028 00029 /* */ 00030 #define __PLUGINGA 1 00031 /* */ 00032 #define MP3_INIT_SPEED 2000000 /* 2Mbit */ 00033 #define MP3_RUN_SPEED 3000000 /* 3Mbit */ 00034 #define MP3_BUS_MODE 3 00035 00036 /** This library drive a VS1053 codec to play VAW/MP3/Ogg audio file. 00037 * 00038 * @code 00039 * 00040 * VS1053Codec codec( p11, p12, p13, p21, p22, p23, p24); 00041 * 00042 * int main( void) 00043 * { 00044 * codec.init(); // initialize the codec 00045 * codec.loadpatch(); // load the patch code (rev 1.5) 00046 * codec.setbassboost( 15, 150); // set the bass boost value 00047 * codec.settrebleboost( 7, 15000); // set the treable boost value 00048 * codec.setvolume( 0x0, 0x0); // set the volume 00049 * 00050 * // snd_piano is a Ogg file stored in flash memory. 00051 * int cnt=sizeof(snd_piano)/sizeof(snd_piano[0]); 00052 * // 00053 * printf("Start playing file snd_piano [%d]\n\r", cnt); 00054 * // 00055 * int iSize=0; 00056 * while( iSize < cnt) { 00057 * int count=32; 00058 * codec.testdreq(); 00059 * while( count--) { 00060 * codec.writedata( snd_piano[ iSize++]); 00061 * if ( iSize > cnt) 00062 * break; 00063 * } 00064 * } 00065 * } 00066 * 00067 * @endcode 00068 * 00069 */ 00070 00071 // 00072 class VS1053Codec { 00073 00074 public: 00075 00076 /** Initialize the SPI connection to the codec and other services pins. 00077 * 00078 * @param mosi, miso, sclk the SPI connection pins 00079 * @param cs the chip select SPI pin 00080 * @param dreq If DREQ is high, VS1053b can take at least 32 bytes of SDI data or one SCI command. DREQ is turned 00081 * low when the stream buffer is too full and for the duration of a SCI command. 00082 * @param rst hardware reset, active low 00083 * @param xdcs the xdcs pin is the "data chip select". 00084 */ 00085 VS1053Codec( PinName mosi, PinName miso, PinName sclk, PinName cs, PinName dreq, PinName rst, PinName xdcs); 00086 00087 /** Initialize the connection to the VS1053 codec. 00088 * Never return if fail. 00089 */ 00090 void init(void); 00091 00092 /** Test the dreq pin. If high the VS1053 can take data. 00093 * The function never return until the pin goes high. 00094 */ 00095 void testdreq( void); 00096 00097 /** Checks dreq and returns true if the VS1053 can take data. 00098 * Crucially this call does not block allow other operations to be performed. 00099 */ 00100 bool checkdreq(void); 00101 00102 /** Reset the codec 00103 */ 00104 void reset( void); 00105 00106 /** write a byte to the codec 00107 * 00108 * @param data 00109 */ 00110 void writedata( unsigned char data); 00111 00112 /** Set the bass boost value 00113 * 00114 * @param bass Bass gain in dB, range from 0 to 15 00115 * @param freq Limit frequency for bass boost, 10 Hz steps (range from 00116 * 20 to 150) 00117 */ 00118 void setbassboost(unsigned char bass, unsigned char gfreq); 00119 00120 /** Set treable boost value 00121 * 00122 * @param treable Bass gain in dB, range from -8 to 7 00123 * @param freq Limit frequency for bass boost, 1000 Hz steps (range from 00124 * 1000 to 15000) 00125 */ 00126 void settrebleboost( char treble, unsigned int gfreq); 00127 00128 /** Set the volume. 00129 * Note, that after hardware reset the volume is set to full volume. Resetting the software does not reset the 00130 * volume setting. 00131 * 00132 * @param right vol right channel attenuation from maximum volume, 0.5dB steps 00133 * (0x00 = full volume, 0xFE= muted, 0xFF analog power down mode) 00134 * @param left vol left channel attenuation from maximum volume, 0.5dB steps 00135 * (0x00 = full volume, 0xFE= muted, 0xFF analog power down mode) 00136 * 00137 */ 00138 void setvolume(unsigned char vRight, unsigned char vLeft); 00139 00140 /** Get the current decodet time 00141 * 00142 * @param playtime a pointer to a string where the function will write the time in the format 00143 * MM:SS 00144 */ 00145 void getplaytime(char *playtime); 00146 00147 /** Clears the playtime string ready for the next file. 00148 */ 00149 void resetplaytime(); 00150 00151 void cancelplayback(); 00152 00153 /** Start a sine test to verify codec functionality 00154 * 00155 * @param pitch is a composite value. Use 0x7E to play a tone at 5KHz sampled at 22050Hz. 00156 * Use zero to stop to play. 00157 * @code 00158 * codec.sinetest( 0x7E); // start the sine test... 00159 * wait( 1); // wait 1 sec.. 00160 * codec.sinetest( 0x00); // stop the sine test... 00161 * @endcode 00162 */ 00163 void sinetest(unsigned char pitch); 00164 00165 /** Load a VLSI patch code inside the codec. The library is rev 1.5 00166 * This patch enable some features like VMeter. 00167 */ 00168 void loadpatch(void); 00169 00170 #ifdef __ENCODE_OGG 00171 /** Load a VLSI patch code to encode OGG audio file. 00172 * This file will encode at fixed quality. Read the VLSIcodec_OGG.h for more... 00173 */ 00174 void loadoggpatch(void); 00175 #endif 00176 00177 #ifdef __PLUGINGA 00178 /** Load a VLSI patch code that add a 23 band Graphics Analizer. 00179 * 00180 */ 00181 void loadgapatch(void); 00182 void readgavalue( unsigned char *currval, unsigned char *peak); 00183 unsigned short readgabands( void); 00184 #endif 00185 00186 00187 /** Start the Vmeter functionallity. 00188 * 00189 */ 00190 void setvmeter( void); 00191 00192 /** Get vmeter values. Values from 0 to 31 are valid for both channels 00193 * Calculates the peak sample value from both channels and returns the values in 3 dB 00194 * 00195 * @param left left channel value 00196 * @param right right channel value 00197 */ 00198 void getvmeterval( unsigned char* left, unsigned char* right); 00199 00200 /** Put the codec in reset for reduce power consuption. 00201 * Re-init the codec for wake-up. 00202 */ 00203 void lowpower( void); 00204 00205 #ifdef __ENCODE_OGG 00206 /** Start the encoding. Reset and then load the patch to record a audio stream. 00207 */ 00208 void VoggEncoding_Start( void); 00209 00210 /** Read the encoded samples. 00211 * 00212 * @param *voggSample pointer to array to store the sample 00213 * @return number of byte write. 00214 */ 00215 unsigned int VoggEncoding_ReadBuffer( unsigned char *voggSample); 00216 00217 /** Stop the encoding and return the last buffer encoded. 00218 * 00219 * @param *voggSample pointer to array to store the sample 00220 * @return number of byte write. 00221 */ 00222 unsigned int VoggEncoding_Stop( unsigned char *voggSample); 00223 #endif 00224 00225 protected: 00226 // 00227 unsigned short readreg(unsigned char vAddress); 00228 void writereg(unsigned char vAddress, unsigned int wValue); 00229 void writeregnowait(unsigned char vAddress, unsigned int wValue); 00230 // 00231 SPI _spi; 00232 DigitalOut _cs; 00233 DigitalIn _dreq; 00234 DigitalOut _rst; 00235 DigitalOut _xdcs; 00236 00237 }; 00238 00239 #endif
Generated on Fri Jul 22 2022 21:24:21 by
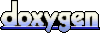