
this transfers data (which is stored in "bin" file in mbed storage) into LPC1114, LPC1115, LPC81x, LPC82x, LPC1768/LPC1769 and LPC11U68/LPC11E68 internal flash memory through ISP.
Dependencies: mbed MODSERIAL DirectoryList
verification.cpp
00001 #include "mbed.h" 00002 #include "verification.h" 00003 #include "command_interface.h" 00004 #include "uu_coding.h" 00005 #include "serial_utilities.h" 00006 #include "writing.h" 00007 #include "isp.h" 00008 #include "_user_settings.h" 00009 00010 00011 00012 int verify_binary_data( FILE *fp, int *transferred_size_p, int file_size ); 00013 int verify_uucoded_data( FILE *fp, int *transferred_size_p, int file_size ); 00014 void get_binary_from_uucode_str( char *b, int size ); 00015 00016 00017 int verify_flash( FILE *fp, target_param *tpp, int *transferred_size_p, int file_size ) 00018 { 00019 if ( tpp->write_type == BINARY ) 00020 return ( verify_binary_data( fp, transferred_size_p, file_size ) ); 00021 else 00022 return ( verify_uucoded_data( fp, transferred_size_p, file_size ) ); 00023 } 00024 00025 00026 int verify_binary_data( FILE *fp, int *read_size_p, int file_size ) 00027 { 00028 char command_str[ STR_BUFF_SIZE ]; 00029 int read_size = 0; 00030 int size; 00031 int flash_reading_size; 00032 char *bf; 00033 char *br; 00034 int error_flag = 0; 00035 unsigned long checksum = 0; 00036 unsigned long checksum_count = 0; 00037 00038 00039 fseek( fp, 0, SEEK_SET ); // seek back to beginning of file 00040 00041 flash_reading_size = 128; 00042 00043 if ( NULL == (bf = (char *)malloc( flash_reading_size * sizeof( char ) )) ) 00044 return( ERROR_AT_MALLOC_FOR_VERIFY_FILE_BUFF ); 00045 00046 if ( NULL == (br = (char *)malloc( flash_reading_size * sizeof( char ) )) ) 00047 return( ERROR_AT_MALLOC_FOR_VERIFY_DATA_BUFF ); 00048 00049 00050 while ( size = fread( bf, sizeof( char ), flash_reading_size, fp ) ) { 00051 00052 if ( read_size < 0x20 ) { 00053 for ( int i = 0; i < flash_reading_size; i += 4 ) { 00054 00055 if ( checksum_count == 7 ) { 00056 checksum = 0xFFFFFFFF - checksum + 1; 00057 *((unsigned int *)(bf + i)) = checksum; 00058 //printf( "\r\n\r\n -- calculated checksum : 0x%08X\r\n", checksum ); 00059 } else { 00060 checksum += *((unsigned int *)(bf + i)); 00061 } 00062 00063 checksum_count++; 00064 } 00065 } 00066 00067 00068 sprintf( command_str, "R %ld %ld\r\n", read_size, (size + 3) & ~0x3 ); // reading size must be 4*N 00069 if ( try_and_check( command_str, "0" ) ) 00070 return ( ERROR_AT_READ_COMMAND ); 00071 00072 get_binary( br, 1 ); 00073 get_binary( br, size ); 00074 00075 for ( int i = 0; i < size; i++ ) { 00076 // printf( " %s 0x%02X --- 0x%02X\r\n", (*(bf + i) != *(br + i)) ? "***" : " ", *(bf + i), *(br + i) ); 00077 if ( (*(bf + i) != *(br + i)) ) { 00078 // printf( " %s 0x%02X --- 0x%02X\r\n", (*(bf + i) != *(br + i)) ? "***" : " ", *(bf + i), *(br + i) ); 00079 error_flag++; 00080 } 00081 } 00082 00083 if ( error_flag ) 00084 break; 00085 00086 read_size += size; 00087 00088 #ifdef ENABLE_PROGRESS_DISPLAY 00089 show_progress( read_size, file_size ); 00090 #endif 00091 } 00092 00093 free( bf ); 00094 free( br ); 00095 00096 *read_size_p = read_size; 00097 00098 return ( error_flag ? ERROR_DATA_DOES_NOT_MATCH : NO_ERROR ); 00099 } 00100 00101 00102 #define LINE_BYTES 44 00103 #define N_OF_LINES 4 00104 #define READ_SIZE (LINE_BYTES * N_OF_LINES) 00105 00106 00107 int verify_uucoded_data( FILE *fp, int *read_size_p, int file_size ) 00108 { 00109 char command_str[ STR_BUFF_SIZE ]; 00110 int read_size = 0; 00111 int size; 00112 int flash_reading_size; 00113 char *bf; 00114 char *br; 00115 int error_flag = 0; 00116 00117 flash_reading_size = READ_SIZE; 00118 00119 initialize_uud_table(); 00120 00121 if ( NULL == (bf = (char *)malloc( flash_reading_size * sizeof( char ) )) ) 00122 error( "malloc error happened (in verify process, file data buffer)\r\n" ); 00123 00124 if ( NULL == (br = (char *)malloc( flash_reading_size * sizeof( char ) )) ) 00125 error( "malloc error happened (in verify process, read data buffer)\r\n" ); 00126 00127 fseek( fp, 0, SEEK_SET ); // seek back to beginning of file 00128 00129 while ( size = fread( bf, sizeof( char ), flash_reading_size, fp ) ) { 00130 00131 if ( !read_size ) { 00132 // overwriting 4 bytes data for address=0x1C 00133 // there is a slot for checksum that is checked in (target's) boot process 00134 add_isp_checksum( bf ); 00135 } 00136 00137 sprintf( command_str, "R %ld %ld\r\n", read_size, (size + 3) & ~0x3 ); // reading size must be 4*N 00138 if ( try_and_check( command_str, "0" ) ) 00139 return ( ERROR_AT_READ_COMMAND ); 00140 00141 get_binary_from_uucode_str( br, size ); 00142 00143 for ( int i = 0; i < size; i++ ) { 00144 // printf( " %s 0x%02X --- 0x%02X\r\n", (*(bf + i) != *(br + i)) ? "***" : " ", *(bf + i), *(br + i) ); 00145 if ( (*(bf + i) != *(br + i)) ) { 00146 // printf( " %s 0x%02X --- 0x%02X\r\n", (*(bf + i) != *(br + i)) ? "***" : " ", *(bf + i), *(br + i) ); 00147 error_flag++; 00148 } 00149 } 00150 00151 if ( error_flag ) 00152 break; 00153 00154 read_size += size; 00155 00156 #ifdef ENABLE_PROGRESS_DISPLAY 00157 show_progress( read_size, file_size ); 00158 #endif 00159 } 00160 00161 free( bf ); 00162 free( br ); 00163 00164 *read_size_p = read_size; 00165 00166 return ( error_flag ? ERROR_DATA_DOES_NOT_MATCH : NO_ERROR ); 00167 } 00168 00169 00170 void get_binary_from_uucode_str( char *b, int size ) 00171 { 00172 char s[ N_OF_LINES ][ STR_BUFF_SIZE ]; 00173 char ss[ STR_BUFF_SIZE ]; 00174 long checksum = 0; 00175 int line_count = 0; 00176 int read_size = 0; 00177 int retry_count = 3; 00178 00179 size = (size + 3) & ~0x3; 00180 00181 while ( retry_count-- ) { 00182 00183 for ( int i = 0; i < ((READ_SIZE < size) ? N_OF_LINES : ((size - 1) / LINE_BYTES) + 1) ; i++ ) 00184 get_string( s[ i ] ); 00185 00186 get_string( ss ); 00187 00188 00189 while ( size ) { 00190 read_size = uudecode_a_line( b, s[ line_count ] ); 00191 00192 for ( int i = 0; i < read_size; i++ ) 00193 checksum += *b++; 00194 00195 size -= read_size; 00196 line_count++; 00197 } 00198 00199 // printf( " checksum -- %s (internal = %ld)\r\n", ss, checksum ); 00200 00201 if ( checksum == atol( ss ) ) { 00202 put_string( "OK\r\n" ); 00203 return; 00204 // printf( " checksum OK\r\n" ); 00205 } else { 00206 printf( " checksum RESEND\r\n" ); 00207 put_string( "RESEND\r\n" ); 00208 } 00209 } 00210 }
Generated on Sat Jul 16 2022 08:56:48 by
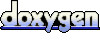