
this transfers data (which is stored in "bin" file in mbed storage) into LPC1114, LPC1115, LPC81x, LPC82x, LPC1768/LPC1769 and LPC11U68/LPC11E68 internal flash memory through ISP.
Dependencies: mbed MODSERIAL DirectoryList
target_handling.cpp
00001 #include "mbed.h" 00002 #include "target_handling.h" 00003 #include "target_table.h" 00004 #include "command_interface.h" 00005 #include "serial_utilities.h" 00006 #include "_user_settings.h" 00007 00008 00009 DigitalOut reset_pin( p26 ); 00010 DigitalOut isp_pin( p25 ); 00011 00012 00013 target_param *open_target( int baud_date ) 00014 { 00015 target_param *tpp; 00016 char str_buf0[ STR_BUFF_SIZE ]; 00017 char str_buf1[ STR_BUFF_SIZE ]; 00018 int retry_count = 3; 00019 00020 set_target_baud_rate( baud_date ); 00021 00022 while ( retry_count-- ) { 00023 reset_target( ENTER_TO_ISP_MODE ); 00024 00025 if ( !try_and_check( "?", "Synchronized" ) ) 00026 break; 00027 } 00028 00029 if ( !retry_count ) 00030 return ( NULL ); 00031 00032 try_and_check2( "Synchronized\r\n", "OK" ); 00033 try_and_check2( "12000\r\n", "OK" ); 00034 try_and_check2( "U 23130\r\n", "0" ); 00035 try_and_check2( "A 0\r\n", "0" ); 00036 00037 try_and_check( "K\r\n", "0" ); 00038 get_string( str_buf0 ); 00039 get_string( str_buf1 ); 00040 00041 #ifndef SUPPRESS_COMMAND_RESULT_MESSAGE 00042 printf( " result of \"K\" = %s %s\r\n", str_buf0, str_buf1 ); 00043 #endif 00044 00045 try_and_check( "J\r\n", "0" ); 00046 get_string( str_buf0 ); 00047 00048 #ifndef SUPPRESS_COMMAND_RESULT_MESSAGE 00049 printf( " result of \"J\" = %s\r\n", str_buf0 ); 00050 #endif 00051 00052 tpp = find_target_param( str_buf0 ); 00053 00054 return ( tpp ); 00055 } 00056 00057 00058 void reset_target( int isp_pin_state ) 00059 { 00060 reset_pin = 1; 00061 isp_pin = isp_pin_state; 00062 wait_ms( 100 ); 00063 00064 reset_pin = 0; 00065 wait_ms( 100 ); 00066 00067 reset_pin = 1; 00068 wait_ms( 100 ); 00069 } 00070
Generated on Sat Jul 16 2022 08:56:48 by
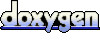