
A sample code for training. Using LPCXpresso baseboard. This program let 16 LEDs blink via I2C (PCA9532). Addition to that, the LED dim control is done by light sensor (ISL29003) output.
main.cpp
00001 /* 00002 * mbed + LPCXpresso_baseboard demo code 00003 * 00004 * This code has been made for a training session. 00005 * 00006 * With this code, the mbed drives PCA9532 to control 16 LEDs. 00007 * The LED blinked in pattern. 00008 * 00009 * On this demo, the ISL29003 is also driven to get ambient 00010 * light level. The ambient light get dark, the LED blightness 00011 * get low. The LEDs are controlled by PWM function of PCA9532. 00012 * 00013 * Copyright (c) 2010 NXP Semiconductors Japan 00014 * Released under the MIT License: http://mbed.org/license/mit 00015 * 00016 * revision 1.0 16-Feb-2010 1st release 00017 */ 00018 00019 #include "mbed.h" 00020 00021 I2C i2c( p28, p27 ); 00022 Serial pc( USBTX, USBRX ); 00023 00024 const int PCA9532_addr = 0xC0; 00025 const int ISL29003_addr = 0x88; 00026 00027 class baseboard_led_array { 00028 public: 00029 00030 baseboard_led_array() { 00031 } 00032 00033 ~baseboard_led_array() { 00034 } 00035 00036 void operator=( int c ) { 00037 00038 char a[ 5 ] = { 0x16, 0x00, 0x00, 0x00, 0x00 }; 00039 const char v = 0x2; 00040 00041 for ( int i = 0; i < 16; i++ ) 00042 a[ (i / 4) + 1 ] |= (((c >> i) & 0x1) ? v : 0x0) << ((i % 4) << 1); 00043 00044 i2c.write( PCA9532_addr, a, 5 ); 00045 } 00046 00047 void pwm0( char c ) { 00048 char cmd[ 2 ]; 00049 00050 cmd[ 0 ] = 0x03; 00051 cmd[ 1 ] = c; 00052 i2c.write( PCA9532_addr, cmd, 2 ); 00053 } 00054 }; 00055 00056 00057 class light_sensor { 00058 public: 00059 00060 light_sensor() { 00061 char cmd[ 2 ]; 00062 00063 cmd[ 0 ] = 0x00; 00064 cmd[ 1 ] = 0x80; 00065 i2c.write( ISL29003_addr, cmd, 2 ); 00066 00067 cmd[ 0 ] = 0x01; 00068 cmd[ 1 ] = 0x00; 00069 i2c.write( ISL29003_addr, cmd, 2 ); 00070 } 00071 00072 ~light_sensor() { 00073 } 00074 00075 operator short( void ) { 00076 00077 short v; 00078 char cmd; 00079 00080 cmd = 0x04; 00081 i2c.write( ISL29003_addr, &cmd, 1 ); 00082 i2c.read( ISL29003_addr, &cmd, 1 ); 00083 v = cmd; 00084 00085 cmd = 0x05; 00086 i2c.write( ISL29003_addr, &cmd, 1 ); 00087 i2c.read( ISL29003_addr, &cmd, 1 ); 00088 00089 return( (((short)cmd) << 8) | v ); 00090 } 00091 }; 00092 00093 baseboard_led_array ledarr; 00094 light_sensor sensor; 00095 00096 int main() { 00097 00098 unsigned long c = 0x00000F0F; 00099 short b; 00100 00101 while ( 1 ) { 00102 00103 b = sensor >> 6; 00104 ledarr.pwm0( b > 255 ? 255 : (char)b ); 00105 00106 c <<= 1; 00107 c |= c & 0x10000 ? 0x1 : 0x0; 00108 ledarr = c; 00109 00110 wait( 0.05 ); 00111 } 00112 } 00113
Generated on Wed Jul 13 2022 20:17:01 by
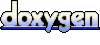