
Hello program for PCA9546A component library. The PCA9546A is a quad bidirectional translating switch controlled via the I2C-bus. The SCL/SDA upstream pair fans out to four downstream pairs, or channels. Any individual SCx/SDx channel or combination of channels can be selected, determined by the contents of the programmable control register.
Dependencies: LM75B PCA9546A mbed
main.cpp
00001 /** 00002 * PCA9546A library "Hello world" sample 00003 * 00004 * @author Tedd OKANO 00005 * @version 0.1 00006 * @date Feb-2015 00007 * 00008 * PCA9546A: an I2C bus switch control library 00009 * 00010 * The PCA9546A is a quad bidirectional translating switch controlled 00011 * via the I2C-bus. The SCL/SDA upstream pair fans out to four downstream 00012 * pairs, or channels. Any individual SCx/SDx channel or combination of 00013 * channels can be selected, determined by the contents of the programmable 00014 * control register. 00015 * 00016 * For more information about PCA9546A: 00017 * http://www.nxp.com/documents/data_sheet/PCA9546A.pdf 00018 * 00019 */ 00020 00021 #include "mbed.h" 00022 #include "LM75B.h" 00023 #include "PCA9546A.h" 00024 00025 PCA9546A i2c_sw( p28, p27, 0xE0 ); 00026 00027 int main() 00028 { 00029 // all PCA9546A's downstream ports are OFF after power-up and hardware-reset 00030 00031 i2c_sw.on( 0 ); // turn-ON the channel 0 00032 00033 LM75B tmp0( p28, p27 ); // making instance after a branch of I2C bus (which is connecting the LM75B) enabled 00034 00035 i2c_sw.off( 0 ); // turn-OFF the channel 0 00036 i2c_sw.on( 1 ); // turn-ON the channel 1 00037 00038 LM75B tmp1( p28, p27 ); // making instance after a branch of I2C bus (which is connecting the LM75B) enabled 00039 00040 while(1) { 00041 00042 i2c_sw.off( 1 ); // turn-OFF the channel 1 00043 i2c_sw.on( 0 ); // turn-ON the channel 0 00044 printf( "%.3f\r\n", tmp0.read() ); 00045 00046 i2c_sw.off( 0 ); // turn-OFF the channel 0 00047 i2c_sw.on( 1 ); // turn-ON the channel 1 00048 printf( "%.3f\r\n", tmp1.read() ); 00049 00050 wait( 1.0 ); 00051 } 00052 }
Generated on Wed Jul 13 2022 09:37:43 by
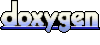