
LPC812 sector size flash write sample code
Fork of IAP_internal_flash_write by
main.cpp
00001 /** 00002 * ISP sector size writing demo 00003 */ 00004 00005 #include "mbed.h" 00006 #include "IAP.h" 00007 00008 #define MEM_SIZE 1024 00009 00010 IAP iap; 00011 00012 void memdump( char *p, int n ); 00013 00014 00015 int main() 00016 { 00017 char mem[ MEM_SIZE ]; // memory, it should be aligned to word boundary 00018 int *serial_number; 00019 int r; 00020 00021 printf( "\r\n\r\n=== IAP: Flash memory writing test ===\r\n" ); 00022 printf( " device-ID = 0x%08X\r\n", iap.read_ID() ); 00023 00024 serial_number = iap.read_serial(); 00025 00026 printf( " serial# =" ); 00027 for ( int i = 0; i < 4; i++ ) 00028 printf( " %08X", *(serial_number + i) ); 00029 printf( "\r\n" ); 00030 00031 printf( " CPU running %dkHz\r\n", SystemCoreClock / 1000 ); 00032 printf( " user reserved flash area: start_address=0x%08X, size=%d bytes\r\n", iap.reserved_flash_area_start(), iap.reserved_flash_area_size() ); 00033 printf( " read_BootVer=0x%08X\r\r\n", iap.read_BootVer() ); 00034 00035 00036 // blank check: The mbed will erase all flash contents after downloading new executable 00037 00038 r = iap.blank_check( 12, 15 ); 00039 printf( "blank check result = 0x%08X\r\n", r ); 00040 00041 // erase sector, if required 00042 00043 if ( r == SECTOR_NOT_BLANK ) { 00044 iap.prepare( 12, 15 ); 00045 r = iap.erase( 12, 15 ); 00046 printf( "erase result = 0x%08X\r\n", r ); 00047 } 00048 00049 // copy RAM to Flash 00050 00051 for ( int target_sector = 12; target_sector < 16; target_sector++ ) { 00052 for ( int i = 0; i < MEM_SIZE; i += 16 ) 00053 sprintf( mem + i, "sctr%02d Osft=%04d", target_sector, i ); 00054 00055 iap.prepare( target_sector, target_sector ); 00056 r = iap.write( mem, sector_start_adress[ target_sector ], MEM_SIZE ); 00057 printf( "copied: SRAM(0x%08X)->Flash(0x%08X) for %d bytes. (result=0x%08X)\r\n", mem, sector_start_adress[ target_sector ], MEM_SIZE, r ); 00058 00059 // compare 00060 00061 r = iap.compare( mem, sector_start_adress[ target_sector ], MEM_SIZE ); 00062 printf( "compare result = \"%s\"\r\n", r ? "FAILED" : "OK" ); 00063 } 00064 00065 for ( int target_sector = 12; target_sector < 16; target_sector++ ) { 00066 printf( "showing the flash contents (sector %02d)...\r\n", target_sector ); 00067 memdump( sector_start_adress[ target_sector ], MEM_SIZE ); 00068 } 00069 00070 printf( "Done! (=== IAP: Flash memory writing test ===)\r\n\r\n" ); 00071 } 00072 00073 #include <ctype.h> 00074 00075 void memdump( char *base, int n ) 00076 { 00077 unsigned int *p; 00078 char s[17] = { '\x0' }; 00079 00080 printf( " memdump from 0x%08X for %d bytes", (unsigned long)base, n ); 00081 00082 p = (unsigned int *)((unsigned int)base & ~(unsigned int)0x3); 00083 00084 for ( int i = 0; i < (n >> 2); i++, p++ ) { 00085 if ( !(i % 4) ) { 00086 printf( " : %s\r\n 0x%08X :", s, (unsigned int)p ); 00087 00088 for ( int j = 0; j < 16; j++) 00089 s[ j ] = isgraph( (int)(*((char *)p + j)) ) ? (*((char *)p + j)) : ' '; 00090 } 00091 00092 printf( " 0x%08X", *p ); 00093 } 00094 00095 printf( " : %s\r\n", s ); 00096 } 00097 00098 00099
Generated on Thu Jul 28 2022 04:17:58 by
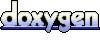