This is SDFileSystem which corrected the bug for MiMicSDK.
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
Fork of SDFileSystem by
FATFileHandle.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2012 ARM Limited 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00020 * SOFTWARE. 00021 */ 00022 #include "ff.h" 00023 #include "ffconf.h" 00024 00025 #include "FATFileHandle.h" 00026 00027 00028 00029 FATFileHandle::FATFileHandle(FIL fh) { 00030 _fh = fh; 00031 } 00032 00033 int FATFileHandle::close() { 00034 int retval = f_close(&_fh); 00035 delete this; 00036 return retval; 00037 } 00038 00039 ssize_t FATFileHandle::write(const void* buffer, size_t length) { 00040 UINT n; 00041 FRESULT res = f_write(&_fh, buffer, length, &n); 00042 if (res) { 00043 return -1; 00044 } 00045 return n; 00046 } 00047 00048 ssize_t FATFileHandle::read(void* buffer, size_t length) { 00049 UINT n; 00050 FRESULT res = f_read(&_fh, buffer, length, &n); 00051 if (res) { 00052 return -1; 00053 } 00054 return n; 00055 } 00056 00057 int FATFileHandle::isatty() { 00058 return 0; 00059 } 00060 00061 off_t FATFileHandle::lseek(off_t position, int whence) { 00062 if (whence == SEEK_END) { 00063 position += _fh.fsize; 00064 } else if(whence==SEEK_CUR) { 00065 position += _fh.fptr; 00066 } 00067 FRESULT res = f_lseek(&_fh, position); 00068 if (res) { 00069 return -1; 00070 } else { 00071 return _fh.fptr; 00072 } 00073 } 00074 00075 int FATFileHandle::fsync() { 00076 FRESULT res = f_sync(&_fh); 00077 if (res) { 00078 return -1; 00079 } 00080 return 0; 00081 } 00082 00083 off_t FATFileHandle::flen() { 00084 return _fh.fsize; 00085 }
Generated on Wed Jul 13 2022 08:47:33 by
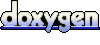