The PCAL9555, PCAL9554 series is a low-voltage 16-bit/8-bit General Purpose Input/Output (GPIO) expander with interrupt. This conponent library is compatible to basic operation os GPIO expanders: PCAL9555, PCA9555, PCA9535, PCA9539, PCAL9554, PCA9554 and PCA9538. On addition to this, this library is including mbed-SDK-style APIs. APIs that similar to DigitaiInOut, DigitalOut, DigitalIn, BusInOUt, BusOut and BusIn are available.
Dependents: PCAL9555_Hello OM13082-JoyStick OM13082_LED OM13082-test ... more
GpioBusOut.h
00001 /** GpioBusOut API for GPIO-expander component class 00002 * 00003 * @author Akifumi (Tedd) OKANO, NXP Semiconductors 00004 * @version 0.6 00005 * @date 19-Mar-2015 00006 * 00007 * Released under the Apache 2 license 00008 */ 00009 00010 #ifndef MBED_GpioBusOut 00011 #define MBED_GpioBusOut 00012 00013 #include "mbed.h" 00014 #include "GpioBusInOut.h" 00015 00016 /** GpioBusOut class 00017 * 00018 * @class GpioBusOut 00019 * 00020 * "GpioBusOut" class works like "BusOut" class of mbed-SDK. 00021 * This class provides pin oriented API, abstracting the GPIO-expander chip. 00022 * 00023 * Example: 00024 * @code 00025 * #include "mbed.h" 00026 * #include "PCAL9555.h" 00027 * 00028 * PCAL9555 gpio_exp( p28, p27, 0xE8 ); // SDA, SCL, Slave_address(option) 00029 * GpioBusOut mypins( gpio_exp, X0_0, X0_1, X0_2, X0_3 ); 00030 * 00031 * int main() { 00032 * while( 1 ) { 00033 * for( int i = 0; i < 16; i++ ) { 00034 * mypins = i; 00035 * wait( 0.25 ); 00036 * } 00037 * } 00038 * } 00039 * @endcode 00040 */ 00041 class GpioBusOut : public GpioBusInOut 00042 { 00043 public: 00044 00045 #if DOXYGEN_ONLY 00046 /** GPIO-Expander pin names */ 00047 typedef enum { 00048 X0_0, /**< P0_0 pin */ 00049 X0_1, /**< P0_1 pin */ 00050 X0_2, /**< P0_2 pin */ 00051 X0_3, /**< P0_3 pin */ 00052 X0_4, /**< P0_4 pin */ 00053 X0_5, /**< P0_5 pin */ 00054 X0_6, /**< P0_6 pin */ 00055 X0_7, /**< P0_7 pin */ 00056 X1_0, /**< P1_0 pin (for 16-bit GPIO device only) */ 00057 X1_1, /**< P1_1 pin (for 16-bit GPIO device only) */ 00058 X1_2, /**< P1_2 pin (for 16-bit GPIO device only) */ 00059 X1_3, /**< P1_3 pin (for 16-bit GPIO device only) */ 00060 X1_4, /**< P1_4 pin (for 16-bit GPIO device only) */ 00061 X1_5, /**< P1_5 pin (for 16-bit GPIO device only) */ 00062 X1_6, /**< P1_6 pin (for 16-bit GPIO device only) */ 00063 X1_7, /**< P1_7 pin (for 16-bit GPIO device only) */ 00064 X0 = X0_0, /**< P0_0 pin */ 00065 X1 = X0_1, /**< P0_1 pin */ 00066 X2 = X0_2, /**< P0_2 pin */ 00067 X3 = X0_3, /**< P0_3 pin */ 00068 X4 = X0_4, /**< P0_4 pin */ 00069 X5 = X0_5, /**< P0_5 pin */ 00070 X6 = X0_6, /**< P0_6 pin */ 00071 X7 = X0_7, /**< P0_7 pin */ 00072 X8 = X1_0, /**< P1_0 pin (for 16-bit GPIO device only) */ 00073 X9 = X1_1, /**< P1_1 pin (for 16-bit GPIO device only) */ 00074 X10 = X1_2, /**< P1_2 pin (for 16-bit GPIO device only) */ 00075 X11 = X1_3, /**< P1_3 pin (for 16-bit GPIO device only) */ 00076 X12 = X1_4, /**< P1_4 pin (for 16-bit GPIO device only) */ 00077 X13 = X1_5, /**< P1_5 pin (for 16-bit GPIO device only) */ 00078 X14 = X1_6, /**< P1_6 pin (for 16-bit GPIO device only) */ 00079 X15 = X1_7, /**< P1_7 pin (for 16-bit GPIO device only) */ 00080 00081 X_NC = ~0x0L /**< for when the pin is left no-connection */ 00082 } GpioPinName; 00083 #endif 00084 00085 /** Create an GpioBusOut, connected to the specified pins 00086 * 00087 * @param gpiop Instance of GPIO expander device 00088 * @param p<n> DigitalInOut pin to connect to bus bit p<n> (GpioPinName) 00089 * 00090 * @note 00091 * It is only required to specify as many pin variables as is required 00092 * for the bus; the rest will default to NC (not connected) 00093 */ 00094 GpioBusOut( CompGpioExp &gpiop, 00095 GpioPinName p0, GpioPinName p1 = X_NC, GpioPinName p2 = X_NC, GpioPinName p3 = X_NC, 00096 GpioPinName p4 = X_NC, GpioPinName p5 = X_NC, GpioPinName p6 = X_NC, GpioPinName p7 = X_NC, 00097 GpioPinName p8 = X_NC, GpioPinName p9 = X_NC, GpioPinName p10 = X_NC, GpioPinName p11 = X_NC, 00098 GpioPinName p12 = X_NC, GpioPinName p13 = X_NC, GpioPinName p14 = X_NC, GpioPinName p15 = X_NC ); 00099 GpioBusOut( CompGpioExp &gpiop, GpioPinName pins[ 16 ] ); 00100 00101 /** 00102 * Destractor 00103 */ 00104 virtual ~GpioBusOut(); 00105 00106 /** A shorthand for write() 00107 */ 00108 GpioBusOut& operator= ( int rhs ); 00109 GpioBusOut& operator= ( GpioBusOut& rhs ); 00110 00111 private: 00112 void init( void ); 00113 } 00114 ; 00115 00116 #endif // MBED_GpioBusOut
Generated on Tue Jul 12 2022 18:40:41 by
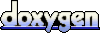