This is a driver code for the PCA9632 is an I2C-bus controlled 4-bit LED driver optimized for Red/Green/Blue/Amber (RGBA) color mixing applications. In Individual brightness control mode, each LED output has its own 8-bit resolution (256 steps) fixed frequency Individual PWM controller that operates at 1.5625 kHz with a duty cycle that is adjustable from 0 % to 99.6 % to allow the LED to be set to a specific brightness value. This library including device class and "LedPwmOut class API" for ease of use.
PCA9632 Class Reference
#include <PCA9632.h>
Public Types | |
enum | LedPinName { L0, L1, L2, L3, L_NC = ~0x0L } |
PCA9626 pin names high-level API i.e. More... | |
enum | command_reg { MODE1, MODE2, PWM0, PWM1, PWM2, PWM3, GRPPWM, GRPFREQ, LEDOUT, SUBADR1, SUBADR2, SUBADR3, ALLCALLADR } |
Name of the PCA9632 registers (for direct register access) More... | |
enum | |
Difinition of the number of LED pins. More... | |
Public Member Functions | |
PCA9632 (PinName i2c_sda, PinName i2c_scl, char i2c_address=DEFAULT_I2C_ADDR) | |
Create a PCA9632 instance connected to specified I2C pins with specified address. | |
PCA9632 (I2C &i2c_obj, char i2c_address=DEFAULT_I2C_ADDR) | |
Create a PCA9632 instance connected to specified I2C pins with specified address. | |
virtual | ~PCA9632 () |
Destractor. | |
void | reset (void) |
Performs Software reset via I2C bus. | |
virtual void | pwm (int port, float v) |
Set the output duty-cycle, specified as a percentage (float) | |
void | pwm (float *vp) |
Set all output port duty-cycle, specified as a percentage (array of float) | |
void | write (char reg_addr, char data) |
Register write (single byte) : Low level access to device register. | |
void | write (char *data, int length) |
Register write (multiple bytes) : Low level access to device register. | |
char | read (char reg_addr) |
Register read (single byte) : Low level access to device register. | |
void | read (char reg_addr, char *data, int length) |
Register write (multiple bytes) : Low level access to device register. |
Detailed Description
PCA9632 class.
This is a driver code for the PCA9632 is an I2C-bus controlled 4-bit LED driver optimized for Red/Green/Blue/Amber (RGBA) color mixing applications. In Individual brightness control mode, each LED output has its own 8-bit resolution (256 steps) fixed frequency Individual PWM controller that operates at 1.5625 kHz with a duty cycle that is adjustable from 0 % to 99.6 % to allow the LED to be set to a specific brightness value. This class provides interface for PCA9632 operation and accessing its registers. Detail information is available on next URL. http://www.jp.nxp.com/products/interface_and_connectivity/i2c/i2c_led_display_control/series/PCA9632.html
Example:
#include "mbed.h" #include "PCA9632.h" PCA9632 led_cntlr( p28, p27, 0xC4 ); // SDA, SCL, Slave_address(option) LedPwmOut led( led_cntlr, L0 ); // for LED0 pin int main() { // // Here are two types of PWM control samples // (User can choose one of those interface to set the PWM.) // // 1st sample is using LedPwmOut API. // It provides similar interface like PwmOut of mbed-SDK // // 2nd sample is using PCA9632 class function. // the 'pwm()' function takes LED channel number and duty-ratio value // while ( 1 ) { // // 1st sample is using LedPwmOut API. // PWM control via LedPwmOut // for ( int i = 0; i < 3; i++ ) { for( float p = 0.0f; p < 1.0f; p += 0.1f ) { led = p; // Controls LED0 pin wait( 0.1 ); } } // // 2nd sample is using PCA9632 class function. // PWM control by device class function call // for ( int i = 0; i < 3; i++ ) { for( float p = 0.0f; p < 1.0f; p += 0.1f ) { led_cntlr.pwm( 1, p ); // Controls LED1 pin wait( 0.1 ); } } } }
Definition at line 88 of file PCA9632.h.
Member Enumeration Documentation
enum command_reg |
Name of the PCA9632 registers (for direct register access)
- Enumerator:
enum LedPinName |
Constructor & Destructor Documentation
PCA9632 | ( | PinName | i2c_sda, |
PinName | i2c_scl, | ||
char | i2c_address = DEFAULT_I2C_ADDR |
||
) |
Create a PCA9632 instance connected to specified I2C pins with specified address.
- Parameters:
-
i2c_sda I2C-bus SDA pin i2c_sda I2C-bus SCL pin i2c_address I2C-bus address (default: 0xC4)
Definition at line 4 of file PCA9632.cpp.
PCA9632 | ( | I2C & | i2c_obj, |
char | i2c_address = DEFAULT_I2C_ADDR |
||
) |
Create a PCA9632 instance connected to specified I2C pins with specified address.
- Parameters:
-
i2c_obj I2C object (instance) i2c_address I2C-bus address (default: 0xC4)
Definition at line 10 of file PCA9632.cpp.
~PCA9632 | ( | ) | [virtual] |
Destractor.
Definition at line 16 of file PCA9632.cpp.
Member Function Documentation
void pwm | ( | int | port, |
float | v | ||
) | [virtual] |
Set the output duty-cycle, specified as a percentage (float)
- Parameters:
-
port Selecting output port 'ALLPORTS' can be used to set all port duty-cycle same value. v A floating-point value representing the output duty-cycle, specified as a percentage. The value should lie between 0.0f (representing on 0%) and 1.0f (representing on 99.6%). Values outside this range will have undefined behavior.
Definition at line 43 of file PCA9632.cpp.
void pwm | ( | float * | vp ) |
Set all output port duty-cycle, specified as a percentage (array of float)
- Parameters:
-
vp Aray to floating-point values representing the output duty-cycle, specified as a percentage. The value should lie between 0.0f (representing on 0%) and 1.0f (representing on 99.6%).
- Note:
- The aray should have length of 4
Definition at line 60 of file PCA9632.cpp.
char read | ( | char | reg_addr ) |
Register read (single byte) : Low level access to device register.
- Parameters:
-
reg_addr Register address
- Returns:
- Read value from register
Definition at line 95 of file PCA9632.cpp.
void read | ( | char | reg_addr, |
char * | data, | ||
int | length | ||
) |
Register write (multiple bytes) : Low level access to device register.
- Parameters:
-
reg_addr Register address data Pointer to an array. The values are stored in this array. length Length of data
Definition at line 88 of file PCA9632.cpp.
void reset | ( | void | ) |
Performs Software reset via I2C bus.
Definition at line 37 of file PCA9632.cpp.
void write | ( | char * | data, |
int | length | ||
) |
Register write (multiple bytes) : Low level access to device register.
- Parameters:
-
data Pointer to an array. First 1 byte should be the writing start register address length Length of data
Definition at line 72 of file PCA9632.cpp.
void write | ( | char | reg_addr, |
char | data | ||
) |
Register write (single byte) : Low level access to device register.
- Parameters:
-
reg_addr Register address data Value for setting into the register
Definition at line 78 of file PCA9632.cpp.
Generated on Wed Jul 13 2022 08:58:56 by
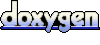