
Small Internet Protocol Stack using a standard serial port.
Go to the source code of this file.
Functions | |
void | pppInitStruct () |
Initialize the ppp structure and clear the receive buffer. | |
void | led1Toggle () |
Toggle the LED. | |
int | connectedPpp () |
Returns 1 after a connect message, 0 at startup or after a disconnect message. | |
void | pppReceiveHandler () |
PPP serial port receive interrupt handler. | |
void | fcsDo (register int x) |
update the cumulative PPP FCS (frame check sequence) | |
int | fcsBuf (char *buf, int size) |
calculate the PPP FCS (frame check sequence) on an entire block of memory | |
int | pc_getBuf () |
Get one character from our received PPP buffer. | |
void | dumpPPPFrame () |
Dump a PPP frame to the debug serial port Note - the hex output of dumpPPPFrame() can be imported into WireShark Capture the frame's hex output in your terminal program and save as a text file In WireShark, use "Import Hex File". | |
void | processPPPFrame (int start, int end) |
Process a received PPP frame. | |
void | hdlcPut (int ch) |
do PPP HDLC-like handling of special (flag) characters | |
void | sendPppFrame () |
send a PPP frame in HDLC format | |
int | bufferToIP (char *buffer) |
convert a network ip address in the buffer to an integer (IP adresses are big-endian, i.e most significant byte first) | |
unsigned int | integersToIp (int a, int b, int c, int d) |
convert 4-byte ip address to 32-bit | |
void | ipcpConfigRequestHandler () |
handle IPCP configuration requests | |
void | ipcpAckHandler () |
handle IPCP acknowledge (do nothing) | |
void | ipcpNackHandler () |
Handle IPCP NACK by sending our suggested IP address if there is an IP involved. | |
void | ipcpDefaultHandler () |
handle all other IPCP requests (by ignoring them) | |
void | IPCPframe () |
process an incoming IPCP packet | |
unsigned int | dataCheckSum (char *ptr, int len, int restart) |
perform a 16-bit checksum. if the byte count is odd, stuff in an extra zero byte. | |
void | IpHeaderCheckSum () |
perform the checksum on an IP header | |
void | swapIpAddresses () |
swap the IP source and destination addresses | |
void | swapIpPorts () |
swap the IP source and destination ports | |
void | checkSumPseudoHeader (unsigned int packetLength) |
Build the "pseudo header" required for UDP and TCP, then calculate its checksum. | |
void | initIP (unsigned int srcIp, unsigned int dstIp, unsigned int srcPort, unsigned int dstPort, unsigned int protocol) |
initialize an IP packet to send | |
void | sendUdp (unsigned int srcIp, unsigned int dstIp, unsigned int srcPort, unsigned int dstPort, char *message, int msgLen) |
Build a UDP packet from scratch. | |
void | UDPpacket () |
Process an incoming UDP packet. | |
void | sendUdpData () |
UDP demo that sends a udp packet containing a character received from the second debug serial port. | |
void | ICMPpacket () |
handle a PING ICMP (internet control message protocol) packet | |
void | IGMPpacket () |
handle an IGMP (internet group managment protocol) packet (by ignoring it) | |
void | dumpHeaderIP (int outGoing) |
dump the header of an IP pakcet on the (optional) debug serial port | |
void | dumpHeaderTCP (int outGoing) |
dump a TCP header on the optional debug serial port | |
int | webSocketHandler (char *dataStart) |
Handle a request for an http websocket. | |
int | httpResponse (char *dataStart, int *flags) |
respond to an HTTP request | |
int | tcpResponse (char *dataStart, int len, int *outFlags) |
Handle TCP data that is not an HTTP GET. | |
void | dumpDataTCP (int outGoing) |
dump the TCP data to the debug serial port | |
void | tcpHandler () |
handle an incoming TCP packet use the first few bytes to figure out if it's a websocket, an http request or just pure incoming TCP data | |
void | TCPpacket () |
handle an incoming TCP packet | |
void | otherProtocol () |
handle the remaining IP protocols by ignoring them | |
void | IPframe () |
process an incoming IP packet | |
void | LCPframe () |
process LCP packets | |
void | discardedFrame () |
discard packets that are not IP, IPCP, or LCP | |
void | determinePacketType () |
determine the packet type (IP, IPCP or LCP) of incoming packets | |
void | sniff () |
a sniffer tool to assist in figuring out where in the code we are having characters in the input buffer | |
void | waitForPppFrame () |
scan the PPP serial input stream for frame start markers | |
void | waitForPcConnectString () |
Wait for a dial-up modem connect command ("CLIENT") from the host PC, if found, we set ppp.online to true, which starts the IP packet scanner. | |
void | initializePpp () |
Initialize PPP data structure and set serial port(s) baud rate(s) | |
Variables | |
static const char | lut [] = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/=" |
Encode a buffer in base-64. |
Detailed Description
Definition in file ppp-blinky.cpp.
Function Documentation
int bufferToIP | ( | char * | buffer ) |
convert a network ip address in the buffer to an integer (IP adresses are big-endian, i.e most significant byte first)
Definition at line 386 of file ppp-blinky.cpp.
void checkSumPseudoHeader | ( | unsigned int | packetLength ) |
Build the "pseudo header" required for UDP and TCP, then calculate its checksum.
Definition at line 519 of file ppp-blinky.cpp.
int connectedPpp | ( | ) |
Returns 1 after a connect message, 0 at startup or after a disconnect message.
Definition at line 208 of file ppp-blinky.cpp.
unsigned int dataCheckSum | ( | char * | ptr, |
int | len, | ||
int | restart | ||
) |
perform a 16-bit checksum. if the byte count is odd, stuff in an extra zero byte.
Definition at line 464 of file ppp-blinky.cpp.
void determinePacketType | ( | ) |
determine the packet type (IP, IPCP or LCP) of incoming packets
Definition at line 1216 of file ppp-blinky.cpp.
void discardedFrame | ( | ) |
discard packets that are not IP, IPCP, or LCP
Definition at line 1210 of file ppp-blinky.cpp.
void dumpDataTCP | ( | int | outGoing ) |
dump the TCP data to the debug serial port
Definition at line 1009 of file ppp-blinky.cpp.
void dumpHeaderIP | ( | int | outGoing ) |
dump the header of an IP pakcet on the (optional) debug serial port
Definition at line 749 of file ppp-blinky.cpp.
void dumpHeaderTCP | ( | int | outGoing ) |
dump a TCP header on the optional debug serial port
Definition at line 772 of file ppp-blinky.cpp.
void dumpPPPFrame | ( | ) |
Dump a PPP frame to the debug serial port Note - the hex output of dumpPPPFrame() can be imported into WireShark Capture the frame's hex output in your terminal program and save as a text file In WireShark, use "Import Hex File".
Import options are: Offset=None, Protocol=PPP.
Definition at line 290 of file ppp-blinky.cpp.
int fcsBuf | ( | char * | buf, |
int | size | ||
) |
calculate the PPP FCS (frame check sequence) on an entire block of memory
Definition at line 268 of file ppp-blinky.cpp.
void fcsDo | ( | register int | x ) |
update the cumulative PPP FCS (frame check sequence)
Definition at line 248 of file ppp-blinky.cpp.
void hdlcPut | ( | int | ch ) |
do PPP HDLC-like handling of special (flag) characters
Definition at line 359 of file ppp-blinky.cpp.
int httpResponse | ( | char * | dataStart, |
int * | flags | ||
) |
respond to an HTTP request
Definition at line 888 of file ppp-blinky.cpp.
void ICMPpacket | ( | ) |
handle a PING ICMP (internet control message protocol) packet
Definition at line 679 of file ppp-blinky.cpp.
void IGMPpacket | ( | ) |
handle an IGMP (internet group managment protocol) packet (by ignoring it)
Definition at line 743 of file ppp-blinky.cpp.
void initializePpp | ( | ) |
Initialize PPP data structure and set serial port(s) baud rate(s)
Definition at line 1550 of file ppp-blinky.cpp.
void initIP | ( | unsigned int | srcIp, |
unsigned int | dstIp, | ||
unsigned int | srcPort, | ||
unsigned int | dstPort, | ||
unsigned int | protocol | ||
) |
initialize an IP packet to send
Definition at line 532 of file ppp-blinky.cpp.
unsigned int integersToIp | ( | int | a, |
int | b, | ||
int | c, | ||
int | d | ||
) |
convert 4-byte ip address to 32-bit
Definition at line 394 of file ppp-blinky.cpp.
void ipcpAckHandler | ( | ) |
handle IPCP acknowledge (do nothing)
Definition at line 419 of file ppp-blinky.cpp.
void ipcpConfigRequestHandler | ( | ) |
handle IPCP configuration requests
Definition at line 400 of file ppp-blinky.cpp.
void ipcpDefaultHandler | ( | ) |
handle all other IPCP requests (by ignoring them)
Definition at line 439 of file ppp-blinky.cpp.
void IPCPframe | ( | ) |
process an incoming IPCP packet
Definition at line 445 of file ppp-blinky.cpp.
void ipcpNackHandler | ( | ) |
Handle IPCP NACK by sending our suggested IP address if there is an IP involved.
This is how Linux responds to an IPCP request with no options - Windows assumes any IP address on the submnet is OK.
Definition at line 426 of file ppp-blinky.cpp.
void IPframe | ( | ) |
process an incoming IP packet
Definition at line 1154 of file ppp-blinky.cpp.
void IpHeaderCheckSum | ( | ) |
perform the checksum on an IP header
Definition at line 489 of file ppp-blinky.cpp.
void LCPframe | ( | ) |
process LCP packets
Definition at line 1176 of file ppp-blinky.cpp.
void led1Toggle | ( | ) |
Toggle the LED.
Definition at line 201 of file ppp-blinky.cpp.
void otherProtocol | ( | ) |
handle the remaining IP protocols by ignoring them
Definition at line 1148 of file ppp-blinky.cpp.
int pc_getBuf | ( | ) |
Get one character from our received PPP buffer.
Definition at line 276 of file ppp-blinky.cpp.
void pppInitStruct | ( | ) |
Initialize the ppp structure and clear the receive buffer.
Definition at line 179 of file ppp-blinky.cpp.
void pppReceiveHandler | ( | ) |
PPP serial port receive interrupt handler.
Check for available characters from the PC and read them into our own circular serial receive buffer at ppp.rx.buf. Also, if we are offline and a 0x7e frame start character is seen, we go online immediately
Definition at line 216 of file ppp-blinky.cpp.
void processPPPFrame | ( | int | start, |
int | end | ||
) |
Process a received PPP frame.
Definition at line 306 of file ppp-blinky.cpp.
void sendPppFrame | ( | ) |
send a PPP frame in HDLC format
Definition at line 370 of file ppp-blinky.cpp.
void sendUdp | ( | unsigned int | srcIp, |
unsigned int | dstIp, | ||
unsigned int | srcPort, | ||
unsigned int | dstPort, | ||
char * | message, | ||
int | msgLen | ||
) |
Build a UDP packet from scratch.
Definition at line 552 of file ppp-blinky.cpp.
void sendUdpData | ( | ) |
UDP demo that sends a udp packet containing a character received from the second debug serial port.
Sends a 48 byte IP/UDP header for every 1 byte of data so line-mode would probably be better. If you want ip packets from ppp blinky to be routed to other networks, ensure you have ip routing enabled. See http://www.wikihow.com/Enable-IP-Routing. Also ensure that the firewall on the receiving machine has the receiving UDP port (12345 in this example) enabled. The netcat UDP receive command on the remote host would be: nc -ul 12345
Definition at line 665 of file ppp-blinky.cpp.
void sniff | ( | ) |
a sniffer tool to assist in figuring out where in the code we are having characters in the input buffer
Definition at line 1498 of file ppp-blinky.cpp.
void swapIpAddresses | ( | ) |
swap the IP source and destination addresses
Definition at line 498 of file ppp-blinky.cpp.
void swapIpPorts | ( | ) |
swap the IP source and destination ports
Definition at line 507 of file ppp-blinky.cpp.
void tcpHandler | ( | ) |
handle an incoming TCP packet use the first few bytes to figure out if it's a websocket, an http request or just pure incoming TCP data
Definition at line 1041 of file ppp-blinky.cpp.
void TCPpacket | ( | ) |
handle an incoming TCP packet
Definition at line 1139 of file ppp-blinky.cpp.
int tcpResponse | ( | char * | dataStart, |
int | len, | ||
int * | outFlags | ||
) |
Handle TCP data that is not an HTTP GET.
This is handy when for example you want to use netcat (nc.exe) to talk to PPP-Blinky. This could also be a websocket receive event - especially if the first byte is 0x81 (websocket data push)
Definition at line 985 of file ppp-blinky.cpp.
void UDPpacket | ( | ) |
Process an incoming UDP packet.
If the packet starts with the string "echo " or "test" we echo back a special packet
Definition at line 580 of file ppp-blinky.cpp.
void waitForPcConnectString | ( | ) |
Wait for a dial-up modem connect command ("CLIENT") from the host PC, if found, we set ppp.online to true, which starts the IP packet scanner.
Definition at line 1535 of file ppp-blinky.cpp.
void waitForPppFrame | ( | ) |
scan the PPP serial input stream for frame start markers
Definition at line 1504 of file ppp-blinky.cpp.
int webSocketHandler | ( | char * | dataStart ) |
Handle a request for an http websocket.
We end up here if we enter the following javascript in a web browser console: x = new WebSocket("ws://172.10.10.2");
Definition at line 838 of file ppp-blinky.cpp.
Variable Documentation
const char lut[] = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/=" [static] |
Encode a buffer in base-64.
Definition at line 810 of file ppp-blinky.cpp.
Generated on Thu Jul 14 2022 20:53:47 by
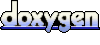