
Small Internet Protocol Stack using a standard serial port.
Embed:
(wiki syntax)
Show/hide line numbers
ppp-blinky.h
00001 /// ppp-blinky.h 00002 00003 #include "mbed.h" 00004 00005 void initializePpp(); 00006 int connectedPpp(); 00007 void waitForPcConnectString(); 00008 void waitForPppFrame(); 00009 void determinePacketType(); 00010 void sendUdpData(); 00011 00012 /// PPP header 00013 typedef struct { // [ff 03 00 21] 00014 unsigned int address : 8; // always 0xff 00015 unsigned int control : 8; // always 03 00016 unsigned int protocolR : 16; // byte reversed, 0x0021 for ip 00017 } pppHeaderType; 00018 00019 /// LCP and IPCP header 00020 typedef struct { 00021 // ppp part 00022 unsigned int address : 8; // always 0xff 00023 unsigned int control : 8; // always 03 00024 unsigned int protocolR : 16; // byte reversed, 0x0021 for ip 00025 00026 // ipcp and lcp part 00027 unsigned int code : 8; // IPCP and LCP contain a code field which identifies the requested action or response 00028 unsigned int identifier : 8 ; 00029 unsigned int lengthR : 16; 00030 char request [0]; 00031 } ipcpHeaderType; 00032 00033 /// IP header 00034 typedef struct { 00035 unsigned int headerLength : 4; // ip headerlength / 4 00036 unsigned int version : 4; // ip version number 00037 unsigned int ect : 1; // ecn capable transport 00038 unsigned int ce : 1; // ecn-ce 00039 unsigned int dscp : 6; // differentiated services 00040 unsigned int lengthR : 16; // ip packet length (byte-reversed) 00041 00042 unsigned int identR : 16; // ident, byte reversed 00043 unsigned int fragmentOffsHi : 5; 00044 unsigned int lastFragment : 1; 00045 unsigned int dontFragment : 1; 00046 unsigned int reservedIP : 1; 00047 unsigned int fragmentOffsLo : 8; 00048 00049 unsigned int ttl : 8; 00050 unsigned int protocol : 8; // next protocol 00051 unsigned int checksumR : 16; // ip checksum, byte reversed 00052 union { 00053 unsigned int srcAdrR; // source IP address 00054 char srcAdrPtr [0]; // so we also have a char * to srcAdrR 00055 }; 00056 union { 00057 unsigned int dstAdrR; // destination IP address 00058 char dstAdrPtr [0]; // so we also have a char * to dstAdrR 00059 }; 00060 } ipHeaderType; 00061 00062 /// IP pseudoheader. Used in TCP/UDP checksum calculations. 00063 typedef struct { 00064 union { 00065 char start [0]; // a char * to avoid type conversions 00066 unsigned int srcAdrR; // source IP address 00067 }; 00068 unsigned int dstAdrR; // destination IP address 00069 unsigned int zero : 8; 00070 unsigned int protocol : 8; 00071 unsigned int lengthR : 16; // byte reversed 00072 } pseudoIpHeaderType; 00073 00074 /// TCP header 00075 typedef struct { 00076 unsigned int srcPortR : 16; // byte reversed 00077 unsigned int dstPortR : 16; // byte reversed 00078 unsigned int seqTcpR; // byte reversed 00079 unsigned int ackTcpR; // byte reversed 00080 unsigned int resvd1 : 4; // reserved 00081 unsigned int offset : 4; // tcp header length [5..15] 00082 union { 00083 unsigned char All; // all 8 flag bits 00084 struct { // individual flag bits 00085 unsigned char fin: 1, // fin 00086 syn : 1, // syn 00087 rst : 1, // rst 00088 psh : 1, // psh 00089 ack : 1, // ack 00090 urg : 1, // urg 00091 ece : 1, // ece 00092 cwr : 1; // cwr 00093 }; 00094 } flag; 00095 unsigned int windowR : 16; // byte reversed 00096 unsigned int checksumR : 16; // byte reversed 00097 unsigned int urgentPointerR : 16; // byte reversed; 00098 unsigned int tcpOptions[10]; // up to 10 words of options possible 00099 } tcpHeaderType; 00100 00101 /// UDP header. 00102 typedef struct { 00103 unsigned int srcPortR : 16; // byte reversed 00104 unsigned int dstPortR : 16; // byte reversed 00105 unsigned int lengthR : 16; // byte reversed 00106 unsigned int checksumR : 16; // byte reversed 00107 char data [0]; // data area 00108 } udpHeaderType; 00109 00110 /// ICMP header. 00111 typedef struct { 00112 unsigned int type : 8; 00113 unsigned int code : 8; 00114 unsigned int checkSumR : 16; // byte reversed 00115 unsigned int idR : 16; // byte reversed 00116 unsigned int sequenceR : 16; // byte reversed 00117 char data [0]; // data area 00118 } icmpHeaderType; 00119 00120 /// Structure to manage all ppp variables. 00121 typedef struct pppType { 00122 union { 00123 pppHeaderType * ppp; // pointer to ppp structure 00124 ipcpHeaderType * ipcp; // pointer to ipcp structure 00125 ipcpHeaderType * lcp; // pointer to lcp structure (same as ipcp) 00126 }; 00127 union { 00128 ipHeaderType * ip; // pointer to ip header struct 00129 char * ipStart; // char pointer to ip header struct (need a char pointer for byte offset calculations) 00130 }; 00131 union { // a union for the packet type contained in the IP packet 00132 tcpHeaderType * tcp; // pointer to tcp header struct 00133 udpHeaderType * udp; // pointer to udp header struct 00134 icmpHeaderType * icmp; // pointer to udp header struct 00135 char * tcpStart; // char pointer to tcp header struct (need a char pointer for byte offset calculations) 00136 char * udpStart; // char pointer to udp header struct (need a char pointer for byte offset calculations) 00137 char * icmpStart; // char pointer to icmp header struct (need a char pointer for byte offset calculations) 00138 }; 00139 char * tcpData; // char pointer to where tcp data starts 00140 volatile int online; // we hunt for a PPP connection if this is zero 00141 int hostIP; // ip address of host 00142 int fcs; // PPP "frame check sequence" - a 16-bit HDLC-like checksum used in all PPP frames 00143 int ledState; // state of LED1 00144 int responseCounter; 00145 unsigned int pppCount; // counts how many ppp packets we receive 00146 int firstFrame; // cleared after first frame 00147 unsigned int sum; // a checksum used in headers 00148 struct { 00149 #define RXBUFLEN 4000 00150 // the serial port receive buffer and packet buffer, size is RXBUFLEN 00151 // TODO - not sure why this buffer has to be this big 00152 volatile char buf[RXBUFLEN+1]; 00153 volatile int head; // declared volatile so user code knows this variable changes in the interrupt handler 00154 volatile int tail; 00155 volatile int rtail; 00156 volatile int buflevel; // how full the buffer is 00157 volatile int maxbuflevel; // maximum value that buflevel ever got to 00158 volatile int bufferfull; // flag when buffer is full 00159 } rx; // serial port objects 00160 struct { 00161 int len; // number of bytes in buffer 00162 int crc; // PPP CRC (frame check) 00163 #define PPP_max_size 1600 00164 // we are assuming 100 bytes more than MTU size of 1500 00165 char buf[PPP_max_size]; // send and receive buffer large enough for largest IP packet 00166 } pkt; // ppp buffer objects 00167 struct { 00168 int frameStartIndex; // frame start marker 00169 int frameEndIndex; // frame end marker 00170 } hdlc; // hdlc frame objects 00171 struct { 00172 unsigned int ident; // our IP ident value (outgoing frame count) 00173 } ipData; // ip related object 00174 } pppVariables; 00175 00176 /* 00177 SHA-1 in C 00178 By Steve Reid <steve@edmweb.com> 00179 100% Public Domain 00180 */ 00181 00182 typedef struct { 00183 uint32_t state[5]; 00184 uint32_t count[2]; 00185 unsigned char buffer[64]; 00186 } SHA1_CTX; 00187 00188 void SHA1Transform( 00189 uint32_t state[5], 00190 const unsigned char buffer[64] 00191 ); 00192 00193 void SHA1Init( 00194 SHA1_CTX * context 00195 ); 00196 00197 void SHA1Update( 00198 SHA1_CTX * context, 00199 const unsigned char *data, 00200 uint32_t len 00201 ); 00202 00203 void SHA1Final( 00204 unsigned char digest[20], 00205 SHA1_CTX * context 00206 ); 00207 00208 void sha1( 00209 char *hash_out, 00210 const char *str, 00211 int len);
Generated on Thu Jul 14 2022 20:53:47 by
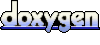