Sensiron SHT 7x Temperature and humidity device library
Dependents: temp xj-Nucleo-F303K8-SHT75-TEST
SHT75 Class Reference
SHT7X driver class. More...
#include <sht7X.h>
Public Member Functions | |
SHT75 (PinName pclock, PinName pdata) | |
Create an SHT object connected to the specified Digital pins. | |
bool | readTempTicks (int *temp) |
read the temperature ticks value 14 bit resolution | |
bool | readHumidityTicks (int *temp) |
read the humidity ticks value 12 bit resolution | |
void | reset (void) |
start up reset |
Detailed Description
SHT7X driver class.
Example:
//initialise the device read temperature ticks, read humidity ticks and then // calculate the liniarised humidity value. #include "mbed.h" #include "sht7X.h" SHT75 sht(p12, p11); Serial pc(USBTX, USBRX); int main() { float temperature; // temperature -40 to 120 deg C float humidity; // relative humidity 1% to 100% float humi_f,rh_lin,rh_true; // working registers for Illustration purposes int t; // temporary store for the temp ticks int h; // temp store for the humidity ticks pc.baud(115200); while(1) { sht.readTempTicks(&t); temperature = ((float)(t) * 0.01) - 39.61; sht.readHumidityTicks(&h); humi_f = (float)(h); rh_lin = C3 * humi_f * humi_f + C2 * humi_f + C1; rh_true=(((temperature/100)-25)*(T1+T2*humi_f)+rh_lin); if(rh_true>100)rh_true=100; //cut if the value is outside if(rh_true<1)rh_true=1; //the physical possible range humidity = rh_true; pc.printf("Temp: %2.2f RH %2.2f\n\r",temperature, humidity); } }
Definition at line 76 of file sht7X.h.
Constructor & Destructor Documentation
SHT75 | ( | PinName | pclock, |
PinName | pdata | ||
) |
Member Function Documentation
bool readHumidityTicks | ( | int * | temp ) |
bool readTempTicks | ( | int * | temp ) |
Generated on Wed Jul 13 2022 20:31:40 by
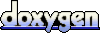