A feature complete driver for the ISL1208 real time clock from Intersil.
Dependents: ISL1208_HelloWorld
ISL1208 Class Reference
#include <ISL1208.h>
Public Types | |
enum | OscillatorMode { OSCILLATOR_CRYSTAL, OSCILLATOR_EXTERNAL } |
Represents the different oscillator modes for the ISL1208. More... | |
enum | OutputFrequency { FOUT_DISABLED = 0, FOUT_32768_HZ, FOUT_4096_HZ, FOUT_1024_HZ, FOUT_64_HZ, FOUT_32_HZ, FOUT_16_HZ, FOUT_8_HZ, FOUT_4_HZ, FOUT_2_HZ, FOUT_1_HZ, FOUT_1_2_HZ, FOUT_1_4_HZ, FOUT_1_8_HZ, FOUT_1_16_HZ, FOUT_1_32_HZ } |
Represents the different output frequencies for the IRQ/fOUT pin on the ISL1208. More... | |
enum | PowerMode { POWER_NORMAL, POWER_LPMODE } |
Represents the power mode of the ISL1208. More... | |
enum | AlarmMode { ALARM_DISABLED, ALARM_SINGLE, ALARM_INTERRUPT } |
Represents the alarm mode of the ISL1208. More... | |
enum | BatteryModeATR { BMATR_ZERO = 0, BMATR_MINUS_0_5, BMATR_PLUS_0_5, BMATR_PLUS_1_0 } |
Represents the different battery mode ATR selections of the ISL1208. More... | |
enum | DigitalTrim { DTR_ZERO = 0, DTR_PLUS_20, DTR_PLUS_40, DTR_PLUS_60, DTR_MINUS_20 = 5, DTR_MINUS_40, DTR_MINUS_60 } |
Represents the different digital trim selections of the ISL1208. More... | |
Public Member Functions | |
ISL1208 (PinName sda, PinName scl, int hz=400000) | |
Create a ISL1208 object connected to the specified I2C pins. | |
bool | open () |
Probe for the ISL1208 and configure auto reset if present. | |
time_t | time () |
Get the current time from the ISL1208. | |
void | time (time_t t) |
Set the current time on the ISL1208 from a Unix timestamp. | |
bool | powerFailed () |
Determine whether the RTC has completely lost power (and hence the time and SRAM) | |
bool | batteryFlag () |
Determine whether the RTC has been on battery backup. | |
void | clearBatteryFlag () |
Clear the battery backup flag. | |
bool | alarmFlag () |
Determine whether the alarm has matched the RTC. | |
void | clearAlarmFlag () |
Clear the alarm flag. | |
ISL1208::OscillatorMode | oscillatorMode () |
Get the current oscillator mode of the ISL1208. | |
void | oscillatorMode (OscillatorMode mode) |
Set the oscillator mode of the ISL1208. | |
ISL1208::OutputFrequency | foutFrequency () |
Get the current output frequency on the IRQ/fOUT pin. | |
void | foutFrequency (OutputFrequency freq) |
Set the output frequency on the IRQ/fOUT pin. | |
bool | outputOnBattery () |
Determine whether or not the IRQ/fOUT pin will continue to output on battery power. | |
void | outputOnBattery (bool output) |
Set whether or not the IRQ/fOUT pin should continue to output on battery power. | |
ISL1208::PowerMode | powerMode () |
Get the current power mode of the ISL1208. | |
void | powerMode (PowerMode mode) |
Set the power mode of the ISL1208. | |
ISL1208::AlarmMode | alarmMode () |
Get the current alarm mode of the ISL1208. | |
void | alarmMode (AlarmMode mode) |
Set the alarm mode of the ISL1208. | |
float | analogTrim () |
Get the current analog trim of the ISL1208. | |
void | analogTrim (float trim) |
Set the analog trim of the ISL1208. | |
ISL1208::BatteryModeATR | batteryModeATR () |
Get the current battery mode analog trim of the ISL1208. | |
void | batteryModeATR (BatteryModeATR atr) |
Set the battery mode analog trim of the ISL1208. | |
ISL1208::DigitalTrim | digitalTrim () |
Get the current digital trim of the ISL1208. | |
void | digitalTrim (DigitalTrim dtr) |
Set the digital trim of the ISL1208. | |
time_t | alarmTime () |
Get the alarm time from the ISL1208. | |
void | alarmTime (time_t t, bool sc=true, bool mn=true, bool hr=true, bool dt=true, bool mo=true, bool dw=true) |
Set the alarm time on the ISL1208 from a Unix timestamp. | |
unsigned short | sram () |
Read the contents of the battery-backed SRAM. | |
void | sram (unsigned short data) |
Write new data to the battery-backed SRAM. |
Detailed Description
ISL1208 class.
Used for controlling an ISL1208 real time clock connected via I2C.
Example:
#include "mbed.h" #include "ISL1208.h" ISL1208 rtc(p28, p27); int main() { //Try to open the ISL1208 if (rtc.open()) { printf("Device detected!\n"); //Configure the oscillator for a 32.768kHz crystal rtc.oscillatorMode(ISL1208::OSCILLATOR_CRYSTAL); //Check if we need to reset the time if (rtc.powerFailed()) { //The time has been lost due to a power complete power failure printf("Device has lost power! Resetting time...\n"); //Set RTC time to Wed, 28 Oct 2009 11:35:37 rtc.time(1256729737); } while(1) { //Get the current time time_t seconds = rtc.time(); //Print the time in various formats printf("\nTime as seconds since January 1, 1970 = %d\n", seconds); printf("Time as a basic string = %s", ctime(&seconds)); char buffer[32]; strftime(buffer, 32, "%I:%M %p\n", localtime(&seconds)); printf("Time as a custom formatted string = %s", buffer); //Delay for 1.0 seconds wait(1.0); } } else { error("Device not detected!\n"); } }
Definition at line 70 of file ISL1208.h.
Member Enumeration Documentation
enum AlarmMode |
Represents the alarm mode of the ISL1208.
- Enumerator:
enum BatteryModeATR |
Represents the different battery mode ATR selections of the ISL1208.
enum DigitalTrim |
Represents the different digital trim selections of the ISL1208.
enum OscillatorMode |
enum OutputFrequency |
Represents the different output frequencies for the IRQ/fOUT pin on the ISL1208.
- Enumerator:
enum PowerMode |
Constructor & Destructor Documentation
ISL1208 | ( | PinName | sda, |
PinName | scl, | ||
int | hz = 400000 |
||
) |
Create a ISL1208 object connected to the specified I2C pins.
- Parameters:
-
sda The I2C data pin. scl The I2C clock pin. hz The I2C bus frequency (defaults to 400kHz).
Definition at line 21 of file ISL1208.cpp.
Member Function Documentation
bool alarmFlag | ( | ) |
Determine whether the alarm has matched the RTC.
- Returns:
- Whether or not the alarm has matched the RTC.
Definition at line 146 of file ISL1208.cpp.
ISL1208::AlarmMode alarmMode | ( | ) |
Get the current alarm mode of the ISL1208.
- Returns:
- The current alarm mode as an AlarmMode enum.
Definition at line 275 of file ISL1208.cpp.
void alarmMode | ( | AlarmMode | mode ) |
Set the alarm mode of the ISL1208.
- Parameters:
-
mode The new alarm mode as an AlarmMode enum.
Definition at line 290 of file ISL1208.cpp.
time_t alarmTime | ( | ) |
Get the alarm time from the ISL1208.
- Returns:
- The current alarm time as a Unix timestamp (with the current year)
Definition at line 411 of file ISL1208.cpp.
void alarmTime | ( | time_t | t, |
bool | sc = true , |
||
bool | mn = true , |
||
bool | hr = true , |
||
bool | dt = true , |
||
bool | mo = true , |
||
bool | dw = true |
||
) |
Set the alarm time on the ISL1208 from a Unix timestamp.
- Parameters:
-
t The alarm time as a Unix timestamp. sc Enable or disable seconds matching. mn Enable or disable minutes matching. hr Enable or disable hours matching. dt Enable or disable date matching. mo Enable or disable month matching. dw Enable or disable day of week matching.
Definition at line 431 of file ISL1208.cpp.
float analogTrim | ( | ) |
Get the current analog trim of the ISL1208.
- Returns:
- The current analog trim in pF.
Definition at line 309 of file ISL1208.cpp.
void analogTrim | ( | float | trim ) |
Set the analog trim of the ISL1208.
- Parameters:
-
trim The new analog trim in pF (valid range 4.5pF to 20.25pF in 0.25pF steps).
Definition at line 327 of file ISL1208.cpp.
bool batteryFlag | ( | ) |
Determine whether the RTC has been on battery backup.
- Returns:
- Whether or not the RTC has been on battery backup.
Definition at line 122 of file ISL1208.cpp.
ISL1208::BatteryModeATR batteryModeATR | ( | ) |
Get the current battery mode analog trim of the ISL1208.
- Returns:
- The current battery mode analog trim as a BatteryModeATR enum.
Definition at line 357 of file ISL1208.cpp.
void batteryModeATR | ( | BatteryModeATR | atr ) |
Set the battery mode analog trim of the ISL1208.
- Parameters:
-
mode The new battery mode analog trim as a BatteryModeATR enum.
Definition at line 369 of file ISL1208.cpp.
void clearAlarmFlag | ( | ) |
Clear the alarm flag.
Definition at line 158 of file ISL1208.cpp.
void clearBatteryFlag | ( | ) |
Clear the battery backup flag.
Definition at line 134 of file ISL1208.cpp.
ISL1208::DigitalTrim digitalTrim | ( | ) |
Get the current digital trim of the ISL1208.
- Returns:
- The current digital trim as a DigitalTrim enum.
Definition at line 384 of file ISL1208.cpp.
void digitalTrim | ( | DigitalTrim | dtr ) |
Set the digital trim of the ISL1208.
- Parameters:
-
mode The new digital trim as a DigitalTrim enum.
Definition at line 396 of file ISL1208.cpp.
ISL1208::OutputFrequency foutFrequency | ( | ) |
Get the current output frequency on the IRQ/fOUT pin.
- Returns:
- The current output frequency.
Definition at line 197 of file ISL1208.cpp.
void foutFrequency | ( | OutputFrequency | freq ) |
Set the output frequency on the IRQ/fOUT pin.
- Parameters:
-
freq The new output frequency.
Definition at line 206 of file ISL1208.cpp.
bool open | ( | ) |
Probe for the ISL1208 and configure auto reset if present.
- Returns:
- 'true' if the device exists on the bus, 'false' if the device doesn't exist on the bus.
Definition at line 27 of file ISL1208.cpp.
ISL1208::OscillatorMode oscillatorMode | ( | ) |
Get the current oscillator mode of the ISL1208.
- Returns:
- The current oscillator mode as a OscillatorMode enum.
Definition at line 170 of file ISL1208.cpp.
void oscillatorMode | ( | OscillatorMode | mode ) |
Set the oscillator mode of the ISL1208.
- Parameters:
-
mode The new oscillator mode as a OscillatorMode enum.
Definition at line 182 of file ISL1208.cpp.
bool outputOnBattery | ( | ) |
Determine whether or not the IRQ/fOUT pin will continue to output on battery power.
- Returns:
- Whether or not the IRQ/fOUT pin will continue to output on battery power.
Definition at line 221 of file ISL1208.cpp.
void outputOnBattery | ( | bool | output ) |
Set whether or not the IRQ/fOUT pin should continue to output on battery power.
- Parameters:
-
output Whether or not the IRQ/fOUT pin should continue to output on battery power.
Definition at line 233 of file ISL1208.cpp.
bool powerFailed | ( | ) |
Determine whether the RTC has completely lost power (and hence the time and SRAM)
- Returns:
- Whether or not the RTC has completely lost power.
Definition at line 110 of file ISL1208.cpp.
ISL1208::PowerMode powerMode | ( | ) |
Get the current power mode of the ISL1208.
- Returns:
- The current power mode as a PowerMode enum.
Definition at line 248 of file ISL1208.cpp.
void powerMode | ( | PowerMode | mode ) |
Set the power mode of the ISL1208.
- Parameters:
-
mode The new power mode as a PowerMode enum.
Definition at line 260 of file ISL1208.cpp.
void sram | ( | unsigned short | data ) |
Write new data to the battery-backed SRAM.
- Parameters:
-
data The new data for the SRAM.
Definition at line 469 of file ISL1208.cpp.
unsigned short sram | ( | ) |
Read the contents of the battery-backed SRAM.
- Returns:
- The current contents of the SRAM.
Definition at line 463 of file ISL1208.cpp.
time_t time | ( | ) |
Get the current time from the ISL1208.
- Returns:
- The current time as a Unix timestamp.
Definition at line 48 of file ISL1208.cpp.
void time | ( | time_t | t ) |
Set the current time on the ISL1208 from a Unix timestamp.
- Parameters:
-
t The current time as a Unix timestamp.
Definition at line 79 of file ISL1208.cpp.
Generated on Tue Jul 12 2022 23:47:46 by
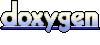