
Mitutoyo ABS Digimatic Caliper Digital Read Out
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "TextLCD.h" 00003 00004 TextLCD mylcd(p24, p26, p27, p28, p29, p30); // rs, e, d4-d7 00005 00006 volatile int n; 00007 int Raw_data[52]; 00008 00009 DigitalOut REQUEST(p20); 00010 DigitalIn DATA(p9); 00011 InterruptIn CLOCK(p17); 00012 00013 void trigger() { //interrupt when CLOCK falled 00014 Raw_data[n] = DATA.read(); 00015 n++; 00016 } 00017 00018 00019 int main() { 00020 int Y; 00021 int Measured_Data[6]; 00022 int Decimal_point; 00023 double temp_data , Indicate; 00024 00025 while(1){ 00026 wait(0.03); 00027 n=0; 00028 00029 REQUEST.write(1); 00030 CLOCK.fall(&trigger); 00031 00032 while(1){ 00033 if(n>52) break; //number of data 00034 } 00035 00036 CLOCK.fall(NULL); 00037 REQUEST.write(0); 00038 00039 Y=20; 00040 for(int i=0;i<=5;i++){ //Raw_data[20]-[43] is Measured_Data 00041 Measured_Data[i] = Raw_data[Y+3]*pow(2.0,3) + Raw_data[Y+2]*pow(2.0,2) + Raw_data[Y+1]*pow(2.0,1) + Raw_data[Y]; 00042 Y=Y+4; 00043 } 00044 00045 Decimal_point = Raw_data[Y+3]*pow(2.0,3)+ Raw_data[Y+2]*pow(2.0,2) +Raw_data[Y+1]*pow(2.0,1)+ Raw_data[Y]; ///Raw_data[44]-[47] is Decimal_point 00046 00047 temp_data = Measured_Data[0]*100000 + Measured_Data[1]*10000 + Measured_Data[2]*1000 + Measured_Data[3]*100 + Measured_Data[4]*10 + Measured_Data[5]; 00048 00049 if (Raw_data[19]==1) //19 is Sign. 0 is '+' , 1 is '-' 00050 temp_data = -1* temp_data; 00051 00052 Indicate = temp_data /pow(10.0,Decimal_point); 00053 00054 mylcd.cls(); 00055 mylcd.printf("%7.2f", Indicate); 00056 00057 if(Raw_data[48]==0) 00058 mylcd.printf("mm"); 00059 else 00060 mylcd.printf("inch"); 00061 } 00062 }
Generated on Sun Jul 17 2022 01:01:19 by
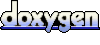