Thanks Erik Olieman for his beta library, that saved me a huge amount of time when getting Raw data from MPU6050 module! I was able to update this library by adding additional functions, which would allow a fast angle calculation by using complementary filter. I will probably be updating this library more soon to add additional functionality or make some changes that would look sensible.
Dependents: QuadcopterProgram 3DTracking ControlYokutan2017 Gyro ... more
Fork of MPU6050 by
MPU6050 Class Reference
#include <MPU6050.h>
Public Member Functions | |
MPU6050 (PinName sda, PinName scl) | |
Constructor. | |
bool | testConnection (void) |
Tests the I2C connection by reading the WHO_AM_I register. | |
void | setBW (char BW) |
Sets the bandwidth of the digital low-pass filter. | |
void | setI2CBypass (bool state) |
Sets the auxiliary I2C bus in bypass mode to read the sensors behind the MPU6050 (useful for eval board, otherwise just connect them to primary I2C bus) | |
void | setAcceleroRange (char range) |
Sets the Accelero full-scale range. | |
int | getAcceleroRawX (void) |
Reads the accelero x-axis. | |
int | getAcceleroRawY (void) |
Reads the accelero y-axis. | |
int | getAcceleroRawZ (void) |
Reads the accelero z-axis. | |
void | getAcceleroRaw (int *data) |
Reads all accelero data. | |
void | getAccelero (float *data) |
Reads all accelero data, gives the acceleration in m/s2. | |
void | setGyroRange (char range) |
Sets the Gyro full-scale range. | |
int | getGyroRawX (void) |
Reads the gyro x-axis. | |
int | getGyroRawY (void) |
Reads the gyro y-axis. | |
int | getGyroRawZ (void) |
Reads the gyro z-axis. | |
void | getGyroRaw (int *data) |
Reads all gyro data. | |
void | getGyro (float *data) |
Reads all gyro data, gives the gyro in rad/s. | |
int | getTempRaw (void) |
Reads temperature data. | |
float | getTemp (void) |
Returns current temperature. | |
void | setSleepMode (bool state) |
Sets the sleep mode of the MPU6050. | |
void | write (char address, char data) |
Writes data to the device, could be private, but public is handy so you can transmit directly to the MPU. | |
char | read (char adress) |
Read data from the device, could be private, but public is handy so you can transmit directly to the MPU. | |
void | read (char adress, char *data, int length) |
Read multtiple regigsters from the device, more efficient than using multiple normal reads. | |
void | getAcceleroAngle (float *data) |
function for calculating the angle from the accelerometer. | |
void | getOffset (float *accOffset, float *gyroOffset, int sampleSize) |
function which allows to produce the offset values for gyro and accelerometer. | |
void | computeAngle (float *angle, float *accOffset, float *gyroOffset, float interval) |
function for computing the angle, when accelerometer angle offset and gyro offset are both known. | |
void | enableInt (void) |
function, which enables interrupts on MPU6050 INT pin | |
void | disableInt (void) |
disables interrupts | |
void | setAlpha (float val) |
function which sets the alpha value - constant for the complementary filter. |
Detailed Description
MPU6050 IMU library.
Example:
Later, maybe
Definition at line 96 of file MPU6050.h.
Constructor & Destructor Documentation
MPU6050 | ( | PinName | sda, |
PinName | scl | ||
) |
Constructor.
Includes.
Sleep mode of MPU6050 is immediatly disabled
- Parameters:
-
sda - mbed pin to use for the SDA I2C line. scl - mbed pin to use for the SCL I2C line.
Definition at line 6 of file MPU6050.cpp.
Member Function Documentation
void computeAngle | ( | float * | angle, |
float * | accOffset, | ||
float * | gyroOffset, | ||
float | interval | ||
) |
function for computing the angle, when accelerometer angle offset and gyro offset are both known.
function for computing angles for roll, pitch anf yaw
we also need to know how much time passed from previous calculation to now it produces the angle in degrees. However angles are produced from -90.0 to 90.0 degrees if anyone need smth different, they can update this library...
- Parameters:
-
angle - calculated accurate angle from complemetary filter accOffset - offset in angle for the accelerometer gyroOffset - offset in rad/s for the gyroscope interval - time before previous angle calculation and now
Definition at line 276 of file MPU6050.cpp.
void disableInt | ( | void | ) |
disables interrupts
function for disbling interrupts on MPU6050 INT pin, when the data is ready to take
Definition at line 310 of file MPU6050.cpp.
void enableInt | ( | void | ) |
function, which enables interrupts on MPU6050 INT pin
function for enabling interrupts on MPU6050 INT pin, when the data is ready to take
Definition at line 302 of file MPU6050.cpp.
void getAccelero | ( | float * | data ) |
Reads all accelero data, gives the acceleration in m/s2.
Function uses the last setup value of the full scale range, if you manually set in another range, this won't work.
- Parameters:
-
data - pointer to float array with length three: data[0] = X, data[1] = Y, data[2] = Z
Definition at line 121 of file MPU6050.cpp.
void getAcceleroAngle | ( | float * | data ) |
function for calculating the angle from the accelerometer.
Additional function added by Montvydas Klumbys, which will allow easy offset, angle calculation and much more.
it takes 3 values which correspond acceleration in X, Y and Z direction and calculates angles in degrees for pitch, roll and one more direction.. (NOT YAW!)
- Parameters:
-
data - angle calculated using only accelerometer
function for getting angles in degrees from accelerometer
Definition at line 241 of file MPU6050.cpp.
void getAcceleroRaw | ( | int * | data ) |
Reads all accelero data.
- Parameters:
-
data - pointer to signed integer array with length three: data[0] = X, data[1] = Y, data[2] = Z
Definition at line 113 of file MPU6050.cpp.
int getAcceleroRawX | ( | void | ) |
Reads the accelero x-axis.
- Returns:
- 16-bit signed integer x-axis accelero data
Definition at line 89 of file MPU6050.cpp.
int getAcceleroRawY | ( | void | ) |
Reads the accelero y-axis.
- Returns:
- 16-bit signed integer y-axis accelero data
Definition at line 97 of file MPU6050.cpp.
int getAcceleroRawZ | ( | void | ) |
Reads the accelero z-axis.
- Returns:
- 16-bit signed integer z-axis accelero data
Definition at line 105 of file MPU6050.cpp.
void getGyro | ( | float * | data ) |
Reads all gyro data, gives the gyro in rad/s.
Function uses the last setup value of the full scale range, if you manually set in another range, this won't work.
- Parameters:
-
data - pointer to float array with length three: data[0] = X, data[1] = Y, data[2] = Z
Definition at line 196 of file MPU6050.cpp.
void getGyroRaw | ( | int * | data ) |
Reads all gyro data.
- Parameters:
-
data - pointer to signed integer array with length three: data[0] = X, data[1] = Y, data[2] = Z
Definition at line 188 of file MPU6050.cpp.
int getGyroRawX | ( | void | ) |
Reads the gyro x-axis.
- Returns:
- 16-bit signed integer x-axis gyro data
Definition at line 164 of file MPU6050.cpp.
int getGyroRawY | ( | void | ) |
Reads the gyro y-axis.
- Returns:
- 16-bit signed integer y-axis gyro data
Definition at line 172 of file MPU6050.cpp.
int getGyroRawZ | ( | void | ) |
Reads the gyro z-axis.
- Returns:
- 16-bit signed integer z-axis gyro data
Definition at line 180 of file MPU6050.cpp.
void getOffset | ( | float * | accOffset, |
float * | gyroOffset, | ||
int | sampleSize | ||
) |
function which allows to produce the offset values for gyro and accelerometer.
function for getting offset values for the gyro & accelerometer
offset for gyro is simply a value, which needs to be substracted from original gyro rad/sec speed but offset for accelerometer is calculated in angles... later on might change that function simply takes the number of samples to be taken and calculated the average
- Parameters:
-
accOffset - accelerometer offset in angle gyroOffset - gyroscope offset in rad/s sampleSize - number of samples to be taken for calculating offsets
Definition at line 254 of file MPU6050.cpp.
float getTemp | ( | void | ) |
Returns current temperature.
- Returns:
- float with the current temperature
Definition at line 231 of file MPU6050.cpp.
int getTempRaw | ( | void | ) |
Reads temperature data.
- Returns:
- 16 bit signed integer with the raw temperature register value
Definition at line 223 of file MPU6050.cpp.
char read | ( | char | adress ) |
Read data from the device, could be private, but public is handy so you can transmit directly to the MPU.
- Parameters:
-
adress - register address to write to
- Returns:
- - data from the register specified by RA
Definition at line 27 of file MPU6050.cpp.
void read | ( | char | adress, |
char * | data, | ||
int | length | ||
) |
Read multtiple regigsters from the device, more efficient than using multiple normal reads.
- Parameters:
-
adress - register address to write to length - number of bytes to read data - pointer where the data needs to be written to
Definition at line 34 of file MPU6050.cpp.
void setAcceleroRange | ( | char | range ) |
Sets the Accelero full-scale range.
Macros: MPU6050_ACCELERO_RANGE_2G - MPU6050_ACCELERO_RANGE_4G - MPU6050_ACCELERO_RANGE_8G - MPU6050_ACCELERO_RANGE_16G
- Parameters:
-
range - The two bits that set the full-scale range (use the predefined macros)
Definition at line 78 of file MPU6050.cpp.
void setAlpha | ( | float | val ) |
function which sets the alpha value - constant for the complementary filter.
function for setting a different Alpha value, which is used in complemetary filter calculations
default alpha = 0.97
- Parameters:
-
val - value the alpha (complementary filter constant) should be set to
Definition at line 297 of file MPU6050.cpp.
void setBW | ( | char | BW ) |
Sets the bandwidth of the digital low-pass filter.
Macros: MPU6050_BW_256 - MPU6050_BW_188 - MPU6050_BW_98 - MPU6050_BW_42 - MPU6050_BW_20 - MPU6050_BW_10 - MPU6050_BW_5 Last number is the gyro's BW in Hz (accelero BW is virtually identical)
- Parameters:
-
BW - The three bits that set the bandwidth (use the predefined macros)
Definition at line 55 of file MPU6050.cpp.
void setGyroRange | ( | char | range ) |
Sets the Gyro full-scale range.
Macros: MPU6050_GYRO_RANGE_250 - MPU6050_GYRO_RANGE_500 - MPU6050_GYRO_RANGE_1000 - MPU6050_GYRO_RANGE_2000
- Parameters:
-
range - The two bits that set the full-scale range (use the predefined macros)
Definition at line 154 of file MPU6050.cpp.
void setI2CBypass | ( | bool | state ) |
Sets the auxiliary I2C bus in bypass mode to read the sensors behind the MPU6050 (useful for eval board, otherwise just connect them to primary I2C bus)
- Parameters:
-
state - Enables/disables the I2C bypass mode
Definition at line 64 of file MPU6050.cpp.
void setSleepMode | ( | bool | state ) |
Sets the sleep mode of the MPU6050.
- Parameters:
-
state - true for sleeping, false for wake up
Definition at line 39 of file MPU6050.cpp.
bool testConnection | ( | void | ) |
Tests the I2C connection by reading the WHO_AM_I register.
- Returns:
- True for a working connection, false for an error
Definition at line 49 of file MPU6050.cpp.
void write | ( | char | address, |
char | data | ||
) |
Writes data to the device, could be private, but public is handy so you can transmit directly to the MPU.
- Parameters:
-
adress - register address to write to data - data to write
Definition at line 19 of file MPU6050.cpp.
Generated on Thu Jul 14 2022 20:41:43 by
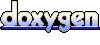