
Basestation code for portable breathalyzer
Dependencies: GP-20U7 PinDetect Servo mbed nRF24L01P
Fork of GP20U7_HelloWorld by
main.cpp
00001 #include "mbed.h" 00002 #include "GPS.h" 00003 #include "Servo.h" 00004 #include "PinDetect.h" 00005 #include "nRF24L01P.h" 00006 00007 nRF24L01P myTransceiver(p5, p6, p7, p8, p9, p10); // mosi, miso, sck, csn, ce, irq 00008 00009 Serial pc(USBTX, USBRX); 00010 GPS gps(p13, p14); 00011 PinDetect pb1(p19); 00012 PinDetect pb2(p20); 00013 Servo servo(p21); 00014 00015 Serial esp(p28, p27); // tx, rx 00016 DigitalOut reset(p26); 00017 DigitalOut led1(LED1); 00018 DigitalOut led2(LED2); 00019 DigitalOut led3(LED3); 00020 Timer t; 00021 00022 bool drunk = true; 00023 00024 int count,ended,timeout,killSwitch = 0,Sig = 0; 00025 char buf[2024]; 00026 char snd[1024]; 00027 00028 char ssid[32] = "AndroidAP"; // enter WiFi router ssid inside the quotes 00029 char pwd [32] = "ece4180a"; // enter WiFi router password inside the quotes 00030 00031 void SendCMD(),getreply(),ESPconfig(),ESPsetbaudrate(),ESPServerSetup(); 00032 00033 void signal(void){ 00034 Sig = 1; 00035 } 00036 00037 void kill_switch(void){ 00038 if (killSwitch == 0){ 00039 killSwitch = 1; 00040 } 00041 } 00042 00043 int main() { 00044 pb1.mode(PullUp); 00045 pb2.mode(PullUp); 00046 00047 reset=0; //hardware reset for 8266 00048 pc.baud(9600); 00049 wait(0.5); 00050 reset=1; 00051 00052 esp.baud(9600); 00053 00054 pb1.attach_deasserted(&kill_switch); 00055 pb1.setSampleFrequency(); 00056 00057 pb2.attach_deasserted(&signal); 00058 pb2.setSampleFrequency(); 00059 00060 led1 = 0; 00061 led2 = 0; 00062 servo = 0; 00063 while (1){ 00064 if (killSwitch == 1){ 00065 led2 = 1; 00066 led1 = 1; 00067 servo = 1.0; 00068 ESPconfig(); 00069 killSwitch = 2; 00070 led1 = 0; 00071 } 00072 if (Sig){ 00073 if(drunk){ 00074 servo = 1.0; 00075 led3 = 1; 00076 //wait(120); 00077 if (drunk) 00078 ESPServerSetup(); 00079 } 00080 else{ 00081 servo = 0; 00082 led2 = 0; 00083 } 00084 killSwitch = 0; 00085 Sig = 0; 00086 } 00087 sleep(); 00088 } 00089 } 00090 00091 void ESPsetbaudrate() 00092 { 00093 strcpy(snd, "AT+CIOBAUD=115200\r\n"); // change the numeric value to the required baudrate 00094 SendCMD(); 00095 } 00096 00097 void ESPconfig() 00098 { 00099 pc.printf("---------- Reset & get Firmware ----------\r\n"); 00100 strcpy(snd,"node.restart()\r\n"); 00101 SendCMD(); 00102 timeout=5; 00103 getreply(); 00104 00105 wait(2); 00106 00107 pc.printf("\n---------- Connecting to AP ----------\r\n"); 00108 pc.printf("ssid = %s pwd = %s\r\n",ssid,pwd); 00109 strcpy(snd, "wifi.sta.config(\""); 00110 strcat(snd, ssid); 00111 strcat(snd, "\",\""); 00112 strcat(snd, pwd); 00113 strcat(snd, "\")\r\n"); 00114 SendCMD(); 00115 timeout=10; 00116 getreply(); 00117 pc.printf(buf); 00118 00119 wait(5); 00120 00121 pc.printf("\n---------- Get IP's ----------\r\n"); 00122 strcpy(snd, "print(wifi.sta.getip())\r\n"); 00123 SendCMD(); 00124 timeout=3; 00125 getreply(); 00126 pc.printf(buf); 00127 00128 wait(1); 00129 } 00130 00131 void ESPServerSetup(){ 00132 while (!gps.sample()){ 00133 wait(0.1); 00134 } 00135 pc.printf("\n---------- Setting up http server ----------\r\n"); 00136 strcpy(snd, "srv=net.createServer(net.TCP)\r\n"); 00137 SendCMD(); 00138 wait(0.2); 00139 strcpy(snd, "srv:listen(80,function(conn)\r\n"); 00140 SendCMD(); 00141 wait(0.2); 00142 strcpy(snd, "conn:on(\"receive\",function(conn,payload)\r\n"); 00143 SendCMD(); 00144 wait(0.2); 00145 strcpy(snd, "print(payload)\r\n"); 00146 SendCMD(); 00147 wait(0.2); 00148 strcpy(snd, "conn:send(\"<!DOCTYPE html>\")\r\n"); 00149 SendCMD(); 00150 wait(0.2); 00151 strcpy(snd, "conn:send(\"<html>\")\r\n"); 00152 SendCMD(); 00153 wait(0.2); 00154 strcpy(snd, "conn:send(\"<h1> Come Pick Me up, I'm Drunk</h1>\")\r\n"); 00155 SendCMD(); 00156 wait(0.2); 00157 gps.sample(); 00158 int n = sprintf(snd, "conn:send(\"<h2>I'm at Longitude: %f degrees %c, Latitude %f degrees %c</h2>\")\r\n", gps.longitude, gps.ew, gps.latitude, gps.ns); 00159 SendCMD(); 00160 wait(0.2); 00161 strcpy(snd, "conn:send(\"</html>\")\r\n"); 00162 SendCMD(); 00163 wait(0.2); 00164 strcpy(snd, "end)\r\n"); 00165 SendCMD(); 00166 wait(0.2); 00167 strcpy(snd, "conn:on(\"sent\",function(conn) conn:close() end)\r\n"); 00168 SendCMD(); 00169 wait(0.2); 00170 strcpy(snd, "end)\r\n"); 00171 SendCMD(); 00172 wait(0.2); 00173 timeout=5; 00174 getreply(); 00175 pc.printf(buf); 00176 pc.printf("\r\nDONE"); 00177 } 00178 00179 void SendCMD() 00180 { 00181 esp.printf("%s", snd); 00182 } 00183 00184 void getreply() 00185 { 00186 memset(buf, '\0', sizeof(buf)); 00187 t.start(); 00188 ended=0; 00189 count=0; 00190 while(!ended) { 00191 if(esp.readable()) { 00192 buf[count] = esp.getc(); 00193 count++; 00194 } 00195 if(t.read() > timeout) { 00196 ended = 1; 00197 t.stop(); 00198 t.reset(); 00199 } 00200 } 00201 }
Generated on Thu Jul 14 2022 13:39:08 by
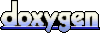