An example program for the S25FL216K flash memory
Dependencies: S25FL216K_FATFileSystem mbed
Fork of S25FL216K_HelloWorld by
main.cpp
00001 #include "mbed.h" 00002 #include "Flash_FileSystem.h" 00003 00004 FlashSPI flash(P0_9, P0_8, P0_7, P0_6, "flash"); 00005 00006 int main() 00007 { 00008 wait(0.1); 00009 printf("Hello World!\r\n"); 00010 char buffer[100]; 00011 FILE *fp; 00012 00013 int i; 00014 00015 printf("directory list\r\n"); 00016 DIR *d = opendir("/flash"); // Opens the root directory of the local file system 00017 struct dirent *p; 00018 i = 0; 00019 while((p = readdir(d)) != NULL) { // Print the names of the files in the local file system 00020 printf("%s\r\n", p->d_name); // to stdout. 00021 i++; 00022 } 00023 closedir(d); 00024 printf("total %d files found.\r\n\n", i); 00025 00026 fp = fopen("/flash/in1.txt", "a"); 00027 printf("-0-\r\n"); 00028 00029 if(fp == NULL) { 00030 printf("Could not open file, assuming unformatted disk!\r\n"); 00031 printf("Formatting disk!\r\n"); 00032 flash.format(); 00033 printf("Disk formatted!\r\n"); 00034 printf("Reset your device!\r\n"); 00035 while(1); 00036 } else { 00037 wait(0.2); 00038 fprintf(fp, "hello "); 00039 fclose(fp); 00040 } 00041 00042 wait(0.5); 00043 00044 fp = fopen("/flash/in2.txt", "a"); 00045 if (fp == NULL) { 00046 printf("out.txt can't created.\r\n"); 00047 } else { 00048 wait(0.2); 00049 fprintf(fp, "Hello 2 "); 00050 fclose(fp); 00051 } 00052 00053 wait(0.5); 00054 00055 fp = fopen("/flash/in1.txt", "r"); 00056 if (fp == NULL) { 00057 printf("in1.txt can't open to read.\r\n"); 00058 } else 00059 { 00060 fgets (buffer, 100, fp); 00061 printf("%s\r\n", buffer); 00062 fclose(fp); 00063 } 00064 00065 wait(0.5); 00066 00067 fp = fopen("/flash/in2.txt", "r"); 00068 if (fp == NULL) { 00069 printf("in2.txt can't open to read.\r\n"); 00070 } else 00071 { 00072 fgets (buffer, 100, fp); 00073 printf("%s\r\n", buffer); 00074 fclose(fp); 00075 } 00076 00077 }
Generated on Thu Aug 25 2022 07:21:13 by
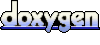