
OV7670 with FIFO AL422B (TORAGI Camera TYPE B) test program
Embed:
(wiki syntax)
Show/hide line numbers
ov7670.h
00001 #include "mbed.h" 00002 #include "ov7670reg.h" 00003 00004 #define OV7670_WRITE (0x42) 00005 #define OV7670_READ (0x43) 00006 #define OV7670_WRITEWAIT (20) 00007 #define OV7670_NOACK (0) 00008 #define OV7670_REGMAX (201) 00009 #define OV7670_I2CFREQ (50000) 00010 00011 // 00012 // OV7670 + FIFO AL422B camera board test 00013 // 00014 class OV7670 : public Base 00015 { 00016 public: 00017 I2C camera ; 00018 InterruptIn vsync,href; 00019 DigitalOut wen ; 00020 BusIn data ; 00021 DigitalOut rrst,oe,rclk ; 00022 volatile int LineCounter ; 00023 volatile int LastLines ; 00024 volatile bool CaptureReq ; 00025 volatile bool Busy ; 00026 volatile bool Done ; 00027 00028 OV7670( 00029 PinName sda,// Camera I2C port 00030 PinName scl,// Camera I2C port 00031 PinName vs, // VSYNC 00032 PinName hr, // HREF 00033 PinName we, // WEN 00034 PinName d7, // D7 00035 PinName d6, // D6 00036 PinName d5, // D5 00037 PinName d4, // D4 00038 PinName d3, // D3 00039 PinName d2, // D2 00040 PinName d1, // D1 00041 PinName d0, // D0 00042 PinName rt, // /RRST 00043 PinName o, // /OE 00044 PinName rc // RCLK 00045 ) : camera(sda,scl),vsync(vs),href(hr),wen(we),data(d0,d1,d2,d3,d4,d5,d6,d7),rrst(rt),oe(o),rclk(rc) 00046 { 00047 camera.stop() ; 00048 camera.frequency(OV7670_I2CFREQ) ; 00049 vsync.fall(this,&OV7670::VsyncHandler) ; 00050 href.rise(this,&OV7670::HrefHandler) ; 00051 CaptureReq = false ; 00052 Busy = false ; 00053 Done = false ; 00054 LineCounter = 0 ; 00055 rrst = 1 ; 00056 oe = 1 ; 00057 rclk = 1 ; 00058 wen = 0 ; 00059 } 00060 00061 // capture request 00062 void CaptureNext(void) 00063 { 00064 CaptureReq = true ; 00065 Busy = true ; 00066 } 00067 00068 // capture done? (with clear) 00069 bool CaptureDone(void) 00070 { 00071 bool result ; 00072 if (Busy) { 00073 result = false ; 00074 } else { 00075 result = Done ; 00076 Done = false ; 00077 } 00078 return result ; 00079 } 00080 00081 // write to camera 00082 void WriteReg(int addr,int data) 00083 { 00084 // WRITE 0x42,ADDR,DATA 00085 camera.start() ; 00086 camera.write(OV7670_WRITE) ; 00087 wait_us(OV7670_WRITEWAIT); 00088 camera.write(addr) ; 00089 wait_us(OV7670_WRITEWAIT); 00090 camera.write(data) ; 00091 camera.stop() ; 00092 } 00093 00094 // read from camera 00095 int ReadReg(int addr) 00096 { 00097 int data ; 00098 00099 // WRITE 0x42,ADDR 00100 camera.start() ; 00101 camera.write(OV7670_WRITE) ; 00102 wait_us(OV7670_WRITEWAIT); 00103 camera.write(addr) ; 00104 camera.stop() ; 00105 wait_us(OV7670_WRITEWAIT); 00106 00107 // WRITE 0x43,READ 00108 camera.start() ; 00109 camera.write(OV7670_READ) ; 00110 wait_us(OV7670_WRITEWAIT); 00111 data = camera.read(OV7670_NOACK) ; 00112 camera.stop() ; 00113 00114 return data ; 00115 } 00116 00117 void Reset(void) { 00118 WriteReg(0x12,0x80) ; // RESET CAMERA 00119 wait_ms(200) ; 00120 } 00121 00122 void InitQQVGA() { 00123 // QQVGA RGB444 00124 WriteReg(REG_CLKRC,0x80); 00125 WriteReg(REG_COM11,0x0A) ; 00126 WriteReg(REG_TSLB,0x04); 00127 WriteReg(REG_COM7,0x04) ; 00128 WriteReg(REG_RGB444, 0x02); 00129 WriteReg(REG_COM15, 0xd0); 00130 WriteReg(REG_HSTART,0x16) ; 00131 WriteReg(REG_HSTOP,0x04) ; 00132 WriteReg(REG_HREF,0x24) ; 00133 WriteReg(REG_VSTART,0x02) ; 00134 WriteReg(REG_VSTOP,0x7a) ; 00135 WriteReg(REG_VREF,0x0a) ; 00136 WriteReg(REG_COM10,0x02) ; 00137 WriteReg(REG_COM3, 0x04); 00138 WriteReg(REG_COM14, 0x1a); 00139 WriteReg(0x72, 0x22); 00140 WriteReg(0x73, 0xf2); 00141 00142 // COLOR SETTING 00143 WriteReg(0x4f,0x80); 00144 WriteReg(0x50,0x80); 00145 WriteReg(0x51,0x00); 00146 WriteReg(0x52,0x22); 00147 WriteReg(0x53,0x5e); 00148 WriteReg(0x54,0x80); 00149 WriteReg(0x56,0x40); 00150 WriteReg(0x58,0x9e); 00151 WriteReg(0x59,0x88); 00152 WriteReg(0x5a,0x88); 00153 WriteReg(0x5b,0x44); 00154 WriteReg(0x5c,0x67); 00155 WriteReg(0x5d,0x49); 00156 WriteReg(0x5e,0x0e); 00157 WriteReg(0x69,0x00); 00158 WriteReg(0x6a,0x40); 00159 WriteReg(0x6b,0x0a); 00160 WriteReg(0x6c,0x0a); 00161 WriteReg(0x6d,0x55); 00162 WriteReg(0x6e,0x11); 00163 WriteReg(0x6f,0x9f); 00164 00165 WriteReg(0xb0,0x84); 00166 } 00167 00168 00169 00170 // vsync handler 00171 void VsyncHandler(void) 00172 { 00173 // Capture Enable 00174 if (CaptureReq) { 00175 wen = 1 ; 00176 Done = false ; 00177 CaptureReq = false ; 00178 } else { 00179 wen = 0 ; 00180 if (Busy) { 00181 Busy = false ; 00182 Done = true ; 00183 } 00184 } 00185 00186 // Hline Counter 00187 LastLines = LineCounter ; 00188 LineCounter = 0 ; 00189 } 00190 00191 // href handler 00192 void HrefHandler(void) 00193 { 00194 LineCounter++ ; 00195 } 00196 00197 // Data Read 00198 int ReadOneByte(void) 00199 { 00200 int result ; 00201 rclk = 1 ; 00202 // wait_us(1) ; 00203 result = data ; 00204 rclk = 0 ; 00205 return result ; 00206 } 00207 00208 // Data Start 00209 void ReadStart(void) 00210 { 00211 rrst = 0 ; 00212 oe = 0 ; 00213 wait_us(1) ; 00214 rclk = 0 ; 00215 wait_us(1) ; 00216 rclk = 1 ; 00217 wait_us(1) ; 00218 rrst = 1 ; 00219 } 00220 00221 // Data Stop 00222 void ReadStop(void) 00223 { 00224 oe = 1 ; 00225 ReadOneByte() ; 00226 rclk = 1 ; 00227 } 00228 };
Generated on Fri Jul 15 2022 11:52:59 by
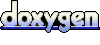