observe fixes
Dependencies: nanoservice_client_1_12_X Nanostack_lib
Fork of mbedEndpointNetwork_6LowPAN by
low_level_RF.cpp
00001 /* this file needs customization 00002 according the target hardware 00003 */ 00004 00005 00006 #include "mbed.h" 00007 00008 extern "C" void rf_if_interrupt_handler(void); 00009 00010 #if defined(TARGET_K64F) 00011 SPI spi(PTD2, PTD3, PTD1); 00012 #elif defined(TARGET_NUCLEO_F401RE) 00013 SPI spi(SPI_MOSI, SPI_MISO, SPI_SCK); 00014 #elif defined(TARGET_NUCLEO_F411RE) 00015 SPI spi(SPI_MOSI, SPI_MISO, SPI_SCK); 00016 #else 00017 /* "SPI not defined for this platform" */ 00018 SPI spi(D11, D12, D13); 00019 #endif 00020 00021 DigitalOut RF_CS(D10); 00022 DigitalOut RF_RST(D5); 00023 DigitalOut RF_SLP_TR(D7); 00024 InterruptIn RF_IRQ(D9); 00025 00026 00027 extern "C" void RF_IRQ_Init(void) { 00028 00029 RF_IRQ.rise(&rf_if_interrupt_handler); 00030 } 00031 00032 extern "C" void RF_RST_Set(int state) { 00033 RF_RST = state; 00034 } 00035 00036 extern "C" void RF_SLP_TR_Set(int state) { 00037 RF_SLP_TR = state; 00038 } 00039 00040 extern "C" void RF_CS_while_active(void) { 00041 00042 while(!RF_CS); 00043 } 00044 00045 00046 extern "C" int spi_read(char addr) { 00047 00048 // Select the device by seting chip select low 00049 RF_CS = 0; 00050 // Write the reg. address 00051 spi.write(addr); 00052 // write a dummy value to read the reg. value 00053 int val = spi.write(0x00); 00054 // Deselect the device 00055 RF_CS = 1; 00056 return val; 00057 } 00058 00059 extern "C" void spi_write(char addr, char val) { 00060 00061 // Select the device by seting chip select low 00062 RF_CS = 0; 00063 // Write the reg. address 00064 spi.write(addr); 00065 // write the value to the addresses register 00066 spi.write(val); 00067 // Deselect the device 00068 RF_CS = 1; 00069 } 00070 00071 extern "C" void RF_CS_Set(int state) { 00072 RF_CS = state; 00073 } 00074 00075 extern "C" int spi_exchange(char value) { 00076 00077 // write the value 00078 int val = spi.write(value); 00079 return val; 00080 }
Generated on Fri Jul 15 2022 08:02:55 by
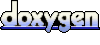