observe fixes
Dependencies: nanoservice_client_1_12_X Nanostack_lib
Fork of mbedEndpointNetwork_6LowPAN by
arm_timer.cpp
00001 #include "mbed.h" 00002 #include "arm_hal_timer.h" 00003 00004 static void (*sn_callback)(void) = NULL; 00005 static Ticker sn_timer; 00006 //static uint32_t sn_compare_value; 00007 static timestamp_t sn_compare_value; 00008 static bool timer_enabled = false; 00009 00010 #define MAXIMUM_SLOTS 10000 00011 00012 extern "C" void arm_timer_init(void) 00013 { 00014 //init in Ticker ctor 00015 } 00016 00017 extern "C" void arm_timer_set_cb(void (*callback)(void)) 00018 { 00019 sn_callback = callback; 00020 } 00021 00022 extern "C" void arm_timer_start(uint16_t slots) 00023 { 00024 sn_compare_value = slots * 50; // 1 slot = 50us 00025 sn_timer.attach_us(sn_callback, sn_compare_value); 00026 timer_enabled = true; 00027 } 00028 00029 extern "C" void arm_timer_stop(void) 00030 { 00031 sn_timer.detach(); 00032 timer_enabled = false; 00033 } 00034 00035 extern "C" uint16_t arm_timer_get_remaining_slots(void) 00036 { 00037 uint32_t counter = us_ticker_read(); 00038 uint32_t slots = ((sn_compare_value - counter) / 50); 00039 00040 if ((slots > MAXIMUM_SLOTS) || (timer_enabled == false)) { 00041 slots = 0; 00042 } 00043 00044 return (uint16_t)slots; 00045 }
Generated on Fri Jul 15 2022 08:02:55 by
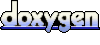