
motor i senzor
Dependencies: TextLCD mbed PinDetect
Motor.cpp
00001 #include "Motor.h" 00002 00003 Motor::Motor(PinName positivePin, PinName negativePin, PinName pwmPin): 00004 positiveOut(positivePin), negativeOut(negativePin), pwmOut(pwmPin) { 00005 00006 pwmOut.period(motorPwmPeriod); 00007 pwmOut = motorPwmInitDutyCycle; 00008 00009 positiveOut = 0; 00010 negativeOut = 0; 00011 direction = 0; 00012 pwmOut = 1; 00013 } 00014 00015 void Motor::start() { 00016 00017 for (float i = 1; i > 0; i -= motorPwmChangeSpeed) { 00018 pwmOut = i; 00019 wait(motorPwmWaitTime); 00020 } 00021 pwmOut = 0; 00022 } 00023 00024 void Motor::stop() { 00025 00026 if(direction == 0) 00027 return; 00028 00029 for (float i = 0; i < 1; i += motorPwmChangeSpeed) { 00030 pwmOut = i; 00031 wait(motorPwmWaitTime); 00032 } 00033 pwmOut = 1; 00034 00035 positiveOut = 0; 00036 negativeOut = 0; 00037 direction = 0; 00038 wait_ms(1000); 00039 } 00040 00041 void Motor::movePositive() { 00042 00043 if(direction == 1) 00044 return; 00045 00046 if(direction == -1) 00047 stop(); 00048 00049 positiveOut = 1; 00050 direction = 1; 00051 start(); 00052 } 00053 00054 void Motor::moveNegative() { 00055 00056 if(direction == -1) 00057 return; 00058 00059 if(direction == 1) 00060 stop(); 00061 00062 negativeOut = 1; 00063 direction = -1; 00064 start(); 00065 } 00066 00067 bool Motor::isMoving() { 00068 00069 return direction == 0; 00070 }
Generated on Mon Jul 18 2022 17:38:21 by
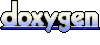