
Basic example showing the Mutex API
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 00004 Mutex stdio_mutex; 00005 00006 void notify(const char* name, int state) { 00007 stdio_mutex.lock(); 00008 printf("%s: %d\n\r", name, state); 00009 stdio_mutex.unlock(); 00010 } 00011 00012 void test_thread(void const *args) { 00013 while (true) { 00014 notify((const char*)args, 0); Thread::wait(1000); 00015 notify((const char*)args, 1); Thread::wait(1000); 00016 } 00017 } 00018 00019 int main() { 00020 Thread t2; 00021 Thread t3; 00022 00023 t2.start(callback(test_thread, (void *)"Th 2")); 00024 t3.start(callback(test_thread, (void *)"Th 3")); 00025 00026 test_thread((void *)"Th 1"); 00027 }
Generated on Tue Jul 12 2022 11:27:35 by
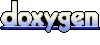