mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
ATCmdParser Class Reference
[ATCmdParser class]
Parser class for parsing AT commands. More...
#include <ATCmdParser.h>
Inherits NonCopyable< ATCmdParser >, and NonCopyable< ATCmdParser >.
Public Member Functions | |
ATCmdParser (FileHandle *fh, const char *output_delimiter="\r", int buffer_size=256, int timeout=8000, bool debug=false) | |
Constructor. | |
~ATCmdParser () | |
Destructor. | |
void | set_timeout (int timeout) |
Allows timeout to be changed between commands. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.5.0","Replaced with set_timeout for consistency") void setTimeout(int timeout) | |
For backward compatibility. | |
void | set_delimiter (const char *output_delimiter) |
Sets string of characters to use as line delimiters. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.5.0","Replaced with set_delimiter for consistency") void setDelimiter(const char *output_delimiter) | |
For backwards compatibility. | |
void | debug_on (uint8_t on) |
Allows traces from modem to be turned on or off. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.5.0","Replaced with debug_on for consistency") void debugOn(uint8_t on) | |
For backward compatibility. | |
bool | send (const char *command,...) MBED_PRINTF_METHOD(1 |
Sends an AT command. | |
bool | recv (const char *response,...) MBED_SCANF_METHOD(1 |
Receive an AT response. | |
int | putc (char c) |
Write a single byte to the underlying stream. | |
int | getc () |
Get a single byte from the underlying stream. | |
int | write (const char *data, int size) |
Write an array of bytes to the underlying stream. | |
int | read (char *data, int size) |
Read an array of bytes from the underlying stream. | |
int | printf (const char *format,...) MBED_PRINTF_METHOD(1 |
Direct printf to underlying stream. | |
int | scanf (const char *format,...) MBED_SCANF_METHOD(1 |
Direct scanf on underlying stream. | |
void | oob (const char *prefix, mbed::Callback< void()> func) |
Attach a callback for out-of-band data. | |
void | flush () |
Flushes the underlying stream. | |
void | abort () |
Abort current recv. | |
bool | process_oob (void) |
Process out-of-band data. | |
ATCmdParser (FileHandle *fh, const char *output_delimiter="\r", int buffer_size=256, int timeout=8000, bool debug=false) | |
Constructor. | |
~ATCmdParser () | |
Destructor. | |
void | set_timeout (int timeout) |
Allows timeout to be changed between commands. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.5.0","Replaced with set_timeout for consistency") void setTimeout(int timeout) | |
For backward compatibility. | |
void | set_delimiter (const char *output_delimiter) |
Sets string of characters to use as line delimiters. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.5.0","Replaced with set_delimiter for consistency") void setDelimiter(const char *output_delimiter) | |
For backwards compatibility. | |
void | debug_on (uint8_t on) |
Allows traces from modem to be turned on or off. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.5.0","Replaced with debug_on for consistency") void debugOn(uint8_t on) | |
For backward compatibility. | |
bool | send (const char *command,...) MBED_PRINTF_METHOD(1 |
Sends an AT command. | |
bool | recv (const char *response,...) MBED_SCANF_METHOD(1 |
Receive an AT response. | |
int | putc (char c) |
Write a single byte to the underlying stream. | |
int | getc () |
Get a single byte from the underlying stream. | |
int | write (const char *data, int size) |
Write an array of bytes to the underlying stream. | |
int | read (char *data, int size) |
Read an array of bytes from the underlying stream. | |
int | printf (const char *format,...) MBED_PRINTF_METHOD(1 |
Direct printf to underlying stream. | |
int | scanf (const char *format,...) MBED_SCANF_METHOD(1 |
Direct scanf on underlying stream. | |
void | oob (const char *prefix, mbed::Callback< void()> func) |
Attach a callback for out-of-band data. | |
void | flush () |
Flushes the underlying stream. | |
void | abort () |
Abort current recv. | |
bool | process_oob (void) |
Process out-of-band data. | |
Private Member Functions | |
MBED_DEPRECATED ("Invalid copy construction of a NonCopyable resource.") NonCopyable(const NonCopyable &) | |
NonCopyable copy constructor. | |
MBED_DEPRECATED ("Invalid copy assignment of a NonCopyable resource.") NonCopyable &operator | |
NonCopyable copy assignment operator. | |
MBED_DEPRECATED ("Invalid copy construction of a NonCopyable resource.") NonCopyable(const NonCopyable &) | |
NonCopyable copy constructor. | |
MBED_DEPRECATED ("Invalid copy assignment of a NonCopyable resource.") NonCopyable &operator | |
NonCopyable copy assignment operator. |
Detailed Description
Parser class for parsing AT commands.
Here are some examples:
UARTSerial serial = UARTSerial(D1, D0); ATCmdParser at = ATCmdParser(&serial, "\r\n"); int value; char buffer[100]; at.send("AT") && at.recv("OK"); at.send("AT+CWMODE=%d", 3) && at.recv("OK"); at.send("AT+CWMODE?") && at.recv("+CWMODE:%d\r\nOK", &value); at.recv("+IPD,%d:", &value); at.read(buffer, value); at.recv("OK");
Definition at line 57 of file cmsis/BUILD/mbed/platform/ATCmdParser.h.
Constructor & Destructor Documentation
ATCmdParser | ( | FileHandle * | fh, |
const char * | output_delimiter = "\r" , |
||
int | buffer_size = 256 , |
||
int | timeout = 8000 , |
||
bool | debug = false |
||
) |
Constructor.
- Parameters:
-
fh A FileHandle to the digital interface, used for AT commands output_delimiter End of command-line termination buffer_size Size of internal buffer for transaction timeout Timeout of the connection debug Turns on/off debug output for AT commands
Definition at line 94 of file cmsis/BUILD/mbed/platform/ATCmdParser.h.
~ATCmdParser | ( | ) |
Destructor.
Definition at line 107 of file cmsis/BUILD/mbed/platform/ATCmdParser.h.
ATCmdParser | ( | FileHandle * | fh, |
const char * | output_delimiter = "\r" , |
||
int | buffer_size = 256 , |
||
int | timeout = 8000 , |
||
bool | debug = false |
||
) |
Constructor.
- Parameters:
-
fh A FileHandle to the digital interface, used for AT commands output_delimiter End of command-line termination buffer_size Size of internal buffer for transaction timeout Timeout of the connection debug Turns on/off debug output for AT commands
Definition at line 94 of file platform/ATCmdParser.h.
~ATCmdParser | ( | ) |
Destructor.
Definition at line 107 of file platform/ATCmdParser.h.
Member Function Documentation
void abort | ( | ) |
Abort current recv.
Can be called from out-of-band handler to interrupt the current recv operation.
Definition at line 392 of file ATCmdParser.cpp.
void abort | ( | ) |
Abort current recv.
Can be called from out-of-band handler to interrupt the current recv operation.
void debug_on | ( | uint8_t | on ) |
Allows traces from modem to be turned on or off.
- Parameters:
-
on Set as 1 to turn on traces and 0 to disable traces.
Definition at line 176 of file cmsis/BUILD/mbed/platform/ATCmdParser.h.
void debug_on | ( | uint8_t | on ) |
Allows traces from modem to be turned on or off.
- Parameters:
-
on Set as 1 to turn on traces and 0 to disable traces.
Definition at line 176 of file platform/ATCmdParser.h.
void flush | ( | ) |
Flushes the underlying stream.
Definition at line 75 of file ATCmdParser.cpp.
void flush | ( | ) |
Flushes the underlying stream.
int getc | ( | ) |
Get a single byte from the underlying stream.
- Returns:
- The byte that was read or -1 during a timeout
int getc | ( | ) |
Get a single byte from the underlying stream.
- Returns:
- The byte that was read or -1 during a timeout
Definition at line 60 of file ATCmdParser.cpp.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.5.0" | , |
"Replaced with set_timeout for consistency" | |||
) |
For backward compatibility.
Please use set_timeout(int) API only from now on. Allows timeout to be changed between commands
- Parameters:
-
timeout ATCmdParser APIs (read/write/send/recv ..etc) throw an error if no response is received in `timeout` duration
Definition at line 139 of file cmsis/BUILD/mbed/platform/ATCmdParser.h.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.5.0" | , |
"Replaced with set_delimiter for consistency" | |||
) | const |
For backwards compatibility.
Please use set_delimiter(const char *) API only from now on. Sets string of characters to use as line delimiters
- Parameters:
-
output_delimiter string of characters to use as line delimiters
Definition at line 165 of file cmsis/BUILD/mbed/platform/ATCmdParser.h.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.5.0" | , |
"Replaced with set_timeout for consistency" | |||
) |
For backward compatibility.
Please use set_timeout(int) API only from now on. Allows timeout to be changed between commands
- Parameters:
-
timeout ATCmdParser APIs (read/write/send/recv ..etc) throw an error if no response is received in `timeout` duration
Definition at line 139 of file platform/ATCmdParser.h.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.5.0" | , |
"Replaced with debug_on for consistency" | |||
) |
For backward compatibility.
Allows traces from modem to be turned on or off
- Parameters:
-
on Set as 1 to turn on traces and 0 to disable traces.
Definition at line 189 of file cmsis/BUILD/mbed/platform/ATCmdParser.h.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.5.0" | , |
"Replaced with set_delimiter for consistency" | |||
) | const |
For backwards compatibility.
Please use set_delimiter(const char *) API only from now on. Sets string of characters to use as line delimiters
- Parameters:
-
output_delimiter string of characters to use as line delimiters
Definition at line 165 of file platform/ATCmdParser.h.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.5.0" | , |
"Replaced with debug_on for consistency" | |||
) |
For backward compatibility.
Allows traces from modem to be turned on or off
- Parameters:
-
on Set as 1 to turn on traces and 0 to disable traces.
Definition at line 189 of file platform/ATCmdParser.h.
void oob | ( | const char * | prefix, |
mbed::Callback< void()> | func | ||
) |
Attach a callback for out-of-band data.
- Parameters:
-
prefix String on when to initiate callback func Callback to call when string is read
- Note:
- out-of-band data is only processed during a scanf call
void oob | ( | const char * | prefix, |
mbed::Callback< void()> | func | ||
) |
Attach a callback for out-of-band data.
- Parameters:
-
prefix String on when to initiate callback func Callback to call when string is read
- Note:
- out-of-band data is only processed during a scanf call
int printf | ( | const char * | format, |
... | |||
) |
Direct printf to underlying stream.
- See also:
- printf
- Parameters:
-
format Format string to pass to printf ... Variable arguments to printf
- Returns:
- number of bytes written or -1 on failure
int printf | ( | const char * | format, |
... | |||
) |
Direct printf to underlying stream.
- See also:
- printf
- Parameters:
-
format Format string to pass to printf ... Variable arguments to printf
- Returns:
- number of bytes written or -1 on failure
Definition at line 345 of file ATCmdParser.cpp.
bool process_oob | ( | void | ) |
Process out-of-band data.
Process out-of-band data in the receive buffer. This function returns immediately if there is no data to process.
- Returns:
- true if out-of-band data processed, false otherwise
Definition at line 397 of file ATCmdParser.cpp.
bool process_oob | ( | void | ) |
Process out-of-band data.
Process out-of-band data in the receive buffer. This function returns immediately if there is no data to process.
- Returns:
- true if out-of-band data processed, false otherwise
int putc | ( | char | c ) |
Write a single byte to the underlying stream.
- Parameters:
-
c The byte to write
- Returns:
- The byte that was written or -1 during a timeout
int putc | ( | char | c ) |
Write a single byte to the underlying stream.
- Parameters:
-
c The byte to write
- Returns:
- The byte that was written or -1 during a timeout
Definition at line 46 of file ATCmdParser.cpp.
int read | ( | char * | data, |
int | size | ||
) |
Read an array of bytes from the underlying stream.
- Parameters:
-
data The buffer for filling the read bytes size Number of bytes to read
- Returns:
- number of bytes read or -1 on failure
int read | ( | char * | data, |
int | size | ||
) |
Read an array of bytes from the underlying stream.
- Parameters:
-
data The buffer for filling the read bytes size Number of bytes to read
- Returns:
- number of bytes read or -1 on failure
Definition at line 96 of file ATCmdParser.cpp.
bool recv | ( | const char * | response, |
... | |||
) |
Receive an AT response.
Receives a formatted response using scanf style formatting
- See also:
- scanf
Responses are parsed line at a time. Any received data that does not match the response is ignored until a timeout occurs.
- Parameters:
-
response scanf-like format string of response to expect ... all scanf-like arguments to extract from response
- Returns:
- true only if response is successfully matched
Definition at line 372 of file ATCmdParser.cpp.
bool recv | ( | const char * | response, |
... | |||
) |
Receive an AT response.
Receives a formatted response using scanf style formatting
- See also:
- scanf
Responses are parsed line at a time. Any received data that does not match the response is ignored until a timeout occurs.
- Parameters:
-
response scanf-like format string of response to expect ... all scanf-like arguments to extract from response
- Returns:
- true only if response is successfully matched
int scanf | ( | const char * | format, |
... | |||
) |
Direct scanf on underlying stream.
- See also:
- scanf
- Parameters:
-
format Format string to pass to scanf ... Variable arguments to scanf
- Returns:
- number of bytes read or -1 on failure
Definition at line 354 of file ATCmdParser.cpp.
int scanf | ( | const char * | format, |
... | |||
) |
Direct scanf on underlying stream.
- See also:
- scanf
- Parameters:
-
format Format string to pass to scanf ... Variable arguments to scanf
- Returns:
- number of bytes read or -1 on failure
bool send | ( | const char * | command, |
... | |||
) |
Sends an AT command.
Sends a formatted command using printf style formatting
- See also:
- printf
- Parameters:
-
command printf-like format string of command to send which is appended with a newline ... all printf-like arguments to insert into command
- Returns:
- true only if command is successfully sent
bool send | ( | const char * | command, |
... | |||
) |
Sends an AT command.
Sends a formatted command using printf style formatting
- See also:
- printf
- Parameters:
-
command printf-like format string of command to send which is appended with a newline ... all printf-like arguments to insert into command
- Returns:
- true only if command is successfully sent
Definition at line 363 of file ATCmdParser.cpp.
void set_delimiter | ( | const char * | output_delimiter ) |
Sets string of characters to use as line delimiters.
- Parameters:
-
output_delimiter String of characters to use as line delimiters
Definition at line 150 of file cmsis/BUILD/mbed/platform/ATCmdParser.h.
void set_delimiter | ( | const char * | output_delimiter ) |
Sets string of characters to use as line delimiters.
- Parameters:
-
output_delimiter String of characters to use as line delimiters
Definition at line 150 of file platform/ATCmdParser.h.
void set_timeout | ( | int | timeout ) |
Allows timeout to be changed between commands.
- Parameters:
-
timeout ATCmdParser APIs (read/write/send/recv ..etc) throw an error if no response is received in `timeout` duration
Definition at line 123 of file cmsis/BUILD/mbed/platform/ATCmdParser.h.
void set_timeout | ( | int | timeout ) |
Allows timeout to be changed between commands.
- Parameters:
-
timeout ATCmdParser APIs (read/write/send/recv ..etc) throw an error if no response is received in `timeout` duration
Definition at line 123 of file platform/ATCmdParser.h.
int write | ( | const char * | data, |
int | size | ||
) |
Write an array of bytes to the underlying stream.
- Parameters:
-
data The array of bytes to write size Number of bytes to write
- Returns:
- number of bytes written or -1 on failure
int write | ( | const char * | data, |
int | size | ||
) |
Write an array of bytes to the underlying stream.
- Parameters:
-
data The array of bytes to write size Number of bytes to write
- Returns:
- number of bytes written or -1 on failure
Definition at line 85 of file ATCmdParser.cpp.
Generated on Tue Jul 12 2022 20:41:17 by
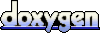