USBHost library. NOTE: This library is only officially supported on the LPC1768 platform. For more information, please see the handbook page.
Dependencies: FATFileSystem mbed-rtos
Dependents: BTstack WallbotWii SD to Flash Data Transfer USBHost-MSD_HelloWorld ... more
USBHostSerial.h
00001 /* mbed USBHost Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef USBHOSTSERIAL_H 00018 #define USBHOSTSERIAL_H 00019 00020 #include "USBHostConf.h" 00021 00022 #if USBHOST_SERIAL 00023 00024 #include "USBHost.h" 00025 #include "Stream.h" 00026 #include "MtxCircBuffer.h" 00027 #include "Callback.h" 00028 00029 /** 00030 * A class to communicate a USB virtual serial port 00031 */ 00032 class USBHostSerialPort : public Stream { 00033 public: 00034 /** 00035 * Constructor 00036 */ 00037 USBHostSerialPort(); 00038 00039 enum IrqType { 00040 RxIrq, 00041 TxIrq 00042 }; 00043 00044 enum Parity { 00045 None = 0, 00046 Odd, 00047 Even, 00048 Mark, 00049 Space 00050 }; 00051 00052 void connect(USBHost* _host, USBDeviceConnected * _dev, 00053 uint8_t _serial_intf, USBEndpoint* _bulk_in, USBEndpoint* _bulk_out); 00054 00055 /** 00056 * Check the number of bytes available. 00057 * 00058 * @returns the number of bytes available 00059 */ 00060 uint8_t available(); 00061 00062 /** 00063 * Attach a member function to call when a packet is received. 00064 * 00065 * @param tptr pointer to the object to call the member function on 00066 * @param mptr pointer to the member function to be called 00067 * @param irq irq type 00068 */ 00069 template<typename T> 00070 inline void attach(T* tptr, void (T::*mptr)(void), IrqType irq = RxIrq) { 00071 if ((mptr != NULL) && (tptr != NULL)) { 00072 if (irq == RxIrq) { 00073 rx.attach(tptr, mptr); 00074 } else { 00075 tx.attach(tptr, mptr); 00076 } 00077 } 00078 } 00079 00080 /** 00081 * Attach a callback called when a packet is received 00082 * 00083 * @param ptr function pointer 00084 */ 00085 inline void attach(void (*fn)(void), IrqType irq = RxIrq) { 00086 if (fn != NULL) { 00087 if (irq == RxIrq) { 00088 rx.attach(fn); 00089 } else { 00090 tx.attach(fn); 00091 } 00092 } 00093 } 00094 00095 /** Set the baud rate of the serial port 00096 * 00097 * @param baudrate The baudrate of the serial port (default = 9600). 00098 */ 00099 void baud(int baudrate = 9600); 00100 00101 /** Set the transmission format used by the Serial port 00102 * 00103 * @param bits The number of bits in a word (default = 8) 00104 * @param parity The parity used (USBHostSerialPort::None, USBHostSerialPort::Odd, USBHostSerialPort::Even, USBHostSerialPort::Mark, USBHostSerialPort::Space; default = USBHostSerialPort::None) 00105 * @param stop The number of stop bits (1 or 2; default = 1) 00106 */ 00107 void format(int bits = 8, Parity parity = USBHostSerialPort::None, int stop_bits = 1); 00108 virtual int writeBuf(const char* b, int s); 00109 virtual int readBuf(char* b, int s); 00110 00111 protected: 00112 virtual int _getc(); 00113 virtual int _putc(int c); 00114 00115 private: 00116 USBHost * host; 00117 USBDeviceConnected * dev; 00118 00119 USBEndpoint * bulk_in; 00120 USBEndpoint * bulk_out; 00121 uint32_t size_bulk_in; 00122 uint32_t size_bulk_out; 00123 00124 void init(); 00125 00126 MtxCircBuffer<uint8_t, 128> circ_buf; 00127 00128 uint8_t buf[64]; 00129 00130 typedef struct { 00131 uint32_t baudrate; 00132 uint8_t stop_bits; 00133 uint8_t parity; 00134 uint8_t data_bits; 00135 } PACKED LINE_CODING; 00136 00137 LINE_CODING line_coding; 00138 00139 void rxHandler(); 00140 void txHandler(); 00141 Callback<void()> rx; 00142 Callback<void()> tx; 00143 00144 uint8_t serial_intf; 00145 }; 00146 00147 #if (USBHOST_SERIAL <= 1) 00148 00149 class USBHostSerial : public IUSBEnumerator, public USBHostSerialPort 00150 { 00151 public: 00152 USBHostSerial(); 00153 00154 /** 00155 * Try to connect a serial device 00156 * 00157 * @return true if connection was successful 00158 */ 00159 bool connect(); 00160 00161 void disconnect(); 00162 00163 /** 00164 * Check if a any serial port is connected 00165 * 00166 * @returns true if a serial device is connected 00167 */ 00168 bool connected(); 00169 00170 protected: 00171 USBHost* host; 00172 USBDeviceConnected* dev; 00173 uint8_t port_intf; 00174 int ports_found; 00175 00176 //From IUSBEnumerator 00177 virtual void setVidPid(uint16_t vid, uint16_t pid); 00178 virtual bool parseInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol); //Must return true if the interface should be parsed 00179 virtual bool useEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir); //Must return true if the endpoint will be used 00180 00181 private: 00182 bool dev_connected; 00183 }; 00184 00185 #else // (USBHOST_SERIAL > 1) 00186 00187 class USBHostMultiSerial : public IUSBEnumerator { 00188 public: 00189 USBHostMultiSerial(); 00190 virtual ~USBHostMultiSerial(); 00191 00192 USBHostSerialPort* getPort(int port) 00193 { 00194 return port < USBHOST_SERIAL ? ports[port] : NULL; 00195 } 00196 00197 /** 00198 * Try to connect a serial device 00199 * 00200 * @return true if connection was successful 00201 */ 00202 bool connect(); 00203 00204 void disconnect(); 00205 00206 /** 00207 * Check if a any serial port is connected 00208 * 00209 * @returns true if a serial device is connected 00210 */ 00211 bool connected(); 00212 00213 protected: 00214 USBHost* host; 00215 USBDeviceConnected* dev; 00216 USBHostSerialPort* ports[USBHOST_SERIAL]; 00217 uint8_t port_intf[USBHOST_SERIAL]; 00218 int ports_found; 00219 00220 //From IUSBEnumerator 00221 virtual void setVidPid(uint16_t vid, uint16_t pid); 00222 virtual bool parseInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol); //Must return true if the interface should be parsed 00223 virtual bool useEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir); //Must return true if the endpoint will be used 00224 00225 private: 00226 bool dev_connected; 00227 }; 00228 #endif // (USBHOST_SERIAL <= 1) 00229 00230 #endif 00231 00232 #endif
Generated on Tue Jul 12 2022 13:32:26 by
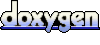