USBHost library. NOTE: This library is only officially supported on the LPC1768 platform. For more information, please see the handbook page.
Dependencies: FATFileSystem mbed-rtos
Dependents: BTstack WallbotWii SD to Flash Data Transfer USBHost-MSD_HelloWorld ... more
USBHostMSD.h
00001 /* mbed USBHost Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef USBHOSTMSD_H 00018 #define USBHOSTMSD_H 00019 00020 #include "USBHostConf.h" 00021 00022 #if USBHOST_MSD 00023 00024 #include "USBHost.h" 00025 #include "FATFileSystem.h" 00026 00027 /** 00028 * A class to communicate a USB flash disk 00029 */ 00030 class USBHostMSD : public IUSBEnumerator, public FATFileSystem { 00031 public: 00032 /** 00033 * Constructor 00034 * 00035 * @param rootdir mount name 00036 */ 00037 USBHostMSD(const char * rootdir); 00038 00039 /** 00040 * Check if a MSD device is connected 00041 * 00042 * @return true if a MSD device is connected 00043 */ 00044 bool connected(); 00045 00046 /** 00047 * Try to connect to a MSD device 00048 * 00049 * @return true if connection was successful 00050 */ 00051 bool connect(); 00052 00053 protected: 00054 //From IUSBEnumerator 00055 virtual void setVidPid(uint16_t vid, uint16_t pid); 00056 virtual bool parseInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol); //Must return true if the interface should be parsed 00057 virtual bool useEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir); //Must return true if the endpoint will be used 00058 00059 // From FATFileSystem 00060 virtual int disk_initialize(); 00061 virtual int disk_status() {return 0;}; 00062 virtual int disk_read(uint8_t* buffer, uint32_t sector, uint32_t count); 00063 virtual int disk_write(const uint8_t* buffer, uint32_t sector, uint32_t count); 00064 virtual int disk_sync() {return 0;}; 00065 virtual uint32_t disk_sectors(); 00066 00067 private: 00068 USBHost * host; 00069 USBDeviceConnected * dev; 00070 bool dev_connected; 00071 USBEndpoint * bulk_in; 00072 USBEndpoint * bulk_out; 00073 uint8_t nb_ep; 00074 00075 // Bulk-only CBW 00076 typedef struct { 00077 uint32_t Signature; 00078 uint32_t Tag; 00079 uint32_t DataLength; 00080 uint8_t Flags; 00081 uint8_t LUN; 00082 uint8_t CBLength; 00083 uint8_t CB[16]; 00084 } PACKED CBW; 00085 00086 // Bulk-only CSW 00087 typedef struct { 00088 uint32_t Signature; 00089 uint32_t Tag; 00090 uint32_t DataResidue; 00091 uint8_t Status; 00092 } PACKED CSW; 00093 00094 CBW cbw; 00095 CSW csw; 00096 00097 int SCSITransfer(uint8_t * cmd, uint8_t cmd_len, int flags, uint8_t * data, uint32_t transfer_len); 00098 int testUnitReady(); 00099 int readCapacity(); 00100 int inquiry(uint8_t lun, uint8_t page_code); 00101 int SCSIRequestSense(); 00102 int dataTransfer(uint8_t * buf, uint32_t block, uint8_t nbBlock, int direction); 00103 int checkResult(uint8_t res, USBEndpoint * ep); 00104 int getMaxLun(); 00105 00106 int blockSize; 00107 uint32_t blockCount; 00108 00109 int msd_intf; 00110 bool msd_device_found; 00111 bool disk_init; 00112 00113 void init(); 00114 00115 }; 00116 00117 #endif 00118 00119 #endif
Generated on Tue Jul 12 2022 13:32:26 by
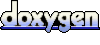