USBHost library. NOTE: This library is only officially supported on the LPC1768 platform. For more information, please see the handbook page.
Dependencies: FATFileSystem mbed-rtos
Dependents: BTstack WallbotWii SD to Flash Data Transfer USBHost-MSD_HelloWorld ... more
USBEndpoint.h
00001 /* mbed USBHost Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef USBENDPOINT_H 00018 #define USBENDPOINT_H 00019 00020 #include "Callback.h" 00021 #include "USBHostTypes.h" 00022 #include "rtos.h" 00023 00024 class USBDeviceConnected; 00025 00026 /** 00027 * USBEndpoint class 00028 */ 00029 class USBEndpoint 00030 { 00031 public: 00032 /** 00033 * Constructor 00034 */ 00035 USBEndpoint() { 00036 #ifdef USBHOST_OTHER 00037 speed = false; 00038 #endif 00039 state = USB_TYPE_FREE; 00040 nextEp = NULL; 00041 }; 00042 00043 /** 00044 * Initialize an endpoint 00045 * 00046 * @param hced hced associated to the endpoint 00047 * @param type endpoint type 00048 * @param dir endpoint direction 00049 * @param size endpoint size 00050 * @param ep_number endpoint number 00051 * @param td_list array of two allocated transfer descriptors 00052 */ 00053 00054 void init(HCED * hced, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir, uint32_t size, uint8_t ep_number, HCTD* td_list[2]); 00055 00056 /** 00057 * Set next token. Warning: only useful for the control endpoint 00058 * 00059 * @param token IN, OUT or SETUP token 00060 */ 00061 void setNextToken(uint32_t token); 00062 00063 /** 00064 * Queue an endpoint 00065 * 00066 * @param endpoint endpoint which will be queued in the linked list 00067 */ 00068 void queueEndpoint(USBEndpoint * endpoint); 00069 00070 00071 /** 00072 * Queue a transfer on the endpoint 00073 */ 00074 USB_TYPE queueTransfer(); 00075 00076 /** 00077 * Unqueue a transfer from the endpoint 00078 * 00079 * @param td hctd which will be unqueued 00080 */ 00081 void unqueueTransfer(volatile HCTD * td); 00082 00083 /** 00084 * Attach a member function to call when a transfer is finished 00085 * 00086 * @param tptr pointer to the object to call the member function on 00087 * @param mptr pointer to the member function to be called 00088 */ 00089 template<typename T> 00090 inline void attach(T* tptr, void (T::*mptr)(void)) { 00091 if((mptr != NULL) && (tptr != NULL)) { 00092 rx.attach(tptr, mptr); 00093 } 00094 } 00095 00096 /** 00097 * Attach a callback called when a transfer is finished 00098 * 00099 * @param fptr function pointer 00100 */ 00101 inline void attach(void (*fptr)(void)) { 00102 if(fptr != NULL) { 00103 rx.attach(fptr); 00104 } 00105 } 00106 00107 /** 00108 * Call the handler associted to the end of a transfer 00109 */ 00110 inline void call() { 00111 if (rx) 00112 rx.call(); 00113 }; 00114 00115 00116 // setters 00117 #ifdef USBHOST_OTHER 00118 void setState(USB_TYPE st); 00119 #else 00120 inline void setState(USB_TYPE st) { state = st; } 00121 #endif 00122 void setState(uint8_t st); 00123 void setDeviceAddress(uint8_t addr); 00124 inline void setLengthTransferred(int len) { transferred = len; }; 00125 void setSpeed(uint8_t speed); 00126 void setSize(uint32_t size); 00127 inline void setDir(ENDPOINT_DIRECTION d) { dir = d; } 00128 inline void setIntfNb(uint8_t intf_nb_) { intf_nb = intf_nb_; }; 00129 00130 // getters 00131 const char * getStateString(); 00132 inline USB_TYPE getState() { return state; } 00133 inline ENDPOINT_TYPE getType() { return type; }; 00134 #ifdef USBHOST_OTHER 00135 inline uint8_t getDeviceAddress() { return device_address; }; 00136 inline uint32_t getSize() { return size; }; 00137 #else 00138 inline uint8_t getDeviceAddress() { return hced->control & 0x7f; }; 00139 inline uint32_t getSize() { return (hced->control >> 16) & 0x3ff; }; 00140 inline volatile HCTD * getHeadTD() { return (volatile HCTD*) ((uint32_t)hced->headTD & ~0xF); }; 00141 #endif 00142 inline int getLengthTransferred() { return transferred; } 00143 inline uint8_t * getBufStart() { return buf_start; } 00144 inline uint8_t getAddress(){ return address; }; 00145 inline volatile HCTD** getTDList() { return td_list; }; 00146 inline volatile HCED * getHCED() { return hced; }; 00147 inline ENDPOINT_DIRECTION getDir() { return dir; } 00148 inline volatile HCTD * getProcessedTD() { return td_current; }; 00149 inline volatile HCTD* getNextTD() { return td_current; }; 00150 inline bool isSetup() { return setup; } 00151 inline USBEndpoint * nextEndpoint() { return (USBEndpoint*)nextEp; }; 00152 inline uint8_t getIntfNb() { return intf_nb; }; 00153 00154 USBDeviceConnected * dev; 00155 00156 Queue<uint8_t, 1> ep_queue; 00157 00158 private: 00159 ENDPOINT_TYPE type; 00160 volatile USB_TYPE state; 00161 ENDPOINT_DIRECTION dir; 00162 #ifdef USBHOST_OTHER 00163 uint32_t size; 00164 uint32_t ep_number; 00165 uint32_t speed; 00166 uint8_t device_address; 00167 #endif 00168 bool setup; 00169 00170 uint8_t address; 00171 00172 int transfer_len; 00173 int transferred; 00174 uint8_t * buf_start; 00175 00176 Callback<void()> rx; 00177 00178 USBEndpoint* nextEp; 00179 00180 // USBEndpoint descriptor 00181 volatile HCED * hced; 00182 00183 volatile HCTD * td_list[2]; 00184 volatile HCTD * td_current; 00185 volatile HCTD * td_next; 00186 00187 uint8_t intf_nb; 00188 00189 }; 00190 00191 #endif
Generated on Tue Jul 12 2022 13:32:26 by
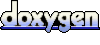