mbed socket API
Dependents: EthernetInterface EthernetInterface_RSF EthernetInterface EthernetInterface ... more
TCPSocketConnection Class Reference
TCP socket connection. More...
#include <TCPSocketConnection.h>
Inherits Socket, and Endpoint.
Public Member Functions | |
TCPSocketConnection () | |
TCP socket connection. | |
int | connect (const char *host, const int port) |
Connects this TCP socket to the server. | |
bool | is_connected (void) |
Check if the socket is connected. | |
int | send (char *data, int length) |
Send data to the remote host. | |
int | send_all (char *data, int length) |
Send all the data to the remote host. | |
int | receive (char *data, int length) |
Receive data from the remote host. | |
int | receive_all (char *data, int length) |
Receive all the data from the remote host. | |
void | set_blocking (bool blocking, unsigned int timeout=1500) |
Set blocking or non-blocking mode of the socket and a timeout on blocking socket operations. | |
int | set_option (int level, int optname, const void *optval, socklen_t optlen) |
Set socket options. | |
int | get_option (int level, int optname, void *optval, socklen_t *optlen) |
Get socket options. | |
int | close (bool shutdown=true) |
Close the socket. | |
void | reset_address (void) |
Reset the address of this endpoint. | |
int | set_address (const char *host, const int port) |
Set the address of this endpoint. | |
char * | get_address (void) |
Get the IP address of this endpoint. | |
int | get_port (void) |
Get the port of this endpoint. | |
Friends | |
class | TCPSocketServer |
Detailed Description
TCP socket connection.
Definition at line 28 of file TCPSocketConnection.h.
Constructor & Destructor Documentation
TCP socket connection.
Definition at line 24 of file TCPSocketConnection.cpp.
Member Function Documentation
int close | ( | bool | shutdown = true ) |
[inherited] |
Close the socket.
- Parameters:
-
shutdown free the left-over data in message queues
Definition at line 72 of file Socket.cpp.
int connect | ( | const char * | host, |
const int | port | ||
) |
Connects this TCP socket to the server.
- Parameters:
-
host The host to connect to. It can either be an IP Address or a hostname that will be resolved with DNS. port The host's port to connect to.
- Returns:
- 0 on success, -1 on failure.
Definition at line 28 of file TCPSocketConnection.cpp.
char * get_address | ( | void | ) | [inherited] |
Get the IP address of this endpoint.
- Returns:
- The IP address of this endpoint.
Definition at line 65 of file Endpoint.cpp.
int get_option | ( | int | level, |
int | optname, | ||
void * | optval, | ||
socklen_t * | optlen | ||
) | [inherited] |
Get socket options.
- Parameters:
-
level stack level (see: lwip/sockets.h) optname option ID optval buffer pointer where to write the option value socklen_t length of the option value
- Returns:
- 0 on success, -1 on failure
Definition at line 48 of file Socket.cpp.
int get_port | ( | void | ) | [inherited] |
Get the port of this endpoint.
- Returns:
- The port of this endpoint
Definition at line 71 of file Endpoint.cpp.
bool is_connected | ( | void | ) |
Check if the socket is connected.
- Returns:
- true if connected, false otherwise.
Definition at line 44 of file TCPSocketConnection.cpp.
int receive | ( | char * | data, |
int | length | ||
) |
Receive data from the remote host.
- Parameters:
-
data The buffer in which to store the data received from the host. length The maximum length of the buffer.
- Returns:
- the number of received bytes on success (>=0) or -1 on failure
Definition at line 92 of file TCPSocketConnection.cpp.
int receive_all | ( | char * | data, |
int | length | ||
) |
Receive all the data from the remote host.
- Parameters:
-
data The buffer in which to store the data received from the host. length The maximum length of the buffer.
- Returns:
- the number of received bytes on success (>=0) or -1 on failure
Definition at line 109 of file TCPSocketConnection.cpp.
void reset_address | ( | void | ) | [inherited] |
Reset the address of this endpoint.
Definition at line 28 of file Endpoint.cpp.
int send | ( | char * | data, |
int | length | ||
) |
Send data to the remote host.
- Parameters:
-
data The buffer to send to the host. length The length of the buffer to send.
- Returns:
- the number of written bytes on success (>=0) or -1 on failure
Definition at line 48 of file TCPSocketConnection.cpp.
int send_all | ( | char * | data, |
int | length | ||
) |
Send all the data to the remote host.
- Parameters:
-
data The buffer to send to the host. length The length of the buffer to send.
- Returns:
- the number of written bytes on success (>=0) or -1 on failure
Definition at line 65 of file TCPSocketConnection.cpp.
int set_address | ( | const char * | host, |
const int | port | ||
) | [inherited] |
Set the address of this endpoint.
- Parameters:
-
host The endpoint address (it can either be an IP Address or a hostname that will be resolved with DNS). port The endpoint port
- Returns:
- 0 on success, -1 on failure (when an hostname cannot be resolved by DNS).
Definition at line 35 of file Endpoint.cpp.
void set_blocking | ( | bool | blocking, |
unsigned int | timeout = 1500 |
||
) | [inherited] |
Set blocking or non-blocking mode of the socket and a timeout on blocking socket operations.
- Parameters:
-
blocking true for blocking mode, false for non-blocking mode. timeout timeout in ms [Default: (1500)ms].
Definition at line 27 of file Socket.cpp.
int set_option | ( | int | level, |
int | optname, | ||
const void * | optval, | ||
socklen_t | optlen | ||
) | [inherited] |
Set socket options.
- Parameters:
-
level stack level (see: lwip/sockets.h) optname option ID optval option value socklen_t length of the option value
- Returns:
- 0 on success, -1 on failure
Definition at line 44 of file Socket.cpp.
Generated on Tue Jul 12 2022 11:05:10 by
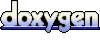