mbed IP library over Ethernet
Dependencies: lwip-eth Socket lwip lwip-sys
Dependents: denki-yohou_b Network-RTOS NTPClient_HelloWorld temp_FIAP ... more
EthernetInterface.cpp
00001 /* EthernetInterface.cpp */ 00002 /* Copyright (C) 2012 mbed.org, MIT License 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 #include "EthernetInterface.h" 00020 00021 #include "lwip/inet.h" 00022 #include "lwip/netif.h" 00023 #include "netif/etharp.h" 00024 #include "lwip/dhcp.h" 00025 #include "eth_arch.h" 00026 #include "lwip/tcpip.h" 00027 00028 #include "mbed.h" 00029 00030 /* TCP/IP and Network Interface Initialisation */ 00031 static struct netif netif; 00032 00033 static char mac_addr[19]; 00034 static char ip_addr[17] = "\0"; 00035 static char gateway[17] = "\0"; 00036 static char networkmask[17] = "\0"; 00037 static bool use_dhcp = false; 00038 00039 static Semaphore tcpip_inited(0); 00040 static Semaphore netif_linked(0); 00041 static Semaphore netif_up(0); 00042 00043 static void tcpip_init_done(void *arg) { 00044 tcpip_inited.release(); 00045 } 00046 00047 static void netif_link_callback(struct netif *netif) { 00048 if (netif_is_link_up(netif)) { 00049 netif_linked.release(); 00050 } 00051 } 00052 00053 static void netif_status_callback(struct netif *netif) { 00054 if (netif_is_up(netif)) { 00055 strcpy(ip_addr, inet_ntoa(netif->ip_addr)); 00056 strcpy(gateway, inet_ntoa(netif->gw)); 00057 strcpy(networkmask, inet_ntoa(netif->netmask)); 00058 netif_up.release(); 00059 } 00060 } 00061 00062 static void init_netif(ip_addr_t *ipaddr, ip_addr_t *netmask, ip_addr_t *gw) { 00063 tcpip_init(tcpip_init_done, NULL); 00064 tcpip_inited.wait(); 00065 00066 memset((void*) &netif, 0, sizeof(netif)); 00067 netif_add(&netif, ipaddr, netmask, gw, NULL, eth_arch_enetif_init, tcpip_input); 00068 netif_set_default(&netif); 00069 00070 netif_set_link_callback (&netif, netif_link_callback); 00071 netif_set_status_callback(&netif, netif_status_callback); 00072 } 00073 00074 static void set_mac_address(void) { 00075 #if (MBED_MAC_ADDRESS_SUM != MBED_MAC_ADDR_INTERFACE) 00076 snprintf(mac_addr, 19, "%02x:%02x:%02x:%02x:%02x:%02x", MBED_MAC_ADDR_0, MBED_MAC_ADDR_1, MBED_MAC_ADDR_2, 00077 MBED_MAC_ADDR_3, MBED_MAC_ADDR_4, MBED_MAC_ADDR_5); 00078 #else 00079 char mac[6]; 00080 mbed_mac_address(mac); 00081 snprintf(mac_addr, 19, "%02x:%02x:%02x:%02x:%02x:%02x", mac[0], mac[1], mac[2], mac[3], mac[4], mac[5]); 00082 #endif 00083 } 00084 00085 int EthernetInterface::init() { 00086 use_dhcp = true; 00087 set_mac_address(); 00088 init_netif(NULL, NULL, NULL); 00089 return 0; 00090 } 00091 00092 int EthernetInterface::init(const char* ip, const char* mask, const char* gateway) { 00093 use_dhcp = false; 00094 00095 set_mac_address(); 00096 strcpy(ip_addr, ip); 00097 00098 ip_addr_t ip_n, mask_n, gateway_n; 00099 inet_aton(ip, &ip_n); 00100 inet_aton(mask, &mask_n); 00101 inet_aton(gateway, &gateway_n); 00102 init_netif(&ip_n, &mask_n, &gateway_n); 00103 00104 return 0; 00105 } 00106 00107 int EthernetInterface::connect(unsigned int timeout_ms) { 00108 eth_arch_enable_interrupts(); 00109 00110 int inited; 00111 if (use_dhcp) { 00112 dhcp_start(&netif); 00113 00114 // Wait for an IP Address 00115 // -1: error, 0: timeout 00116 inited = netif_up.wait(timeout_ms); 00117 } else { 00118 netif_set_up(&netif); 00119 00120 // Wait for the link up 00121 inited = netif_linked.wait(timeout_ms); 00122 } 00123 00124 return (inited > 0) ? (0) : (-1); 00125 } 00126 00127 int EthernetInterface::disconnect() { 00128 if (use_dhcp) { 00129 dhcp_release(&netif); 00130 dhcp_stop(&netif); 00131 } else { 00132 netif_set_down(&netif); 00133 } 00134 00135 eth_arch_disable_interrupts(); 00136 00137 return 0; 00138 } 00139 00140 char* EthernetInterface::getMACAddress() { 00141 return mac_addr; 00142 } 00143 00144 char* EthernetInterface::getIPAddress() { 00145 return ip_addr; 00146 } 00147 00148 char* EthernetInterface::getGateway() { 00149 return gateway; 00150 } 00151 00152 char* EthernetInterface::getNetworkMask() { 00153 return networkmask; 00154 } 00155 00156
Generated on Tue Jul 12 2022 12:22:09 by
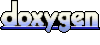