
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
test_udp.c
00001 #include "test_udp.h" 00002 00003 #include "lwip/udp.h" 00004 #include "lwip/stats.h" 00005 00006 #if !LWIP_STATS || !UDP_STATS || !MEMP_STATS 00007 #error "This tests needs UDP- and MEMP-statistics enabled" 00008 #endif 00009 00010 /* Helper functions */ 00011 static void 00012 udp_remove_all(void) 00013 { 00014 struct udp_pcb *pcb = udp_pcbs; 00015 struct udp_pcb *pcb2; 00016 00017 while(pcb != NULL) { 00018 pcb2 = pcb; 00019 pcb = pcb->next; 00020 udp_remove(pcb2); 00021 } 00022 fail_unless(MEMP_STATS_GET(used, MEMP_UDP_PCB) == 0); 00023 } 00024 00025 /* Setups/teardown functions */ 00026 00027 static void 00028 udp_setup(void) 00029 { 00030 udp_remove_all(); 00031 } 00032 00033 static void 00034 udp_teardown(void) 00035 { 00036 udp_remove_all(); 00037 } 00038 00039 00040 /* Test functions */ 00041 00042 START_TEST(test_udp_new_remove) 00043 { 00044 struct udp_pcb* pcb; 00045 LWIP_UNUSED_ARG(_i); 00046 00047 fail_unless(MEMP_STATS_GET(used, MEMP_UDP_PCB) == 0); 00048 00049 pcb = udp_new(); 00050 fail_unless(pcb != NULL); 00051 if (pcb != NULL) { 00052 fail_unless(MEMP_STATS_GET(used, MEMP_UDP_PCB) == 1); 00053 udp_remove(pcb); 00054 fail_unless(MEMP_STATS_GET(used, MEMP_UDP_PCB) == 0); 00055 } 00056 } 00057 END_TEST 00058 00059 00060 /** Create the suite including all tests for this module */ 00061 Suite * 00062 udp_suite(void) 00063 { 00064 testfunc tests[] = { 00065 TESTFUNC(test_udp_new_remove), 00066 }; 00067 return create_suite("UDP", tests, sizeof(tests)/sizeof(testfunc), udp_setup, udp_teardown); 00068 }
Generated on Sun Jul 17 2022 08:25:32 by
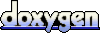