
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
test_coap_message_handler.c
00001 /* 00002 * Copyright (c) 2015-2017 ARM Limited. All Rights Reserved. 00003 */ 00004 #include "test_coap_message_handler.h" 00005 #include <string.h> 00006 #include "coap_message_handler.h" 00007 #include "sn_coap_protocol_stub.h" 00008 #include "nsdynmemLIB_stub.h" 00009 #include "sn_coap_builder_stub.h" 00010 #include "sn_coap_parser_stub.h" 00011 #include "socket_api.h" 00012 00013 int retCounter = 0; 00014 int retValue = 0; 00015 00016 static void *own_alloc(uint16_t size) 00017 { 00018 if( retCounter > 0 ){ 00019 retCounter--; 00020 return malloc(size); 00021 } 00022 return NULL; 00023 } 00024 00025 static void own_free(void *ptr) 00026 { 00027 if (ptr) { 00028 free(ptr); 00029 } 00030 } 00031 00032 static uint8_t coap_tx_function(uint8_t *data_ptr, uint16_t data_len, sn_nsdl_addr_s *address_ptr, void *param) 00033 { 00034 return 0; 00035 } 00036 00037 int resp_recv(int8_t service_id, uint16_t msg_id, sn_coap_hdr_s *response_ptr){ 00038 return retValue; 00039 } 00040 00041 int16_t process_cb(int8_t a, sn_coap_hdr_s *b, coap_transaction_t *c) 00042 { 00043 return retValue; 00044 } 00045 00046 bool test_coap_message_handler_init() 00047 { 00048 if( NULL != coap_message_handler_init(NULL, NULL, NULL) ) 00049 return false; 00050 if( NULL != coap_message_handler_init(&own_alloc, NULL, NULL) ) 00051 return false; 00052 if( NULL != coap_message_handler_init(&own_alloc, &own_free, NULL) ) 00053 return false; 00054 if( NULL != coap_message_handler_init(&own_alloc, &own_free, &coap_tx_function) ) 00055 return false; 00056 retCounter = 1; 00057 sn_coap_protocol_stub.expectedCoap = NULL; 00058 if( NULL != coap_message_handler_init(&own_alloc, &own_free, &coap_tx_function) ) 00059 return false; 00060 retCounter = 1; 00061 sn_coap_protocol_stub.expectedCoap = (struct coap_s*)malloc(sizeof(struct coap_s)); 00062 memset(sn_coap_protocol_stub.expectedCoap, 0, sizeof(struct coap_s)); 00063 coap_msg_handler_t *handle = coap_message_handler_init(&own_alloc, &own_free, &coap_tx_function); 00064 if( NULL == handle ) 00065 return false; 00066 free(sn_coap_protocol_stub.expectedCoap); 00067 sn_coap_protocol_stub.expectedCoap = NULL; 00068 free(handle); 00069 return true; 00070 } 00071 00072 bool test_coap_message_handler_destroy() 00073 { 00074 if( -1 != coap_message_handler_destroy(NULL) ) 00075 return false; 00076 retCounter = 1; 00077 sn_coap_protocol_stub.expectedCoap = (struct coap_s*)malloc(sizeof(struct coap_s)); 00078 memset(sn_coap_protocol_stub.expectedCoap, 0, sizeof(struct coap_s)); 00079 coap_msg_handler_t *handle = coap_message_handler_init(&own_alloc, &own_free, &coap_tx_function); 00080 00081 if( 0 != coap_message_handler_destroy(handle) ) 00082 return false; 00083 00084 free(sn_coap_protocol_stub.expectedCoap); 00085 return true; 00086 } 00087 00088 bool test_coap_message_handler_find_transaction() 00089 { 00090 if( NULL != coap_message_handler_find_transaction(NULL, 0)) 00091 return false; 00092 retCounter = 1; 00093 sn_coap_protocol_stub.expectedCoap = (struct coap_s*)malloc(sizeof(struct coap_s)); 00094 memset(sn_coap_protocol_stub.expectedCoap, 0, sizeof(struct coap_s)); 00095 coap_msg_handler_t *handle = coap_message_handler_init(&own_alloc, &own_free, &coap_tx_function); 00096 00097 uint8_t buf[16]; 00098 memset(&buf, 1, 16); 00099 char uri[3]; 00100 uri[0] = "r"; 00101 uri[1] = "s"; 00102 uri[2] = "\0"; 00103 00104 sn_coap_builder_stub.expectedUint16 = 1; 00105 nsdynmemlib_stub.returnCounter = 3; 00106 if( 2 != coap_message_handler_request_send(handle, 3, 0, buf, 24, 1, 2, &uri, 4, NULL, 0, &resp_recv)) 00107 return false; 00108 00109 if( NULL == coap_message_handler_find_transaction(&buf, 24)) 00110 return false; 00111 00112 free(sn_coap_protocol_stub.expectedCoap); 00113 sn_coap_protocol_stub.expectedCoap = NULL; 00114 coap_message_handler_destroy(handle); 00115 return true; 00116 } 00117 00118 bool test_coap_message_handler_coap_msg_process() 00119 { 00120 uint8_t buf[16]; 00121 memset(&buf, 1, 16); 00122 if( -1 != coap_message_handler_coap_msg_process(NULL, 0, buf, 22, ns_in6addr_any, NULL, 0, NULL)) 00123 return false; 00124 00125 retCounter = 1; 00126 sn_coap_protocol_stub.expectedCoap = (struct coap_s*)malloc(sizeof(struct coap_s)); 00127 memset(sn_coap_protocol_stub.expectedCoap, 0, sizeof(struct coap_s)); 00128 coap_msg_handler_t *handle = coap_message_handler_init(&own_alloc, &own_free, &coap_tx_function); 00129 00130 sn_coap_protocol_stub.expectedHeader = NULL; 00131 if( -1 != coap_message_handler_coap_msg_process(handle, 0, buf, 22, ns_in6addr_any, NULL, 0, process_cb)) 00132 return false; 00133 00134 sn_coap_protocol_stub.expectedHeader = (sn_coap_hdr_s *)malloc(sizeof(sn_coap_hdr_s)); 00135 memset(sn_coap_protocol_stub.expectedHeader, 0, sizeof(sn_coap_hdr_s)); 00136 sn_coap_protocol_stub.expectedHeader->coap_status = 66; 00137 if( -1 != coap_message_handler_coap_msg_process(handle, 0, buf, 22, ns_in6addr_any, NULL, 0, process_cb)) 00138 return false; 00139 00140 sn_coap_protocol_stub.expectedHeader = (sn_coap_hdr_s *)malloc(sizeof(sn_coap_hdr_s)); 00141 memset(sn_coap_protocol_stub.expectedHeader, 0, sizeof(sn_coap_hdr_s)); 00142 sn_coap_protocol_stub.expectedHeader->coap_status = COAP_STATUS_OK; 00143 sn_coap_protocol_stub.expectedHeader->msg_code = 1; 00144 retValue = 0; 00145 if( 0 != coap_message_handler_coap_msg_process(handle, 0, buf, 22, ns_in6addr_any, NULL, 0, process_cb)) 00146 return false; 00147 00148 nsdynmemlib_stub.returnCounter = 1; 00149 retValue = -1; 00150 if( -1 != coap_message_handler_coap_msg_process(handle, 0, buf, 22, ns_in6addr_any, NULL, 0, process_cb)) 00151 return false; 00152 00153 sn_coap_protocol_stub.expectedHeader = (sn_coap_hdr_s *)malloc(sizeof(sn_coap_hdr_s)); 00154 memset(sn_coap_protocol_stub.expectedHeader, 0, sizeof(sn_coap_hdr_s)); 00155 sn_coap_protocol_stub.expectedHeader->coap_status = COAP_STATUS_OK; 00156 sn_coap_protocol_stub.expectedHeader->msg_code = 333; 00157 00158 if( -1 != coap_message_handler_coap_msg_process(handle, 0, buf, 22, ns_in6addr_any, NULL, 0, process_cb)) 00159 return false; 00160 00161 sn_coap_protocol_stub.expectedHeader = (sn_coap_hdr_s *)malloc(sizeof(sn_coap_hdr_s)); 00162 memset(sn_coap_protocol_stub.expectedHeader, 0, sizeof(sn_coap_hdr_s)); 00163 sn_coap_protocol_stub.expectedHeader->coap_status = COAP_STATUS_OK; 00164 sn_coap_protocol_stub.expectedHeader->msg_code = 333; 00165 00166 char uri[3]; 00167 uri[0] = "r"; 00168 uri[1] = "s"; 00169 uri[2] = "\0"; 00170 00171 sn_coap_builder_stub.expectedUint16 = 1; 00172 nsdynmemlib_stub.returnCounter = 3; 00173 if( 2 != coap_message_handler_request_send(handle, 3, 0, buf, 24, 1, 2, &uri, 4, NULL, 0, &resp_recv)) 00174 return false; 00175 00176 sn_coap_protocol_stub.expectedHeader->msg_id = 2; 00177 // sn_coap_protocol_stub.expectedHeader->token_ptr = (uint8_t*)malloc(4); 00178 // memset(sn_coap_protocol_stub.expectedHeader->token_ptr, 1, 4); 00179 if( -1 != coap_message_handler_coap_msg_process(handle, 0, buf, 22, ns_in6addr_any, NULL, 0, process_cb)) 00180 return false; 00181 00182 // free(sn_coap_protocol_stub.expectedHeader->token_ptr); 00183 00184 free(sn_coap_protocol_stub.expectedCoap); 00185 sn_coap_protocol_stub.expectedCoap = NULL; 00186 coap_message_handler_destroy(handle); 00187 return true; 00188 } 00189 00190 bool test_coap_message_handler_request_send() 00191 { 00192 retCounter = 1; 00193 sn_coap_protocol_stub.expectedCoap = (struct coap_s*)malloc(sizeof(struct coap_s)); 00194 memset(sn_coap_protocol_stub.expectedCoap, 0, sizeof(struct coap_s)); 00195 coap_msg_handler_t *handle = coap_message_handler_init(&own_alloc, &own_free, &coap_tx_function); 00196 00197 uint8_t buf[16]; 00198 memset(&buf, 1, 16); 00199 char uri[3]; 00200 uri[0] = "r"; 00201 uri[1] = "s"; 00202 uri[2] = "\0"; 00203 if( 0 != coap_message_handler_request_send(handle, 3, 0, buf, 24, 1, 2, &uri, 4, NULL, 0, NULL)) 00204 return false; 00205 00206 sn_coap_builder_stub.expectedUint16 = 1; 00207 nsdynmemlib_stub.returnCounter = 1; 00208 if( 0 != coap_message_handler_request_send(handle, 3, 0, buf, 24, 1, 2, &uri, 4, NULL, 0, NULL)) 00209 return false; 00210 00211 sn_coap_builder_stub.expectedUint16 = 1; 00212 nsdynmemlib_stub.returnCounter = 3; 00213 if( 0 != coap_message_handler_request_send(handle, 3, 0, buf, 24, 1, 2, &uri, 4, NULL, 0, NULL)) 00214 return false; 00215 00216 sn_coap_builder_stub.expectedUint16 = 1; 00217 nsdynmemlib_stub.returnCounter = 3; 00218 if( 0 != coap_message_handler_request_send(handle, 3, 0, buf, 24, 1, 2, &uri, 4, NULL, 0, NULL)) 00219 return false; 00220 00221 sn_coap_builder_stub.expectedUint16 = 1; 00222 nsdynmemlib_stub.returnCounter = 3; 00223 if( 2 != coap_message_handler_request_send(handle, 3, 0, buf, 24, 1, 2, &uri, 4, NULL, 0, &resp_recv)) 00224 return false; 00225 00226 free(sn_coap_protocol_stub.expectedCoap); 00227 sn_coap_protocol_stub.expectedCoap = NULL; 00228 coap_message_handler_destroy(handle); 00229 return true; 00230 } 00231 00232 bool test_coap_message_handler_request_delete() 00233 { 00234 retCounter = 1; 00235 sn_coap_protocol_stub.expectedCoap = (struct coap_s*)malloc(sizeof(struct coap_s)); 00236 memset(sn_coap_protocol_stub.expectedCoap, 0, sizeof(struct coap_s)); 00237 coap_msg_handler_t *handle = coap_message_handler_init(&own_alloc, &own_free, &coap_tx_function); 00238 00239 uint8_t buf[16]; 00240 memset(&buf, 1, 16); 00241 char uri[3]; 00242 uri[0] = "r"; 00243 uri[1] = "s"; 00244 uri[2] = "\0"; 00245 if( 0 == coap_message_handler_request_delete(NULL, 1, 1)) 00246 return false; 00247 00248 if( 0 == coap_message_handler_request_delete(handle, 1, 1)) 00249 return false; 00250 00251 sn_coap_builder_stub.expectedUint16 = 1; 00252 nsdynmemlib_stub.returnCounter = 3; 00253 if( 2 != coap_message_handler_request_send(handle, 3, 0, buf, 24, 1, 2, &uri, 4, NULL, 0, &resp_recv)) 00254 return false; 00255 00256 if( 0 != coap_message_handler_request_delete(handle, 1, 2)) 00257 return false; 00258 00259 free(sn_coap_protocol_stub.expectedCoap); 00260 sn_coap_protocol_stub.expectedCoap = NULL; 00261 coap_message_handler_destroy(handle); 00262 return true; 00263 } 00264 00265 bool test_coap_message_handler_response_send() 00266 { 00267 if( -1 != coap_message_handler_response_send(NULL, 2, 0, NULL, 1,3,NULL, 0)) 00268 return false; 00269 00270 retCounter = 1; 00271 sn_coap_protocol_stub.expectedCoap = (struct coap_s*)malloc(sizeof(struct coap_s)); 00272 memset(sn_coap_protocol_stub.expectedCoap, 0, sizeof(struct coap_s)); 00273 coap_msg_handler_t *handle = coap_message_handler_init(&own_alloc, &own_free, &coap_tx_function); 00274 sn_coap_hdr_s *header = (sn_coap_hdr_s *)malloc(sizeof(sn_coap_hdr_s)); 00275 memset(header, 0, sizeof(sn_coap_hdr_s)); 00276 00277 if( -2 != coap_message_handler_response_send(handle, 2, 0, header, 1,3,NULL, 0)) 00278 return false; 00279 00280 uint8_t buf[16]; 00281 memset(&buf, 1, 16); 00282 char uri[3]; 00283 uri[0] = "r"; 00284 uri[1] = "s"; 00285 uri[2] = "\0"; 00286 sn_coap_builder_stub.expectedUint16 = 1; 00287 nsdynmemlib_stub.returnCounter = 3; 00288 if( 2 != coap_message_handler_request_send(handle, 3, 0, buf, 24, 1, 2, &uri, 4, NULL, 0, &resp_recv)) 00289 return false; 00290 00291 header->msg_id = 2; 00292 sn_coap_builder_stub.expectedUint16 = 2; 00293 coap_transaction_t * tx = coap_message_handler_find_transaction(&buf, 24); 00294 if( tx ){ 00295 tx->client_request = false; 00296 } 00297 sn_coap_builder_stub.expectedHeader = NULL; 00298 if( -1 != coap_message_handler_response_send(handle, 2, 0, header, 1,3,NULL, 0)) 00299 return false; 00300 00301 sn_coap_builder_stub.expectedHeader = (sn_coap_hdr_s *)malloc(sizeof(sn_coap_hdr_s)); 00302 memset(sn_coap_builder_stub.expectedHeader, 0, sizeof(sn_coap_hdr_s)); 00303 nsdynmemlib_stub.returnCounter = 0; 00304 if( -1 != coap_message_handler_response_send(handle, 2, 0, header, 1,3,NULL, 0)) 00305 return false; 00306 00307 sn_coap_builder_stub.expectedHeader = (sn_coap_hdr_s *)malloc(sizeof(sn_coap_hdr_s)); 00308 memset(sn_coap_builder_stub.expectedHeader, 0, sizeof(sn_coap_hdr_s)); 00309 nsdynmemlib_stub.returnCounter = 1; 00310 if( 0 != coap_message_handler_response_send(handle, 2, 0, header, 1,3,NULL, 0)) 00311 return false; 00312 00313 // free(header); 00314 free(sn_coap_protocol_stub.expectedCoap); 00315 sn_coap_protocol_stub.expectedCoap = NULL; 00316 coap_message_handler_destroy(handle); 00317 return true; 00318 } 00319 00320 bool test_coap_message_handler_exec() 00321 { 00322 if( -1 != coap_message_handler_exec(NULL, 0)) 00323 return false; 00324 retCounter = 1; 00325 sn_coap_protocol_stub.expectedCoap = (struct coap_s*)malloc(sizeof(struct coap_s)); 00326 memset(sn_coap_protocol_stub.expectedCoap, 0, sizeof(struct coap_s)); 00327 coap_msg_handler_t *handle = coap_message_handler_init(&own_alloc, &own_free, &coap_tx_function); 00328 if( 0 != coap_message_handler_exec(handle, 0)) 00329 return false; 00330 00331 free(sn_coap_protocol_stub.expectedCoap); 00332 sn_coap_protocol_stub.expectedCoap = NULL; 00333 coap_message_handler_destroy(handle); 00334 return true; 00335 }
Generated on Sun Jul 17 2022 08:25:32 by
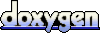