
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
test_coap_connection_handler.c
00001 /* 00002 * Copyright (c) 2015-2017 ARM Limited. All Rights Reserved. 00003 */ 00004 #include "test_coap_connection_handler.h" 00005 #include <string.h> 00006 #include <inttypes.h> 00007 #include "nsdynmemLIB_stub.h" 00008 #include "mbedtls/platform.h" 00009 #include "mbedtls/ssl.h" 00010 #include "coap_connection_handler.h" 00011 #include "coap_security_handler_stub.h" 00012 #include "ns_timer_stub.h" 00013 #include "socket_api.h" 00014 #include "socket_api_stub.h" 00015 #include "net_interface.h" 00016 00017 int send_to_sock_cb(int8_t socket_id, uint8_t address, uint16_t port, const unsigned char *a, int b) 00018 { 00019 return 1; 00020 } 00021 00022 int receive_from_sock_cb(int8_t socket_id, uint8_t address, uint16_t port, unsigned char *a, int b) 00023 { 00024 return 1; 00025 } 00026 00027 int get_passwd_cb(int8_t socket_id, uint8_t address, uint16_t port, uint8_t *pw_ptr, uint8_t *pw_len) 00028 { 00029 return 0; 00030 } 00031 00032 void sec_done_cb(int8_t socket_id, uint8_t address[static 16], uint16_t port, uint8_t keyblock[static 40]) 00033 { 00034 return 1; 00035 } 00036 00037 bool test_connection_handler_create() 00038 { 00039 coap_security_handler_stub.counter = -1; 00040 if( NULL != connection_handler_create(NULL, NULL, NULL, NULL) ) 00041 return false; 00042 00043 if( NULL != connection_handler_create(&receive_from_sock_cb, NULL, NULL, NULL) ) 00044 return false; 00045 00046 nsdynmemlib_stub.returnCounter = 1; 00047 coap_conn_handler_t *handler = connection_handler_create(&receive_from_sock_cb, NULL, NULL, NULL); 00048 if( NULL == handler ) 00049 return false; 00050 ns_dyn_mem_free(handler); 00051 return true; 00052 } 00053 00054 bool test_connection_handler_destroy() 00055 { 00056 coap_security_handler_stub.counter = -1; 00057 nsdynmemlib_stub.returnCounter = 1; 00058 coap_conn_handler_t *handler = connection_handler_create(&receive_from_sock_cb, NULL, NULL, NULL); 00059 00060 connection_handler_destroy(handler, false); 00061 return true; 00062 } 00063 00064 bool test_coap_connection_handler_open_connection() 00065 { 00066 coap_security_handler_stub.counter = -1; 00067 nsdynmemlib_stub.returnCounter = 1; 00068 coap_conn_handler_t *handler = connection_handler_create(&receive_from_sock_cb, NULL, NULL, NULL); 00069 00070 if( -1 != coap_connection_handler_open_connection(NULL, 0,false,false,false,false) ) 00071 return false; 00072 00073 if( -1 != coap_connection_handler_open_connection(handler, 0,false,false,false,false) ) 00074 return false; 00075 00076 ns_dyn_mem_free(handler); 00077 nsdynmemlib_stub.returnCounter = 1; 00078 handler = connection_handler_create(&receive_from_sock_cb, &send_to_sock_cb, NULL, NULL); 00079 if( -1 != coap_connection_handler_open_connection(handler, 0,true,true,true,false) ) 00080 return false; 00081 00082 nsdynmemlib_stub.returnCounter = 2; 00083 if( 0 != coap_connection_handler_open_connection(handler, 0,true,true,true,false) ) 00084 return false; 00085 00086 nsdynmemlib_stub.returnCounter = 2; 00087 if( 0 != coap_connection_handler_open_connection(handler, 22,false,true,true,true) ) 00088 return false; 00089 00090 //open second one 00091 nsdynmemlib_stub.returnCounter = 1; 00092 coap_conn_handler_t *handler2 = connection_handler_create(&receive_from_sock_cb, &send_to_sock_cb, NULL, NULL); 00093 nsdynmemlib_stub.returnCounter = 2; 00094 if( 0 != coap_connection_handler_open_connection(handler2, 22,false,true,true,true) ) 00095 return false; 00096 00097 if( 0 != coap_connection_handler_open_connection(handler, 23,false,false,false,false) ) 00098 return false; 00099 00100 connection_handler_destroy(handler2, false); 00101 connection_handler_destroy(handler, false); 00102 return true; 00103 } 00104 00105 bool test_coap_connection_handler_send_data() 00106 { 00107 coap_security_handler_stub.counter = -1; 00108 if( -1 != coap_connection_handler_send_data(NULL, NULL, ns_in6addr_any, NULL, 0, false)) 00109 return false; 00110 00111 ns_address_t addr; 00112 memset(addr.address, 1, 16); 00113 addr.identifier = 22; 00114 00115 nsdynmemlib_stub.returnCounter = 1; 00116 coap_conn_handler_t *handler = connection_handler_create(&receive_from_sock_cb, &send_to_sock_cb, NULL, NULL); 00117 nsdynmemlib_stub.returnCounter = 2; 00118 if( 0 != coap_connection_handler_open_connection(handler, 22,false,true,false,false) ) 00119 return false; 00120 00121 if( -1 != coap_connection_handler_send_data(handler, &addr, ns_in6addr_any, NULL, 0, true)) 00122 return false; 00123 00124 connection_handler_destroy(handler, false); 00125 00126 coap_security_handler_stub.sec_obj = coap_security_handler_stub_alloc(); 00127 00128 nsdynmemlib_stub.returnCounter = 1; 00129 handler = connection_handler_create(&receive_from_sock_cb, &send_to_sock_cb, NULL, NULL); 00130 nsdynmemlib_stub.returnCounter = 4; 00131 if( 0 != coap_connection_handler_open_connection(handler, 22,false,true,false,false) ) 00132 return false; 00133 00134 if( -1 != coap_connection_handler_send_data(handler, &addr, ns_in6addr_any, NULL, 0, true)) 00135 return false; 00136 00137 if( -1 != coap_connection_handler_send_data(handler, &addr, ns_in6addr_any, NULL, 0, true)) 00138 return false; 00139 00140 connection_handler_destroy(handler, false); 00141 00142 free(coap_security_handler_stub.sec_obj); 00143 coap_security_handler_stub.sec_obj = NULL; 00144 00145 //NON SECURE HERE --> 00146 nsdynmemlib_stub.returnCounter = 1; 00147 handler = connection_handler_create(&receive_from_sock_cb, &send_to_sock_cb, NULL, NULL); 00148 nsdynmemlib_stub.returnCounter = 2; 00149 if( 0 != coap_connection_handler_open_connection(handler, 22,false,false,false,false) ) 00150 return false; 00151 00152 00153 if( 1 != coap_connection_handler_send_data(handler, &addr, ns_in6addr_any, NULL, 0, true)) 00154 return false; 00155 connection_handler_destroy(handler ,false); 00156 00157 nsdynmemlib_stub.returnCounter = 1; 00158 handler = connection_handler_create(&receive_from_sock_cb, &send_to_sock_cb, NULL, NULL); 00159 nsdynmemlib_stub.returnCounter = 2; 00160 if( 0 != coap_connection_handler_open_connection(handler, 22,false,false,true,false) ) 00161 return false; 00162 00163 socket_api_stub.int8_value = 7; 00164 if( 7 != coap_connection_handler_send_data(handler, &addr, ns_in6addr_any, NULL, 0, true)) 00165 return false; 00166 connection_handler_destroy(handler, false); 00167 00168 //<-- NON SECURE HERE 00169 00170 return true; 00171 } 00172 00173 bool test_coap_connection_handler_virtual_recv() 00174 { 00175 coap_security_handler_stub.counter = -1; 00176 uint8_t buf[16]; 00177 memset(&buf, 1, 16); 00178 if( -1 != coap_connection_handler_virtual_recv(NULL,buf, 12, NULL, 0) ) 00179 return false; 00180 00181 nsdynmemlib_stub.returnCounter = 1; 00182 coap_conn_handler_t *handler = connection_handler_create(&receive_from_sock_cb, &send_to_sock_cb, NULL, NULL); 00183 nsdynmemlib_stub.returnCounter = 2; 00184 if( 0 != coap_connection_handler_open_connection(handler, 22,false,true,true,false) ) 00185 return false; 00186 00187 if( -1 != coap_connection_handler_virtual_recv(handler,buf, 12, NULL, 0) ) 00188 return false; 00189 00190 nsdynmemlib_stub.returnCounter = 1; 00191 if( -1 != coap_connection_handler_virtual_recv(handler,buf, 12, NULL, 0) ) 00192 return false; 00193 00194 ns_timer_stub.int8_value = 0; 00195 nsdynmemlib_stub.returnCounter = 3; 00196 if( -1 != coap_connection_handler_virtual_recv(handler,buf, 12, &buf, 1) ) 00197 return false; 00198 00199 //handler->socket->data still in memory 00200 coap_security_handler_stub.sec_obj = coap_security_handler_stub_alloc(); 00201 00202 ns_timer_stub.int8_value = -1; 00203 nsdynmemlib_stub.returnCounter = 3; 00204 if( -1 != coap_connection_handler_virtual_recv(handler,buf, 12, &buf, 1) ) 00205 return false; 00206 00207 ns_timer_stub.int8_value = 0; 00208 nsdynmemlib_stub.returnCounter = 3; 00209 if( -1 != coap_connection_handler_virtual_recv(handler,buf, 12, &buf, 1) ) 00210 return false; 00211 00212 connection_handler_destroy(handler, false); 00213 00214 nsdynmemlib_stub.returnCounter = 1; 00215 coap_conn_handler_t *handler2 = connection_handler_create(&receive_from_sock_cb, &send_to_sock_cb, &get_passwd_cb, &sec_done_cb); 00216 nsdynmemlib_stub.returnCounter = 2; 00217 if( 0 != coap_connection_handler_open_connection(handler2, 24,false,true,true,false) ) 00218 return false; 00219 00220 nsdynmemlib_stub.returnCounter = 3; 00221 if( 0 != coap_connection_handler_virtual_recv(handler2,buf, 12, &buf, 1) ) 00222 return false; 00223 00224 nsdynmemlib_stub.returnCounter = 1; 00225 coap_security_handler_stub.int_value = 0; 00226 if( 0 != coap_connection_handler_virtual_recv(handler2,buf, 12, &buf, 1) ) 00227 return false; 00228 00229 nsdynmemlib_stub.returnCounter = 1; 00230 if( 0 != coap_connection_handler_virtual_recv(handler2,buf, 12, &buf, 1) ) 00231 return false; 00232 00233 nsdynmemlib_stub.returnCounter = 1; 00234 coap_security_handler_stub.int_value = MBEDTLS_ERR_SSL_PEER_CLOSE_NOTIFY; 00235 if( 0 != coap_connection_handler_virtual_recv(handler2,buf, 12, &buf, 1) ) 00236 return false; 00237 00238 connection_handler_destroy(handler2, false); 00239 00240 free(coap_security_handler_stub.sec_obj); 00241 coap_security_handler_stub.sec_obj = NULL; 00242 00243 nsdynmemlib_stub.returnCounter = 1; 00244 coap_conn_handler_t *handler3 = connection_handler_create(&receive_from_sock_cb, &send_to_sock_cb, &get_passwd_cb, &sec_done_cb); 00245 nsdynmemlib_stub.returnCounter = 2; 00246 if( 0 != coap_connection_handler_open_connection(handler3, 26,false,false,true,false) ) 00247 return false; 00248 00249 nsdynmemlib_stub.returnCounter = 3; 00250 if( 0 != coap_connection_handler_virtual_recv(handler3,buf, 12, &buf, 1) ) 00251 return false; 00252 00253 connection_handler_destroy(handler3, false); 00254 00255 return true; 00256 } 00257 00258 bool test_coap_connection_handler_socket_belongs_to() 00259 { 00260 coap_security_handler_stub.counter = -1; 00261 if( false != coap_connection_handler_socket_belongs_to(NULL, 2) ) 00262 return false; 00263 00264 socket_api_stub.int8_value = 0; 00265 nsdynmemlib_stub.returnCounter = 1; 00266 coap_conn_handler_t *handler = connection_handler_create(&receive_from_sock_cb, &send_to_sock_cb, NULL, NULL); 00267 nsdynmemlib_stub.returnCounter = 2; 00268 if( 0 != coap_connection_handler_open_connection(handler, 22,false,true,true,false) ) 00269 return false; 00270 00271 if( true != coap_connection_handler_socket_belongs_to(handler, 0) ) 00272 return false; 00273 00274 if( false != coap_connection_handler_socket_belongs_to(handler, 3) ) 00275 return false; 00276 connection_handler_destroy(handler, false); 00277 00278 nsdynmemlib_stub.returnCounter = 0; 00279 return true; 00280 } 00281 00282 bool test_timer_callbacks() 00283 { 00284 coap_security_handler_stub.counter = -1; 00285 uint8_t buf[16]; 00286 memset(&buf, 1, 16); 00287 00288 nsdynmemlib_stub.returnCounter = 1; 00289 coap_conn_handler_t *handler = connection_handler_create(&receive_from_sock_cb, &send_to_sock_cb, NULL, NULL); 00290 nsdynmemlib_stub.returnCounter = 2; 00291 if( 0 != coap_connection_handler_open_connection(handler, 22,false,true,true,false) ) 00292 return false; 00293 00294 //handler->socket->data still in memory 00295 coap_security_handler_stub.sec_obj = coap_security_handler_stub_alloc(); 00296 00297 ns_timer_stub.int8_value = 0; 00298 nsdynmemlib_stub.returnCounter = 3; 00299 ns_timer_stub.int8_value = 5; 00300 if( -1 != coap_connection_handler_virtual_recv(handler,buf, 12, &buf, 1) ) 00301 return false; 00302 00303 //Note next tests will affect ns_timer test (cycle & cycle_count 00304 if( coap_security_handler_stub.start_timer_cb ){ 00305 coap_security_handler_stub.start_timer_cb(1, 0, 0); 00306 00307 coap_security_handler_stub.start_timer_cb(1, 1, 2); 00308 } 00309 00310 if( coap_security_handler_stub.timer_status_cb ){ 00311 if( -1 != coap_security_handler_stub.timer_status_cb(4) ) 00312 return false; 00313 00314 if( 0 != coap_security_handler_stub.timer_status_cb(1) ) 00315 return false; 00316 } 00317 00318 if( ns_timer_stub.cb ){ 00319 ns_timer_stub.cb(4, 0); 00320 00321 ns_timer_stub.cb(5, 0); 00322 00323 coap_security_handler_stub.int_value = MBEDTLS_ERR_SSL_TIMEOUT; 00324 00325 ns_timer_stub.cb(5, 0); 00326 coap_security_handler_stub.int_value = 0; 00327 } 00328 00329 connection_handler_destroy(handler, false); 00330 free(coap_security_handler_stub.sec_obj); 00331 coap_security_handler_stub.sec_obj = NULL; 00332 return true; 00333 } 00334 00335 bool test_socket_api_callbacks() 00336 { 00337 coap_security_handler_stub.counter = -1; 00338 uint8_t buf[16]; 00339 memset(&buf, 1, 16); 00340 00341 socket_callback_t *sckt_data = (socket_callback_t *)malloc(sizeof(socket_callback_t)); 00342 memset(sckt_data, 0, sizeof(socket_callback_t)); 00343 00344 coap_security_handler_stub.sec_obj = coap_security_handler_stub_alloc(); 00345 00346 socket_api_stub.int8_value = 0; 00347 nsdynmemlib_stub.returnCounter = 1; 00348 coap_conn_handler_t *handler = connection_handler_create(&receive_from_sock_cb, &send_to_sock_cb, NULL, NULL); 00349 nsdynmemlib_stub.returnCounter = 2; 00350 if( 0 != coap_connection_handler_open_connection(handler, 22,false,false,true,false) ) 00351 return false; 00352 00353 if( socket_api_stub.recv_cb ){ 00354 sckt_data->event_type = SOCKET_DATA; 00355 sckt_data->d_len = 1; 00356 socket_api_stub.int8_value = -1; 00357 socket_api_stub.recv_cb(sckt_data); 00358 00359 nsdynmemlib_stub.returnCounter = 1; 00360 socket_api_stub.recv_cb(sckt_data); 00361 00362 nsdynmemlib_stub.returnCounter = 1; 00363 socket_api_stub.int8_value = 1; 00364 socket_api_stub.recv_cb(sckt_data); 00365 } 00366 00367 connection_handler_destroy(handler, false); 00368 00369 nsdynmemlib_stub.returnCounter = 1; 00370 coap_conn_handler_t *handler2 = connection_handler_create(&receive_from_sock_cb, &send_to_sock_cb, &get_passwd_cb, &sec_done_cb); 00371 nsdynmemlib_stub.returnCounter = 2; 00372 if( 0 != coap_connection_handler_open_connection(handler2, 22,false,true,true,false) ) 00373 return false; 00374 00375 if( socket_api_stub.recv_cb ){ 00376 nsdynmemlib_stub.returnCounter = 1; 00377 socket_api_stub.int8_value = 1; 00378 sckt_data->socket_id = 1; 00379 socket_api_stub.recv_cb(sckt_data); 00380 00381 nsdynmemlib_stub.returnCounter = 2; 00382 socket_api_stub.int8_value = 1; 00383 sckt_data->socket_id = 1; 00384 socket_api_stub.recv_cb(sckt_data); 00385 00386 nsdynmemlib_stub.returnCounter = 1; 00387 socket_api_stub.int8_value = 1; 00388 sckt_data->socket_id = 1; 00389 socket_api_stub.recv_cb(sckt_data); 00390 00391 coap_security_handler_stub.int_value = 4; 00392 nsdynmemlib_stub.returnCounter = 1; 00393 socket_api_stub.int8_value = 1; 00394 sckt_data->socket_id = 1; 00395 socket_api_stub.recv_cb(sckt_data); 00396 00397 coap_security_handler_stub.int_value = MBEDTLS_ERR_SSL_PEER_CLOSE_NOTIFY; 00398 nsdynmemlib_stub.returnCounter = 1; 00399 socket_api_stub.int8_value = 1; 00400 sckt_data->socket_id = 1; 00401 socket_api_stub.recv_cb(sckt_data); 00402 } 00403 00404 connection_handler_destroy(handler2, false); 00405 00406 free(coap_security_handler_stub.sec_obj); 00407 coap_security_handler_stub.sec_obj = NULL; 00408 00409 free(sckt_data); 00410 sckt_data = NULL; 00411 return true; 00412 } 00413 00414 bool test_security_callbacks() 00415 { 00416 coap_security_handler_stub.counter = -1; 00417 uint8_t buf[16]; 00418 memset(&buf, 1, 16); 00419 00420 socket_callback_t *sckt_data = (socket_callback_t *)malloc(sizeof(socket_callback_t)); 00421 memset(sckt_data, 0, sizeof(socket_callback_t)); 00422 00423 coap_security_handler_stub.sec_obj = coap_security_handler_stub_alloc(); 00424 00425 nsdynmemlib_stub.returnCounter = 1; 00426 coap_conn_handler_t *handler = connection_handler_create(&receive_from_sock_cb, &send_to_sock_cb, NULL, NULL); 00427 nsdynmemlib_stub.returnCounter = 2; 00428 if( 0 != coap_connection_handler_open_connection(handler, 22,false,true,true,false) ) 00429 return false; 00430 00431 if( socket_api_stub.recv_cb ){ 00432 sckt_data->event_type = SOCKET_DATA; 00433 sckt_data->d_len = 1; 00434 nsdynmemlib_stub.returnCounter = 2; 00435 socket_api_stub.int8_value = 1; 00436 sckt_data->socket_id = 0; 00437 socket_api_stub.recv_cb(sckt_data); 00438 } 00439 00440 if( coap_security_handler_stub.send_cb ){ 00441 coap_security_handler_stub.send_cb(0, buf, 22, &buf, 16); 00442 } 00443 if( coap_security_handler_stub.receive_cb ){ 00444 coap_security_handler_stub.receive_cb(0, &buf, 16); 00445 } 00446 00447 connection_handler_destroy(handler, false); 00448 00449 free(coap_security_handler_stub.sec_obj); 00450 coap_security_handler_stub.sec_obj = NULL; 00451 00452 free(sckt_data); 00453 sckt_data = NULL; 00454 return true; 00455 }
Generated on Sun Jul 17 2022 08:25:32 by
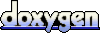