
Rtos API example
TCP internal implementations (do not use in application code) More...
Go to the source code of this file.
Functions | |
void | tcp_init (void) |
Initialize this module. | |
void | tcp_tmr (void) |
Called periodically to dispatch TCP timers. | |
void | tcp_slowtmr (void) |
Called every 500 ms and implements the retransmission timer and the timer that removes PCBs that have been in TIME-WAIT for enough time. | |
void | tcp_fasttmr (void) |
Is called every TCP_FAST_INTERVAL (250 ms) and process data previously "refused" by upper layer (application) and sends delayed ACKs. | |
void | tcp_txnow (void) |
Call tcp_output for all active pcbs that have TF_NAGLEMEMERR set. | |
void | tcp_input (struct pbuf *p, struct netif *inp) |
The initial input processing of TCP. | |
struct tcp_pcb * | tcp_alloc (u8_t prio) |
Allocate a new tcp_pcb structure. | |
void | tcp_abandon (struct tcp_pcb *pcb, int reset) |
Abandons a connection and optionally sends a RST to the remote host. | |
err_t | tcp_send_empty_ack (struct tcp_pcb *pcb) |
Send an ACK without data. | |
void | tcp_rexmit (struct tcp_pcb *pcb) |
Requeue the first unacked segment for retransmission. | |
void | tcp_rexmit_rto (struct tcp_pcb *pcb) |
Requeue all unacked segments for retransmission. | |
void | tcp_rexmit_fast (struct tcp_pcb *pcb) |
Handle retransmission after three dupacks received. | |
u32_t | tcp_update_rcv_ann_wnd (struct tcp_pcb *pcb) |
Update the state that tracks the available window space to advertise. | |
err_t | tcp_process_refused_data (struct tcp_pcb *pcb) |
Pass pcb->refused_data to the recv callback. | |
void | tcp_pcb_purge (struct tcp_pcb *pcb) |
Purges a TCP PCB. | |
void | tcp_pcb_remove (struct tcp_pcb **pcblist, struct tcp_pcb *pcb) |
Purges the PCB and removes it from a PCB list. | |
void | tcp_segs_free (struct tcp_seg *seg) |
Deallocates a list of TCP segments (tcp_seg structures). | |
void | tcp_seg_free (struct tcp_seg *seg) |
Frees a TCP segment (tcp_seg structure). | |
struct tcp_seg * | tcp_seg_copy (struct tcp_seg *seg) |
Returns a copy of the given TCP segment. | |
err_t | tcp_send_fin (struct tcp_pcb *pcb) |
Called by tcp_close() to send a segment including FIN flag but not data. | |
err_t | tcp_enqueue_flags (struct tcp_pcb *pcb, u8_t flags) |
Enqueue TCP options for transmission. | |
void | tcp_rst (u32_t seqno, u32_t ackno, const ip_addr_t *local_ip, const ip_addr_t *remote_ip, u16_t local_port, u16_t remote_port) |
Send a TCP RESET packet (empty segment with RST flag set) either to abort a connection or to show that there is no matching local connection for a received segment. | |
u32_t | tcp_next_iss (struct tcp_pcb *pcb) |
Calculates a new initial sequence number for new connections. | |
err_t | tcp_keepalive (struct tcp_pcb *pcb) |
Send keepalive packets to keep a connection active although no data is sent over it. | |
err_t | tcp_zero_window_probe (struct tcp_pcb *pcb) |
Send persist timer zero-window probes to keep a connection active when a window update is lost. | |
u16_t | tcp_eff_send_mss_impl (u16_t sendmss, const ip_addr_t *dest#if LWIP_IPV6||LWIP_IPV4_SRC_ROUTING, const ip_addr_t *src#endif) |
Calculates the effective send mss that can be used for a specific IP address by using ip_route to determine the netif used to send to the address and calculating the minimum of TCP_MSS and that netif's mtu (if set). | |
err_t | tcp_recv_null (void *arg, struct tcp_pcb *pcb, struct pbuf *p, err_t err) |
Default receive callback that is called if the user didn't register a recv callback for the pcb. | |
void | tcp_debug_print (struct tcp_hdr *tcphdr) |
Print a tcp header for debugging purposes. | |
void | tcp_debug_print_flags (u8_t flags) |
Print tcp flags for debugging purposes. | |
void | tcp_debug_print_state (enum tcp_state s) |
Print a tcp state for debugging purposes. | |
void | tcp_debug_print_pcbs (void) |
Print all tcp_pcbs in every list for debugging purposes. | |
s16_t | tcp_pcbs_sane (void) |
Check state consistency of the tcp_pcb lists. | |
void | tcp_timer_needed (void) |
External function (implemented in timers.c), called when TCP detects that a timer is needed (i.e. | |
void | tcp_netif_ip_addr_changed (const ip_addr_t *old_addr, const ip_addr_t *new_addr) |
This function is called from netif.c when address is changed or netif is removed. | |
Variables | |
struct tcp_pcb * | tcp_bound_pcbs |
List of all TCP PCBs bound but not yet (connected || listening) | |
union tcp_listen_pcbs_t | tcp_listen_pcbs |
List of all TCP PCBs in LISTEN state. | |
struct tcp_pcb * | tcp_active_pcbs |
List of all TCP PCBs that are in a state in which they accept or send data. | |
struct tcp_pcb * | tcp_tw_pcbs |
List of all TCP PCBs in TIME-WAIT state. | |
struct tcp_pcb **const | tcp_pcb_lists [NUM_TCP_PCB_LISTS] |
An array with all (non-temporary) PCB lists, mainly used for smaller code size. |
Detailed Description
TCP internal implementations (do not use in application code)
Definition in file tcp_priv.h.
Function Documentation
void tcp_abandon | ( | struct tcp_pcb * | pcb, |
int | reset | ||
) |
Abandons a connection and optionally sends a RST to the remote host.
Deletes the local protocol control block. This is done when a connection is killed because of shortage of memory.
- Parameters:
-
pcb the tcp_pcb to abort reset boolean to indicate whether a reset should be sent
Definition at line 464 of file lwip_tcp.c.
struct tcp_pcb* tcp_alloc | ( | u8_t | prio ) | [read] |
Allocate a new tcp_pcb structure.
- Parameters:
-
prio priority for the new pcb
- Returns:
- a new tcp_pcb that initially is in state CLOSED
Definition at line 1549 of file lwip_tcp.c.
void tcp_debug_print | ( | struct tcp_hdr * | tcphdr ) |
Print a tcp header for debugging purposes.
- Parameters:
-
tcphdr pointer to a struct tcp_hdr
Definition at line 2036 of file lwip_tcp.c.
void tcp_debug_print_flags | ( | u8_t | flags ) |
Print tcp flags for debugging purposes.
- Parameters:
-
flags tcp flags, all active flags are printed
Definition at line 2083 of file lwip_tcp.c.
void tcp_debug_print_pcbs | ( | void | ) |
Print all tcp_pcbs in every list for debugging purposes.
Definition at line 2116 of file lwip_tcp.c.
void tcp_debug_print_state | ( | enum tcp_state | s ) |
Print a tcp state for debugging purposes.
- Parameters:
-
s enum tcp_state to print
Definition at line 2072 of file lwip_tcp.c.
u16_t tcp_eff_send_mss_impl | ( | u16_t | sendmss, |
const ip_addr_t *dest#if LWIP_IPV6|| | LWIP_IPV4_SRC_ROUTING, | ||
const ip_addr_t *src# | endif | ||
) |
Calculates the effective send mss that can be used for a specific IP address by using ip_route to determine the netif used to send to the address and calculating the minimum of TCP_MSS and that netif's mtu (if set).
Definition at line 1910 of file lwip_tcp.c.
Enqueue TCP options for transmission.
Called by tcp_connect(), tcp_listen_input(), and tcp_send_ctrl().
- Parameters:
-
pcb Protocol control block for the TCP connection. flags TCP header flags to set in the outgoing segment.
Definition at line 785 of file lwip_tcp_out.c.
void tcp_fasttmr | ( | void | ) |
Is called every TCP_FAST_INTERVAL (250 ms) and process data previously "refused" by upper layer (application) and sends delayed ACKs.
Automatically called from tcp_tmr().
Definition at line 1245 of file lwip_tcp.c.
void tcp_init | ( | void | ) |
Initialize this module.
Definition at line 145 of file lwip_tcp.c.
The initial input processing of TCP.
It verifies the TCP header, demultiplexes the segment between the PCBs and passes it on to tcp_process(), which implements the TCP finite state machine. This function is called by the IP layer (in ip_input()).
- Parameters:
-
p received TCP segment to process (p->payload pointing to the TCP header) inp network interface on which this segment was received
Called by tcp_input() when a segment arrives for a listening connection (from tcp_input()).
- Parameters:
-
pcb the tcp_pcb_listen for which a segment arrived
- Note:
- the segment which arrived is saved in global variables, therefore only the pcb involved is passed as a parameter to this function
Called by tcp_input() when a segment arrives for a connection in TIME_WAIT.
- Parameters:
-
pcb the tcp_pcb for which a segment arrived
- Note:
- the segment which arrived is saved in global variables, therefore only the pcb involved is passed as a parameter to this function
Implements the TCP state machine. Called by tcp_input. In some states tcp_receive() is called to receive data. The tcp_seg argument will be freed by the caller (tcp_input()) unless the recv_data pointer in the pcb is set.
- Parameters:
-
pcb the tcp_pcb for which a segment arrived
- Note:
- the segment which arrived is saved in global variables, therefore only the pcb involved is passed as a parameter to this function
Insert segment into the list (segments covered with new one will be deleted)
Called from tcp_receive()
Called by tcp_process. Checks if the given segment is an ACK for outstanding data, and if so frees the memory of the buffered data. Next, it places the segment on any of the receive queues (pcb->recved or pcb->ooseq). If the segment is buffered, the pbuf is referenced by pbuf_ref so that it will not be freed until it has been removed from the buffer.
If the incoming segment constitutes an ACK for a segment that was used for RTT estimation, the RTT is estimated here as well.
Called from tcp_process().
Parses the options contained in the incoming segment.
Called from tcp_listen_input() and tcp_process(). Currently, only the MSS option is supported!
- Parameters:
-
pcb the tcp_pcb for which a segment arrived
Definition at line 102 of file lwip_tcp_in.c.
Send keepalive packets to keep a connection active although no data is sent over it.
Called by tcp_slowtmr()
- Parameters:
-
pcb the tcp_pcb for which to send a keepalive packet
Definition at line 1532 of file lwip_tcp_out.c.
This function is called from netif.c when address is changed or netif is removed.
- Parameters:
-
old_addr IP address of the netif before change new_addr IP address of the netif after change or NULL if netif has been removed
Definition at line 2000 of file lwip_tcp.c.
u32_t tcp_next_iss | ( | struct tcp_pcb * | pcb ) |
Calculates a new initial sequence number for new connections.
- Returns:
- u32_t pseudo random sequence number
Definition at line 1889 of file lwip_tcp.c.
void tcp_pcb_purge | ( | struct tcp_pcb * | pcb ) |
Purges a TCP PCB.
Removes any buffered data and frees the buffer memory (pcb->ooseq, pcb->unsent and pcb->unacked are freed).
- Parameters:
-
pcb tcp_pcb to purge. The pcb itself is not deallocated!
Definition at line 1805 of file lwip_tcp.c.
Purges the PCB and removes it from a PCB list.
Any delayed ACKs are sent first.
- Parameters:
-
pcblist PCB list to purge. pcb tcp_pcb to purge. The pcb itself is NOT deallocated!
Definition at line 1854 of file lwip_tcp.c.
s16_t tcp_pcbs_sane | ( | void | ) |
Check state consistency of the tcp_pcb lists.
Definition at line 2148 of file lwip_tcp.c.
Pass pcb->refused_data to the recv callback.
Definition at line 1305 of file lwip_tcp.c.
Default receive callback that is called if the user didn't register a recv callback for the pcb.
Definition at line 1438 of file lwip_tcp.c.
void tcp_rexmit | ( | struct tcp_pcb * | pcb ) |
Requeue the first unacked segment for retransmission.
Called by tcp_receive() for fast retransmit.
- Parameters:
-
pcb the tcp_pcb for which to retransmit the first unacked segment
Definition at line 1442 of file lwip_tcp_out.c.
void tcp_rexmit_fast | ( | struct tcp_pcb * | pcb ) |
Handle retransmission after three dupacks received.
- Parameters:
-
pcb the tcp_pcb for which to retransmit the first unacked segment
Definition at line 1490 of file lwip_tcp_out.c.
void tcp_rexmit_rto | ( | struct tcp_pcb * | pcb ) |
Requeue all unacked segments for retransmission.
Called by tcp_slowtmr() for slow retransmission.
- Parameters:
-
pcb the tcp_pcb for which to re-enqueue all unacked segments
Definition at line 1399 of file lwip_tcp_out.c.
void tcp_rst | ( | u32_t | seqno, |
u32_t | ackno, | ||
const ip_addr_t * | local_ip, | ||
const ip_addr_t * | remote_ip, | ||
u16_t | local_port, | ||
u16_t | remote_port | ||
) |
Send a TCP RESET packet (empty segment with RST flag set) either to abort a connection or to show that there is no matching local connection for a received segment.
Called by tcp_abort() (to abort a local connection), tcp_input() (if no matching local pcb was found), tcp_listen_input() (if incoming segment has ACK flag set) and tcp_process() (received segment in the wrong state)
Since a RST segment is in most cases not sent for an active connection, tcp_rst() has a number of arguments that are taken from a tcp_pcb for most other segment output functions.
- Parameters:
-
seqno the sequence number to use for the outgoing segment ackno the acknowledge number to use for the outgoing segment local_ip the local IP address to send the segment from remote_ip the remote IP address to send the segment to local_port the local TCP port to send the segment from remote_port the remote TCP port to send the segment to
Definition at line 1344 of file lwip_tcp_out.c.
struct tcp_seg* tcp_seg_copy | ( | struct tcp_seg * | seg ) | [read] |
Returns a copy of the given TCP segment.
The pbuf and data are not copied, only the pointers
- Parameters:
-
seg the old tcp_seg
- Returns:
- a copy of seg
Definition at line 1418 of file lwip_tcp.c.
void tcp_seg_free | ( | struct tcp_seg * | seg ) |
Frees a TCP segment (tcp_seg structure).
- Parameters:
-
seg single tcp_seg to free
Definition at line 1384 of file lwip_tcp.c.
void tcp_segs_free | ( | struct tcp_seg * | seg ) |
Deallocates a list of TCP segments (tcp_seg structures).
- Parameters:
-
seg tcp_seg list of TCP segments to free
Definition at line 1369 of file lwip_tcp.c.
Send an ACK without data.
- Parameters:
-
pcb Protocol control block for the TCP connection to send the ACK
Definition at line 918 of file lwip_tcp_out.c.
Called by tcp_close() to send a segment including FIN flag but not data.
- Parameters:
-
pcb the tcp_pcb over which to send a segment
- Returns:
- ERR_OK if sent, another err_t otherwise
Definition at line 139 of file lwip_tcp_out.c.
void tcp_slowtmr | ( | void | ) |
Called every 500 ms and implements the retransmission timer and the timer that removes PCBs that have been in TIME-WAIT for enough time.
It also increments various timers such as the inactivity timer in each PCB.
Automatically called from tcp_tmr().
Definition at line 980 of file lwip_tcp.c.
void tcp_timer_needed | ( | void | ) |
External function (implemented in timers.c), called when TCP detects that a timer is needed (i.e.
active- or time-wait-pcb found).
Definition at line 147 of file lwip_timeouts.c.
void tcp_tmr | ( | void | ) |
Called periodically to dispatch TCP timers.
Definition at line 156 of file lwip_tcp.c.
void tcp_txnow | ( | void | ) |
Call tcp_output for all active pcbs that have TF_NAGLEMEMERR set.
Definition at line 1292 of file lwip_tcp.c.
u32_t tcp_update_rcv_ann_wnd | ( | struct tcp_pcb * | pcb ) |
Update the state that tracks the available window space to advertise.
Returns how much extra window would be advertised if we sent an update now.
Definition at line 753 of file lwip_tcp.c.
Send persist timer zero-window probes to keep a connection active when a window update is lost.
Called by tcp_slowtmr()
- Parameters:
-
pcb the tcp_pcb for which to send a zero-window probe packet
Definition at line 1586 of file lwip_tcp_out.c.
Variable Documentation
struct tcp_pcb* tcp_active_pcbs |
List of all TCP PCBs that are in a state in which they accept or send data.
Definition at line 124 of file lwip_tcp.c.
struct tcp_pcb* tcp_bound_pcbs |
List of all TCP PCBs bound but not yet (connected || listening)
Definition at line 119 of file lwip_tcp.c.
union tcp_listen_pcbs_t tcp_listen_pcbs |
List of all TCP PCBs in LISTEN state.
Definition at line 121 of file lwip_tcp.c.
struct tcp_pcb** const tcp_pcb_lists[NUM_TCP_PCB_LISTS] |
An array with all (non-temporary) PCB lists, mainly used for smaller code size.
Definition at line 129 of file lwip_tcp.c.
struct tcp_pcb* tcp_tw_pcbs |
List of all TCP PCBs in TIME-WAIT state.
Definition at line 126 of file lwip_tcp.c.
Generated on Sun Jul 17 2022 08:25:36 by
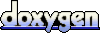