
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
lwip_tcp.c
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * Transmission Control Protocol for IP 00004 * See also @ref tcp_raw 00005 * 00006 * @defgroup tcp_raw TCP 00007 * @ingroup callbackstyle_api 00008 * Transmission Control Protocol for IP\n 00009 * @see @ref raw_api and @ref netconn 00010 * 00011 * Common functions for the TCP implementation, such as functinos 00012 * for manipulating the data structures and the TCP timer functions. TCP functions 00013 * related to input and output is found in tcp_in.c and tcp_out.c respectively.\n 00014 */ 00015 00016 /* 00017 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00018 * All rights reserved. 00019 * 00020 * Redistribution and use in source and binary forms, with or without modification, 00021 * are permitted provided that the following conditions are met: 00022 * 00023 * 1. Redistributions of source code must retain the above copyright notice, 00024 * this list of conditions and the following disclaimer. 00025 * 2. Redistributions in binary form must reproduce the above copyright notice, 00026 * this list of conditions and the following disclaimer in the documentation 00027 * and/or other materials provided with the distribution. 00028 * 3. The name of the author may not be used to endorse or promote products 00029 * derived from this software without specific prior written permission. 00030 * 00031 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00032 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00033 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00034 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00035 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00036 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00037 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00038 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00039 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00040 * OF SUCH DAMAGE. 00041 * 00042 * This file is part of the lwIP TCP/IP stack. 00043 * 00044 * Author: Adam Dunkels <adam@sics.se> 00045 * 00046 */ 00047 00048 #include "lwip/opt.h" 00049 00050 #if LWIP_TCP /* don't build if not configured for use in lwipopts.h */ 00051 00052 #include "lwip/def.h" 00053 #include "lwip/mem.h" 00054 #include "lwip/memp.h" 00055 #include "lwip/tcp.h" 00056 #include "lwip/priv/tcp_priv.h" 00057 #include "lwip/debug.h" 00058 #include "lwip/stats.h" 00059 #include "lwip/ip6.h" 00060 #include "lwip/ip6_addr.h" 00061 #include "lwip/nd6.h" 00062 00063 #include <string.h> 00064 00065 #ifdef LWIP_HOOK_FILENAME 00066 #include LWIP_HOOK_FILENAME 00067 #endif 00068 00069 #ifndef TCP_LOCAL_PORT_RANGE_START 00070 /* From http://www.iana.org/assignments/port-numbers: 00071 "The Dynamic and/or Private Ports are those from 49152 through 65535" */ 00072 #define TCP_LOCAL_PORT_RANGE_START 0xc000 00073 #define TCP_LOCAL_PORT_RANGE_END 0xffff 00074 #define TCP_ENSURE_LOCAL_PORT_RANGE(port) ((u16_t)(((port) & ~TCP_LOCAL_PORT_RANGE_START) + TCP_LOCAL_PORT_RANGE_START)) 00075 #endif 00076 00077 #if LWIP_TCP_KEEPALIVE 00078 #define TCP_KEEP_DUR(pcb) ((pcb)->keep_cnt * (pcb)->keep_intvl) 00079 #define TCP_KEEP_INTVL(pcb) ((pcb)->keep_intvl) 00080 #else /* LWIP_TCP_KEEPALIVE */ 00081 #define TCP_KEEP_DUR(pcb) TCP_MAXIDLE 00082 #define TCP_KEEP_INTVL(pcb) TCP_KEEPINTVL_DEFAULT 00083 #endif /* LWIP_TCP_KEEPALIVE */ 00084 00085 /* As initial send MSS, we use TCP_MSS but limit it to 536. */ 00086 #if TCP_MSS > 536 00087 #define INITIAL_MSS 536 00088 #else 00089 #define INITIAL_MSS TCP_MSS 00090 #endif 00091 00092 static const char * const tcp_state_str[] = { 00093 "CLOSED", 00094 "LISTEN", 00095 "SYN_SENT", 00096 "SYN_RCVD", 00097 "ESTABLISHED", 00098 "FIN_WAIT_1", 00099 "FIN_WAIT_2", 00100 "CLOSE_WAIT", 00101 "CLOSING", 00102 "LAST_ACK", 00103 "TIME_WAIT" 00104 }; 00105 00106 /* last local TCP port */ 00107 static u16_t tcp_port = TCP_LOCAL_PORT_RANGE_START; 00108 00109 /* Incremented every coarse grained timer shot (typically every 500 ms). */ 00110 u32_t tcp_ticks; 00111 static const u8_t tcp_backoff[13] = 00112 { 1, 2, 3, 4, 5, 6, 7, 7, 7, 7, 7, 7, 7}; 00113 /* Times per slowtmr hits */ 00114 static const u8_t tcp_persist_backoff[7] = { 3, 6, 12, 24, 48, 96, 120 }; 00115 00116 /* The TCP PCB lists. */ 00117 00118 /** List of all TCP PCBs bound but not yet (connected || listening) */ 00119 struct tcp_pcb *tcp_bound_pcbs; 00120 /** List of all TCP PCBs in LISTEN state */ 00121 union tcp_listen_pcbs_t tcp_listen_pcbs; 00122 /** List of all TCP PCBs that are in a state in which 00123 * they accept or send data. */ 00124 struct tcp_pcb *tcp_active_pcbs; 00125 /** List of all TCP PCBs in TIME-WAIT state */ 00126 struct tcp_pcb *tcp_tw_pcbs; 00127 00128 /** An array with all (non-temporary) PCB lists, mainly used for smaller code size */ 00129 struct tcp_pcb ** const tcp_pcb_lists[] = {&tcp_listen_pcbs.pcbs, &tcp_bound_pcbs, 00130 &tcp_active_pcbs, &tcp_tw_pcbs}; 00131 00132 u8_t tcp_active_pcbs_changed; 00133 00134 /** Timer counter to handle calling slow-timer from tcp_tmr() */ 00135 static u8_t tcp_timer; 00136 static u8_t tcp_timer_ctr; 00137 static u16_t tcp_new_port(void); 00138 00139 static err_t tcp_close_shutdown_fin(struct tcp_pcb *pcb); 00140 00141 /** 00142 * Initialize this module. 00143 */ 00144 void 00145 tcp_init(void) 00146 { 00147 #if LWIP_RANDOMIZE_INITIAL_LOCAL_PORTS && defined(LWIP_RAND) 00148 tcp_port = TCP_ENSURE_LOCAL_PORT_RANGE(LWIP_RAND()); 00149 #endif /* LWIP_RANDOMIZE_INITIAL_LOCAL_PORTS && defined(LWIP_RAND) */ 00150 } 00151 00152 /** 00153 * Called periodically to dispatch TCP timers. 00154 */ 00155 void 00156 tcp_tmr(void) 00157 { 00158 /* Call tcp_fasttmr() every 250 ms */ 00159 tcp_fasttmr(); 00160 00161 if (++tcp_timer & 1) { 00162 /* Call tcp_slowtmr() every 500 ms, i.e., every other timer 00163 tcp_tmr() is called. */ 00164 tcp_slowtmr(); 00165 } 00166 } 00167 00168 #if LWIP_CALLBACK_API || TCP_LISTEN_BACKLOG 00169 /** Called when a listen pcb is closed. Iterates one pcb list and removes the 00170 * closed listener pcb from pcb->listener if matching. 00171 */ 00172 static void 00173 tcp_remove_listener(struct tcp_pcb *list, struct tcp_pcb_listen *lpcb) 00174 { 00175 struct tcp_pcb *pcb; 00176 for (pcb = list; pcb != NULL; pcb = pcb->next) { 00177 if (pcb->listener == lpcb) { 00178 pcb->listener = NULL; 00179 } 00180 } 00181 } 00182 #endif 00183 00184 /** Called when a listen pcb is closed. Iterates all pcb lists and removes the 00185 * closed listener pcb from pcb->listener if matching. 00186 */ 00187 static void 00188 tcp_listen_closed(struct tcp_pcb *pcb) 00189 { 00190 #if LWIP_CALLBACK_API || TCP_LISTEN_BACKLOG 00191 size_t i; 00192 LWIP_ASSERT("pcb != NULL", pcb != NULL); 00193 LWIP_ASSERT("pcb->state == LISTEN", pcb->state == LISTEN); 00194 for (i = 1; i < LWIP_ARRAYSIZE(tcp_pcb_lists); i++) { 00195 tcp_remove_listener(*tcp_pcb_lists[i], (struct tcp_pcb_listen*)pcb); 00196 } 00197 #endif 00198 LWIP_UNUSED_ARG(pcb); 00199 } 00200 00201 #if TCP_LISTEN_BACKLOG 00202 /** @ingroup tcp_raw 00203 * Delay accepting a connection in respect to the listen backlog: 00204 * the number of outstanding connections is increased until 00205 * tcp_backlog_accepted() is called. 00206 * 00207 * ATTENTION: the caller is responsible for calling tcp_backlog_accepted() 00208 * or else the backlog feature will get out of sync! 00209 * 00210 * @param pcb the connection pcb which is not fully accepted yet 00211 */ 00212 void 00213 tcp_backlog_delayed(struct tcp_pcb* pcb) 00214 { 00215 LWIP_ASSERT("pcb != NULL", pcb != NULL); 00216 if ((pcb->flags & TF_BACKLOGPEND) == 0) { 00217 if (pcb->listener != NULL) { 00218 pcb->listener->accepts_pending++; 00219 LWIP_ASSERT("accepts_pending != 0", pcb->listener->accepts_pending != 0); 00220 pcb->flags |= TF_BACKLOGPEND; 00221 } 00222 } 00223 } 00224 00225 /** @ingroup tcp_raw 00226 * A delayed-accept a connection is accepted (or closed/aborted): decreases 00227 * the number of outstanding connections after calling tcp_backlog_delayed(). 00228 * 00229 * ATTENTION: the caller is responsible for calling tcp_backlog_accepted() 00230 * or else the backlog feature will get out of sync! 00231 * 00232 * @param pcb the connection pcb which is now fully accepted (or closed/aborted) 00233 */ 00234 void 00235 tcp_backlog_accepted(struct tcp_pcb* pcb) 00236 { 00237 LWIP_ASSERT("pcb != NULL", pcb != NULL); 00238 if ((pcb->flags & TF_BACKLOGPEND) != 0) { 00239 if (pcb->listener != NULL) { 00240 LWIP_ASSERT("accepts_pending != 0", pcb->listener->accepts_pending != 0); 00241 pcb->listener->accepts_pending--; 00242 pcb->flags &= ~TF_BACKLOGPEND; 00243 } 00244 } 00245 } 00246 #endif /* TCP_LISTEN_BACKLOG */ 00247 00248 /** 00249 * Closes the TX side of a connection held by the PCB. 00250 * For tcp_close(), a RST is sent if the application didn't receive all data 00251 * (tcp_recved() not called for all data passed to recv callback). 00252 * 00253 * Listening pcbs are freed and may not be referenced any more. 00254 * Connection pcbs are freed if not yet connected and may not be referenced 00255 * any more. If a connection is established (at least SYN received or in 00256 * a closing state), the connection is closed, and put in a closing state. 00257 * The pcb is then automatically freed in tcp_slowtmr(). It is therefore 00258 * unsafe to reference it. 00259 * 00260 * @param pcb the tcp_pcb to close 00261 * @return ERR_OK if connection has been closed 00262 * another err_t if closing failed and pcb is not freed 00263 */ 00264 static err_t 00265 tcp_close_shutdown(struct tcp_pcb *pcb, u8_t rst_on_unacked_data) 00266 { 00267 if (rst_on_unacked_data && ((pcb->state == ESTABLISHED) || (pcb->state == CLOSE_WAIT))) { 00268 if ((pcb->refused_data != NULL) || (pcb->rcv_wnd != TCP_WND_MAX(pcb))) { 00269 /* Not all data received by application, send RST to tell the remote 00270 side about this. */ 00271 LWIP_ASSERT("pcb->flags & TF_RXCLOSED", pcb->flags & TF_RXCLOSED); 00272 00273 /* don't call tcp_abort here: we must not deallocate the pcb since 00274 that might not be expected when calling tcp_close */ 00275 tcp_rst(pcb->snd_nxt, pcb->rcv_nxt, &pcb->local_ip, &pcb->remote_ip, 00276 pcb->local_port, pcb->remote_port); 00277 00278 tcp_pcb_purge(pcb); 00279 TCP_RMV_ACTIVE(pcb); 00280 if (pcb->state == ESTABLISHED) { 00281 /* move to TIME_WAIT since we close actively */ 00282 pcb->state = TIME_WAIT; 00283 TCP_REG(&tcp_tw_pcbs, pcb); 00284 } else { 00285 /* CLOSE_WAIT: deallocate the pcb since we already sent a RST for it */ 00286 if (tcp_input_pcb == pcb) { 00287 /* prevent using a deallocated pcb: free it from tcp_input later */ 00288 tcp_trigger_input_pcb_close(); 00289 } else { 00290 memp_free(MEMP_TCP_PCB, pcb); 00291 } 00292 } 00293 return ERR_OK; 00294 } 00295 } 00296 00297 /* - states which free the pcb are handled here, 00298 - states which send FIN and change state are handled in tcp_close_shutdown_fin() */ 00299 switch (pcb->state) { 00300 case CLOSED: 00301 /* Closing a pcb in the CLOSED state might seem erroneous, 00302 * however, it is in this state once allocated and as yet unused 00303 * and the user needs some way to free it should the need arise. 00304 * Calling tcp_close() with a pcb that has already been closed, (i.e. twice) 00305 * or for a pcb that has been used and then entered the CLOSED state 00306 * is erroneous, but this should never happen as the pcb has in those cases 00307 * been freed, and so any remaining handles are bogus. */ 00308 if (pcb->local_port != 0) { 00309 TCP_RMV(&tcp_bound_pcbs, pcb); 00310 } 00311 memp_free(MEMP_TCP_PCB, pcb); 00312 break; 00313 case LISTEN: 00314 tcp_listen_closed(pcb); 00315 tcp_pcb_remove(&tcp_listen_pcbs.pcbs, pcb); 00316 memp_free(MEMP_TCP_PCB_LISTEN, pcb); 00317 break; 00318 case SYN_SENT: 00319 TCP_PCB_REMOVE_ACTIVE(pcb); 00320 memp_free(MEMP_TCP_PCB, pcb); 00321 MIB2_STATS_INC(mib2.tcpattemptfails); 00322 break; 00323 default: 00324 return tcp_close_shutdown_fin(pcb); 00325 } 00326 return ERR_OK; 00327 } 00328 00329 static err_t 00330 tcp_close_shutdown_fin(struct tcp_pcb *pcb) 00331 { 00332 err_t err; 00333 LWIP_ASSERT("pcb != NULL", pcb != NULL); 00334 00335 switch (pcb->state) { 00336 case SYN_RCVD: 00337 err = tcp_send_fin(pcb); 00338 if (err == ERR_OK) { 00339 tcp_backlog_accepted(pcb); 00340 MIB2_STATS_INC(mib2.tcpattemptfails); 00341 pcb->state = FIN_WAIT_1; 00342 } 00343 break; 00344 case ESTABLISHED: 00345 err = tcp_send_fin(pcb); 00346 if (err == ERR_OK) { 00347 MIB2_STATS_INC(mib2.tcpestabresets); 00348 pcb->state = FIN_WAIT_1; 00349 } 00350 break; 00351 case CLOSE_WAIT: 00352 err = tcp_send_fin(pcb); 00353 if (err == ERR_OK) { 00354 MIB2_STATS_INC(mib2.tcpestabresets); 00355 pcb->state = LAST_ACK; 00356 } 00357 break; 00358 default: 00359 /* Has already been closed, do nothing. */ 00360 return ERR_OK; 00361 } 00362 00363 if (err == ERR_OK) { 00364 /* To ensure all data has been sent when tcp_close returns, we have 00365 to make sure tcp_output doesn't fail. 00366 Since we don't really have to ensure all data has been sent when tcp_close 00367 returns (unsent data is sent from tcp timer functions, also), we don't care 00368 for the return value of tcp_output for now. */ 00369 tcp_output(pcb); 00370 } else if (err == ERR_MEM) { 00371 /* Mark this pcb for closing. Closing is retried from tcp_tmr. */ 00372 pcb->flags |= TF_CLOSEPEND; 00373 } 00374 return err; 00375 } 00376 00377 /** 00378 * @ingroup tcp_raw 00379 * Closes the connection held by the PCB. 00380 * 00381 * Listening pcbs are freed and may not be referenced any more. 00382 * Connection pcbs are freed if not yet connected and may not be referenced 00383 * any more. If a connection is established (at least SYN received or in 00384 * a closing state), the connection is closed, and put in a closing state. 00385 * The pcb is then automatically freed in tcp_slowtmr(). It is therefore 00386 * unsafe to reference it (unless an error is returned). 00387 * 00388 * @param pcb the tcp_pcb to close 00389 * @return ERR_OK if connection has been closed 00390 * another err_t if closing failed and pcb is not freed 00391 */ 00392 err_t 00393 tcp_close(struct tcp_pcb *pcb) 00394 { 00395 LWIP_DEBUGF(TCP_DEBUG, ("tcp_close: closing in ")); 00396 tcp_debug_print_state(pcb->state); 00397 00398 if (pcb->state != LISTEN) { 00399 /* Set a flag not to receive any more data... */ 00400 pcb->flags |= TF_RXCLOSED; 00401 } 00402 /* ... and close */ 00403 return tcp_close_shutdown(pcb, 1); 00404 } 00405 00406 /** 00407 * @ingroup tcp_raw 00408 * Causes all or part of a full-duplex connection of this PCB to be shut down. 00409 * This doesn't deallocate the PCB unless shutting down both sides! 00410 * Shutting down both sides is the same as calling tcp_close, so if it succeds, 00411 * the PCB should not be referenced any more. 00412 * 00413 * @param pcb PCB to shutdown 00414 * @param shut_rx shut down receive side if this is != 0 00415 * @param shut_tx shut down send side if this is != 0 00416 * @return ERR_OK if shutdown succeeded (or the PCB has already been shut down) 00417 * another err_t on error. 00418 */ 00419 err_t 00420 tcp_shutdown(struct tcp_pcb *pcb, int shut_rx, int shut_tx) 00421 { 00422 if (pcb->state == LISTEN) { 00423 return ERR_CONN; 00424 } 00425 if (shut_rx) { 00426 /* shut down the receive side: set a flag not to receive any more data... */ 00427 pcb->flags |= TF_RXCLOSED; 00428 if (shut_tx) { 00429 /* shutting down the tx AND rx side is the same as closing for the raw API */ 00430 return tcp_close_shutdown(pcb, 1); 00431 } 00432 /* ... and free buffered data */ 00433 if (pcb->refused_data != NULL) { 00434 pbuf_free(pcb->refused_data); 00435 pcb->refused_data = NULL; 00436 } 00437 } 00438 if (shut_tx) { 00439 /* This can't happen twice since if it succeeds, the pcb's state is changed. 00440 Only close in these states as the others directly deallocate the PCB */ 00441 switch (pcb->state) { 00442 case SYN_RCVD: 00443 case ESTABLISHED: 00444 case CLOSE_WAIT: 00445 return tcp_close_shutdown(pcb, (u8_t)shut_rx); 00446 default: 00447 /* Not (yet?) connected, cannot shutdown the TX side as that would bring us 00448 into CLOSED state, where the PCB is deallocated. */ 00449 return ERR_CONN; 00450 } 00451 } 00452 return ERR_OK; 00453 } 00454 00455 /** 00456 * Abandons a connection and optionally sends a RST to the remote 00457 * host. Deletes the local protocol control block. This is done when 00458 * a connection is killed because of shortage of memory. 00459 * 00460 * @param pcb the tcp_pcb to abort 00461 * @param reset boolean to indicate whether a reset should be sent 00462 */ 00463 void 00464 tcp_abandon(struct tcp_pcb *pcb, int reset) 00465 { 00466 u32_t seqno, ackno; 00467 #if LWIP_CALLBACK_API 00468 tcp_err_fn errf; 00469 #endif /* LWIP_CALLBACK_API */ 00470 void *errf_arg; 00471 00472 /* pcb->state LISTEN not allowed here */ 00473 LWIP_ASSERT("don't call tcp_abort/tcp_abandon for listen-pcbs", 00474 pcb->state != LISTEN); 00475 /* Figure out on which TCP PCB list we are, and remove us. If we 00476 are in an active state, call the receive function associated with 00477 the PCB with a NULL argument, and send an RST to the remote end. */ 00478 if (pcb->state == TIME_WAIT) { 00479 tcp_pcb_remove(&tcp_tw_pcbs, pcb); 00480 memp_free(MEMP_TCP_PCB, pcb); 00481 } else { 00482 int send_rst = 0; 00483 u16_t local_port = 0; 00484 enum tcp_state last_state; 00485 seqno = pcb->snd_nxt; 00486 ackno = pcb->rcv_nxt; 00487 #if LWIP_CALLBACK_API 00488 errf = pcb->errf; 00489 #endif /* LWIP_CALLBACK_API */ 00490 errf_arg = pcb->callback_arg; 00491 if (pcb->state == CLOSED) { 00492 if (pcb->local_port != 0) { 00493 /* bound, not yet opened */ 00494 TCP_RMV(&tcp_bound_pcbs, pcb); 00495 } 00496 } else { 00497 send_rst = reset; 00498 local_port = pcb->local_port; 00499 TCP_PCB_REMOVE_ACTIVE(pcb); 00500 } 00501 if (pcb->unacked != NULL) { 00502 tcp_segs_free(pcb->unacked); 00503 } 00504 if (pcb->unsent != NULL) { 00505 tcp_segs_free(pcb->unsent); 00506 } 00507 #if TCP_QUEUE_OOSEQ 00508 if (pcb->ooseq != NULL) { 00509 tcp_segs_free(pcb->ooseq); 00510 } 00511 #endif /* TCP_QUEUE_OOSEQ */ 00512 tcp_backlog_accepted(pcb); 00513 if (send_rst) { 00514 LWIP_DEBUGF(TCP_RST_DEBUG, ("tcp_abandon: sending RST\n")); 00515 tcp_rst(seqno, ackno, &pcb->local_ip, &pcb->remote_ip, local_port, pcb->remote_port); 00516 } 00517 last_state = pcb->state; 00518 memp_free(MEMP_TCP_PCB, pcb); 00519 TCP_EVENT_ERR(last_state, errf, errf_arg, ERR_ABRT); 00520 } 00521 } 00522 00523 /** 00524 * @ingroup tcp_raw 00525 * Aborts the connection by sending a RST (reset) segment to the remote 00526 * host. The pcb is deallocated. This function never fails. 00527 * 00528 * ATTENTION: When calling this from one of the TCP callbacks, make 00529 * sure you always return ERR_ABRT (and never return ERR_ABRT otherwise 00530 * or you will risk accessing deallocated memory or memory leaks! 00531 * 00532 * @param pcb the tcp pcb to abort 00533 */ 00534 void 00535 tcp_abort(struct tcp_pcb *pcb) 00536 { 00537 tcp_abandon(pcb, 1); 00538 } 00539 00540 /** 00541 * @ingroup tcp_raw 00542 * Binds the connection to a local port number and IP address. If the 00543 * IP address is not given (i.e., ipaddr == NULL), the IP address of 00544 * the outgoing network interface is used instead. 00545 * 00546 * @param pcb the tcp_pcb to bind (no check is done whether this pcb is 00547 * already bound!) 00548 * @param ipaddr the local ip address to bind to (use IP4_ADDR_ANY to bind 00549 * to any local address 00550 * @param port the local port to bind to 00551 * @return ERR_USE if the port is already in use 00552 * ERR_VAL if bind failed because the PCB is not in a valid state 00553 * ERR_OK if bound 00554 */ 00555 err_t 00556 tcp_bind(struct tcp_pcb *pcb, const ip_addr_t *ipaddr, u16_t port) 00557 { 00558 int i; 00559 int max_pcb_list = NUM_TCP_PCB_LISTS; 00560 struct tcp_pcb *cpcb; 00561 00562 #if LWIP_IPV4 00563 /* Don't propagate NULL pointer (IPv4 ANY) to subsequent functions */ 00564 if (ipaddr == NULL) { 00565 ipaddr = IP4_ADDR_ANY; 00566 } 00567 #endif /* LWIP_IPV4 */ 00568 00569 /* still need to check for ipaddr == NULL in IPv6 only case */ 00570 if ((pcb == NULL) || (ipaddr == NULL)) { 00571 return ERR_VAL; 00572 } 00573 00574 LWIP_ERROR("tcp_bind: can only bind in state CLOSED", pcb->state == CLOSED, return ERR_VAL); 00575 00576 #if SO_REUSE 00577 /* Unless the REUSEADDR flag is set, 00578 we have to check the pcbs in TIME-WAIT state, also. 00579 We do not dump TIME_WAIT pcb's; they can still be matched by incoming 00580 packets using both local and remote IP addresses and ports to distinguish. 00581 */ 00582 if (ip_get_option(pcb, SOF_REUSEADDR)) { 00583 max_pcb_list = NUM_TCP_PCB_LISTS_NO_TIME_WAIT; 00584 } 00585 #endif /* SO_REUSE */ 00586 00587 if (port == 0) { 00588 port = tcp_new_port(); 00589 if (port == 0) { 00590 return ERR_BUF; 00591 } 00592 } else { 00593 /* Check if the address already is in use (on all lists) */ 00594 for (i = 0; i < max_pcb_list; i++) { 00595 for (cpcb = *tcp_pcb_lists[i]; cpcb != NULL; cpcb = cpcb->next) { 00596 if (cpcb->local_port == port) { 00597 #if SO_REUSE 00598 /* Omit checking for the same port if both pcbs have REUSEADDR set. 00599 For SO_REUSEADDR, the duplicate-check for a 5-tuple is done in 00600 tcp_connect. */ 00601 if (!ip_get_option(pcb, SOF_REUSEADDR) || 00602 !ip_get_option(cpcb, SOF_REUSEADDR)) 00603 #endif /* SO_REUSE */ 00604 { 00605 /* @todo: check accept_any_ip_version */ 00606 if ((IP_IS_V6(ipaddr) == IP_IS_V6_VAL(cpcb->local_ip)) && 00607 (ip_addr_isany(&cpcb->local_ip) || 00608 ip_addr_isany(ipaddr) || 00609 ip_addr_cmp(&cpcb->local_ip, ipaddr))) { 00610 return ERR_USE; 00611 } 00612 } 00613 } 00614 } 00615 } 00616 } 00617 00618 if (!ip_addr_isany(ipaddr)) { 00619 ip_addr_set(&pcb->local_ip, ipaddr); 00620 } 00621 pcb->local_port = port; 00622 TCP_REG(&tcp_bound_pcbs, pcb); 00623 LWIP_DEBUGF(TCP_DEBUG, ("tcp_bind: bind to port %"U16_F"\n", port)); 00624 return ERR_OK; 00625 } 00626 #if LWIP_CALLBACK_API 00627 /** 00628 * Default accept callback if no accept callback is specified by the user. 00629 */ 00630 static err_t 00631 tcp_accept_null(void *arg, struct tcp_pcb *pcb, err_t err) 00632 { 00633 LWIP_UNUSED_ARG(arg); 00634 LWIP_UNUSED_ARG(err); 00635 00636 tcp_abort(pcb); 00637 00638 return ERR_ABRT; 00639 } 00640 #endif /* LWIP_CALLBACK_API */ 00641 00642 /** 00643 * @ingroup tcp_raw 00644 * Set the state of the connection to be LISTEN, which means that it 00645 * is able to accept incoming connections. The protocol control block 00646 * is reallocated in order to consume less memory. Setting the 00647 * connection to LISTEN is an irreversible process. 00648 * 00649 * @param pcb the original tcp_pcb 00650 * @param backlog the incoming connections queue limit 00651 * @return tcp_pcb used for listening, consumes less memory. 00652 * 00653 * @note The original tcp_pcb is freed. This function therefore has to be 00654 * called like this: 00655 * tpcb = tcp_listen_with_backlog(tpcb, backlog); 00656 */ 00657 struct tcp_pcb * 00658 tcp_listen_with_backlog(struct tcp_pcb *pcb, u8_t backlog) 00659 { 00660 return tcp_listen_with_backlog_and_err(pcb, backlog, NULL); 00661 } 00662 00663 /** 00664 * @ingroup tcp_raw 00665 * Set the state of the connection to be LISTEN, which means that it 00666 * is able to accept incoming connections. The protocol control block 00667 * is reallocated in order to consume less memory. Setting the 00668 * connection to LISTEN is an irreversible process. 00669 * 00670 * @param pcb the original tcp_pcb 00671 * @param backlog the incoming connections queue limit 00672 * @param err when NULL is returned, this contains the error reason 00673 * @return tcp_pcb used for listening, consumes less memory. 00674 * 00675 * @note The original tcp_pcb is freed. This function therefore has to be 00676 * called like this: 00677 * tpcb = tcp_listen_with_backlog_and_err(tpcb, backlog, &err); 00678 */ 00679 struct tcp_pcb * 00680 tcp_listen_with_backlog_and_err(struct tcp_pcb *pcb, u8_t backlog, err_t *err) 00681 { 00682 struct tcp_pcb_listen *lpcb = NULL; 00683 err_t res; 00684 00685 LWIP_UNUSED_ARG(backlog); 00686 LWIP_ERROR("tcp_listen: pcb already connected", pcb->state == CLOSED, res = ERR_CLSD; goto done); 00687 00688 /* already listening? */ 00689 if (pcb->state == LISTEN) { 00690 lpcb = (struct tcp_pcb_listen*)pcb; 00691 res = ERR_ALREADY; 00692 goto done; 00693 } 00694 #if SO_REUSE 00695 if (ip_get_option(pcb, SOF_REUSEADDR)) { 00696 /* Since SOF_REUSEADDR allows reusing a local address before the pcb's usage 00697 is declared (listen-/connection-pcb), we have to make sure now that 00698 this port is only used once for every local IP. */ 00699 for (lpcb = tcp_listen_pcbs.listen_pcbs; lpcb != NULL; lpcb = lpcb->next) { 00700 if ((lpcb->local_port == pcb->local_port) && 00701 ip_addr_cmp(&lpcb->local_ip, &pcb->local_ip)) { 00702 /* this address/port is already used */ 00703 lpcb = NULL; 00704 res = ERR_USE; 00705 goto done; 00706 } 00707 } 00708 } 00709 #endif /* SO_REUSE */ 00710 lpcb = (struct tcp_pcb_listen *)memp_malloc(MEMP_TCP_PCB_LISTEN); 00711 if (lpcb == NULL) { 00712 res = ERR_MEM; 00713 goto done; 00714 } 00715 lpcb->callback_arg = pcb->callback_arg; 00716 lpcb->local_port = pcb->local_port; 00717 lpcb->state = LISTEN; 00718 lpcb->prio = pcb->prio; 00719 lpcb->so_options = pcb->so_options; 00720 lpcb->ttl = pcb->ttl; 00721 lpcb->tos = pcb->tos; 00722 #if LWIP_IPV4 && LWIP_IPV6 00723 IP_SET_TYPE_VAL(lpcb->remote_ip, pcb->local_ip.type); 00724 #endif /* LWIP_IPV4 && LWIP_IPV6 */ 00725 ip_addr_copy(lpcb->local_ip, pcb->local_ip); 00726 if (pcb->local_port != 0) { 00727 TCP_RMV(&tcp_bound_pcbs, pcb); 00728 } 00729 memp_free(MEMP_TCP_PCB, pcb); 00730 #if LWIP_CALLBACK_API 00731 lpcb->accept = tcp_accept_null; 00732 #endif /* LWIP_CALLBACK_API */ 00733 #if TCP_LISTEN_BACKLOG 00734 lpcb->accepts_pending = 0; 00735 tcp_backlog_set(lpcb, backlog); 00736 #endif /* TCP_LISTEN_BACKLOG */ 00737 TCP_REG(&tcp_listen_pcbs.pcbs, (struct tcp_pcb *)lpcb); 00738 res = ERR_OK; 00739 done: 00740 if (err != NULL) { 00741 *err = res; 00742 } 00743 return (struct tcp_pcb *)lpcb; 00744 } 00745 00746 /** 00747 * Update the state that tracks the available window space to advertise. 00748 * 00749 * Returns how much extra window would be advertised if we sent an 00750 * update now. 00751 */ 00752 u32_t 00753 tcp_update_rcv_ann_wnd(struct tcp_pcb *pcb) 00754 { 00755 u32_t new_right_edge = pcb->rcv_nxt + pcb->rcv_wnd; 00756 00757 if (TCP_SEQ_GEQ(new_right_edge, pcb->rcv_ann_right_edge + LWIP_MIN((TCP_WND / 2), pcb->mss))) { 00758 /* we can advertise more window */ 00759 pcb->rcv_ann_wnd = pcb->rcv_wnd; 00760 return new_right_edge - pcb->rcv_ann_right_edge; 00761 } else { 00762 if (TCP_SEQ_GT(pcb->rcv_nxt, pcb->rcv_ann_right_edge)) { 00763 /* Can happen due to other end sending out of advertised window, 00764 * but within actual available (but not yet advertised) window */ 00765 pcb->rcv_ann_wnd = 0; 00766 } else { 00767 /* keep the right edge of window constant */ 00768 u32_t new_rcv_ann_wnd = pcb->rcv_ann_right_edge - pcb->rcv_nxt; 00769 #if !LWIP_WND_SCALE 00770 LWIP_ASSERT("new_rcv_ann_wnd <= 0xffff", new_rcv_ann_wnd <= 0xffff); 00771 #endif 00772 pcb->rcv_ann_wnd = (tcpwnd_size_t)new_rcv_ann_wnd; 00773 } 00774 return 0; 00775 } 00776 } 00777 00778 /** 00779 * @ingroup tcp_raw 00780 * This function should be called by the application when it has 00781 * processed the data. The purpose is to advertise a larger window 00782 * when the data has been processed. 00783 * 00784 * @param pcb the tcp_pcb for which data is read 00785 * @param len the amount of bytes that have been read by the application 00786 */ 00787 void 00788 tcp_recved(struct tcp_pcb *pcb, u16_t len) 00789 { 00790 int wnd_inflation; 00791 00792 /* pcb->state LISTEN not allowed here */ 00793 LWIP_ASSERT("don't call tcp_recved for listen-pcbs", 00794 pcb->state != LISTEN); 00795 00796 pcb->rcv_wnd += len; 00797 if (pcb->rcv_wnd > TCP_WND_MAX(pcb)) { 00798 pcb->rcv_wnd = TCP_WND_MAX(pcb); 00799 } else if (pcb->rcv_wnd == 0) { 00800 /* rcv_wnd overflowed */ 00801 if ((pcb->state == CLOSE_WAIT) || (pcb->state == LAST_ACK)) { 00802 /* In passive close, we allow this, since the FIN bit is added to rcv_wnd 00803 by the stack itself, since it is not mandatory for an application 00804 to call tcp_recved() for the FIN bit, but e.g. the netconn API does so. */ 00805 pcb->rcv_wnd = TCP_WND_MAX(pcb); 00806 } else { 00807 LWIP_ASSERT("tcp_recved: len wrapped rcv_wnd\n", 0); 00808 } 00809 } 00810 00811 wnd_inflation = tcp_update_rcv_ann_wnd(pcb); 00812 00813 /* If the change in the right edge of window is significant (default 00814 * watermark is TCP_WND/4), then send an explicit update now. 00815 * Otherwise wait for a packet to be sent in the normal course of 00816 * events (or more window to be available later) */ 00817 if (wnd_inflation >= TCP_WND_UPDATE_THRESHOLD) { 00818 tcp_ack_now(pcb); 00819 tcp_output(pcb); 00820 } 00821 00822 LWIP_DEBUGF(TCP_DEBUG, ("tcp_recved: received %"U16_F" bytes, wnd %"TCPWNDSIZE_F" (%"TCPWNDSIZE_F").\n", 00823 len, pcb->rcv_wnd, (u16_t)(TCP_WND_MAX(pcb) - pcb->rcv_wnd))); 00824 } 00825 00826 /** 00827 * Allocate a new local TCP port. 00828 * 00829 * @return a new (free) local TCP port number 00830 */ 00831 static u16_t 00832 tcp_new_port(void) 00833 { 00834 u8_t i; 00835 u16_t n = 0; 00836 struct tcp_pcb *pcb; 00837 00838 again: 00839 if (tcp_port++ == TCP_LOCAL_PORT_RANGE_END) { 00840 tcp_port = TCP_LOCAL_PORT_RANGE_START; 00841 } 00842 /* Check all PCB lists. */ 00843 for (i = 0; i < NUM_TCP_PCB_LISTS; i++) { 00844 for (pcb = *tcp_pcb_lists[i]; pcb != NULL; pcb = pcb->next) { 00845 if (pcb->local_port == tcp_port) { 00846 if (++n > (TCP_LOCAL_PORT_RANGE_END - TCP_LOCAL_PORT_RANGE_START)) { 00847 return 0; 00848 } 00849 goto again; 00850 } 00851 } 00852 } 00853 return tcp_port; 00854 } 00855 00856 /** 00857 * @ingroup tcp_raw 00858 * Connects to another host. The function given as the "connected" 00859 * argument will be called when the connection has been established. 00860 * 00861 * @param pcb the tcp_pcb used to establish the connection 00862 * @param ipaddr the remote ip address to connect to 00863 * @param port the remote tcp port to connect to 00864 * @param connected callback function to call when connected (on error, 00865 the err calback will be called) 00866 * @return ERR_VAL if invalid arguments are given 00867 * ERR_OK if connect request has been sent 00868 * other err_t values if connect request couldn't be sent 00869 */ 00870 err_t 00871 tcp_connect(struct tcp_pcb *pcb, const ip_addr_t *ipaddr, u16_t port, 00872 tcp_connected_fn connected) 00873 { 00874 err_t ret; 00875 u32_t iss; 00876 u16_t old_local_port; 00877 00878 if ((pcb == NULL) || (ipaddr == NULL)) { 00879 return ERR_VAL; 00880 } 00881 00882 LWIP_ERROR("tcp_connect: can only connect from state CLOSED", pcb->state == CLOSED, return ERR_ISCONN); 00883 00884 LWIP_DEBUGF(TCP_DEBUG, ("tcp_connect to port %"U16_F"\n", port)); 00885 ip_addr_set(&pcb->remote_ip, ipaddr); 00886 pcb->remote_port = port; 00887 00888 /* check if we have a route to the remote host */ 00889 if (ip_addr_isany(&pcb->local_ip)) { 00890 /* no local IP address set, yet. */ 00891 struct netif *netif; 00892 const ip_addr_t *local_ip; 00893 ip_route_get_local_ip(&pcb->local_ip, &pcb->remote_ip, netif, local_ip); 00894 if ((netif == NULL) || (local_ip == NULL)) { 00895 /* Don't even try to send a SYN packet if we have no route 00896 since that will fail. */ 00897 return ERR_RTE; 00898 } 00899 /* Use the address as local address of the pcb. */ 00900 ip_addr_copy(pcb->local_ip, *local_ip); 00901 } 00902 00903 old_local_port = pcb->local_port; 00904 if (pcb->local_port == 0) { 00905 pcb->local_port = tcp_new_port(); 00906 if (pcb->local_port == 0) { 00907 return ERR_BUF; 00908 } 00909 } else { 00910 #if SO_REUSE 00911 if (ip_get_option(pcb, SOF_REUSEADDR)) { 00912 /* Since SOF_REUSEADDR allows reusing a local address, we have to make sure 00913 now that the 5-tuple is unique. */ 00914 struct tcp_pcb *cpcb; 00915 int i; 00916 /* Don't check listen- and bound-PCBs, check active- and TIME-WAIT PCBs. */ 00917 for (i = 2; i < NUM_TCP_PCB_LISTS; i++) { 00918 for (cpcb = *tcp_pcb_lists[i]; cpcb != NULL; cpcb = cpcb->next) { 00919 if ((cpcb->local_port == pcb->local_port) && 00920 (cpcb->remote_port == port) && 00921 ip_addr_cmp(&cpcb->local_ip, &pcb->local_ip) && 00922 ip_addr_cmp(&cpcb->remote_ip, ipaddr)) { 00923 /* linux returns EISCONN here, but ERR_USE should be OK for us */ 00924 return ERR_USE; 00925 } 00926 } 00927 } 00928 } 00929 #endif /* SO_REUSE */ 00930 } 00931 00932 iss = tcp_next_iss(pcb); 00933 pcb->rcv_nxt = 0; 00934 pcb->snd_nxt = iss; 00935 pcb->lastack = iss - 1; 00936 pcb->snd_wl2 = iss - 1; 00937 pcb->snd_lbb = iss - 1; 00938 /* Start with a window that does not need scaling. When window scaling is 00939 enabled and used, the window is enlarged when both sides agree on scaling. */ 00940 pcb->rcv_wnd = pcb->rcv_ann_wnd = TCPWND_MIN16(TCP_WND); 00941 pcb->rcv_ann_right_edge = pcb->rcv_nxt; 00942 pcb->snd_wnd = TCP_WND; 00943 /* As initial send MSS, we use TCP_MSS but limit it to 536. 00944 The send MSS is updated when an MSS option is received. */ 00945 pcb->mss = INITIAL_MSS; 00946 #if TCP_CALCULATE_EFF_SEND_MSS 00947 pcb->mss = tcp_eff_send_mss(pcb->mss, &pcb->local_ip, &pcb->remote_ip); 00948 #endif /* TCP_CALCULATE_EFF_SEND_MSS */ 00949 pcb->cwnd = 1; 00950 #if LWIP_CALLBACK_API 00951 pcb->connected = connected; 00952 #else /* LWIP_CALLBACK_API */ 00953 LWIP_UNUSED_ARG(connected); 00954 #endif /* LWIP_CALLBACK_API */ 00955 00956 /* Send a SYN together with the MSS option. */ 00957 ret = tcp_enqueue_flags(pcb, TCP_SYN); 00958 if (ret == ERR_OK) { 00959 /* SYN segment was enqueued, changed the pcbs state now */ 00960 pcb->state = SYN_SENT; 00961 if (old_local_port != 0) { 00962 TCP_RMV(&tcp_bound_pcbs, pcb); 00963 } 00964 TCP_REG_ACTIVE(pcb); 00965 MIB2_STATS_INC(mib2.tcpactiveopens); 00966 00967 tcp_output(pcb); 00968 } 00969 return ret; 00970 } 00971 00972 /** 00973 * Called every 500 ms and implements the retransmission timer and the timer that 00974 * removes PCBs that have been in TIME-WAIT for enough time. It also increments 00975 * various timers such as the inactivity timer in each PCB. 00976 * 00977 * Automatically called from tcp_tmr(). 00978 */ 00979 void 00980 tcp_slowtmr(void) 00981 { 00982 struct tcp_pcb *pcb, *prev; 00983 tcpwnd_size_t eff_wnd; 00984 u8_t pcb_remove; /* flag if a PCB should be removed */ 00985 u8_t pcb_reset; /* flag if a RST should be sent when removing */ 00986 err_t err; 00987 00988 err = ERR_OK; 00989 00990 ++tcp_ticks; 00991 ++tcp_timer_ctr; 00992 00993 tcp_slowtmr_start: 00994 /* Steps through all of the active PCBs. */ 00995 prev = NULL; 00996 pcb = tcp_active_pcbs; 00997 if (pcb == NULL) { 00998 LWIP_DEBUGF(TCP_DEBUG, ("tcp_slowtmr: no active pcbs\n")); 00999 } 01000 while (pcb != NULL) { 01001 LWIP_DEBUGF(TCP_DEBUG, ("tcp_slowtmr: processing active pcb\n")); 01002 LWIP_ASSERT("tcp_slowtmr: active pcb->state != CLOSED\n", pcb->state != CLOSED); 01003 LWIP_ASSERT("tcp_slowtmr: active pcb->state != LISTEN\n", pcb->state != LISTEN); 01004 LWIP_ASSERT("tcp_slowtmr: active pcb->state != TIME-WAIT\n", pcb->state != TIME_WAIT); 01005 if (pcb->last_timer == tcp_timer_ctr) { 01006 /* skip this pcb, we have already processed it */ 01007 pcb = pcb->next; 01008 continue; 01009 } 01010 pcb->last_timer = tcp_timer_ctr; 01011 01012 pcb_remove = 0; 01013 pcb_reset = 0; 01014 01015 if (pcb->state == SYN_SENT && pcb->nrtx >= TCP_SYNMAXRTX) { 01016 ++pcb_remove; 01017 LWIP_DEBUGF(TCP_DEBUG, ("tcp_slowtmr: max SYN retries reached\n")); 01018 } 01019 else if (pcb->nrtx >= TCP_MAXRTX) { 01020 ++pcb_remove; 01021 LWIP_DEBUGF(TCP_DEBUG, ("tcp_slowtmr: max DATA retries reached\n")); 01022 } else { 01023 if (pcb->persist_backoff > 0) { 01024 /* If snd_wnd is zero, use persist timer to send 1 byte probes 01025 * instead of using the standard retransmission mechanism. */ 01026 u8_t backoff_cnt = tcp_persist_backoff[pcb->persist_backoff-1]; 01027 if (pcb->persist_cnt < backoff_cnt) { 01028 pcb->persist_cnt++; 01029 } 01030 if (pcb->persist_cnt >= backoff_cnt) { 01031 if (tcp_zero_window_probe(pcb) == ERR_OK) { 01032 pcb->persist_cnt = 0; 01033 if (pcb->persist_backoff < sizeof(tcp_persist_backoff)) { 01034 pcb->persist_backoff++; 01035 } 01036 } 01037 } 01038 } else { 01039 /* Increase the retransmission timer if it is running */ 01040 if (pcb->rtime >= 0) { 01041 ++pcb->rtime; 01042 } 01043 01044 if (pcb->unacked != NULL && pcb->rtime >= pcb->rto) { 01045 /* Time for a retransmission. */ 01046 LWIP_DEBUGF(TCP_RTO_DEBUG, ("tcp_slowtmr: rtime %"S16_F 01047 " pcb->rto %"S16_F"\n", 01048 pcb->rtime, pcb->rto)); 01049 01050 /* Double retransmission time-out unless we are trying to 01051 * connect to somebody (i.e., we are in SYN_SENT). */ 01052 if (pcb->state != SYN_SENT) { 01053 u8_t backoff_idx = LWIP_MIN(pcb->nrtx, sizeof(tcp_backoff)-1); 01054 pcb->rto = ((pcb->sa >> 3) + pcb->sv) << tcp_backoff[backoff_idx]; 01055 } 01056 01057 /* Reset the retransmission timer. */ 01058 pcb->rtime = 0; 01059 01060 /* Reduce congestion window and ssthresh. */ 01061 eff_wnd = LWIP_MIN(pcb->cwnd, pcb->snd_wnd); 01062 pcb->ssthresh = eff_wnd >> 1; 01063 if (pcb->ssthresh < (tcpwnd_size_t)(pcb->mss << 1)) { 01064 pcb->ssthresh = (pcb->mss << 1); 01065 } 01066 pcb->cwnd = pcb->mss; 01067 LWIP_DEBUGF(TCP_CWND_DEBUG, ("tcp_slowtmr: cwnd %"TCPWNDSIZE_F 01068 " ssthresh %"TCPWNDSIZE_F"\n", 01069 pcb->cwnd, pcb->ssthresh)); 01070 01071 /* The following needs to be called AFTER cwnd is set to one 01072 mss - STJ */ 01073 tcp_rexmit_rto(pcb); 01074 } 01075 } 01076 } 01077 /* Check if this PCB has stayed too long in FIN-WAIT-2 */ 01078 if (pcb->state == FIN_WAIT_2) { 01079 /* If this PCB is in FIN_WAIT_2 because of SHUT_WR don't let it time out. */ 01080 if (pcb->flags & TF_RXCLOSED) { 01081 /* PCB was fully closed (either through close() or SHUT_RDWR): 01082 normal FIN-WAIT timeout handling. */ 01083 if ((u32_t)(tcp_ticks - pcb->tmr) > 01084 TCP_FIN_WAIT_TIMEOUT / TCP_SLOW_INTERVAL) { 01085 ++pcb_remove; 01086 LWIP_DEBUGF(TCP_DEBUG, ("tcp_slowtmr: removing pcb stuck in FIN-WAIT-2\n")); 01087 } 01088 } 01089 } 01090 01091 /* Check if KEEPALIVE should be sent */ 01092 if (ip_get_option(pcb, SOF_KEEPALIVE) && 01093 ((pcb->state == ESTABLISHED) || 01094 (pcb->state == CLOSE_WAIT))) { 01095 if ((u32_t)(tcp_ticks - pcb->tmr) > 01096 (pcb->keep_idle + TCP_KEEP_DUR(pcb)) / TCP_SLOW_INTERVAL) 01097 { 01098 LWIP_DEBUGF(TCP_DEBUG, ("tcp_slowtmr: KEEPALIVE timeout. Aborting connection to ")); 01099 ip_addr_debug_print(TCP_DEBUG, &pcb->remote_ip); 01100 LWIP_DEBUGF(TCP_DEBUG, ("\n")); 01101 01102 ++pcb_remove; 01103 ++pcb_reset; 01104 } else if ((u32_t)(tcp_ticks - pcb->tmr) > 01105 (pcb->keep_idle + pcb->keep_cnt_sent * TCP_KEEP_INTVL(pcb)) 01106 / TCP_SLOW_INTERVAL) 01107 { 01108 err = tcp_keepalive(pcb); 01109 if (err == ERR_OK) { 01110 pcb->keep_cnt_sent++; 01111 } 01112 } 01113 } 01114 01115 /* If this PCB has queued out of sequence data, but has been 01116 inactive for too long, will drop the data (it will eventually 01117 be retransmitted). */ 01118 #if TCP_QUEUE_OOSEQ 01119 if (pcb->ooseq != NULL && 01120 (u32_t)tcp_ticks - pcb->tmr >= pcb->rto * TCP_OOSEQ_TIMEOUT) { 01121 tcp_segs_free(pcb->ooseq); 01122 pcb->ooseq = NULL; 01123 LWIP_DEBUGF(TCP_CWND_DEBUG, ("tcp_slowtmr: dropping OOSEQ queued data\n")); 01124 } 01125 #endif /* TCP_QUEUE_OOSEQ */ 01126 01127 /* Check if this PCB has stayed too long in SYN-RCVD */ 01128 if (pcb->state == SYN_RCVD) { 01129 if ((u32_t)(tcp_ticks - pcb->tmr) > 01130 TCP_SYN_RCVD_TIMEOUT / TCP_SLOW_INTERVAL) { 01131 ++pcb_remove; 01132 LWIP_DEBUGF(TCP_DEBUG, ("tcp_slowtmr: removing pcb stuck in SYN-RCVD\n")); 01133 } 01134 } 01135 01136 /* Check if this PCB has stayed too long in LAST-ACK */ 01137 if (pcb->state == LAST_ACK) { 01138 if ((u32_t)(tcp_ticks - pcb->tmr) > 2 * TCP_MSL / TCP_SLOW_INTERVAL) { 01139 ++pcb_remove; 01140 LWIP_DEBUGF(TCP_DEBUG, ("tcp_slowtmr: removing pcb stuck in LAST-ACK\n")); 01141 } 01142 } 01143 01144 /* If the PCB should be removed, do it. */ 01145 if (pcb_remove) { 01146 struct tcp_pcb *pcb2; 01147 #if LWIP_CALLBACK_API 01148 tcp_err_fn err_fn = pcb->errf; 01149 #endif /* LWIP_CALLBACK_API */ 01150 void *err_arg; 01151 enum tcp_state last_state; 01152 tcp_pcb_purge(pcb); 01153 /* Remove PCB from tcp_active_pcbs list. */ 01154 if (prev != NULL) { 01155 LWIP_ASSERT("tcp_slowtmr: middle tcp != tcp_active_pcbs", pcb != tcp_active_pcbs); 01156 prev->next = pcb->next; 01157 } else { 01158 /* This PCB was the first. */ 01159 LWIP_ASSERT("tcp_slowtmr: first pcb == tcp_active_pcbs", tcp_active_pcbs == pcb); 01160 tcp_active_pcbs = pcb->next; 01161 } 01162 01163 if (pcb_reset) { 01164 tcp_rst(pcb->snd_nxt, pcb->rcv_nxt, &pcb->local_ip, &pcb->remote_ip, 01165 pcb->local_port, pcb->remote_port); 01166 } 01167 01168 err_arg = pcb->callback_arg; 01169 last_state = pcb->state; 01170 pcb2 = pcb; 01171 pcb = pcb->next; 01172 memp_free(MEMP_TCP_PCB, pcb2); 01173 01174 tcp_active_pcbs_changed = 0; 01175 TCP_EVENT_ERR(last_state, err_fn, err_arg, ERR_ABRT); 01176 if (tcp_active_pcbs_changed) { 01177 goto tcp_slowtmr_start; 01178 } 01179 } else { 01180 /* get the 'next' element now and work with 'prev' below (in case of abort) */ 01181 prev = pcb; 01182 pcb = pcb->next; 01183 01184 /* We check if we should poll the connection. */ 01185 ++prev->polltmr; 01186 if (prev->polltmr >= prev->pollinterval) { 01187 prev->polltmr = 0; 01188 LWIP_DEBUGF(TCP_DEBUG, ("tcp_slowtmr: polling application\n")); 01189 tcp_active_pcbs_changed = 0; 01190 TCP_EVENT_POLL(prev, err); 01191 if (tcp_active_pcbs_changed) { 01192 goto tcp_slowtmr_start; 01193 } 01194 /* if err == ERR_ABRT, 'prev' is already deallocated */ 01195 if (err == ERR_OK) { 01196 tcp_output(prev); 01197 } 01198 } 01199 } 01200 } 01201 01202 01203 /* Steps through all of the TIME-WAIT PCBs. */ 01204 prev = NULL; 01205 pcb = tcp_tw_pcbs; 01206 while (pcb != NULL) { 01207 LWIP_ASSERT("tcp_slowtmr: TIME-WAIT pcb->state == TIME-WAIT", pcb->state == TIME_WAIT); 01208 pcb_remove = 0; 01209 01210 /* Check if this PCB has stayed long enough in TIME-WAIT */ 01211 if ((u32_t)(tcp_ticks - pcb->tmr) > 2 * TCP_MSL / TCP_SLOW_INTERVAL) { 01212 ++pcb_remove; 01213 } 01214 01215 /* If the PCB should be removed, do it. */ 01216 if (pcb_remove) { 01217 struct tcp_pcb *pcb2; 01218 tcp_pcb_purge(pcb); 01219 /* Remove PCB from tcp_tw_pcbs list. */ 01220 if (prev != NULL) { 01221 LWIP_ASSERT("tcp_slowtmr: middle tcp != tcp_tw_pcbs", pcb != tcp_tw_pcbs); 01222 prev->next = pcb->next; 01223 } else { 01224 /* This PCB was the first. */ 01225 LWIP_ASSERT("tcp_slowtmr: first pcb == tcp_tw_pcbs", tcp_tw_pcbs == pcb); 01226 tcp_tw_pcbs = pcb->next; 01227 } 01228 pcb2 = pcb; 01229 pcb = pcb->next; 01230 memp_free(MEMP_TCP_PCB, pcb2); 01231 } else { 01232 prev = pcb; 01233 pcb = pcb->next; 01234 } 01235 } 01236 } 01237 01238 /** 01239 * Is called every TCP_FAST_INTERVAL (250 ms) and process data previously 01240 * "refused" by upper layer (application) and sends delayed ACKs. 01241 * 01242 * Automatically called from tcp_tmr(). 01243 */ 01244 void 01245 tcp_fasttmr(void) 01246 { 01247 struct tcp_pcb *pcb; 01248 01249 ++tcp_timer_ctr; 01250 01251 tcp_fasttmr_start: 01252 pcb = tcp_active_pcbs; 01253 01254 while (pcb != NULL) { 01255 if (pcb->last_timer != tcp_timer_ctr) { 01256 struct tcp_pcb *next; 01257 pcb->last_timer = tcp_timer_ctr; 01258 /* send delayed ACKs */ 01259 if (pcb->flags & TF_ACK_DELAY) { 01260 LWIP_DEBUGF(TCP_DEBUG, ("tcp_fasttmr: delayed ACK\n")); 01261 tcp_ack_now(pcb); 01262 tcp_output(pcb); 01263 pcb->flags &= ~(TF_ACK_DELAY | TF_ACK_NOW); 01264 } 01265 /* send pending FIN */ 01266 if (pcb->flags & TF_CLOSEPEND) { 01267 LWIP_DEBUGF(TCP_DEBUG, ("tcp_fasttmr: pending FIN\n")); 01268 pcb->flags &= ~(TF_CLOSEPEND); 01269 tcp_close_shutdown_fin(pcb); 01270 } 01271 01272 next = pcb->next; 01273 01274 /* If there is data which was previously "refused" by upper layer */ 01275 if (pcb->refused_data != NULL) { 01276 tcp_active_pcbs_changed = 0; 01277 tcp_process_refused_data(pcb); 01278 if (tcp_active_pcbs_changed) { 01279 /* application callback has changed the pcb list: restart the loop */ 01280 goto tcp_fasttmr_start; 01281 } 01282 } 01283 pcb = next; 01284 } else { 01285 pcb = pcb->next; 01286 } 01287 } 01288 } 01289 01290 /** Call tcp_output for all active pcbs that have TF_NAGLEMEMERR set */ 01291 void 01292 tcp_txnow(void) 01293 { 01294 struct tcp_pcb *pcb; 01295 01296 for (pcb = tcp_active_pcbs; pcb != NULL; pcb = pcb->next) { 01297 if (pcb->flags & TF_NAGLEMEMERR) { 01298 tcp_output(pcb); 01299 } 01300 } 01301 } 01302 01303 /** Pass pcb->refused_data to the recv callback */ 01304 err_t 01305 tcp_process_refused_data(struct tcp_pcb *pcb) 01306 { 01307 #if TCP_QUEUE_OOSEQ && LWIP_WND_SCALE 01308 struct pbuf *rest; 01309 while (pcb->refused_data != NULL) 01310 #endif /* TCP_QUEUE_OOSEQ && LWIP_WND_SCALE */ 01311 { 01312 err_t err; 01313 u8_t refused_flags = pcb->refused_data->flags; 01314 /* set pcb->refused_data to NULL in case the callback frees it and then 01315 closes the pcb */ 01316 struct pbuf *refused_data = pcb->refused_data; 01317 #if TCP_QUEUE_OOSEQ && LWIP_WND_SCALE 01318 pbuf_split_64k(refused_data, &rest); 01319 pcb->refused_data = rest; 01320 #else /* TCP_QUEUE_OOSEQ && LWIP_WND_SCALE */ 01321 pcb->refused_data = NULL; 01322 #endif /* TCP_QUEUE_OOSEQ && LWIP_WND_SCALE */ 01323 /* Notify again application with data previously received. */ 01324 LWIP_DEBUGF(TCP_INPUT_DEBUG, ("tcp_input: notify kept packet\n")); 01325 TCP_EVENT_RECV(pcb, refused_data, ERR_OK, err); 01326 if (err == ERR_OK) { 01327 /* did refused_data include a FIN? */ 01328 if (refused_flags & PBUF_FLAG_TCP_FIN 01329 #if TCP_QUEUE_OOSEQ && LWIP_WND_SCALE 01330 && (rest == NULL) 01331 #endif /* TCP_QUEUE_OOSEQ && LWIP_WND_SCALE */ 01332 ) { 01333 /* correct rcv_wnd as the application won't call tcp_recved() 01334 for the FIN's seqno */ 01335 if (pcb->rcv_wnd != TCP_WND_MAX(pcb)) { 01336 pcb->rcv_wnd++; 01337 } 01338 TCP_EVENT_CLOSED(pcb, err); 01339 if (err == ERR_ABRT) { 01340 return ERR_ABRT; 01341 } 01342 } 01343 } else if (err == ERR_ABRT) { 01344 /* if err == ERR_ABRT, 'pcb' is already deallocated */ 01345 /* Drop incoming packets because pcb is "full" (only if the incoming 01346 segment contains data). */ 01347 LWIP_DEBUGF(TCP_INPUT_DEBUG, ("tcp_input: drop incoming packets, because pcb is \"full\"\n")); 01348 return ERR_ABRT; 01349 } else { 01350 /* data is still refused, pbuf is still valid (go on for ACK-only packets) */ 01351 #if TCP_QUEUE_OOSEQ && LWIP_WND_SCALE 01352 if (rest != NULL) { 01353 pbuf_cat(refused_data, rest); 01354 } 01355 #endif /* TCP_QUEUE_OOSEQ && LWIP_WND_SCALE */ 01356 pcb->refused_data = refused_data; 01357 return ERR_INPROGRESS; 01358 } 01359 } 01360 return ERR_OK; 01361 } 01362 01363 /** 01364 * Deallocates a list of TCP segments (tcp_seg structures). 01365 * 01366 * @param seg tcp_seg list of TCP segments to free 01367 */ 01368 void 01369 tcp_segs_free(struct tcp_seg *seg) 01370 { 01371 while (seg != NULL) { 01372 struct tcp_seg *next = seg->next; 01373 tcp_seg_free(seg); 01374 seg = next; 01375 } 01376 } 01377 01378 /** 01379 * Frees a TCP segment (tcp_seg structure). 01380 * 01381 * @param seg single tcp_seg to free 01382 */ 01383 void 01384 tcp_seg_free(struct tcp_seg *seg) 01385 { 01386 if (seg != NULL) { 01387 if (seg->p != NULL) { 01388 pbuf_free(seg->p); 01389 #if TCP_DEBUG 01390 seg->p = NULL; 01391 #endif /* TCP_DEBUG */ 01392 } 01393 memp_free(MEMP_TCP_SEG, seg); 01394 } 01395 } 01396 01397 /** 01398 * Sets the priority of a connection. 01399 * 01400 * @param pcb the tcp_pcb to manipulate 01401 * @param prio new priority 01402 */ 01403 void 01404 tcp_setprio(struct tcp_pcb *pcb, u8_t prio) 01405 { 01406 pcb->prio = prio; 01407 } 01408 01409 #if TCP_QUEUE_OOSEQ 01410 /** 01411 * Returns a copy of the given TCP segment. 01412 * The pbuf and data are not copied, only the pointers 01413 * 01414 * @param seg the old tcp_seg 01415 * @return a copy of seg 01416 */ 01417 struct tcp_seg * 01418 tcp_seg_copy(struct tcp_seg *seg) 01419 { 01420 struct tcp_seg *cseg; 01421 01422 cseg = (struct tcp_seg *)memp_malloc(MEMP_TCP_SEG); 01423 if (cseg == NULL) { 01424 return NULL; 01425 } 01426 SMEMCPY((u8_t *)cseg, (const u8_t *)seg, sizeof(struct tcp_seg)); 01427 pbuf_ref(cseg->p); 01428 return cseg; 01429 } 01430 #endif /* TCP_QUEUE_OOSEQ */ 01431 01432 #if LWIP_CALLBACK_API 01433 /** 01434 * Default receive callback that is called if the user didn't register 01435 * a recv callback for the pcb. 01436 */ 01437 err_t 01438 tcp_recv_null(void *arg, struct tcp_pcb *pcb, struct pbuf *p, err_t err) 01439 { 01440 LWIP_UNUSED_ARG(arg); 01441 if (p != NULL) { 01442 tcp_recved(pcb, p->tot_len); 01443 pbuf_free(p); 01444 } else if (err == ERR_OK) { 01445 return tcp_close(pcb); 01446 } 01447 return ERR_OK; 01448 } 01449 #endif /* LWIP_CALLBACK_API */ 01450 01451 /** 01452 * Kills the oldest active connection that has the same or lower priority than 01453 * 'prio'. 01454 * 01455 * @param prio minimum priority 01456 */ 01457 static void 01458 tcp_kill_prio(u8_t prio) 01459 { 01460 struct tcp_pcb *pcb, *inactive; 01461 u32_t inactivity; 01462 u8_t mprio; 01463 01464 mprio = LWIP_MIN(TCP_PRIO_MAX, prio); 01465 01466 /* We kill the oldest active connection that has lower priority than prio. */ 01467 inactivity = 0; 01468 inactive = NULL; 01469 for (pcb = tcp_active_pcbs; pcb != NULL; pcb = pcb->next) { 01470 if (pcb->prio <= mprio && 01471 (u32_t)(tcp_ticks - pcb->tmr) >= inactivity) { 01472 inactivity = tcp_ticks - pcb->tmr; 01473 inactive = pcb; 01474 mprio = pcb->prio; 01475 } 01476 } 01477 if (inactive != NULL) { 01478 LWIP_DEBUGF(TCP_DEBUG, ("tcp_kill_prio: killing oldest PCB %p (%"S32_F")\n", 01479 (void *)inactive, inactivity)); 01480 tcp_abort(inactive); 01481 } 01482 } 01483 01484 /** 01485 * Kills the oldest connection that is in specific state. 01486 * Called from tcp_alloc() for LAST_ACK and CLOSING if no more connections are available. 01487 */ 01488 static void 01489 tcp_kill_state(enum tcp_state state) 01490 { 01491 struct tcp_pcb *pcb, *inactive; 01492 u32_t inactivity; 01493 01494 LWIP_ASSERT("invalid state", (state == CLOSING) || (state == LAST_ACK)); 01495 01496 inactivity = 0; 01497 inactive = NULL; 01498 /* Go through the list of active pcbs and get the oldest pcb that is in state 01499 CLOSING/LAST_ACK. */ 01500 for (pcb = tcp_active_pcbs; pcb != NULL; pcb = pcb->next) { 01501 if (pcb->state == state) { 01502 if ((u32_t)(tcp_ticks - pcb->tmr) >= inactivity) { 01503 inactivity = tcp_ticks - pcb->tmr; 01504 inactive = pcb; 01505 } 01506 } 01507 } 01508 if (inactive != NULL) { 01509 LWIP_DEBUGF(TCP_DEBUG, ("tcp_kill_closing: killing oldest %s PCB %p (%"S32_F")\n", 01510 tcp_state_str[state], (void *)inactive, inactivity)); 01511 /* Don't send a RST, since no data is lost. */ 01512 tcp_abandon(inactive, 0); 01513 } 01514 } 01515 01516 /** 01517 * Kills the oldest connection that is in TIME_WAIT state. 01518 * Called from tcp_alloc() if no more connections are available. 01519 */ 01520 static void 01521 tcp_kill_timewait(void) 01522 { 01523 struct tcp_pcb *pcb, *inactive; 01524 u32_t inactivity; 01525 01526 inactivity = 0; 01527 inactive = NULL; 01528 /* Go through the list of TIME_WAIT pcbs and get the oldest pcb. */ 01529 for (pcb = tcp_tw_pcbs; pcb != NULL; pcb = pcb->next) { 01530 if ((u32_t)(tcp_ticks - pcb->tmr) >= inactivity) { 01531 inactivity = tcp_ticks - pcb->tmr; 01532 inactive = pcb; 01533 } 01534 } 01535 if (inactive != NULL) { 01536 LWIP_DEBUGF(TCP_DEBUG, ("tcp_kill_timewait: killing oldest TIME-WAIT PCB %p (%"S32_F")\n", 01537 (void *)inactive, inactivity)); 01538 tcp_abort(inactive); 01539 } 01540 } 01541 01542 /** 01543 * Allocate a new tcp_pcb structure. 01544 * 01545 * @param prio priority for the new pcb 01546 * @return a new tcp_pcb that initially is in state CLOSED 01547 */ 01548 struct tcp_pcb * 01549 tcp_alloc(u8_t prio) 01550 { 01551 struct tcp_pcb *pcb; 01552 01553 pcb = (struct tcp_pcb *)memp_malloc(MEMP_TCP_PCB); 01554 if (pcb == NULL) { 01555 /* Try killing oldest connection in TIME-WAIT. */ 01556 LWIP_DEBUGF(TCP_DEBUG, ("tcp_alloc: killing off oldest TIME-WAIT connection\n")); 01557 tcp_kill_timewait(); 01558 /* Try to allocate a tcp_pcb again. */ 01559 pcb = (struct tcp_pcb *)memp_malloc(MEMP_TCP_PCB); 01560 if (pcb == NULL) { 01561 /* Try killing oldest connection in LAST-ACK (these wouldn't go to TIME-WAIT). */ 01562 LWIP_DEBUGF(TCP_DEBUG, ("tcp_alloc: killing off oldest LAST-ACK connection\n")); 01563 tcp_kill_state(LAST_ACK); 01564 /* Try to allocate a tcp_pcb again. */ 01565 pcb = (struct tcp_pcb *)memp_malloc(MEMP_TCP_PCB); 01566 if (pcb == NULL) { 01567 /* Try killing oldest connection in CLOSING. */ 01568 LWIP_DEBUGF(TCP_DEBUG, ("tcp_alloc: killing off oldest CLOSING connection\n")); 01569 tcp_kill_state(CLOSING); 01570 /* Try to allocate a tcp_pcb again. */ 01571 pcb = (struct tcp_pcb *)memp_malloc(MEMP_TCP_PCB); 01572 if (pcb == NULL) { 01573 /* Try killing active connections with lower priority than the new one. */ 01574 LWIP_DEBUGF(TCP_DEBUG, ("tcp_alloc: killing connection with prio lower than %d\n", prio)); 01575 tcp_kill_prio(prio); 01576 /* Try to allocate a tcp_pcb again. */ 01577 pcb = (struct tcp_pcb *)memp_malloc(MEMP_TCP_PCB); 01578 if (pcb != NULL) { 01579 /* adjust err stats: memp_malloc failed multiple times before */ 01580 MEMP_STATS_DEC(err, MEMP_TCP_PCB); 01581 } 01582 } 01583 if (pcb != NULL) { 01584 /* adjust err stats: memp_malloc failed multiple times before */ 01585 MEMP_STATS_DEC(err, MEMP_TCP_PCB); 01586 } 01587 } 01588 if (pcb != NULL) { 01589 /* adjust err stats: memp_malloc failed multiple times before */ 01590 MEMP_STATS_DEC(err, MEMP_TCP_PCB); 01591 } 01592 } 01593 if (pcb != NULL) { 01594 /* adjust err stats: memp_malloc failed above */ 01595 MEMP_STATS_DEC(err, MEMP_TCP_PCB); 01596 } 01597 } 01598 if (pcb != NULL) { 01599 /* zero out the whole pcb, so there is no need to initialize members to zero */ 01600 memset(pcb, 0, sizeof(struct tcp_pcb)); 01601 pcb->prio = prio; 01602 pcb->snd_buf = TCP_SND_BUF; 01603 /* Start with a window that does not need scaling. When window scaling is 01604 enabled and used, the window is enlarged when both sides agree on scaling. */ 01605 pcb->rcv_wnd = pcb->rcv_ann_wnd = TCPWND_MIN16(TCP_WND); 01606 pcb->ttl = TCP_TTL; 01607 /* As initial send MSS, we use TCP_MSS but limit it to 536. 01608 The send MSS is updated when an MSS option is received. */ 01609 pcb->mss = INITIAL_MSS; 01610 pcb->rto = 3000 / TCP_SLOW_INTERVAL; 01611 pcb->sv = 3000 / TCP_SLOW_INTERVAL; 01612 pcb->rtime = -1; 01613 pcb->cwnd = 1; 01614 pcb->tmr = tcp_ticks; 01615 pcb->last_timer = tcp_timer_ctr; 01616 01617 /* RFC 5681 recommends setting ssthresh abritrarily high and gives an example 01618 of using the largest advertised receive window. We've seen complications with 01619 receiving TCPs that use window scaling and/or window auto-tuning where the 01620 initial advertised window is very small and then grows rapidly once the 01621 connection is established. To avoid these complications, we set ssthresh to the 01622 largest effective cwnd (amount of in-flight data) that the sender can have. */ 01623 pcb->ssthresh = TCP_SND_BUF; 01624 01625 #if LWIP_CALLBACK_API 01626 pcb->recv = tcp_recv_null; 01627 #endif /* LWIP_CALLBACK_API */ 01628 01629 /* Init KEEPALIVE timer */ 01630 pcb->keep_idle = TCP_KEEPIDLE_DEFAULT; 01631 01632 #if LWIP_TCP_KEEPALIVE 01633 pcb->keep_intvl = TCP_KEEPINTVL_DEFAULT; 01634 pcb->keep_cnt = TCP_KEEPCNT_DEFAULT; 01635 #endif /* LWIP_TCP_KEEPALIVE */ 01636 } 01637 return pcb; 01638 } 01639 01640 /** 01641 * @ingroup tcp_raw 01642 * Creates a new TCP protocol control block but doesn't place it on 01643 * any of the TCP PCB lists. 01644 * The pcb is not put on any list until binding using tcp_bind(). 01645 * 01646 * @internal: Maybe there should be a idle TCP PCB list where these 01647 * PCBs are put on. Port reservation using tcp_bind() is implemented but 01648 * allocated pcbs that are not bound can't be killed automatically if wanting 01649 * to allocate a pcb with higher prio (@see tcp_kill_prio()) 01650 * 01651 * @return a new tcp_pcb that initially is in state CLOSED 01652 */ 01653 struct tcp_pcb * 01654 tcp_new(void) 01655 { 01656 return tcp_alloc(TCP_PRIO_NORMAL); 01657 } 01658 01659 /** 01660 * @ingroup tcp_raw 01661 * Creates a new TCP protocol control block but doesn't 01662 * place it on any of the TCP PCB lists. 01663 * The pcb is not put on any list until binding using tcp_bind(). 01664 * 01665 * @param type IP address type, see @ref lwip_ip_addr_type definitions. 01666 * If you want to listen to IPv4 and IPv6 (dual-stack) connections, 01667 * supply @ref IPADDR_TYPE_ANY as argument and bind to @ref IP_ANY_TYPE. 01668 * @return a new tcp_pcb that initially is in state CLOSED 01669 */ 01670 struct tcp_pcb * 01671 tcp_new_ip_type(u8_t type) 01672 { 01673 struct tcp_pcb * pcb; 01674 pcb = tcp_alloc(TCP_PRIO_NORMAL); 01675 #if LWIP_IPV4 && LWIP_IPV6 01676 if (pcb != NULL) { 01677 IP_SET_TYPE_VAL(pcb->local_ip, type); 01678 IP_SET_TYPE_VAL(pcb->remote_ip, type); 01679 } 01680 #else 01681 LWIP_UNUSED_ARG(type); 01682 #endif /* LWIP_IPV4 && LWIP_IPV6 */ 01683 return pcb; 01684 } 01685 01686 /** 01687 * @ingroup tcp_raw 01688 * Used to specify the argument that should be passed callback 01689 * functions. 01690 * 01691 * @param pcb tcp_pcb to set the callback argument 01692 * @param arg void pointer argument to pass to callback functions 01693 */ 01694 void 01695 tcp_arg(struct tcp_pcb *pcb, void *arg) 01696 { 01697 /* This function is allowed to be called for both listen pcbs and 01698 connection pcbs. */ 01699 if (pcb != NULL) { 01700 pcb->callback_arg = arg; 01701 } 01702 } 01703 #if LWIP_CALLBACK_API 01704 01705 /** 01706 * @ingroup tcp_raw 01707 * Used to specify the function that should be called when a TCP 01708 * connection receives data. 01709 * 01710 * @param pcb tcp_pcb to set the recv callback 01711 * @param recv callback function to call for this pcb when data is received 01712 */ 01713 void 01714 tcp_recv(struct tcp_pcb *pcb, tcp_recv_fn recv) 01715 { 01716 if (pcb != NULL) { 01717 LWIP_ASSERT("invalid socket state for recv callback", pcb->state != LISTEN); 01718 pcb->recv = recv; 01719 } 01720 } 01721 01722 /** 01723 * @ingroup tcp_raw 01724 * Used to specify the function that should be called when TCP data 01725 * has been successfully delivered to the remote host. 01726 * 01727 * @param pcb tcp_pcb to set the sent callback 01728 * @param sent callback function to call for this pcb when data is successfully sent 01729 */ 01730 void 01731 tcp_sent(struct tcp_pcb *pcb, tcp_sent_fn sent) 01732 { 01733 if (pcb != NULL) { 01734 LWIP_ASSERT("invalid socket state for sent callback", pcb->state != LISTEN); 01735 pcb->sent = sent; 01736 } 01737 } 01738 01739 /** 01740 * @ingroup tcp_raw 01741 * Used to specify the function that should be called when a fatal error 01742 * has occurred on the connection. 01743 * 01744 * @note The corresponding pcb is already freed when this callback is called! 01745 * 01746 * @param pcb tcp_pcb to set the err callback 01747 * @param err callback function to call for this pcb when a fatal error 01748 * has occurred on the connection 01749 */ 01750 void 01751 tcp_err(struct tcp_pcb *pcb, tcp_err_fn err) 01752 { 01753 if (pcb != NULL) { 01754 LWIP_ASSERT("invalid socket state for err callback", pcb->state != LISTEN); 01755 pcb->errf = err; 01756 } 01757 } 01758 01759 /** 01760 * @ingroup tcp_raw 01761 * Used for specifying the function that should be called when a 01762 * LISTENing connection has been connected to another host. 01763 * 01764 * @param pcb tcp_pcb to set the accept callback 01765 * @param accept callback function to call for this pcb when LISTENing 01766 * connection has been connected to another host 01767 */ 01768 void 01769 tcp_accept(struct tcp_pcb *pcb, tcp_accept_fn accept) 01770 { 01771 if ((pcb != NULL) && (pcb->state == LISTEN)) { 01772 struct tcp_pcb_listen *lpcb = (struct tcp_pcb_listen*)pcb; 01773 lpcb->accept = accept; 01774 } 01775 } 01776 #endif /* LWIP_CALLBACK_API */ 01777 01778 01779 /** 01780 * @ingroup tcp_raw 01781 * Used to specify the function that should be called periodically 01782 * from TCP. The interval is specified in terms of the TCP coarse 01783 * timer interval, which is called twice a second. 01784 * 01785 */ 01786 void 01787 tcp_poll(struct tcp_pcb *pcb, tcp_poll_fn poll, u8_t interval) 01788 { 01789 LWIP_ASSERT("invalid socket state for poll", pcb->state != LISTEN); 01790 #if LWIP_CALLBACK_API 01791 pcb->poll = poll; 01792 #else /* LWIP_CALLBACK_API */ 01793 LWIP_UNUSED_ARG(poll); 01794 #endif /* LWIP_CALLBACK_API */ 01795 pcb->pollinterval = interval; 01796 } 01797 01798 /** 01799 * Purges a TCP PCB. Removes any buffered data and frees the buffer memory 01800 * (pcb->ooseq, pcb->unsent and pcb->unacked are freed). 01801 * 01802 * @param pcb tcp_pcb to purge. The pcb itself is not deallocated! 01803 */ 01804 void 01805 tcp_pcb_purge(struct tcp_pcb *pcb) 01806 { 01807 if (pcb->state != CLOSED && 01808 pcb->state != TIME_WAIT && 01809 pcb->state != LISTEN) { 01810 01811 LWIP_DEBUGF(TCP_DEBUG, ("tcp_pcb_purge\n")); 01812 01813 tcp_backlog_accepted(pcb); 01814 01815 if (pcb->refused_data != NULL) { 01816 LWIP_DEBUGF(TCP_DEBUG, ("tcp_pcb_purge: data left on ->refused_data\n")); 01817 pbuf_free(pcb->refused_data); 01818 pcb->refused_data = NULL; 01819 } 01820 if (pcb->unsent != NULL) { 01821 LWIP_DEBUGF(TCP_DEBUG, ("tcp_pcb_purge: not all data sent\n")); 01822 } 01823 if (pcb->unacked != NULL) { 01824 LWIP_DEBUGF(TCP_DEBUG, ("tcp_pcb_purge: data left on ->unacked\n")); 01825 } 01826 #if TCP_QUEUE_OOSEQ 01827 if (pcb->ooseq != NULL) { 01828 LWIP_DEBUGF(TCP_DEBUG, ("tcp_pcb_purge: data left on ->ooseq\n")); 01829 } 01830 tcp_segs_free(pcb->ooseq); 01831 pcb->ooseq = NULL; 01832 #endif /* TCP_QUEUE_OOSEQ */ 01833 01834 /* Stop the retransmission timer as it will expect data on unacked 01835 queue if it fires */ 01836 pcb->rtime = -1; 01837 01838 tcp_segs_free(pcb->unsent); 01839 tcp_segs_free(pcb->unacked); 01840 pcb->unacked = pcb->unsent = NULL; 01841 #if TCP_OVERSIZE 01842 pcb->unsent_oversize = 0; 01843 #endif /* TCP_OVERSIZE */ 01844 } 01845 } 01846 01847 /** 01848 * Purges the PCB and removes it from a PCB list. Any delayed ACKs are sent first. 01849 * 01850 * @param pcblist PCB list to purge. 01851 * @param pcb tcp_pcb to purge. The pcb itself is NOT deallocated! 01852 */ 01853 void 01854 tcp_pcb_remove(struct tcp_pcb **pcblist, struct tcp_pcb *pcb) 01855 { 01856 TCP_RMV(pcblist, pcb); 01857 01858 tcp_pcb_purge(pcb); 01859 01860 /* if there is an outstanding delayed ACKs, send it */ 01861 if (pcb->state != TIME_WAIT && 01862 pcb->state != LISTEN && 01863 pcb->flags & TF_ACK_DELAY) { 01864 pcb->flags |= TF_ACK_NOW; 01865 tcp_output(pcb); 01866 } 01867 01868 if (pcb->state != LISTEN) { 01869 LWIP_ASSERT("unsent segments leaking", pcb->unsent == NULL); 01870 LWIP_ASSERT("unacked segments leaking", pcb->unacked == NULL); 01871 #if TCP_QUEUE_OOSEQ 01872 LWIP_ASSERT("ooseq segments leaking", pcb->ooseq == NULL); 01873 #endif /* TCP_QUEUE_OOSEQ */ 01874 } 01875 01876 pcb->state = CLOSED; 01877 /* reset the local port to prevent the pcb from being 'bound' */ 01878 pcb->local_port = 0; 01879 01880 LWIP_ASSERT("tcp_pcb_remove: tcp_pcbs_sane()", tcp_pcbs_sane()); 01881 } 01882 01883 /** 01884 * Calculates a new initial sequence number for new connections. 01885 * 01886 * @return u32_t pseudo random sequence number 01887 */ 01888 u32_t 01889 tcp_next_iss(struct tcp_pcb *pcb) 01890 { 01891 #ifdef LWIP_HOOK_TCP_ISN 01892 return LWIP_HOOK_TCP_ISN(&pcb->local_ip, pcb->local_port, &pcb->remote_ip, pcb->remote_port); 01893 #else /* LWIP_HOOK_TCP_ISN */ 01894 static u32_t iss = 6510; 01895 01896 LWIP_UNUSED_ARG(pcb); 01897 01898 iss += tcp_ticks; /* XXX */ 01899 return iss; 01900 #endif /* LWIP_HOOK_TCP_ISN */ 01901 } 01902 01903 #if TCP_CALCULATE_EFF_SEND_MSS 01904 /** 01905 * Calculates the effective send mss that can be used for a specific IP address 01906 * by using ip_route to determine the netif used to send to the address and 01907 * calculating the minimum of TCP_MSS and that netif's mtu (if set). 01908 */ 01909 u16_t 01910 tcp_eff_send_mss_impl(u16_t sendmss, const ip_addr_t *dest 01911 #if LWIP_IPV6 || LWIP_IPV4_SRC_ROUTING 01912 , const ip_addr_t *src 01913 #endif /* LWIP_IPV6 || LWIP_IPV4_SRC_ROUTING */ 01914 ) 01915 { 01916 u16_t mss_s; 01917 struct netif *outif; 01918 s16_t mtu; 01919 01920 outif = ip_route(src, dest); 01921 #if LWIP_IPV6 01922 #if LWIP_IPV4 01923 if (IP_IS_V6(dest)) 01924 #endif /* LWIP_IPV4 */ 01925 { 01926 /* First look in destination cache, to see if there is a Path MTU. */ 01927 mtu = nd6_get_destination_mtu(ip_2_ip6(dest), outif); 01928 } 01929 #if LWIP_IPV4 01930 else 01931 #endif /* LWIP_IPV4 */ 01932 #endif /* LWIP_IPV6 */ 01933 #if LWIP_IPV4 01934 { 01935 if (outif == NULL) { 01936 return sendmss; 01937 } 01938 mtu = outif->mtu; 01939 } 01940 #endif /* LWIP_IPV4 */ 01941 01942 if (mtu != 0) { 01943 #if LWIP_IPV6 01944 #if LWIP_IPV4 01945 if (IP_IS_V6(dest)) 01946 #endif /* LWIP_IPV4 */ 01947 { 01948 mss_s = mtu - IP6_HLEN - TCP_HLEN; 01949 } 01950 #if LWIP_IPV4 01951 else 01952 #endif /* LWIP_IPV4 */ 01953 #endif /* LWIP_IPV6 */ 01954 #if LWIP_IPV4 01955 { 01956 mss_s = mtu - IP_HLEN - TCP_HLEN; 01957 } 01958 #endif /* LWIP_IPV4 */ 01959 /* RFC 1122, chap 4.2.2.6: 01960 * Eff.snd.MSS = min(SendMSS+20, MMS_S) - TCPhdrsize - IPoptionsize 01961 * We correct for TCP options in tcp_write(), and don't support IP options. 01962 */ 01963 sendmss = LWIP_MIN(sendmss, mss_s); 01964 } 01965 return sendmss; 01966 } 01967 #endif /* TCP_CALCULATE_EFF_SEND_MSS */ 01968 01969 /** Helper function for tcp_netif_ip_addr_changed() that iterates a pcb list */ 01970 static void 01971 tcp_netif_ip_addr_changed_pcblist(const ip_addr_t* old_addr, struct tcp_pcb* pcb_list) 01972 { 01973 struct tcp_pcb *pcb; 01974 pcb = pcb_list; 01975 while (pcb != NULL) { 01976 /* PCB bound to current local interface address? */ 01977 if (ip_addr_cmp(&pcb->local_ip, old_addr) 01978 #if LWIP_AUTOIP 01979 /* connections to link-local addresses must persist (RFC3927 ch. 1.9) */ 01980 && (!IP_IS_V4_VAL(pcb->local_ip) || !ip4_addr_islinklocal(ip_2_ip4(&pcb->local_ip))) 01981 #endif /* LWIP_AUTOIP */ 01982 ) { 01983 /* this connection must be aborted */ 01984 struct tcp_pcb *next = pcb->next; 01985 LWIP_DEBUGF(NETIF_DEBUG | LWIP_DBG_STATE, ("netif_set_ipaddr: aborting TCP pcb %p\n", (void *)pcb)); 01986 tcp_abort(pcb); 01987 pcb = next; 01988 } else { 01989 pcb = pcb->next; 01990 } 01991 } 01992 } 01993 01994 /** This function is called from netif.c when address is changed or netif is removed 01995 * 01996 * @param old_addr IP address of the netif before change 01997 * @param new_addr IP address of the netif after change or NULL if netif has been removed 01998 */ 01999 void 02000 tcp_netif_ip_addr_changed(const ip_addr_t* old_addr, const ip_addr_t* new_addr) 02001 { 02002 struct tcp_pcb_listen *lpcb, *next; 02003 02004 if (!ip_addr_isany(old_addr)) { 02005 tcp_netif_ip_addr_changed_pcblist(old_addr, tcp_active_pcbs); 02006 tcp_netif_ip_addr_changed_pcblist(old_addr, tcp_bound_pcbs); 02007 02008 if (!ip_addr_isany(new_addr)) { 02009 /* PCB bound to current local interface address? */ 02010 for (lpcb = tcp_listen_pcbs.listen_pcbs; lpcb != NULL; lpcb = next) { 02011 next = lpcb->next; 02012 /* PCB bound to current local interface address? */ 02013 if (ip_addr_cmp(&lpcb->local_ip, old_addr)) { 02014 /* The PCB is listening to the old ipaddr and 02015 * is set to listen to the new one instead */ 02016 ip_addr_copy(lpcb->local_ip, *new_addr); 02017 } 02018 } 02019 } 02020 } 02021 } 02022 02023 const char* 02024 tcp_debug_state_str(enum tcp_state s) 02025 { 02026 return tcp_state_str[s]; 02027 } 02028 02029 #if TCP_DEBUG || TCP_INPUT_DEBUG || TCP_OUTPUT_DEBUG 02030 /** 02031 * Print a tcp header for debugging purposes. 02032 * 02033 * @param tcphdr pointer to a struct tcp_hdr 02034 */ 02035 void 02036 tcp_debug_print(struct tcp_hdr *tcphdr) 02037 { 02038 LWIP_DEBUGF(TCP_DEBUG, ("TCP header:\n")); 02039 LWIP_DEBUGF(TCP_DEBUG, ("+-------------------------------+\n")); 02040 LWIP_DEBUGF(TCP_DEBUG, ("| %5"U16_F" | %5"U16_F" | (src port, dest port)\n", 02041 lwip_ntohs(tcphdr->src), lwip_ntohs(tcphdr->dest))); 02042 LWIP_DEBUGF(TCP_DEBUG, ("+-------------------------------+\n")); 02043 LWIP_DEBUGF(TCP_DEBUG, ("| %010"U32_F" | (seq no)\n", 02044 lwip_ntohl(tcphdr->seqno))); 02045 LWIP_DEBUGF(TCP_DEBUG, ("+-------------------------------+\n")); 02046 LWIP_DEBUGF(TCP_DEBUG, ("| %010"U32_F" | (ack no)\n", 02047 lwip_ntohl(tcphdr->ackno))); 02048 LWIP_DEBUGF(TCP_DEBUG, ("+-------------------------------+\n")); 02049 LWIP_DEBUGF(TCP_DEBUG, ("| %2"U16_F" | |%"U16_F"%"U16_F"%"U16_F"%"U16_F"%"U16_F"%"U16_F"| %5"U16_F" | (hdrlen, flags (", 02050 TCPH_HDRLEN(tcphdr), 02051 (u16_t)(TCPH_FLAGS(tcphdr) >> 5 & 1), 02052 (u16_t)(TCPH_FLAGS(tcphdr) >> 4 & 1), 02053 (u16_t)(TCPH_FLAGS(tcphdr) >> 3 & 1), 02054 (u16_t)(TCPH_FLAGS(tcphdr) >> 2 & 1), 02055 (u16_t)(TCPH_FLAGS(tcphdr) >> 1 & 1), 02056 (u16_t)(TCPH_FLAGS(tcphdr) & 1), 02057 lwip_ntohs(tcphdr->wnd))); 02058 tcp_debug_print_flags(TCPH_FLAGS(tcphdr)); 02059 LWIP_DEBUGF(TCP_DEBUG, ("), win)\n")); 02060 LWIP_DEBUGF(TCP_DEBUG, ("+-------------------------------+\n")); 02061 LWIP_DEBUGF(TCP_DEBUG, ("| 0x%04"X16_F" | %5"U16_F" | (chksum, urgp)\n", 02062 lwip_ntohs(tcphdr->chksum), lwip_ntohs(tcphdr->urgp))); 02063 LWIP_DEBUGF(TCP_DEBUG, ("+-------------------------------+\n")); 02064 } 02065 02066 /** 02067 * Print a tcp state for debugging purposes. 02068 * 02069 * @param s enum tcp_state to print 02070 */ 02071 void 02072 tcp_debug_print_state(enum tcp_state s) 02073 { 02074 LWIP_DEBUGF(TCP_DEBUG, ("State: %s\n", tcp_state_str[s])); 02075 } 02076 02077 /** 02078 * Print tcp flags for debugging purposes. 02079 * 02080 * @param flags tcp flags, all active flags are printed 02081 */ 02082 void 02083 tcp_debug_print_flags(u8_t flags) 02084 { 02085 if (flags & TCP_FIN) { 02086 LWIP_DEBUGF(TCP_DEBUG, ("FIN ")); 02087 } 02088 if (flags & TCP_SYN) { 02089 LWIP_DEBUGF(TCP_DEBUG, ("SYN ")); 02090 } 02091 if (flags & TCP_RST) { 02092 LWIP_DEBUGF(TCP_DEBUG, ("RST ")); 02093 } 02094 if (flags & TCP_PSH) { 02095 LWIP_DEBUGF(TCP_DEBUG, ("PSH ")); 02096 } 02097 if (flags & TCP_ACK) { 02098 LWIP_DEBUGF(TCP_DEBUG, ("ACK ")); 02099 } 02100 if (flags & TCP_URG) { 02101 LWIP_DEBUGF(TCP_DEBUG, ("URG ")); 02102 } 02103 if (flags & TCP_ECE) { 02104 LWIP_DEBUGF(TCP_DEBUG, ("ECE ")); 02105 } 02106 if (flags & TCP_CWR) { 02107 LWIP_DEBUGF(TCP_DEBUG, ("CWR ")); 02108 } 02109 LWIP_DEBUGF(TCP_DEBUG, ("\n")); 02110 } 02111 02112 /** 02113 * Print all tcp_pcbs in every list for debugging purposes. 02114 */ 02115 void 02116 tcp_debug_print_pcbs(void) 02117 { 02118 struct tcp_pcb *pcb; 02119 struct tcp_pcb_listen *pcbl; 02120 02121 LWIP_DEBUGF(TCP_DEBUG, ("Active PCB states:\n")); 02122 for (pcb = tcp_active_pcbs; pcb != NULL; pcb = pcb->next) { 02123 LWIP_DEBUGF(TCP_DEBUG, ("Local port %"U16_F", foreign port %"U16_F" snd_nxt %"U32_F" rcv_nxt %"U32_F" ", 02124 pcb->local_port, pcb->remote_port, 02125 pcb->snd_nxt, pcb->rcv_nxt)); 02126 tcp_debug_print_state(pcb->state); 02127 } 02128 02129 LWIP_DEBUGF(TCP_DEBUG, ("Listen PCB states:\n")); 02130 for (pcbl = tcp_listen_pcbs.listen_pcbs; pcbl != NULL; pcbl = pcbl->next) { 02131 LWIP_DEBUGF(TCP_DEBUG, ("Local port %"U16_F" ", pcbl->local_port)); 02132 tcp_debug_print_state(pcbl->state); 02133 } 02134 02135 LWIP_DEBUGF(TCP_DEBUG, ("TIME-WAIT PCB states:\n")); 02136 for (pcb = tcp_tw_pcbs; pcb != NULL; pcb = pcb->next) { 02137 LWIP_DEBUGF(TCP_DEBUG, ("Local port %"U16_F", foreign port %"U16_F" snd_nxt %"U32_F" rcv_nxt %"U32_F" ", 02138 pcb->local_port, pcb->remote_port, 02139 pcb->snd_nxt, pcb->rcv_nxt)); 02140 tcp_debug_print_state(pcb->state); 02141 } 02142 } 02143 02144 /** 02145 * Check state consistency of the tcp_pcb lists. 02146 */ 02147 s16_t 02148 tcp_pcbs_sane(void) 02149 { 02150 struct tcp_pcb *pcb; 02151 for (pcb = tcp_active_pcbs; pcb != NULL; pcb = pcb->next) { 02152 LWIP_ASSERT("tcp_pcbs_sane: active pcb->state != CLOSED", pcb->state != CLOSED); 02153 LWIP_ASSERT("tcp_pcbs_sane: active pcb->state != LISTEN", pcb->state != LISTEN); 02154 LWIP_ASSERT("tcp_pcbs_sane: active pcb->state != TIME-WAIT", pcb->state != TIME_WAIT); 02155 } 02156 for (pcb = tcp_tw_pcbs; pcb != NULL; pcb = pcb->next) { 02157 LWIP_ASSERT("tcp_pcbs_sane: tw pcb->state == TIME-WAIT", pcb->state == TIME_WAIT); 02158 } 02159 return 1; 02160 } 02161 #endif /* TCP_DEBUG */ 02162 02163 #endif /* LWIP_TCP */
Generated on Sun Jul 17 2022 08:25:25 by
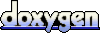