
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
net_mle_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** 00016 * \file net_mle_api.h 00017 * \brief 6LoWPAN MLE options control API 00018 * 00019 */ 00020 #ifndef _NET_MLE_API_H 00021 #define _NET_MLE_API_H 00022 00023 #include "ns_types.h" 00024 00025 #ifdef __cplusplus 00026 extern "C" { 00027 #endif 00028 00029 /** 00030 * \brief Set default MLE neighbor lifetime for a router. 00031 * 00032 * \param interface_id Interface ID. 00033 * \param lifetime Lifetime in seconds. 64 to 2560. 00034 * 00035 * \return 0, Lifetime update OK. 00036 * \return < 0, Lifetime update fail. 00037 * 00038 */ 00039 int8_t arm_nwk_6lowpan_mle_router_lifetime_set(int8_t interface_id, uint16_t lifetime); 00040 00041 /** 00042 * \brief Set default MLE neighbor lifetime for a host. 00043 * 00044 * \param interface_id Interface ID. 00045 * \param lifetime Lifetime in seconds. 64 to 2560. 00046 * 00047 * \return 0, Lifetime update OK. 00048 * \return < 0, Lifetime update fail. 00049 * 00050 */ 00051 int8_t arm_nwk_6lowpan_mle_host_lifetime_set(int8_t interface_id, uint16_t lifetime); 00052 00053 /** 00054 * \brief Set MLE neighbor list limits. 00055 * 00056 * The MLE neighbor limits configuration settings limit the number of neighbors 00057 * added to the MLE neighbor list. 00058 * 00059 * If the number of neighbors reaches the lower threshold, MLE starts to limit addition 00060 * of new neighbors. Multicast MLE messages from unknown neighbors are ignored (ignore probability is randomized). 00061 * The value must be smaller than the upper threshold and maximum value. 00062 * 00063 * If the number of neighbors reaches the upper threshold, MLE stops adding new neighbors 00064 * based on the multicast MLE messages. Only nodes that select this node for a 00065 * parent during the bootstrap will be accepted. The value must be smaller than or the same as 00066 * the maximum value. 00067 * 00068 * If the number of neighbors reaches the maximum value, no new neighbors are added. 00069 * 00070 * If the MLE neighbor list limits are not used, all values must be set to 0. 00071 * 00072 * \param interface_id Interface ID. 00073 * \param lower_threshold Lower threshold. 5 to 499. 0 limits not used. 00074 * \param upper_threshold Upper threshold. 5 to 500. 0 limits not used. 00075 * \param max Maximum number of neighbors. 5 to 500. 0 limits not used. 00076 * 00077 * \return 0, Limits update OK. 00078 * \return < 0, Limits update fail. 00079 * 00080 */ 00081 int8_t arm_nwk_6lowpan_mle_neighbor_limits_set(int8_t interface_id, uint16_t lower_threshold, uint16_t upper_threshold, uint16_t max); 00082 00083 /** 00084 * \brief Set MLE message token bucket settings 00085 * 00086 * The MLE message token bucket limits the MLE message sending rate. The token bucket size 00087 * controls the bucket size. The token bucket rate controls the rate in which 00088 * new tokens are added. The count defines how many tokens at a time are added to the bucket. 00089 * 00090 * The rate is entered in multiplies of 0.1 second minimum interval (for example, if rate is 3 00091 * and count is 4 then 4 new tokens are added to bucket every 0.3 seconds). 00092 * 00093 * If the token bucket is not used, all values must be set to 0. 00094 * 00095 * \param interface_id Interface ID. 00096 * \param size Bucket size. 1 to 255. 0 token bucket not used. 00097 * \param rate Token rate. 1 to 255. 0 token bucket not used. 00098 * \param count Token count. 1 to 255. 0 token bucket not used. 00099 * 00100 * \return 0, Token bucket settings update OK. 00101 * \return < 0, Token bucket settings update fail. 00102 * 00103 */ 00104 int8_t arm_nwk_6lowpan_mle_token_bucket_settings_set(int8_t interface_id, uint8_t size, uint8_t rate, uint8_t count); 00105 00106 #ifdef __cplusplus 00107 } 00108 #endif 00109 #endif /* _NET_MLE_API_H */
Generated on Sun Jul 17 2022 08:25:28 by
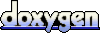