
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
mqtt.h
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * MQTT client 00004 */ 00005 00006 /* 00007 * Copyright (c) 2016 Erik Andersson 00008 * All rights reserved. 00009 * 00010 * Redistribution and use in source and binary forms, with or without modification, 00011 * are permitted provided that the following conditions are met: 00012 * 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. The name of the author may not be used to endorse or promote products 00019 * derived from this software without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00022 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00023 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00024 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00025 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00026 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00027 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00028 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00029 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00030 * OF SUCH DAMAGE. 00031 * 00032 * This file is part of the lwIP TCP/IP stack. 00033 * 00034 * Author: Erik Andersson 00035 * 00036 */ 00037 #ifndef LWIP_HDR_APPS_MQTT_CLIENT_H 00038 #define LWIP_HDR_APPS_MQTT_CLIENT_H 00039 00040 #include "lwip/apps/mqtt_opts.h" 00041 #include "lwip/err.h" 00042 #include "lwip/ip_addr.h" 00043 00044 #ifdef __cplusplus 00045 extern "C" { 00046 #endif 00047 00048 typedef struct mqtt_client_t mqtt_client_t; 00049 00050 /** @ingroup mqtt 00051 * Default MQTT port */ 00052 #define MQTT_PORT 1883 00053 00054 /*---------------------------------------------------------------------------------------------- */ 00055 /* Connection with server */ 00056 00057 /** 00058 * @ingroup mqtt 00059 * Client information and connection parameters */ 00060 struct mqtt_connect_client_info_t { 00061 /** Client identifier, must be set by caller */ 00062 const char *client_id; 00063 /** User name and password, set to NULL if not used */ 00064 const char* client_user; 00065 const char* client_pass; 00066 /** keep alive time in seconds, 0 to disable keep alive functionality*/ 00067 u16_t keep_alive; 00068 /** will topic, set to NULL if will is not to be used, 00069 will_msg, will_qos and will retain are then ignored */ 00070 const char* will_topic; 00071 const char* will_msg; 00072 u8_t will_qos; 00073 u8_t will_retain; 00074 }; 00075 00076 /** 00077 * @ingroup mqtt 00078 * Connection status codes */ 00079 typedef enum 00080 { 00081 MQTT_CONNECT_ACCEPTED = 0, 00082 MQTT_CONNECT_REFUSED_PROTOCOL_VERSION = 1, 00083 MQTT_CONNECT_REFUSED_IDENTIFIER = 2, 00084 MQTT_CONNECT_REFUSED_SERVER = 3, 00085 MQTT_CONNECT_REFUSED_USERNAME_PASS = 4, 00086 MQTT_CONNECT_REFUSED_NOT_AUTHORIZED_ = 5, 00087 MQTT_CONNECT_DISCONNECTED = 256, 00088 MQTT_CONNECT_TIMEOUT = 257 00089 } mqtt_connection_status_t; 00090 00091 /** 00092 * @ingroup mqtt 00093 * Function prototype for mqtt connection status callback. Called when 00094 * client has connected to the server after initiating a mqtt connection attempt by 00095 * calling mqtt_connect() or when connection is closed by server or an error 00096 * 00097 * @param client MQTT client itself 00098 * @param arg Additional argument to pass to the callback function 00099 * @param status Connect result code or disconnection notification @see mqtt_connection_status_t 00100 * 00101 */ 00102 typedef void (*mqtt_connection_cb_t)(mqtt_client_t *client, void *arg, mqtt_connection_status_t status); 00103 00104 00105 /** 00106 * @ingroup mqtt 00107 * Data callback flags */ 00108 enum { 00109 /** Flag set when last fragment of data arrives in data callback */ 00110 MQTT_DATA_FLAG_LAST = 1 00111 }; 00112 00113 /** 00114 * @ingroup mqtt 00115 * Function prototype for MQTT incoming publish data callback function. Called when data 00116 * arrives to a subscribed topic @see mqtt_subscribe 00117 * 00118 * @param arg Additional argument to pass to the callback function 00119 * @param data User data, pointed object, data may not be referenced after callback return, 00120 NULL is passed when all publish data are delivered 00121 * @param len Length of publish data fragment 00122 * @param flags MQTT_DATA_FLAG_LAST set when this call contains the last part of data from publish message 00123 * 00124 */ 00125 typedef void (*mqtt_incoming_data_cb_t)(void *arg, const u8_t *data, u16_t len, u8_t flags); 00126 00127 00128 /** 00129 * @ingroup mqtt 00130 * Function prototype for MQTT incoming publish function. Called when an incoming publish 00131 * arrives to a subscribed topic @see mqtt_subscribe 00132 * 00133 * @param arg Additional argument to pass to the callback function 00134 * @param topic Zero terminated Topic text string, topic may not be referenced after callback return 00135 * @param tot_len Total length of publish data, if set to 0 (no publish payload) data callback will not be invoked 00136 */ 00137 typedef void (*mqtt_incoming_publish_cb_t)(void *arg, const char *topic, u32_t tot_len); 00138 00139 00140 /** 00141 * @ingroup mqtt 00142 * Function prototype for mqtt request callback. Called when a subscribe, unsubscribe 00143 * or publish request has completed 00144 * @param arg Pointer to user data supplied when invoking request 00145 * @param err ERR_OK on success 00146 * ERR_TIMEOUT if no response was received within timeout, 00147 * ERR_ABRT if (un)subscribe was denied 00148 */ 00149 typedef void (*mqtt_request_cb_t)(void *arg, err_t err); 00150 00151 00152 /** 00153 * Pending request item, binds application callback to pending server requests 00154 */ 00155 struct mqtt_request_t 00156 { 00157 /** Next item in list, NULL means this is the last in chain, 00158 next pointing at itself means request is unallocated */ 00159 struct mqtt_request_t *next; 00160 /** Callback to upper layer */ 00161 mqtt_request_cb_t cb; 00162 void *arg; 00163 /** MQTT packet identifier */ 00164 u16_t pkt_id; 00165 /** Expire time relative to element before this */ 00166 u16_t timeout_diff; 00167 }; 00168 00169 /** Ring buffer */ 00170 struct mqtt_ringbuf_t { 00171 u16_t put; 00172 u16_t get; 00173 u8_t buf[MQTT_OUTPUT_RINGBUF_SIZE]; 00174 }; 00175 00176 /** MQTT client */ 00177 struct mqtt_client_t 00178 { 00179 /** Timers and timeouts */ 00180 u16_t cyclic_tick; 00181 u16_t keep_alive; 00182 u16_t server_watchdog; 00183 /** Packet identifier generator*/ 00184 u16_t pkt_id_seq; 00185 /** Packet identifier of pending incoming publish */ 00186 u16_t inpub_pkt_id; 00187 /** Connection state */ 00188 u8_t conn_state; 00189 struct tcp_pcb *conn; 00190 /** Connection callback */ 00191 void *connect_arg; 00192 mqtt_connection_cb_t connect_cb; 00193 /** Pending requests to server */ 00194 struct mqtt_request_t *pend_req_queue; 00195 struct mqtt_request_t req_list[MQTT_REQ_MAX_IN_FLIGHT]; 00196 void *inpub_arg; 00197 /** Incoming data callback */ 00198 mqtt_incoming_data_cb_t data_cb; 00199 mqtt_incoming_publish_cb_t pub_cb; 00200 /** Input */ 00201 u32_t msg_idx; 00202 u8_t rx_buffer[MQTT_VAR_HEADER_BUFFER_LEN]; 00203 /** Output ring-buffer */ 00204 struct mqtt_ringbuf_t output; 00205 }; 00206 00207 00208 /** Connect to server */ 00209 err_t mqtt_client_connect(mqtt_client_t *client, const ip_addr_t *ipaddr, u16_t port, mqtt_connection_cb_t cb, void *arg, 00210 const struct mqtt_connect_client_info_t *client_info); 00211 00212 /** Disconnect from server */ 00213 void mqtt_disconnect(mqtt_client_t *client); 00214 00215 /** Create new client */ 00216 mqtt_client_t *mqtt_client_new(void); 00217 00218 /** Check connection status */ 00219 u8_t mqtt_client_is_connected(mqtt_client_t *client); 00220 00221 /** Set callback to call for incoming publish */ 00222 void mqtt_set_inpub_callback(mqtt_client_t *client, mqtt_incoming_publish_cb_t, 00223 mqtt_incoming_data_cb_t data_cb, void *arg); 00224 00225 /** Common function for subscribe and unsubscribe */ 00226 err_t mqtt_sub_unsub(mqtt_client_t *client, const char *topic, u8_t qos, mqtt_request_cb_t cb, void *arg, u8_t sub); 00227 00228 /** @ingroup mqtt 00229 *Subscribe to topic */ 00230 #define mqtt_subscribe(client, topic, qos, cb, arg) mqtt_sub_unsub(client, topic, qos, cb, arg, 1) 00231 /** @ingroup mqtt 00232 * Unsubscribe to topic */ 00233 #define mqtt_unsubscribe(client, topic, cb, arg) mqtt_sub_unsub(client, topic, 0, cb, arg, 0) 00234 00235 00236 /** Publish data to topic */ 00237 err_t mqtt_publish(mqtt_client_t *client, const char *topic, const void *payload, u16_t payload_length, u8_t qos, u8_t retain, 00238 mqtt_request_cb_t cb, void *arg); 00239 00240 #ifdef __cplusplus 00241 } 00242 #endif 00243 00244 #endif /* LWIP_HDR_APPS_MQTT_CLIENT_H */
Generated on Sun Jul 17 2022 08:25:28 by
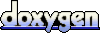