
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "USBHostMSD.h" 00003 DigitalOut led(LED1); 00004 #include "FATFileSystem.h" 00005 #include <stdlib.h> 00006 00007 00008 void msd_task(void const *) 00009 { 00010 00011 USBHostMSD msd; 00012 int i = 0; 00013 FATFileSystem fs("usb"); 00014 int err; 00015 printf("wait for usb memory stick insertion\n"); 00016 while(1) { 00017 00018 // try to connect a MSD device 00019 while(!msd.connect()) { 00020 Thread::wait(500); 00021 } 00022 if (fs.mount(&msd) != 0) { 00023 continue; 00024 } else { 00025 printf("file system mounted\n"); 00026 } 00027 00028 if (!msd.connect()) { 00029 continue; 00030 } 00031 00032 // in a loop, append a file 00033 // if the device is disconnected, we try to connect it again 00034 00035 // append a file 00036 File file; 00037 err = file.open(&fs, "test1.txt", O_WRONLY | O_CREAT |O_APPEND); 00038 00039 if (err == 0) { 00040 char tmp[100]; 00041 sprintf(tmp,"Hello fun USB stick World: %d!\r\n", i++); 00042 file.write(tmp,strlen(tmp)); 00043 sprintf(tmp,"Goodbye World!\r\n"); 00044 file.write(tmp,strlen(tmp)); 00045 file.close(); 00046 } else { 00047 printf("FILE == NULL\r\n"); 00048 } 00049 Thread::wait(500); 00050 printf("again\n"); 00051 // if device disconnected, try to connect again 00052 while (msd.connected()) { 00053 Thread::wait(500); 00054 } 00055 while (fs.unmount() < 0) { 00056 Thread::wait(500); 00057 printf("unmount\n"); 00058 } 00059 } 00060 } 00061 00062 int main() 00063 { 00064 Thread msdTask(msd_task, NULL, osPriorityNormal, 1024 * 4); 00065 00066 while(1) { 00067 led=!led; 00068 Thread::wait(500); 00069 } 00070 }
Generated on Sun Jul 17 2022 08:25:26 by
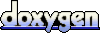