
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "mbed.h" 00017 #include "greentea-client/test_env.h" 00018 #include "unity.h" 00019 #include "utest.h" 00020 00021 #include "HeapBlockDevice.h" 00022 #include "FATFileSystem.h" 00023 #include <stdlib.h> 00024 #include "mbed_retarget.h" 00025 00026 using namespace utest::v1; 00027 00028 #ifndef MBED_EXTENDED_TESTS 00029 #error [NOT_SUPPORTED] Filesystem tests not supported by default 00030 #endif 00031 00032 // Test block device 00033 #define BLOCK_SIZE 512 00034 HeapBlockDevice bd(128*BLOCK_SIZE, BLOCK_SIZE); 00035 00036 00037 // Test formatting 00038 void test_format() { 00039 int err = FATFileSystem::format(&bd); 00040 TEST_ASSERT_EQUAL(0, err); 00041 } 00042 00043 00044 // Simple test for reading/writing files 00045 template <ssize_t TEST_SIZE> 00046 void test_read_write() { 00047 FATFileSystem fs("fat"); 00048 00049 int err = fs.mount(&bd); 00050 TEST_ASSERT_EQUAL(0, err); 00051 00052 uint8_t *buffer = (uint8_t *)malloc(TEST_SIZE); 00053 TEST_ASSERT(buffer); 00054 00055 // Fill with random sequence 00056 srand(1); 00057 for (int i = 0; i < TEST_SIZE; i++) { 00058 buffer[i] = 0xff & rand(); 00059 } 00060 00061 // write and read file 00062 File file; 00063 err = file.open(&fs, "test_read_write.dat", O_WRONLY | O_CREAT); 00064 TEST_ASSERT_EQUAL(0, err); 00065 ssize_t size = file.write(buffer, TEST_SIZE); 00066 TEST_ASSERT_EQUAL(TEST_SIZE, size); 00067 err = file.close(); 00068 TEST_ASSERT_EQUAL(0, err); 00069 00070 err = file.open(&fs, "test_read_write.dat", O_RDONLY); 00071 TEST_ASSERT_EQUAL(0, err); 00072 size = file.read(buffer, TEST_SIZE); 00073 TEST_ASSERT_EQUAL(TEST_SIZE, size); 00074 err = file.close(); 00075 TEST_ASSERT_EQUAL(0, err); 00076 00077 // Check that the data was unmodified 00078 srand(1); 00079 for (int i = 0; i < TEST_SIZE; i++) { 00080 TEST_ASSERT_EQUAL(0xff & rand(), buffer[i]); 00081 } 00082 00083 err = fs.unmount(); 00084 TEST_ASSERT_EQUAL(0, err); 00085 } 00086 00087 00088 // Simple test for iterating dir entries 00089 void test_read_dir() { 00090 FATFileSystem fs("fat"); 00091 00092 int err = fs.mount(&bd); 00093 TEST_ASSERT_EQUAL(0, err); 00094 00095 err = fs.mkdir("test_read_dir", S_IRWXU | S_IRWXG | S_IRWXO); 00096 TEST_ASSERT_EQUAL(0, err); 00097 00098 err = fs.mkdir("test_read_dir/test_dir", S_IRWXU | S_IRWXG | S_IRWXO); 00099 TEST_ASSERT_EQUAL(0, err); 00100 00101 File file; 00102 err = file.open(&fs, "test_read_dir/test_file", O_WRONLY | O_CREAT); 00103 TEST_ASSERT_EQUAL(0, err); 00104 err = file.close(); 00105 TEST_ASSERT_EQUAL(0, err); 00106 00107 // Iterate over dir checking for known files 00108 Dir dir; 00109 err = dir.open(&fs, "test_read_dir"); 00110 TEST_ASSERT_EQUAL(0, err); 00111 00112 struct dirent *de; 00113 bool test_dir_found = false; 00114 bool test_file_found = true; 00115 00116 while ((de = readdir(&dir))) { 00117 printf("d_name: %.32s, d_type: %x\n", de->d_name, de->d_type); 00118 00119 if (strcmp(de->d_name, ".") == 0) { 00120 test_dir_found = true; 00121 TEST_ASSERT_EQUAL(DT_DIR, de->d_type); 00122 } else if (strcmp(de->d_name, "..") == 0) { 00123 test_dir_found = true; 00124 TEST_ASSERT_EQUAL(DT_DIR, de->d_type); 00125 } else if (strcmp(de->d_name, "test_dir") == 0) { 00126 test_dir_found = true; 00127 TEST_ASSERT_EQUAL(DT_DIR, de->d_type); 00128 } else if (strcmp(de->d_name, "test_file") == 0) { 00129 test_file_found = true; 00130 TEST_ASSERT_EQUAL(DT_REG, de->d_type); 00131 } else { 00132 char *buf = new char[NAME_MAX]; 00133 snprintf(buf, NAME_MAX, "Unexpected file \"%s\"", de->d_name); 00134 TEST_ASSERT_MESSAGE(false, buf); 00135 } 00136 } 00137 00138 TEST_ASSERT_MESSAGE(test_dir_found, "Could not find \"test_dir\""); 00139 TEST_ASSERT_MESSAGE(test_file_found, "Could not find \"test_file\""); 00140 00141 err = dir.close(); 00142 TEST_ASSERT_EQUAL(0, err); 00143 00144 err = fs.unmount(); 00145 TEST_ASSERT_EQUAL(0, err); 00146 } 00147 00148 00149 // Test setup 00150 utest::v1::status_t test_setup(const size_t number_of_cases) { 00151 GREENTEA_SETUP(10, "default_auto"); 00152 return verbose_test_setup_handler(number_of_cases); 00153 } 00154 00155 Case cases[] = { 00156 Case("Testing formating", test_format), 00157 Case("Testing read write < block", test_read_write<BLOCK_SIZE/2>), 00158 Case("Testing read write > block", test_read_write<2*BLOCK_SIZE>), 00159 Case("Testing dir iteration", test_read_dir), 00160 }; 00161 00162 Specification specification(test_setup, cases); 00163 00164 int main() { 00165 return !Harness::run(specification); 00166 }
Generated on Sun Jul 17 2022 08:25:26 by
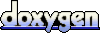