
Rtos API example
Sequential API Main thread module. More...
Go to the source code of this file.
Functions | |
static void | tcpip_thread (void *arg) |
The main lwIP thread. | |
err_t | tcpip_inpkt (struct pbuf *p, struct netif *inp, netif_input_fn input_fn) |
Pass a received packet to tcpip_thread for input processing. | |
err_t | tcpip_input (struct pbuf *p, struct netif *inp) |
Pass a received packet to tcpip_thread for input processing with ethernet_input or ip_input. | |
err_t | tcpip_callback_with_block (tcpip_callback_fn function, void *ctx, u8_t block) |
Call a specific function in the thread context of tcpip_thread for easy access synchronization. | |
err_t | tcpip_timeout (u32_t msecs, sys_timeout_handler h, void *arg) |
call sys_timeout in tcpip_thread | |
err_t | tcpip_untimeout (sys_timeout_handler h, void *arg) |
call sys_untimeout in tcpip_thread | |
err_t | tcpip_send_msg_wait_sem (tcpip_callback_fn fn, void *apimsg, sys_sem_t *sem) |
Sends a message to TCPIP thread to call a function. | |
err_t | tcpip_api_call (tcpip_api_call_fn fn, struct tcpip_api_call_data *call) |
Synchronously calls function in TCPIP thread and waits for its completion. | |
struct tcpip_callback_msg * | tcpip_callbackmsg_new (tcpip_callback_fn function, void *ctx) |
Allocate a structure for a static callback message and initialize it. | |
void | tcpip_callbackmsg_delete (struct tcpip_callback_msg *msg) |
Free a callback message allocated by tcpip_callbackmsg_new(). | |
err_t | tcpip_trycallback (struct tcpip_callback_msg *msg) |
Try to post a callback-message to the tcpip_thread mbox This is intended to be used to send "static" messages from interrupt context. | |
void | tcpip_init (tcpip_init_done_fn initfunc, void *arg) |
Initialize this module:
| |
static void | pbuf_free_int (void *p) |
Simple callback function used with tcpip_callback to free a pbuf (pbuf_free has a wrong signature for tcpip_callback) | |
err_t | pbuf_free_callback (struct pbuf *p) |
A simple wrapper function that allows you to free a pbuf from interrupt context. | |
err_t | mem_free_callback (void *m) |
A simple wrapper function that allows you to free heap memory from interrupt context. | |
Variables | |
sys_mutex_t | lock_tcpip_core |
The global semaphore to lock the stack. |
Detailed Description
Sequential API Main thread module.
Definition in file lwip_tcpip.c.
Function Documentation
err_t mem_free_callback | ( | void * | m ) |
A simple wrapper function that allows you to free heap memory from interrupt context.
- Parameters:
-
m the heap memory to free
- Returns:
- ERR_OK if callback could be enqueued, an err_t if not
Definition at line 513 of file lwip_tcpip.c.
A simple wrapper function that allows you to free a pbuf from interrupt context.
- Parameters:
-
p The pbuf (chain) to be dereferenced.
- Returns:
- ERR_OK if callback could be enqueued, an err_t if not
Definition at line 500 of file lwip_tcpip.c.
static void pbuf_free_int | ( | void * | p ) | [static] |
Simple callback function used with tcpip_callback to free a pbuf (pbuf_free has a wrong signature for tcpip_callback)
- Parameters:
-
p The pbuf (chain) to be dereferenced.
Definition at line 487 of file lwip_tcpip.c.
err_t tcpip_api_call | ( | tcpip_api_call_fn | fn, |
struct tcpip_api_call_data * | call | ||
) |
Synchronously calls function in TCPIP thread and waits for its completion.
It is recommended to use LWIP_TCPIP_CORE_LOCKING (preferred) or LWIP_NETCONN_SEM_PER_THREAD. If not, a semaphore is created and destroyed on every call which is usually an expensive/slow operation.
- Parameters:
-
fn Function to call call Call parameters
- Returns:
- Return value from tcpip_api_call_fn
Definition at line 365 of file lwip_tcpip.c.
err_t tcpip_callback_with_block | ( | tcpip_callback_fn | function, |
void * | ctx, | ||
u8_t | block | ||
) |
Call a specific function in the thread context of tcpip_thread for easy access synchronization.
A function called in that way may access lwIP core code without fearing concurrent access.
- Parameters:
-
function the function to call ctx parameter passed to f block 1 to block until the request is posted, 0 to non-blocking mode
- Returns:
- ERR_OK if the function was called, another err_t if not
Definition at line 234 of file lwip_tcpip.c.
void tcpip_callbackmsg_delete | ( | struct tcpip_callback_msg * | msg ) |
Free a callback message allocated by tcpip_callbackmsg_new().
- Parameters:
-
msg the message to free
Definition at line 433 of file lwip_tcpip.c.
struct tcpip_callback_msg* tcpip_callbackmsg_new | ( | tcpip_callback_fn | function, |
void * | ctx | ||
) | [read] |
Allocate a structure for a static callback message and initialize it.
This is intended to be used to send "static" messages from interrupt context.
- Parameters:
-
function the function to call ctx parameter passed to function
- Returns:
- a struct pointer to pass to tcpip_trycallback().
Definition at line 415 of file lwip_tcpip.c.
err_t tcpip_inpkt | ( | struct pbuf * | p, |
struct netif * | inp, | ||
netif_input_fn | input_fn | ||
) |
Pass a received packet to tcpip_thread for input processing.
- Parameters:
-
p the received packet inp the network interface on which the packet was received input_fn input function to call
Definition at line 169 of file lwip_tcpip.c.
err_t tcpip_send_msg_wait_sem | ( | tcpip_callback_fn | fn, |
void * | apimsg, | ||
sys_sem_t * | sem | ||
) |
Sends a message to TCPIP thread to call a function.
Caller thread blocks on on a provided semaphore, which ist NOT automatically signalled by TCPIP thread, this has to be done by the user. It is recommended to use LWIP_TCPIP_CORE_LOCKING since this is the way with least runtime overhead.
- Parameters:
-
fn function to be called from TCPIP thread apimsg argument to API function sem semaphore to wait on
- Returns:
- ERR_OK if the function was called, another err_t if not
Definition at line 329 of file lwip_tcpip.c.
static void tcpip_thread | ( | void * | arg ) | [static] |
The main lwIP thread.
This thread has exclusive access to lwIP core functions (unless access to them is not locked). Other threads communicate with this thread using message boxes.
It also starts all the timers to make sure they are running in the right thread context.
- Parameters:
-
arg unused argument
Definition at line 87 of file lwip_tcpip.c.
err_t tcpip_timeout | ( | u32_t | msecs, |
sys_timeout_handler | h, | ||
void * | arg | ||
) |
call sys_timeout in tcpip_thread
- Parameters:
-
msecs time in milliseconds for timeout h function to be called on timeout arg argument to pass to timeout function h
- Returns:
- ERR_MEM on memory error, ERR_OK otherwise
Definition at line 269 of file lwip_tcpip.c.
err_t tcpip_trycallback | ( | struct tcpip_callback_msg * | msg ) |
Try to post a callback-message to the tcpip_thread mbox This is intended to be used to send "static" messages from interrupt context.
- Parameters:
-
msg pointer to the message to post
- Returns:
- sys_mbox_trypost() return code
Definition at line 446 of file lwip_tcpip.c.
err_t tcpip_untimeout | ( | sys_timeout_handler | h, |
void * | arg | ||
) |
call sys_untimeout in tcpip_thread
- Parameters:
-
h function to be called on timeout arg argument to pass to timeout function h
- Returns:
- ERR_MEM on memory error, ERR_OK otherwise
Definition at line 296 of file lwip_tcpip.c.
Variable Documentation
sys_mutex_t lock_tcpip_core |
The global semaphore to lock the stack.
Definition at line 65 of file lwip_tcpip.c.
Generated on Sun Jul 17 2022 08:25:35 by
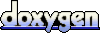