
Rtos API example
lwip_nd6.c File Reference
Neighbor discovery and stateless address autoconfiguration for IPv6. More...
Go to the source code of this file.
Functions | |
static s8_t | nd6_find_neighbor_cache_entry (const ip6_addr_t *ip6addr) |
Search for a neighbor cache entry. | |
static s8_t | nd6_new_neighbor_cache_entry (void) |
Create a new neighbor cache entry. | |
static void | nd6_free_neighbor_cache_entry (s8_t i) |
Will free any resources associated with a neighbor cache entry, and will mark it as unused. | |
static s8_t | nd6_find_destination_cache_entry (const ip6_addr_t *ip6addr) |
Search for a destination cache entry. | |
static s8_t | nd6_new_destination_cache_entry (void) |
Create a new destination cache entry. | |
static s8_t | nd6_is_prefix_in_netif (const ip6_addr_t *ip6addr, struct netif *netif) |
Determine whether an address matches an on-link prefix or the subnet of a statically assigned address. | |
static s8_t | nd6_select_router (const ip6_addr_t *ip6addr, struct netif *netif) |
Select a default router for a destination. | |
static s8_t | nd6_get_router (const ip6_addr_t *router_addr, struct netif *netif) |
Find an entry for a default router. | |
static s8_t | nd6_new_router (const ip6_addr_t *router_addr, struct netif *netif) |
Create a new entry for a default router. | |
static s8_t | nd6_get_onlink_prefix (const ip6_addr_t *prefix, struct netif *netif) |
Find the cached entry for an on-link prefix. | |
static s8_t | nd6_new_onlink_prefix (const ip6_addr_t *prefix, struct netif *netif) |
Creates a new entry for an on-link prefix. | |
static s8_t | nd6_get_next_hop_entry (const ip6_addr_t *ip6addr, struct netif *netif) |
Determine the next hop for a destination. | |
static err_t | nd6_queue_packet (s8_t neighbor_index, struct pbuf *q) |
Queue a packet for a neighbor. | |
static void | nd6_send_ns (struct netif *netif, const ip6_addr_t *target_addr, u8_t flags) |
Send a neighbor solicitation message. | |
static void | nd6_send_na (struct netif *netif, const ip6_addr_t *target_addr, u8_t flags) |
Send a neighbor advertisement message. | |
static void | nd6_send_neighbor_cache_probe (struct nd6_neighbor_cache_entry *entry, u8_t flags) |
Send a neighbor solicitation message for a specific neighbor cache entry. | |
static err_t | nd6_send_rs (struct netif *netif) |
Send a router solicitation message. | |
static void | nd6_free_q (struct nd6_q_entry *q) |
Free a complete queue of nd6 q entries. | |
static void | nd6_send_q (s8_t i) |
Send queued packets for a neighbor. | |
static void | nd6_duplicate_addr_detected (struct netif *netif, s8_t addr_idx) |
A local address has been determined to be a duplicate. | |
static void | nd6_process_autoconfig_prefix (struct netif *netif, struct prefix_option *prefix_opt, const ip6_addr_t *prefix_addr) |
We received a router advertisement that contains a prefix with the autoconfiguration flag set. | |
void | nd6_input (struct pbuf *p, struct netif *inp) |
Process an incoming neighbor discovery message. | |
void | nd6_tmr (void) |
Periodic timer for Neighbor discovery functions: | |
void | nd6_clear_destination_cache (void) |
Clear the destination cache. | |
struct netif * | nd6_find_route (const ip6_addr_t *ip6addr) |
Find a router-announced route to the given destination. | |
err_t | nd6_get_next_hop_addr_or_queue (struct netif *netif, struct pbuf *q, const ip6_addr_t *ip6addr, const u8_t **hwaddrp) |
A packet is to be transmitted to a specific IPv6 destination on a specific interface. | |
u16_t | nd6_get_destination_mtu (const ip6_addr_t *ip6addr, struct netif *netif) |
Get the Path MTU for a destination. | |
void | nd6_reachability_hint (const ip6_addr_t *ip6addr) |
Provide the Neighbor discovery process with a hint that a destination is reachable. | |
void | nd6_cleanup_netif (struct netif *netif) |
Remove all prefix, neighbor_cache and router entries of the specified netif. | |
void | nd6_adjust_mld_membership (struct netif *netif, s8_t addr_idx, u8_t new_state) |
The state of a local IPv6 address entry is about to change. |
Detailed Description
Neighbor discovery and stateless address autoconfiguration for IPv6.
Aims to be compliant with RFC 4861 (Neighbor discovery) and RFC 4862 (Address autoconfiguration).
Definition in file lwip_nd6.c.
Function Documentation
void nd6_adjust_mld_membership | ( | struct netif * | netif, |
s8_t | addr_idx, | ||
u8_t | new_state | ||
) |
The state of a local IPv6 address entry is about to change.
If needed, join or leave the solicited-node multicast group for the address.
- Parameters:
-
netif The netif that owns the address. addr_idx The index of the address. new_state The new (IP6_ADDR_) state for the address.
Definition at line 2314 of file lwip_nd6.c.
void nd6_cleanup_netif | ( | struct netif * | netif ) |
Remove all prefix, neighbor_cache and router entries of the specified netif.
- Parameters:
-
netif points to a network interface
Definition at line 2277 of file lwip_nd6.c.
void nd6_clear_destination_cache | ( | void | ) |
Clear the destination cache.
This operation may be necessary for consistency in the light of changing local addresses and/or use of the gateway hook.
Definition at line 1531 of file lwip_nd6.c.
static void nd6_duplicate_addr_detected | ( | struct netif * | netif, |
s8_t | addr_idx | ||
) | [static] |
A local address has been determined to be a duplicate.
Take the appropriate action(s) on the address and the interface as a whole.
- Parameters:
-
netif the netif that owns the address addr_idx the index of the address detected to be a duplicate
Definition at line 136 of file lwip_nd6.c.
static s8_t nd6_find_destination_cache_entry | ( | const ip6_addr_t * | ip6addr ) | [static] |
Search for a destination cache entry.
- Parameters:
-
ip6addr the IPv6 address of the destination
- Returns:
- The destination cache entry index that matched, -1 if no entry is found
Definition at line 1481 of file lwip_nd6.c.
static s8_t nd6_find_neighbor_cache_entry | ( | const ip6_addr_t * | ip6addr ) | [static] |
Search for a neighbor cache entry.
- Parameters:
-
ip6addr the IPv6 address of the neighbor
- Returns:
- The neighbor cache entry index that matched, -1 if no entry is found
Definition at line 1322 of file lwip_nd6.c.
struct netif* nd6_find_route | ( | const ip6_addr_t * | ip6addr ) | [read] |
Find a router-announced route to the given destination.
This route may be based on an on-link prefix or a default router.
If a suitable route is found, the returned netif is guaranteed to be in a suitable state (up, link up) to be used for packet transmission.
- Parameters:
-
ip6addr the destination IPv6 address
- Returns:
- the netif to use for the destination, or NULL if none found
Definition at line 1660 of file lwip_nd6.c.
static void nd6_free_neighbor_cache_entry | ( | s8_t | i ) | [static] |
Will free any resources associated with a neighbor cache entry, and will mark it as unused.
- Parameters:
-
i the neighbor cache entry index to free
Definition at line 1450 of file lwip_nd6.c.
static void nd6_free_q | ( | struct nd6_q_entry * | q ) | [static] |
Free a complete queue of nd6 q entries.
- Parameters:
-
q a queue of nd6_q_entry to free
Definition at line 2075 of file lwip_nd6.c.
u16_t nd6_get_destination_mtu | ( | const ip6_addr_t * | ip6addr, |
struct netif * | netif | ||
) |
Get the Path MTU for a destination.
- Parameters:
-
ip6addr the destination address netif the netif on which the packet will be sent
- Returns:
- the Path MTU, if known, or the netif default MTU
Definition at line 2204 of file lwip_nd6.c.
err_t nd6_get_next_hop_addr_or_queue | ( | struct netif * | netif, |
struct pbuf * | q, | ||
const ip6_addr_t * | ip6addr, | ||
const u8_t ** | hwaddrp | ||
) |
A packet is to be transmitted to a specific IPv6 destination on a specific interface.
Check if we can find the hardware address of the next hop to use for the packet. If so, give the hardware address to the caller, which should use it to send the packet right away. Otherwise, enqueue the packet for later transmission while looking up the hardware address, if possible.
As such, this function returns one of three different possible results:
- ERR_OK with a non-NULL 'hwaddrp': the caller should send the packet now.
- ERR_OK with a NULL 'hwaddrp': the packet has been enqueued for later.
- not ERR_OK: something went wrong; forward the error upward in the stack.
- Parameters:
-
netif The lwIP network interface on which the IP packet will be sent. q The pbuf(s) containing the IP packet to be sent. ip6addr The destination IPv6 address of the packet. hwaddrp On success, filled with a pointer to a HW address or NULL (meaning the packet has been queued).
- Returns:
- ERR_OK on success, ERR_RTE if no route was found for the packet, or ERR_MEM if low memory conditions prohibit sending the packet at all.
Definition at line 2163 of file lwip_nd6.c.
static s8_t nd6_get_next_hop_entry | ( | const ip6_addr_t * | ip6addr, |
struct netif * | netif | ||
) | [static] |
Determine the next hop for a destination.
Will determine if the destination is on-link, else a suitable on-link router is selected.
The last entry index is cached for fast entry search.
- Parameters:
-
ip6addr the destination address netif the netif on which the packet will be sent
- Returns:
- the neighbor cache entry for the next hop, ERR_RTE if no suitable next hop was found, ERR_MEM if no cache entry could be created
Definition at line 1842 of file lwip_nd6.c.
static s8_t nd6_get_onlink_prefix | ( | const ip6_addr_t * | prefix, |
struct netif * | netif | ||
) | [static] |
Find the cached entry for an on-link prefix.
- Parameters:
-
prefix the IPv6 prefix that is on-link netif the netif on which the prefix is on-link
- Returns:
- the index on the prefix table, or -1 if not found
Definition at line 1786 of file lwip_nd6.c.
static s8_t nd6_get_router | ( | const ip6_addr_t * | router_addr, |
struct netif * | netif | ||
) | [static] |
Find an entry for a default router.
- Parameters:
-
router_addr the IPv6 address of the router netif the netif on which the router is found, if known
- Returns:
- the index of the router entry, or -1 if not found
Definition at line 1696 of file lwip_nd6.c.
Process an incoming neighbor discovery message.
- Parameters:
-
p the nd packet, p->payload pointing to the icmpv6 header inp the netif on which this packet was received
Definition at line 275 of file lwip_nd6.c.
static s8_t nd6_is_prefix_in_netif | ( | const ip6_addr_t * | ip6addr, |
struct netif * | netif | ||
) | [static] |
Determine whether an address matches an on-link prefix or the subnet of a statically assigned address.
- Parameters:
-
ip6addr the IPv6 address to match
- Returns:
- 1 if the address is on-link, 0 otherwise
Definition at line 1548 of file lwip_nd6.c.
static s8_t nd6_new_destination_cache_entry | ( | void | ) | [static] |
Create a new destination cache entry.
If no unused entry is found, will recycle oldest entry.
- Returns:
- The destination cache entry index that was created, -1 if no entry was created
Definition at line 1500 of file lwip_nd6.c.
static s8_t nd6_new_neighbor_cache_entry | ( | void | ) | [static] |
Create a new neighbor cache entry.
If no unused entry is found, will try to recycle an old entry according to ad-hoc "age" heuristic.
- Returns:
- The neighbor cache entry index that was created, -1 if no entry could be created
Definition at line 1343 of file lwip_nd6.c.
static s8_t nd6_new_onlink_prefix | ( | const ip6_addr_t * | prefix, |
struct netif * | netif | ||
) | [static] |
Creates a new entry for an on-link prefix.
- Parameters:
-
prefix the IPv6 prefix that is on-link netif the netif on which the prefix is on-link
- Returns:
- the index on the prefix table, or -1 if not created
Definition at line 1810 of file lwip_nd6.c.
static s8_t nd6_new_router | ( | const ip6_addr_t * | router_addr, |
struct netif * | netif | ||
) | [static] |
Create a new entry for a default router.
- Parameters:
-
router_addr the IPv6 address of the router netif the netif on which the router is connected, if known
- Returns:
- the index on the router table, or -1 if could not be created
Definition at line 1721 of file lwip_nd6.c.
static void nd6_process_autoconfig_prefix | ( | struct netif * | netif, |
struct prefix_option * | prefix_opt, | ||
const ip6_addr_t * | prefix_addr | ||
) | [static] |
We received a router advertisement that contains a prefix with the autoconfiguration flag set.
Add or update an associated autogenerated address.
- Parameters:
-
netif the netif on which the router advertisement arrived prefix_opt a pointer to the prefix option data prefix_addr an aligned copy of the prefix address
Definition at line 175 of file lwip_nd6.c.
Queue a packet for a neighbor.
- Parameters:
-
neighbor_index the index in the neighbor cache table q packet to be queued
- Returns:
- ERR_OK if succeeded, ERR_MEM if out of memory
Definition at line 1964 of file lwip_nd6.c.
void nd6_reachability_hint | ( | const ip6_addr_t * | ip6addr ) |
Provide the Neighbor discovery process with a hint that a destination is reachable.
Called by tcp_receive when ACKs are received or sent (as per RFC). This is useful to avoid sending NS messages every 30 seconds.
- Parameters:
-
ip6addr the destination address which is know to be reachable by an upper layer protocol (TCP)
Definition at line 2234 of file lwip_nd6.c.
static s8_t nd6_select_router | ( | const ip6_addr_t * | ip6addr, |
struct netif * | netif | ||
) | [static] |
Select a default router for a destination.
This function is used both for routing and for finding a next-hop target for a packet. In the former case, the given netif is NULL, and the returned router entry must be for a netif suitable for sending packets (up, link up). In the latter case, the given netif is not NULL and restricts router choice.
- Parameters:
-
ip6addr the destination address netif the netif for the outgoing packet, if known
- Returns:
- the default router entry index, or -1 if no suitable router is found
Definition at line 1588 of file lwip_nd6.c.
static void nd6_send_na | ( | struct netif * | netif, |
const ip6_addr_t * | target_addr, | ||
u8_t | flags | ||
) | [static] |
Send a neighbor advertisement message.
- Parameters:
-
netif the netif on which to send the message target_addr the IPv6 target address for the ND message flags one of ND6_SEND_FLAG_*
Definition at line 1180 of file lwip_nd6.c.
static void nd6_send_neighbor_cache_probe | ( | struct nd6_neighbor_cache_entry * | entry, |
u8_t | flags | ||
) | [static] |
Send a neighbor solicitation message for a specific neighbor cache entry.
- Parameters:
-
entry the neightbor cache entry for wich to send the message flags one of ND6_SEND_FLAG_*
Definition at line 1097 of file lwip_nd6.c.
static void nd6_send_ns | ( | struct netif * | netif, |
const ip6_addr_t * | target_addr, | ||
u8_t | flags | ||
) | [static] |
Send a neighbor solicitation message.
- Parameters:
-
netif the netif on which to send the message target_addr the IPv6 target address for the ND message flags one of ND6_SEND_FLAG_*
Definition at line 1110 of file lwip_nd6.c.
static void nd6_send_q | ( | s8_t | i ) | [static] |
Send queued packets for a neighbor.
- Parameters:
-
i the neighbor to send packets to
Definition at line 2096 of file lwip_nd6.c.
Send a router solicitation message.
- Parameters:
-
netif the netif on which to send the message
Definition at line 1251 of file lwip_nd6.c.
void nd6_tmr | ( | void | ) |
Periodic timer for Neighbor discovery functions:
- Update neighbor reachability states
- Update destination cache entries age
- Update invalidation timers of default routers and on-link prefixes
- Update lifetimes of our addresses
- Perform duplicate address detection (DAD) for our addresses
- Send router solicitations
Definition at line 893 of file lwip_nd6.c.
Generated on Sun Jul 17 2022 08:25:35 by
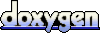