
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
lwip_snmpv3.c
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * Additional SNMPv3 functionality RFC3414 and RFC3826. 00004 */ 00005 00006 /* 00007 * Copyright (c) 2016 Elias Oenal. 00008 * All rights reserved. 00009 * 00010 * Redistribution and use in source and binary forms, with or without modification, 00011 * are permitted provided that the following conditions are met: 00012 * 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. The name of the author may not be used to endorse or promote products 00019 * derived from this software without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00022 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00023 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00024 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00025 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00026 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00027 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00028 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00029 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00030 * OF SUCH DAMAGE. 00031 * 00032 * Author: Elias Oenal <lwip@eliasoenal.com> 00033 */ 00034 00035 #include "snmpv3_priv.h" 00036 #include "lwip/apps/snmpv3.h" 00037 #include "lwip/sys.h" 00038 #include <string.h> 00039 00040 #if LWIP_SNMP && LWIP_SNMP_V3 00041 00042 #ifdef LWIP_SNMPV3_INCLUDE_ENGINE 00043 #include LWIP_SNMPV3_INCLUDE_ENGINE 00044 #endif 00045 00046 #define SNMP_MAX_TIME_BOOT 2147483647UL 00047 00048 /** Call this if engine has been changed. Has to reset boots, see below */ 00049 void 00050 snmpv3_engine_id_changed(void) 00051 { 00052 snmpv3_set_engine_boots(0); 00053 } 00054 00055 /** According to RFC3414 2.2.2. 00056 * 00057 * The number of times that the SNMP engine has 00058 * (re-)initialized itself since snmpEngineID 00059 * was last configured. 00060 */ 00061 u32_t 00062 snmpv3_get_engine_boots_internal(void) 00063 { 00064 if (snmpv3_get_engine_boots() == 0 || 00065 snmpv3_get_engine_boots() < SNMP_MAX_TIME_BOOT) { 00066 return snmpv3_get_engine_boots(); 00067 } 00068 00069 snmpv3_set_engine_boots(SNMP_MAX_TIME_BOOT); 00070 return snmpv3_get_engine_boots(); 00071 } 00072 00073 /** RFC3414 2.2.2. 00074 * 00075 * Once the timer reaches 2147483647 it gets reset to zero and the 00076 * engine boot ups get incremented. 00077 */ 00078 u32_t 00079 snmpv3_get_engine_time_internal(void) 00080 { 00081 if (snmpv3_get_engine_time() >= SNMP_MAX_TIME_BOOT) { 00082 snmpv3_reset_engine_time(); 00083 00084 if (snmpv3_get_engine_boots() < SNMP_MAX_TIME_BOOT - 1) { 00085 snmpv3_set_engine_boots(snmpv3_get_engine_boots() + 1); 00086 } else { 00087 snmpv3_set_engine_boots(SNMP_MAX_TIME_BOOT); 00088 } 00089 } 00090 00091 return snmpv3_get_engine_time(); 00092 } 00093 00094 #if LWIP_SNMP_V3_CRYPTO 00095 00096 /* This function ignores the byte order suggestion in RFC3414 00097 * since it simply doesn't influence the effectiveness of an IV. 00098 * 00099 * Implementing RFC3826 priv param algorithm if LWIP_RAND is available. 00100 * 00101 * @todo: This is a potential thread safety issue. 00102 */ 00103 err_t 00104 snmpv3_build_priv_param(u8_t* priv_param) 00105 { 00106 #ifdef LWIP_RAND /* Based on RFC3826 */ 00107 static u8_t init; 00108 static u32_t priv1, priv2; 00109 00110 /* Lazy initialisation */ 00111 if (init == 0) { 00112 init = 1; 00113 priv1 = LWIP_RAND(); 00114 priv2 = LWIP_RAND(); 00115 } 00116 00117 SMEMCPY(&priv_param[0], &priv1, sizeof(priv1)); 00118 SMEMCPY(&priv_param[4], &priv2, sizeof(priv2)); 00119 00120 /* Emulate 64bit increment */ 00121 priv1++; 00122 if (!priv1) { /* Overflow */ 00123 priv2++; 00124 } 00125 #else /* Based on RFC3414 */ 00126 static u32_t ctr; 00127 u32_t boots = LWIP_SNMPV3_GET_ENGINE_BOOTS(); 00128 SMEMCPY(&priv_param[0], &boots, 4); 00129 SMEMCPY(&priv_param[4], &ctr, 4); 00130 ctr++; 00131 #endif 00132 return ERR_OK; 00133 } 00134 #endif /* LWIP_SNMP_V3_CRYPTO */ 00135 00136 #endif
Generated on Sun Jul 17 2022 08:25:25 by
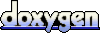