
Rtos API example
OS abstraction layer. More...
Go to the source code of this file.
Typedefs | |
typedef void(* | lwip_thread_fn )(void *arg) |
Function prototype for thread functions. | |
Functions | |
err_t | sys_mutex_new (sys_mutex_t *mutex) |
Create a new mutex. | |
void | sys_mutex_lock (sys_mutex_t *mutex) |
Lock a mutex. | |
void | sys_mutex_unlock (sys_mutex_t *mutex) |
Unlock a mutex. | |
void | sys_mutex_free (sys_mutex_t *mutex) |
Delete a semaphore. | |
int | sys_mutex_valid (sys_mutex_t *mutex) |
Check if a mutex is valid/allocated: return 1 for valid, 0 for invalid. | |
void | sys_mutex_set_invalid (sys_mutex_t *mutex) |
Set a mutex invalid so that sys_mutex_valid returns 0. | |
err_t | sys_sem_new (sys_sem_t *sem, u8_t count) |
Create a new semaphore. | |
void | sys_sem_signal (sys_sem_t *sem) |
Signals a semaphore. | |
u32_t | sys_arch_sem_wait (sys_sem_t *sem, u32_t timeout) |
Wait for a semaphore for the specified timeout. | |
void | sys_sem_free (sys_sem_t *sem) |
Delete a semaphore. | |
int | sys_sem_valid (sys_sem_t *sem) |
Check if a semaphore is valid/allocated: return 1 for valid, 0 for invalid. | |
void | sys_sem_set_invalid (sys_sem_t *sem) |
Set a semaphore invalid so that sys_sem_valid returns 0. | |
void | sys_msleep (u32_t ms) |
Sleep for specified number of ms. | |
err_t | sys_mbox_new (sys_mbox_t *mbox, int size) |
Create a new mbox of specified size. | |
void | sys_mbox_post (sys_mbox_t *mbox, void *msg) |
Post a message to an mbox - may not fail -> blocks if full, only used from tasks not from ISR. | |
err_t | sys_mbox_trypost (sys_mbox_t *mbox, void *msg) |
Try to post a message to an mbox - may fail if full or ISR. | |
u32_t | sys_arch_mbox_fetch (sys_mbox_t *mbox, void **msg, u32_t timeout) |
Wait for a new message to arrive in the mbox. | |
u32_t | sys_arch_mbox_tryfetch (sys_mbox_t *mbox, void **msg) |
Wait for a new message to arrive in the mbox. | |
void | sys_mbox_free (sys_mbox_t *mbox) |
Delete an mbox. | |
int | sys_mbox_valid (sys_mbox_t *mbox) |
Check if an mbox is valid/allocated: return 1 for valid, 0 for invalid. | |
void | sys_mbox_set_invalid (sys_mbox_t *mbox) |
Set an mbox invalid so that sys_mbox_valid returns 0. | |
sys_thread_t | sys_thread_new (const char *name, lwip_thread_fn thread, void *arg, int stacksize, int prio) |
The only thread function: Creates a new thread ATTENTION: although this function returns a value, it MUST NOT FAIL (ports have to assert this!) | |
u32_t | sys_jiffies (void) |
Ticks/jiffies since power up. | |
u32_t | sys_now (void) |
Returns the current time in milliseconds, may be the same as sys_jiffies or at least based on it. |
Detailed Description
OS abstraction layer.
Definition in file sys.h.
Typedef Documentation
typedef void(* lwip_thread_fn)(void *arg) |
Function Documentation
u32_t sys_jiffies | ( | void | ) |
Ticks/jiffies since power up.
Definition at line 432 of file lwip_sys_arch.c.
Generated on Sun Jul 17 2022 08:25:36 by
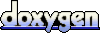