
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
lwip_snmp_netconn.c
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * SNMP netconn frontend. 00004 */ 00005 00006 /* 00007 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00008 * All rights reserved. 00009 * 00010 * Redistribution and use in source and binary forms, with or without modification, 00011 * are permitted provided that the following conditions are met: 00012 * 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. The name of the author may not be used to endorse or promote products 00019 * derived from this software without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00022 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00023 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00024 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00025 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00026 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00027 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00028 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00029 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00030 * OF SUCH DAMAGE. 00031 * 00032 * Author: Dirk Ziegelmeier <dziegel@gmx.de> 00033 */ 00034 00035 #include "lwip/apps/snmp_opts.h" 00036 00037 #if LWIP_SNMP && SNMP_USE_NETCONN 00038 00039 #include <string.h> 00040 #include "lwip/api.h" 00041 #include "lwip/ip.h" 00042 #include "lwip/udp.h" 00043 #include "snmp_msg.h" 00044 #include "lwip/sys.h" 00045 00046 /** SNMP netconn API worker thread */ 00047 static void 00048 snmp_netconn_thread(void *arg) 00049 { 00050 struct netconn *conn; 00051 struct netbuf *buf; 00052 err_t err; 00053 LWIP_UNUSED_ARG(arg); 00054 00055 /* Bind to SNMP port with default IP address */ 00056 #if LWIP_IPV6 00057 conn = netconn_new(NETCONN_UDP_IPV6); 00058 netconn_bind(conn, IP6_ADDR_ANY, SNMP_IN_PORT); 00059 #else /* LWIP_IPV6 */ 00060 conn = netconn_new(NETCONN_UDP); 00061 netconn_bind(conn, IP4_ADDR_ANY, SNMP_IN_PORT); 00062 #endif /* LWIP_IPV6 */ 00063 LWIP_ERROR("snmp_netconn: invalid conn", (conn != NULL), return;); 00064 00065 snmp_traps_handle = conn; 00066 00067 do { 00068 err = netconn_recv(conn, &buf); 00069 00070 if (err == ERR_OK) { 00071 snmp_receive(conn, buf->p, &buf->addr, buf->port); 00072 } 00073 00074 if (buf != NULL) { 00075 netbuf_delete(buf); 00076 } 00077 } while(1); 00078 } 00079 00080 err_t 00081 snmp_sendto(void *handle, struct pbuf *p, const ip_addr_t *dst, u16_t port) 00082 { 00083 err_t result; 00084 struct netbuf buf; 00085 00086 memset(&buf, 0, sizeof(buf)); 00087 buf.p = p; 00088 result = netconn_sendto((struct netconn*)handle, &buf, dst, port); 00089 00090 return result; 00091 } 00092 00093 u8_t 00094 snmp_get_local_ip_for_dst(void* handle, const ip_addr_t *dst, ip_addr_t *result) 00095 { 00096 struct netconn* conn = (struct netconn*)handle; 00097 struct netif *dst_if; 00098 const ip_addr_t* dst_ip; 00099 00100 LWIP_UNUSED_ARG(conn); /* unused in case of IPV4 only configuration */ 00101 00102 ip_route_get_local_ip(&conn->pcb.udp->local_ip, dst, dst_if, dst_ip); 00103 00104 if ((dst_if != NULL) && (dst_ip != NULL)) { 00105 ip_addr_copy(*result, *dst_ip); 00106 return 1; 00107 } else { 00108 return 0; 00109 } 00110 } 00111 00112 /** 00113 * Starts SNMP Agent. 00114 */ 00115 void 00116 snmp_init(void) 00117 { 00118 sys_thread_new("snmp_netconn", snmp_netconn_thread, NULL, SNMP_STACK_SIZE, SNMP_THREAD_PRIO); 00119 } 00120 00121 #endif /* LWIP_SNMP && SNMP_USE_NETCONN */
Generated on Sun Jul 17 2022 08:25:25 by
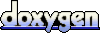