
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
lwip_pppapi.c
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * Point To Point Protocol Sequential API module 00004 * 00005 */ 00006 00007 /* 00008 * Redistribution and use in source and binary forms, with or without modification, 00009 * are permitted provided that the following conditions are met: 00010 * 00011 * 1. Redistributions of source code must retain the above copyright notice, 00012 * this list of conditions and the following disclaimer. 00013 * 2. Redistributions in binary form must reproduce the above copyright notice, 00014 * this list of conditions and the following disclaimer in the documentation 00015 * and/or other materials provided with the distribution. 00016 * 3. The name of the author may not be used to endorse or promote products 00017 * derived from this software without specific prior written permission. 00018 * 00019 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00020 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00021 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00022 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00023 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00024 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00025 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00026 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00027 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00028 * OF SUCH DAMAGE. 00029 * 00030 * This file is part of the lwIP TCP/IP stack. 00031 * 00032 */ 00033 00034 #include "netif/ppp/ppp_opts.h" 00035 00036 #if LWIP_PPP_API /* don't build if not configured for use in lwipopts.h */ 00037 00038 #include "netif/ppp/pppapi.h" 00039 #include "lwip/priv/tcpip_priv.h" 00040 #include "netif/ppp/pppoe.h" 00041 #include "netif/ppp/pppol2tp.h" 00042 #include "netif/ppp/pppos.h" 00043 00044 #if LWIP_MPU_COMPATIBLE 00045 LWIP_MEMPOOL_DECLARE(PPPAPI_MSG, MEMP_NUM_PPP_API_MSG, sizeof(struct pppapi_msg), "PPPAPI_MSG") 00046 #endif 00047 00048 #define PPPAPI_VAR_REF(name) API_VAR_REF(name) 00049 #define PPPAPI_VAR_DECLARE(name) API_VAR_DECLARE(struct pppapi_msg, name) 00050 #define PPPAPI_VAR_ALLOC(name) API_VAR_ALLOC_POOL(struct pppapi_msg, PPPAPI_MSG, name, ERR_MEM) 00051 #define PPPAPI_VAR_ALLOC_RETURN_NULL(name) API_VAR_ALLOC_POOL(struct pppapi_msg, PPPAPI_MSG, name, NULL) 00052 #define PPPAPI_VAR_FREE(name) API_VAR_FREE_POOL(PPPAPI_MSG, name) 00053 00054 /** 00055 * Call ppp_set_default() inside the tcpip_thread context. 00056 */ 00057 static err_t 00058 pppapi_do_ppp_set_default(struct tcpip_api_call_data *m) 00059 { 00060 /* cast through void* to silence alignment warnings. 00061 * We know it works because the structs have been instantiated as struct pppapi_msg */ 00062 struct pppapi_msg *msg = (struct pppapi_msg *)(void*)m; 00063 00064 ppp_set_default(msg->msg.ppp); 00065 return ERR_OK; 00066 } 00067 00068 /** 00069 * Call ppp_set_default() in a thread-safe way by running that function inside the 00070 * tcpip_thread context. 00071 */ 00072 err_t 00073 pppapi_set_default(ppp_pcb *pcb) 00074 { 00075 err_t err; 00076 PPPAPI_VAR_DECLARE(msg); 00077 PPPAPI_VAR_ALLOC(msg); 00078 00079 PPPAPI_VAR_REF(msg).msg.ppp = pcb; 00080 err = tcpip_api_call(pppapi_do_ppp_set_default, &PPPAPI_VAR_REF(msg).call); 00081 PPPAPI_VAR_FREE(msg); 00082 return err; 00083 } 00084 00085 00086 #if PPP_NOTIFY_PHASE 00087 /** 00088 * Call ppp_set_notify_phase_callback() inside the tcpip_thread context. 00089 */ 00090 static err_t 00091 pppapi_do_ppp_set_notify_phase_callback(struct tcpip_api_call_data *m) 00092 { 00093 /* cast through void* to silence alignment warnings. 00094 * We know it works because the structs have been instantiated as struct pppapi_msg */ 00095 struct pppapi_msg *msg = (struct pppapi_msg *)(void*)m; 00096 00097 ppp_set_notify_phase_callback(msg->msg.ppp, msg->msg.msg.setnotifyphasecb.notify_phase_cb); 00098 return ERR_OK; 00099 } 00100 00101 /** 00102 * Call ppp_set_notify_phase_callback() in a thread-safe way by running that function inside the 00103 * tcpip_thread context. 00104 */ 00105 err_t 00106 pppapi_set_notify_phase_callback(ppp_pcb *pcb, ppp_notify_phase_cb_fn notify_phase_cb) 00107 { 00108 err_t err; 00109 PPPAPI_VAR_DECLARE(msg); 00110 PPPAPI_VAR_ALLOC(msg); 00111 00112 PPPAPI_VAR_REF(msg).msg.ppp = pcb; 00113 PPPAPI_VAR_REF(msg).msg.msg.setnotifyphasecb.notify_phase_cb = notify_phase_cb; 00114 err = tcpip_api_call(pppapi_do_ppp_set_notify_phase_callback, &PPPAPI_VAR_REF(msg).call); 00115 PPPAPI_VAR_FREE(msg); 00116 return err; 00117 } 00118 #endif /* PPP_NOTIFY_PHASE */ 00119 00120 00121 #if PPPOS_SUPPORT 00122 /** 00123 * Call pppos_create() inside the tcpip_thread context. 00124 */ 00125 static err_t 00126 pppapi_do_pppos_create(struct tcpip_api_call_data *m) 00127 { 00128 /* cast through void* to silence alignment warnings. 00129 * We know it works because the structs have been instantiated as struct pppapi_msg */ 00130 struct pppapi_msg *msg = (struct pppapi_msg *)(void*)m; 00131 00132 msg->msg.ppp = pppos_create(msg->msg.msg.serialcreate.pppif, msg->msg.msg.serialcreate.output_cb, 00133 msg->msg.msg.serialcreate.link_status_cb, msg->msg.msg.serialcreate.ctx_cb); 00134 return ERR_OK; 00135 } 00136 00137 /** 00138 * Call pppos_create() in a thread-safe way by running that function inside the 00139 * tcpip_thread context. 00140 */ 00141 ppp_pcb* 00142 pppapi_pppos_create(struct netif *pppif, pppos_output_cb_fn output_cb, 00143 ppp_link_status_cb_fn link_status_cb, void *ctx_cb) 00144 { 00145 ppp_pcb* result; 00146 PPPAPI_VAR_DECLARE(msg); 00147 PPPAPI_VAR_ALLOC_RETURN_NULL(msg); 00148 00149 PPPAPI_VAR_REF(msg).msg.ppp = NULL; 00150 PPPAPI_VAR_REF(msg).msg.msg.serialcreate.pppif = pppif; 00151 PPPAPI_VAR_REF(msg).msg.msg.serialcreate.output_cb = output_cb; 00152 PPPAPI_VAR_REF(msg).msg.msg.serialcreate.link_status_cb = link_status_cb; 00153 PPPAPI_VAR_REF(msg).msg.msg.serialcreate.ctx_cb = ctx_cb; 00154 tcpip_api_call(pppapi_do_pppos_create, &PPPAPI_VAR_REF(msg).call); 00155 result = PPPAPI_VAR_REF(msg).msg.ppp; 00156 PPPAPI_VAR_FREE(msg); 00157 return result; 00158 } 00159 #endif /* PPPOS_SUPPORT */ 00160 00161 00162 #if PPPOE_SUPPORT 00163 /** 00164 * Call pppoe_create() inside the tcpip_thread context. 00165 */ 00166 static err_t 00167 pppapi_do_pppoe_create(struct tcpip_api_call_data *m) 00168 { 00169 /* cast through void* to silence alignment warnings. 00170 * We know it works because the structs have been instantiated as struct pppapi_msg */ 00171 struct pppapi_msg *msg = (struct pppapi_msg *)(void*)m; 00172 00173 msg->msg.ppp = pppoe_create(msg->msg.msg.ethernetcreate.pppif, msg->msg.msg.ethernetcreate.ethif, 00174 msg->msg.msg.ethernetcreate.service_name, msg->msg.msg.ethernetcreate.concentrator_name, 00175 msg->msg.msg.ethernetcreate.link_status_cb, msg->msg.msg.ethernetcreate.ctx_cb); 00176 return ERR_OK; 00177 } 00178 00179 /** 00180 * Call pppoe_create() in a thread-safe way by running that function inside the 00181 * tcpip_thread context. 00182 */ 00183 ppp_pcb* 00184 pppapi_pppoe_create(struct netif *pppif, struct netif *ethif, const char *service_name, 00185 const char *concentrator_name, ppp_link_status_cb_fn link_status_cb, 00186 void *ctx_cb) 00187 { 00188 ppp_pcb* result; 00189 PPPAPI_VAR_DECLARE(msg); 00190 PPPAPI_VAR_ALLOC_RETURN_NULL(msg); 00191 00192 PPPAPI_VAR_REF(msg).msg.ppp = NULL; 00193 PPPAPI_VAR_REF(msg).msg.msg.ethernetcreate.pppif = pppif; 00194 PPPAPI_VAR_REF(msg).msg.msg.ethernetcreate.ethif = ethif; 00195 PPPAPI_VAR_REF(msg).msg.msg.ethernetcreate.service_name = service_name; 00196 PPPAPI_VAR_REF(msg).msg.msg.ethernetcreate.concentrator_name = concentrator_name; 00197 PPPAPI_VAR_REF(msg).msg.msg.ethernetcreate.link_status_cb = link_status_cb; 00198 PPPAPI_VAR_REF(msg).msg.msg.ethernetcreate.ctx_cb = ctx_cb; 00199 tcpip_api_call(pppapi_do_pppoe_create, &PPPAPI_VAR_REF(msg).call); 00200 result = PPPAPI_VAR_REF(msg).msg.ppp; 00201 PPPAPI_VAR_FREE(msg); 00202 return result; 00203 } 00204 #endif /* PPPOE_SUPPORT */ 00205 00206 00207 #if PPPOL2TP_SUPPORT 00208 /** 00209 * Call pppol2tp_create() inside the tcpip_thread context. 00210 */ 00211 static err_t 00212 pppapi_do_pppol2tp_create(struct tcpip_api_call_data *m) 00213 { 00214 /* cast through void* to silence alignment warnings. 00215 * We know it works because the structs have been instantiated as struct pppapi_msg */ 00216 struct pppapi_msg *msg = (struct pppapi_msg *)(void*)m; 00217 00218 msg->msg.ppp = pppol2tp_create(msg->msg.msg.l2tpcreate.pppif, 00219 msg->msg.msg.l2tpcreate.netif, API_EXPR_REF(msg->msg.msg.l2tpcreate.ipaddr), msg->msg.msg.l2tpcreate.port, 00220 #if PPPOL2TP_AUTH_SUPPORT 00221 msg->msg.msg.l2tpcreate.secret, 00222 msg->msg.msg.l2tpcreate.secret_len, 00223 #else /* PPPOL2TP_AUTH_SUPPORT */ 00224 NULL, 00225 0, 00226 #endif /* PPPOL2TP_AUTH_SUPPORT */ 00227 msg->msg.msg.l2tpcreate.link_status_cb, msg->msg.msg.l2tpcreate.ctx_cb); 00228 return ERR_OK; 00229 } 00230 00231 /** 00232 * Call pppol2tp_create() in a thread-safe way by running that function inside the 00233 * tcpip_thread context. 00234 */ 00235 ppp_pcb* 00236 pppapi_pppol2tp_create(struct netif *pppif, struct netif *netif, ip_addr_t *ipaddr, u16_t port, 00237 const u8_t *secret, u8_t secret_len, 00238 ppp_link_status_cb_fn link_status_cb, void *ctx_cb) 00239 { 00240 ppp_pcb* result; 00241 PPPAPI_VAR_DECLARE(msg); 00242 PPPAPI_VAR_ALLOC_RETURN_NULL(msg); 00243 #if !PPPOL2TP_AUTH_SUPPORT 00244 LWIP_UNUSED_ARG(secret); 00245 LWIP_UNUSED_ARG(secret_len); 00246 #endif /* !PPPOL2TP_AUTH_SUPPORT */ 00247 00248 PPPAPI_VAR_REF(msg).msg.ppp = NULL; 00249 PPPAPI_VAR_REF(msg).msg.msg.l2tpcreate.pppif = pppif; 00250 PPPAPI_VAR_REF(msg).msg.msg.l2tpcreate.netif = netif; 00251 PPPAPI_VAR_REF(msg).msg.msg.l2tpcreate.ipaddr = PPPAPI_VAR_REF(ipaddr); 00252 PPPAPI_VAR_REF(msg).msg.msg.l2tpcreate.port = port; 00253 #if PPPOL2TP_AUTH_SUPPORT 00254 PPPAPI_VAR_REF(msg).msg.msg.l2tpcreate.secret = secret; 00255 PPPAPI_VAR_REF(msg).msg.msg.l2tpcreate.secret_len = secret_len; 00256 #endif /* PPPOL2TP_AUTH_SUPPORT */ 00257 PPPAPI_VAR_REF(msg).msg.msg.l2tpcreate.link_status_cb = link_status_cb; 00258 PPPAPI_VAR_REF(msg).msg.msg.l2tpcreate.ctx_cb = ctx_cb; 00259 tcpip_api_call(pppapi_do_pppol2tp_create, &PPPAPI_VAR_REF(msg).call); 00260 result = PPPAPI_VAR_REF(msg).msg.ppp; 00261 PPPAPI_VAR_FREE(msg); 00262 return result; 00263 } 00264 #endif /* PPPOL2TP_SUPPORT */ 00265 00266 00267 /** 00268 * Call ppp_connect() inside the tcpip_thread context. 00269 */ 00270 static err_t 00271 pppapi_do_ppp_connect(struct tcpip_api_call_data *m) 00272 { 00273 /* cast through void* to silence alignment warnings. 00274 * We know it works because the structs have been instantiated as struct pppapi_msg */ 00275 struct pppapi_msg *msg = (struct pppapi_msg *)(void*)m; 00276 00277 return ppp_connect(msg->msg.ppp, msg->msg.msg.connect.holdoff); 00278 } 00279 00280 /** 00281 * Call ppp_connect() in a thread-safe way by running that function inside the 00282 * tcpip_thread context. 00283 */ 00284 err_t 00285 pppapi_connect(ppp_pcb *pcb, u16_t holdoff) 00286 { 00287 err_t err; 00288 PPPAPI_VAR_DECLARE(msg); 00289 PPPAPI_VAR_ALLOC(msg); 00290 00291 PPPAPI_VAR_REF(msg).msg.ppp = pcb; 00292 PPPAPI_VAR_REF(msg).msg.msg.connect.holdoff = holdoff; 00293 err = tcpip_api_call(pppapi_do_ppp_connect, &PPPAPI_VAR_REF(msg).call); 00294 PPPAPI_VAR_FREE(msg); 00295 return err; 00296 } 00297 00298 00299 #if PPP_SERVER 00300 /** 00301 * Call ppp_listen() inside the tcpip_thread context. 00302 */ 00303 static err_t 00304 pppapi_do_ppp_listen(struct tcpip_api_call_data *m) 00305 { 00306 /* cast through void* to silence alignment warnings. 00307 * We know it works because the structs have been instantiated as struct pppapi_msg */ 00308 struct pppapi_msg *msg = (struct pppapi_msg *)(void*)m; 00309 00310 return ppp_listen(msg->msg.ppp); 00311 } 00312 00313 /** 00314 * Call ppp_listen() in a thread-safe way by running that function inside the 00315 * tcpip_thread context. 00316 */ 00317 err_t 00318 pppapi_listen(ppp_pcb *pcb) 00319 { 00320 err_t err; 00321 PPPAPI_VAR_DECLARE(msg); 00322 PPPAPI_VAR_ALLOC(msg); 00323 00324 PPPAPI_VAR_REF(msg).msg.ppp = pcb; 00325 err = tcpip_api_call(pppapi_do_ppp_listen, &PPPAPI_VAR_REF(msg).call); 00326 PPPAPI_VAR_FREE(msg); 00327 return err; 00328 } 00329 #endif /* PPP_SERVER */ 00330 00331 00332 /** 00333 * Call ppp_close() inside the tcpip_thread context. 00334 */ 00335 static err_t 00336 pppapi_do_ppp_close(struct tcpip_api_call_data *m) 00337 { 00338 /* cast through void* to silence alignment warnings. 00339 * We know it works because the structs have been instantiated as struct pppapi_msg */ 00340 struct pppapi_msg *msg = (struct pppapi_msg *)(void*)m; 00341 00342 return ppp_close(msg->msg.ppp, msg->msg.msg.close.nocarrier); 00343 } 00344 00345 /** 00346 * Call ppp_close() in a thread-safe way by running that function inside the 00347 * tcpip_thread context. 00348 */ 00349 err_t 00350 pppapi_close(ppp_pcb *pcb, u8_t nocarrier) 00351 { 00352 err_t err; 00353 PPPAPI_VAR_DECLARE(msg); 00354 PPPAPI_VAR_ALLOC(msg); 00355 00356 PPPAPI_VAR_REF(msg).msg.ppp = pcb; 00357 PPPAPI_VAR_REF(msg).msg.msg.close.nocarrier = nocarrier; 00358 err = tcpip_api_call(pppapi_do_ppp_close, &PPPAPI_VAR_REF(msg).call); 00359 PPPAPI_VAR_FREE(msg); 00360 return err; 00361 } 00362 00363 00364 /** 00365 * Call ppp_free() inside the tcpip_thread context. 00366 */ 00367 static err_t 00368 pppapi_do_ppp_free(struct tcpip_api_call_data *m) 00369 { 00370 /* cast through void* to silence alignment warnings. 00371 * We know it works because the structs have been instantiated as struct pppapi_msg */ 00372 struct pppapi_msg *msg = (struct pppapi_msg *)(void*)m; 00373 00374 return ppp_free(msg->msg.ppp); 00375 } 00376 00377 /** 00378 * Call ppp_free() in a thread-safe way by running that function inside the 00379 * tcpip_thread context. 00380 */ 00381 err_t 00382 pppapi_free(ppp_pcb *pcb) 00383 { 00384 err_t err; 00385 PPPAPI_VAR_DECLARE(msg); 00386 PPPAPI_VAR_ALLOC(msg); 00387 00388 PPPAPI_VAR_REF(msg).msg.ppp = pcb; 00389 err = tcpip_api_call(pppapi_do_ppp_free, &PPPAPI_VAR_REF(msg).call); 00390 PPPAPI_VAR_FREE(msg); 00391 return err; 00392 } 00393 00394 00395 /** 00396 * Call ppp_ioctl() inside the tcpip_thread context. 00397 */ 00398 static err_t 00399 pppapi_do_ppp_ioctl(struct tcpip_api_call_data *m) 00400 { 00401 /* cast through void* to silence alignment warnings. 00402 * We know it works because the structs have been instantiated as struct pppapi_msg */ 00403 struct pppapi_msg *msg = (struct pppapi_msg *)(void*)m; 00404 00405 return ppp_ioctl(msg->msg.ppp, msg->msg.msg.ioctl.cmd, msg->msg.msg.ioctl.arg); 00406 } 00407 00408 /** 00409 * Call ppp_ioctl() in a thread-safe way by running that function inside the 00410 * tcpip_thread context. 00411 */ 00412 err_t 00413 pppapi_ioctl(ppp_pcb *pcb, u8_t cmd, void *arg) 00414 { 00415 err_t err; 00416 PPPAPI_VAR_DECLARE(msg); 00417 PPPAPI_VAR_ALLOC(msg); 00418 00419 PPPAPI_VAR_REF(msg).msg.ppp = pcb; 00420 PPPAPI_VAR_REF(msg).msg.msg.ioctl.cmd = cmd; 00421 PPPAPI_VAR_REF(msg).msg.msg.ioctl.arg = arg; 00422 err = tcpip_api_call(pppapi_do_ppp_ioctl, &PPPAPI_VAR_REF(msg).call); 00423 PPPAPI_VAR_FREE(msg); 00424 return err; 00425 } 00426 00427 #endif /* LWIP_PPP_API */
Generated on Sun Jul 17 2022 08:25:25 by
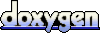