
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
lwip_netifapi.c
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * Network Interface Sequential API module 00004 * 00005 * @defgroup netifapi NETIF API 00006 * @ingroup sequential_api 00007 * Thread-safe functions to be called from non-TCPIP threads 00008 * 00009 * @defgroup netifapi_netif NETIF related 00010 * @ingroup netifapi 00011 * To be called from non-TCPIP threads 00012 */ 00013 00014 /* 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 00018 * 1. Redistributions of source code must retain the above copyright notice, 00019 * this list of conditions and the following disclaimer. 00020 * 2. Redistributions in binary form must reproduce the above copyright notice, 00021 * this list of conditions and the following disclaimer in the documentation 00022 * and/or other materials provided with the distribution. 00023 * 3. The name of the author may not be used to endorse or promote products 00024 * derived from this software without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00027 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00028 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00029 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00030 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00031 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00032 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00033 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00034 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00035 * OF SUCH DAMAGE. 00036 * 00037 * This file is part of the lwIP TCP/IP stack. 00038 * 00039 */ 00040 00041 #include "lwip/opt.h" 00042 00043 #if LWIP_NETIF_API /* don't build if not configured for use in lwipopts.h */ 00044 00045 #include "lwip/netifapi.h" 00046 #include "lwip/memp.h" 00047 #include "lwip/priv/tcpip_priv.h" 00048 00049 #define NETIFAPI_VAR_REF(name) API_VAR_REF(name) 00050 #define NETIFAPI_VAR_DECLARE(name) API_VAR_DECLARE(struct netifapi_msg, name) 00051 #define NETIFAPI_VAR_ALLOC(name) API_VAR_ALLOC(struct netifapi_msg, MEMP_NETIFAPI_MSG, name, ERR_MEM) 00052 #define NETIFAPI_VAR_FREE(name) API_VAR_FREE(MEMP_NETIFAPI_MSG, name) 00053 00054 /** 00055 * Call netif_add() inside the tcpip_thread context. 00056 */ 00057 static err_t 00058 netifapi_do_netif_add(struct tcpip_api_call_data *m) 00059 { 00060 /* cast through void* to silence alignment warnings. 00061 * We know it works because the structs have been instantiated as struct netifapi_msg */ 00062 struct netifapi_msg *msg = (struct netifapi_msg*)(void*)m; 00063 00064 if (!netif_add( msg->netif, 00065 #if LWIP_IPV4 00066 API_EXPR_REF(msg->msg.add.ipaddr), 00067 API_EXPR_REF(msg->msg.add.netmask), 00068 API_EXPR_REF(msg->msg.add.gw), 00069 #endif /* LWIP_IPV4 */ 00070 msg->msg.add.state, 00071 msg->msg.add.init, 00072 msg->msg.add.input)) { 00073 return ERR_IF; 00074 } else { 00075 return ERR_OK; 00076 } 00077 } 00078 00079 #if LWIP_IPV4 00080 /** 00081 * Call netif_set_addr() inside the tcpip_thread context. 00082 */ 00083 static err_t 00084 netifapi_do_netif_set_addr(struct tcpip_api_call_data *m) 00085 { 00086 /* cast through void* to silence alignment warnings. 00087 * We know it works because the structs have been instantiated as struct netifapi_msg */ 00088 struct netifapi_msg *msg = (struct netifapi_msg*)(void*)m; 00089 00090 netif_set_addr( msg->netif, 00091 API_EXPR_REF(msg->msg.add.ipaddr), 00092 API_EXPR_REF(msg->msg.add.netmask), 00093 API_EXPR_REF(msg->msg.add.gw)); 00094 return ERR_OK; 00095 } 00096 #endif /* LWIP_IPV4 */ 00097 00098 /** 00099 * Call the "errtfunc" (or the "voidfunc" if "errtfunc" is NULL) inside the 00100 * tcpip_thread context. 00101 */ 00102 static err_t 00103 netifapi_do_netif_common(struct tcpip_api_call_data *m) 00104 { 00105 /* cast through void* to silence alignment warnings. 00106 * We know it works because the structs have been instantiated as struct netifapi_msg */ 00107 struct netifapi_msg *msg = (struct netifapi_msg*)(void*)m; 00108 00109 if (msg->msg.common.errtfunc != NULL) { 00110 return msg->msg.common.errtfunc(msg->netif); 00111 } else { 00112 msg->msg.common.voidfunc(msg->netif); 00113 return ERR_OK; 00114 } 00115 } 00116 00117 /** 00118 * @ingroup netifapi_netif 00119 * Call netif_add() in a thread-safe way by running that function inside the 00120 * tcpip_thread context. 00121 * 00122 * @note for params @see netif_add() 00123 */ 00124 err_t 00125 netifapi_netif_add(struct netif *netif, 00126 #if LWIP_IPV4 00127 const ip4_addr_t *ipaddr, const ip4_addr_t *netmask, const ip4_addr_t *gw, 00128 #endif /* LWIP_IPV4 */ 00129 void *state, netif_init_fn init, netif_input_fn input) 00130 { 00131 err_t err; 00132 NETIFAPI_VAR_DECLARE(msg); 00133 NETIFAPI_VAR_ALLOC(msg); 00134 00135 #if LWIP_IPV4 00136 if (ipaddr == NULL) { 00137 ipaddr = IP4_ADDR_ANY4; 00138 } 00139 if (netmask == NULL) { 00140 netmask = IP4_ADDR_ANY4; 00141 } 00142 if (gw == NULL) { 00143 gw = IP4_ADDR_ANY4; 00144 } 00145 #endif /* LWIP_IPV4 */ 00146 00147 NETIFAPI_VAR_REF(msg).netif = netif; 00148 #if LWIP_IPV4 00149 NETIFAPI_VAR_REF(msg).msg.add.ipaddr = NETIFAPI_VAR_REF(ipaddr); 00150 NETIFAPI_VAR_REF(msg).msg.add.netmask = NETIFAPI_VAR_REF(netmask); 00151 NETIFAPI_VAR_REF(msg).msg.add.gw = NETIFAPI_VAR_REF(gw); 00152 #endif /* LWIP_IPV4 */ 00153 NETIFAPI_VAR_REF(msg).msg.add.state = state; 00154 NETIFAPI_VAR_REF(msg).msg.add.init = init; 00155 NETIFAPI_VAR_REF(msg).msg.add.input = input; 00156 err = tcpip_api_call(netifapi_do_netif_add, &API_VAR_REF(msg).call); 00157 NETIFAPI_VAR_FREE(msg); 00158 return err; 00159 } 00160 00161 #if LWIP_IPV4 00162 /** 00163 * @ingroup netifapi_netif 00164 * Call netif_set_addr() in a thread-safe way by running that function inside the 00165 * tcpip_thread context. 00166 * 00167 * @note for params @see netif_set_addr() 00168 */ 00169 err_t 00170 netifapi_netif_set_addr(struct netif *netif, 00171 const ip4_addr_t *ipaddr, 00172 const ip4_addr_t *netmask, 00173 const ip4_addr_t *gw) 00174 { 00175 err_t err; 00176 NETIFAPI_VAR_DECLARE(msg); 00177 NETIFAPI_VAR_ALLOC(msg); 00178 00179 if (ipaddr == NULL) { 00180 ipaddr = IP4_ADDR_ANY4; 00181 } 00182 if (netmask == NULL) { 00183 netmask = IP4_ADDR_ANY4; 00184 } 00185 if (gw == NULL) { 00186 gw = IP4_ADDR_ANY4; 00187 } 00188 00189 NETIFAPI_VAR_REF(msg).netif = netif; 00190 NETIFAPI_VAR_REF(msg).msg.add.ipaddr = NETIFAPI_VAR_REF(ipaddr); 00191 NETIFAPI_VAR_REF(msg).msg.add.netmask = NETIFAPI_VAR_REF(netmask); 00192 NETIFAPI_VAR_REF(msg).msg.add.gw = NETIFAPI_VAR_REF(gw); 00193 err = tcpip_api_call(netifapi_do_netif_set_addr, &API_VAR_REF(msg).call); 00194 NETIFAPI_VAR_FREE(msg); 00195 return err; 00196 } 00197 #endif /* LWIP_IPV4 */ 00198 00199 /** 00200 * call the "errtfunc" (or the "voidfunc" if "errtfunc" is NULL) in a thread-safe 00201 * way by running that function inside the tcpip_thread context. 00202 * 00203 * @note use only for functions where there is only "netif" parameter. 00204 */ 00205 err_t 00206 netifapi_netif_common(struct netif *netif, netifapi_void_fn voidfunc, 00207 netifapi_errt_fn errtfunc) 00208 { 00209 err_t err; 00210 NETIFAPI_VAR_DECLARE(msg); 00211 NETIFAPI_VAR_ALLOC(msg); 00212 00213 NETIFAPI_VAR_REF(msg).netif = netif; 00214 NETIFAPI_VAR_REF(msg).msg.common.voidfunc = voidfunc; 00215 NETIFAPI_VAR_REF(msg).msg.common.errtfunc = errtfunc; 00216 err = tcpip_api_call(netifapi_do_netif_common, &API_VAR_REF(msg).call); 00217 NETIFAPI_VAR_FREE(msg); 00218 return err; 00219 } 00220 00221 #endif /* LWIP_NETIF_API */
Generated on Sun Jul 17 2022 08:25:24 by
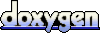