
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
lwip_ip.c
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * Common IPv4 and IPv6 code 00004 * 00005 * @defgroup ip IP 00006 * @ingroup callbackstyle_api 00007 * 00008 * @defgroup ip4 IPv4 00009 * @ingroup ip 00010 * 00011 * @defgroup ip6 IPv6 00012 * @ingroup ip 00013 * 00014 * @defgroup ipaddr IP address handling 00015 * @ingroup infrastructure 00016 * 00017 * @defgroup ip4addr IPv4 only 00018 * @ingroup ipaddr 00019 * 00020 * @defgroup ip6addr IPv6 only 00021 * @ingroup ipaddr 00022 */ 00023 00024 /* 00025 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00026 * All rights reserved. 00027 * 00028 * Redistribution and use in source and binary forms, with or without modification, 00029 * are permitted provided that the following conditions are met: 00030 * 00031 * 1. Redistributions of source code must retain the above copyright notice, 00032 * this list of conditions and the following disclaimer. 00033 * 2. Redistributions in binary form must reproduce the above copyright notice, 00034 * this list of conditions and the following disclaimer in the documentation 00035 * and/or other materials provided with the distribution. 00036 * 3. The name of the author may not be used to endorse or promote products 00037 * derived from this software without specific prior written permission. 00038 * 00039 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00040 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00041 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00042 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00043 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00044 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00045 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00046 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00047 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00048 * OF SUCH DAMAGE. 00049 * 00050 * This file is part of the lwIP TCP/IP stack. 00051 * 00052 * Author: Adam Dunkels <adam@sics.se> 00053 * 00054 */ 00055 00056 #include "lwip/opt.h" 00057 00058 #if LWIP_IPV4 || LWIP_IPV6 00059 00060 #include "lwip/ip_addr.h" 00061 #include "lwip/ip.h" 00062 00063 /** Global data for both IPv4 and IPv6 */ 00064 struct ip_globals ip_data; 00065 00066 #if LWIP_IPV4 && LWIP_IPV6 00067 00068 const ip_addr_t ip_addr_any_type = IPADDR_ANY_TYPE_INIT; 00069 00070 /** 00071 * @ingroup ipaddr 00072 * Convert IP address string (both versions) to numeric. 00073 * The version is auto-detected from the string. 00074 * 00075 * @param cp IP address string to convert 00076 * @param addr conversion result is stored here 00077 * @return 1 on success, 0 on error 00078 */ 00079 int 00080 ipaddr_aton(const char *cp, ip_addr_t *addr) 00081 { 00082 if (cp != NULL) { 00083 const char* c; 00084 for (c = cp; *c != 0; c++) { 00085 if (*c == ':') { 00086 /* contains a colon: IPv6 address */ 00087 if (addr) { 00088 IP_SET_TYPE_VAL(*addr, IPADDR_TYPE_V6); 00089 } 00090 return ip6addr_aton(cp, ip_2_ip6(addr)); 00091 } else if (*c == '.') { 00092 /* contains a dot: IPv4 address */ 00093 break; 00094 } 00095 } 00096 /* call ip4addr_aton as fallback or if IPv4 was found */ 00097 if (addr) { 00098 IP_SET_TYPE_VAL(*addr, IPADDR_TYPE_V4); 00099 } 00100 return ip4addr_aton(cp, ip_2_ip4(addr)); 00101 } 00102 return 0; 00103 } 00104 00105 /** 00106 * @ingroup lwip_nosys 00107 * If both IP versions are enabled, this function can dispatch packets to the correct one. 00108 * Don't call directly, pass to netif_add() and call netif->input(). 00109 */ 00110 err_t 00111 ip_input(struct pbuf *p, struct netif *inp) 00112 { 00113 if (p != NULL) { 00114 if (IP_HDR_GET_VERSION(p->payload) == 6) { 00115 return ip6_input(p, inp); 00116 } 00117 return ip4_input(p, inp); 00118 } 00119 return ERR_VAL; 00120 } 00121 00122 #endif /* LWIP_IPV4 && LWIP_IPV6 */ 00123 00124 #endif /* LWIP_IPV4 || LWIP_IPV6 */
Generated on Sun Jul 17 2022 08:25:24 by
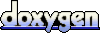