
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
lp_ticker.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include <stdio.h> 00017 #include "TestHarness.h" 00018 #include "mbed.h" 00019 #include "us_ticker_api.h" 00020 00021 /* Low power timer test. 00022 */ 00023 00024 #if !DEVICE_LOWPOWERTIMER 00025 #error This test unit requires low power to be defined for a target 00026 #endif 00027 00028 volatile bool complete; 00029 00030 void cbdone() { 00031 complete = true; 00032 } 00033 00034 TEST_GROUP(LowPowerTimerTest) 00035 { 00036 LowPowerTimeout *obj; 00037 00038 void setup() { 00039 obj = new LowPowerTimeout; 00040 complete = false; 00041 } 00042 00043 void teardown() { 00044 delete obj; 00045 obj = NULL; 00046 } 00047 00048 }; 00049 00050 // 2 second timeout using lp ticker, time is measured using us ticker 00051 TEST(LowPowerTimerTest, lp_ticker_callback_2sec_timeout) 00052 { 00053 timestamp_t start = us_ticker_read(); 00054 obj->attach(&cbdone, 2.0f); 00055 while (!complete); 00056 timestamp_t end = us_ticker_read(); 00057 00058 // Not accurate for longer delays, thus +/- 00059 if ((end - start) > 2100000) { 00060 printf("Error! Start: %u, end: %u. It took longer than 2.1 sec.", start, end); 00061 CHECK_EQUAL(0, 1); 00062 } 00063 CHECK_EQUAL(complete, 1); 00064 } 00065 00066 // 50 microsecond timeout 00067 TEST(LowPowerTimerTest, lp_ticker_callback_50us_timeout) 00068 { 00069 timestamp_t start = us_ticker_read(); 00070 obj->attach_us(&cbdone, 50); 00071 while(!complete); 00072 timestamp_t end = us_ticker_read(); 00073 // roughly should be around 50us +/- 500us, for example with 32kHz, it can be 32us the lowest 00074 CHECK_EQUAL(((end - start) > 1) && ((end - start) < 500) ,1); 00075 CHECK_EQUAL(complete, 1); 00076 } 00077 00078 // 1 milisecond timeout 00079 TEST(LowPowerTimerTest, lp_ticker_callback_1ms_timeout) 00080 { 00081 timestamp_t start = us_ticker_read(); 00082 obj->attach_us(&cbdone, 1000); 00083 while(!complete); 00084 timestamp_t end = us_ticker_read(); 00085 00086 CHECK_EQUAL(((end - start) > 800) && ((end - start) < 1600) ,1); 00087 CHECK_EQUAL(complete, 1); 00088 } 00089 00090 // 5 second wake up from deep sleep 00091 TEST(LowPowerTimerTest, lp_ticker_deepsleep_wakeup_5sec_timeout) 00092 { 00093 timestamp_t start = lp_ticker_read(); 00094 obj->attach(&cbdone, 5.0f); 00095 deepsleep(); 00096 while (!complete); 00097 timestamp_t end = lp_ticker_read(); 00098 // roughly should be around 5seconds +/- 100ms 00099 CHECK_EQUAL(((end - start) > 4900000) && ((end - start) < 5100000) ,1); 00100 CHECK_EQUAL(complete, 1); 00101 } 00102 00103 // 1ms wake up from deep sleep 00104 TEST(LowPowerTimerTest, lp_ticker_deepsleep_wakeup_1ms_timeout) 00105 { 00106 timestamp_t start = lp_ticker_read(); 00107 obj->attach(&cbdone, 0.001f); 00108 deepsleep(); 00109 while (!complete); 00110 timestamp_t end = lp_ticker_read(); 00111 // 1ms timeout +/- 600us 00112 CHECK_EQUAL(((end - start) > 400) && ((end - start) < 1600) ,1); 00113 CHECK_EQUAL(complete, 1); 00114 }
Generated on Sun Jul 17 2022 08:25:24 by
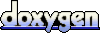