
Rtos API example
DNS
[Callback-style APIs]
Implements a DNS host name to IP address resolver. More...
Functions | |
void | dns_setserver (u8_t numdns, const ip_addr_t *dnsserver) |
Initialize one of the DNS servers. | |
const ip_addr_t * | dns_getserver (u8_t numdns) |
Obtain one of the currently configured DNS server. | |
size_t | dns_local_iterate (dns_found_callback iterator_fn, void *iterator_arg) |
Iterate the local host-list for a hostname. | |
err_t | dns_local_lookup (const char *hostname, ip_addr_t *addr, u8_t dns_addrtype) |
Scans the local host-list for a hostname. | |
int | dns_local_removehost (const char *hostname, const ip_addr_t *addr) |
Remove all entries from the local host-list for a specific hostname and/or IP address. | |
err_t | dns_local_addhost (const char *hostname, const ip_addr_t *addr) |
Add a hostname/IP address pair to the local host-list. | |
static err_t | dns_lookup (const char *name, ip_addr_t *addr LWIP_DNS_ADDRTYPE_ARG(u8_t dns_addrtype)) |
Look up a hostname in the array of known hostnames. | |
err_t | dns_gethostbyname (const char *hostname, ip_addr_t *addr, dns_found_callback found, void *callback_arg) |
Resolve a hostname (string) into an IP address. | |
err_t | dns_gethostbyname_addrtype (const char *hostname, ip_addr_t *addr, dns_found_callback found, void *callback_arg, u8_t dns_addrtype) |
Like dns_gethostbyname, but returned address type can be controlled: |
Detailed Description
Implements a DNS host name to IP address resolver.
The lwIP DNS resolver functions are used to lookup a host name and map it to a numerical IP address. It maintains a list of resolved hostnames that can be queried with the dns_lookup() function. New hostnames can be resolved using the dns_query() function.
The lwIP version of the resolver also adds a non-blocking version of gethostbyname() that will work with a raw API application. This function checks for an IP address string first and converts it if it is valid. gethostbyname() then does a dns_lookup() to see if the name is already in the table. If so, the IP is returned. If not, a query is issued and the function returns with a ERR_INPROGRESS status. The app using the dns client must then go into a waiting state.
Once a hostname has been resolved (or found to be non-existent), the resolver code calls a specified callback function (which must be implemented by the module that uses the resolver).
Multicast DNS queries are supported for names ending on ".local". However, only "One-Shot Multicast DNS Queries" are supported (RFC 6762 chapter 5.1), this is not a fully compliant implementation of continuous mDNS querying!
All functions must be called from TCPIP thread.
- See also:
- Common functions for thread-safe access.
Function Documentation
err_t dns_gethostbyname | ( | const char * | hostname, |
ip_addr_t * | addr, | ||
dns_found_callback | found, | ||
void * | callback_arg | ||
) |
Resolve a hostname (string) into an IP address.
NON-BLOCKING callback version for use with raw API!!!
Returns immediately with one of err_t return codes:
- ERR_OK if hostname is a valid IP address string or the host name is already in the local names table.
- ERR_INPROGRESS enqueue a request to be sent to the DNS server for resolution if no errors are present.
- ERR_ARG: dns client not initialized or invalid hostname
- Parameters:
-
hostname the hostname that is to be queried addr pointer to a ip_addr_t where to store the address if it is already cached in the dns_table (only valid if ERR_OK is returned!) found a callback function to be called on success, failure or timeout (only if ERR_INPROGRESS is returned!) callback_arg argument to pass to the callback function
- Returns:
- a err_t return code.
Definition at line 1468 of file lwip_dns.c.
err_t dns_gethostbyname_addrtype | ( | const char * | hostname, |
ip_addr_t * | addr, | ||
dns_found_callback | found, | ||
void * | callback_arg, | ||
u8_t | dns_addrtype | ||
) |
Like dns_gethostbyname, but returned address type can be controlled:
- Parameters:
-
hostname the hostname that is to be queried addr pointer to a ip_addr_t where to store the address if it is already cached in the dns_table (only valid if ERR_OK is returned!) found a callback function to be called on success, failure or timeout (only if ERR_INPROGRESS is returned!) callback_arg argument to pass to the callback function dns_addrtype - LWIP_DNS_ADDRTYPE_IPV4_IPV6: try to resolve IPv4 first, try IPv6 if IPv4 fails only - LWIP_DNS_ADDRTYPE_IPV6_IPV4: try to resolve IPv6 first, try IPv4 if IPv6 fails only
- LWIP_DNS_ADDRTYPE_IPV4: try to resolve IPv4 only
- LWIP_DNS_ADDRTYPE_IPV6: try to resolve IPv6 only
Definition at line 1489 of file lwip_dns.c.
const ip_addr_t* dns_getserver | ( | u8_t | numdns ) |
Obtain one of the currently configured DNS server.
- Parameters:
-
numdns the index of the DNS server
- Returns:
- IP address of the indexed DNS server or "ip_addr_any" if the DNS server has not been configured.
Definition at line 384 of file lwip_dns.c.
Add a hostname/IP address pair to the local host-list.
Duplicates are not checked.
- Parameters:
-
hostname hostname of the new entry addr IP address of the new entry
- Returns:
- ERR_OK if succeeded or ERR_MEM on memory error
Definition at line 565 of file lwip_dns.c.
size_t dns_local_iterate | ( | dns_found_callback | iterator_fn, |
void * | iterator_arg | ||
) |
Iterate the local host-list for a hostname.
- Parameters:
-
iterator_fn a function that is called for every entry in the local host-list iterator_arg 3rd argument passed to iterator_fn
- Returns:
- the number of entries in the local host-list
Definition at line 443 of file lwip_dns.c.
Scans the local host-list for a hostname.
- Parameters:
-
hostname Hostname to look for in the local host-list addr the first IP address for the hostname in the local host-list or IPADDR_NONE if not found. dns_addrtype - LWIP_DNS_ADDRTYPE_IPV4_IPV6: try to resolve IPv4 (ATTENTION: no fallback here!) - LWIP_DNS_ADDRTYPE_IPV6_IPV4: try to resolve IPv6 (ATTENTION: no fallback here!)
- LWIP_DNS_ADDRTYPE_IPV4: try to resolve IPv4 only
- LWIP_DNS_ADDRTYPE_IPV6: try to resolve IPv6 only
- Returns:
- ERR_OK if found, ERR_ARG if not found
Definition at line 480 of file lwip_dns.c.
int dns_local_removehost | ( | const char * | hostname, |
const ip_addr_t * | addr | ||
) |
Remove all entries from the local host-list for a specific hostname and/or IP address.
- Parameters:
-
hostname hostname for which entries shall be removed from the local host-list addr address for which entries shall be removed from the local host-list
- Returns:
- the number of removed entries
Definition at line 529 of file lwip_dns.c.
static err_t dns_lookup | ( | const char * | name, |
ip_addr_t *addr | LWIP_DNS_ADDRTYPE_ARGu8_t dns_addrtype | ||
) | [static] |
Look up a hostname in the array of known hostnames.
- Note:
- This function only looks in the internal array of known hostnames, it does not send out a query for the hostname if none was found. The function dns_enqueue() can be used to send a query for a hostname.
- Parameters:
-
name the hostname to look up addr the hostname's IP address, as u32_t (instead of ip_addr_t to better check for failure: != IPADDR_NONE) or IPADDR_NONE if the hostname was not found in the cached dns_table.
- Returns:
- ERR_OK if found, ERR_ARG if not found
Definition at line 605 of file lwip_dns.c.
void dns_setserver | ( | u8_t | numdns, |
const ip_addr_t * | dnsserver | ||
) |
Initialize one of the DNS servers.
- Parameters:
-
numdns the index of the DNS server to set must be < DNS_MAX_SERVERS dnsserver IP address of the DNS server to set
Definition at line 364 of file lwip_dns.c.
Generated on Sun Jul 17 2022 08:25:37 by
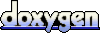